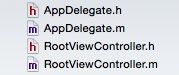
#import "RootViewController.h"
@interface RootViewController ()<UITableViewDataSource, UITableViewDelegate>
@property(nonatomic, retain)UITableView *tableView;
@property(nonatomic, retain)NSMutableArray *arr;
@end
@implementation RootViewController
- (void)dealloc
{
[_arr release];
[_tableView release];
[super dealloc];
}
- (instancetype)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil {
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
[self createData];
}
return self;
}
- (void)createData {
self.arr = [NSMutableArray arrayWithObjects:@"宋江", @"卢俊义", @"吴用", @"公孙胜", @"关胜", @"林冲", @"秦明" ,@"呼延灼" , @"花荣",@"柴进", @"李应", @"朱仝",@"鲁智深",@"武松",nil];
}
- (void)viewDidLoad {
[super viewDidLoad];
self.tableView = [[UITableView alloc] initWithFrame:CGRectMake(0, 0, self.view.frame.size.width, self.view.frame.size.height) style:UITableViewStylePlain];
[self.view addSubview:self.tableView];
[_tableView release];
self.tableView.delegate = self;
self.tableView.dataSource = self;
1.给导航控制器右侧添加编辑按钮.
self.navigationItem.rightBarButtonItem = self.editButtonItem;
}
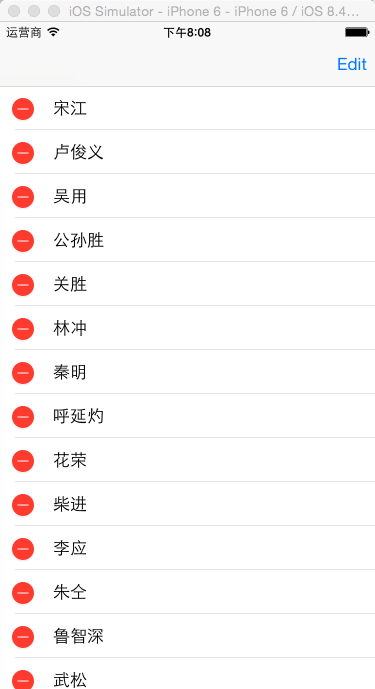
2.重写系统的编辑按钮触发方法,为了使右侧的编辑按钮和编辑模式相关联.
- (void)setEditing:(BOOL)editing animated:(BOOL)animated {
[super setEditing:editing animated:animated];
[self.tableView setEditing:editing animated:animated];
}
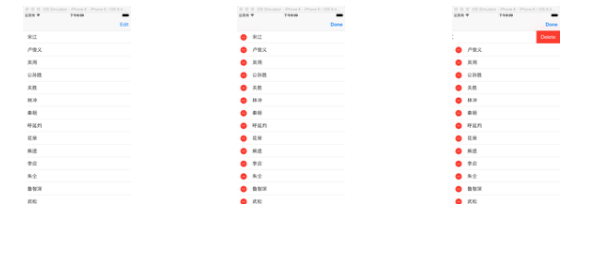
3.设置哪些行可以进行编辑操作.
- (BOOL)tableView:(UITableView *)tableView canEditRowAtIndexPath:(NSIndexPath *)indexPath {
if (indexPath.row % 2 == 0) {
return YES;
} else {
return NO;
}
}
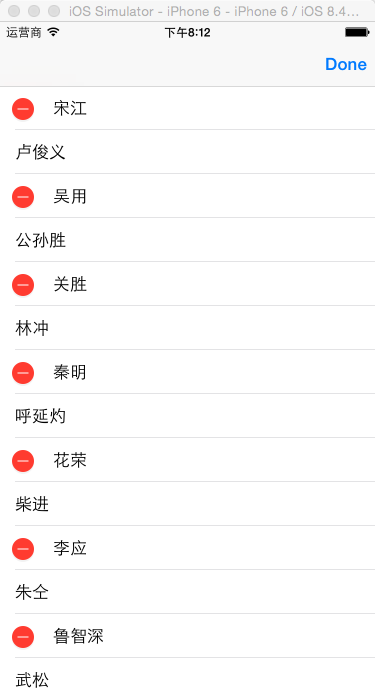
4.修改cell的编辑模式,默认是删除,还可以进行添加等操作.
- (UITableViewCellEditingStyle)tableView:(UITableView *)tableView editingStyleForRowAtIndexPath:(NSIndexPath *)indexPath {
return UITableViewCellEditingStyleInsert;
}
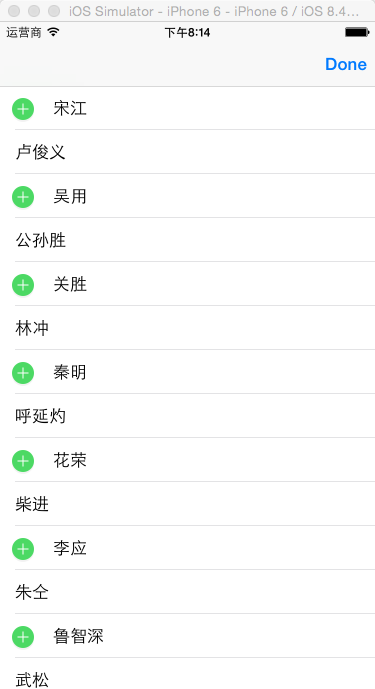
5.实现删除功能,还自带了一个左划显示删除功能的效果.
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath {
if (editingStyle == UITableViewCellEditingStyleDelete) {
[self.arr removeObjectAtIndex:indexPath.row];
[self.tableView deleteRowsAtIndexPaths:@[indexPath] withRowAnimation:UITableViewRowAnimationLeft];
}
}
6.在默认情况下,修改delete按钮的标题.
- (NSString *)tableView:(UITableView *)tableView titleForDeleteConfirmationButtonForRowAtIndexPath:(NSIndexPath *)indexPath {
return @"哈哈";
}
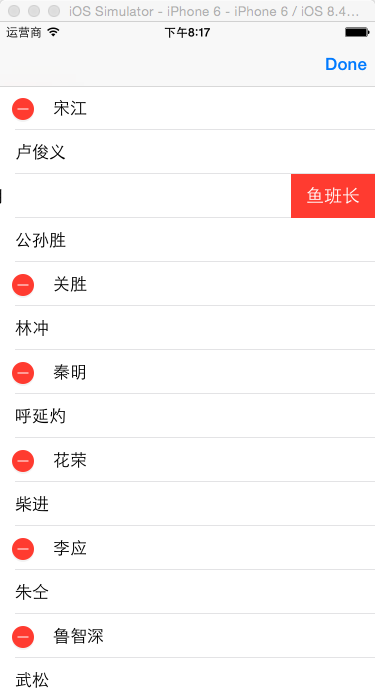
7.在delete按钮位置添加多个按钮,如果执行此方法,那么修改delete按钮的标题的方法就不好使了.
- (NSArray *)tableView:(UITableView *)tableView editActionsForRowAtIndexPath:(NSIndexPath *)indexPath {
UITableViewRowAction *firstAction = [UITableViewRowAction rowActionWithStyle:UITableViewRowActionStyleDefault title:@"添加" handler:^(UITableViewRowAction *action, NSIndexPath *indexPath) {
NSLog(@"测试一下");
}];
firstAction.backgroundColor = [UIColor greenColor];
UITableViewRowAction *secondAction = [UITableViewRowAction rowActionWithStyle:UITableViewRowActionStyleDefault title:@"通话" handler:^(UITableViewRowAction *action, NSIndexPath *indexPath) {
NSLog(@"hhhhh");
}];
return @[firstAction, secondAction];
}
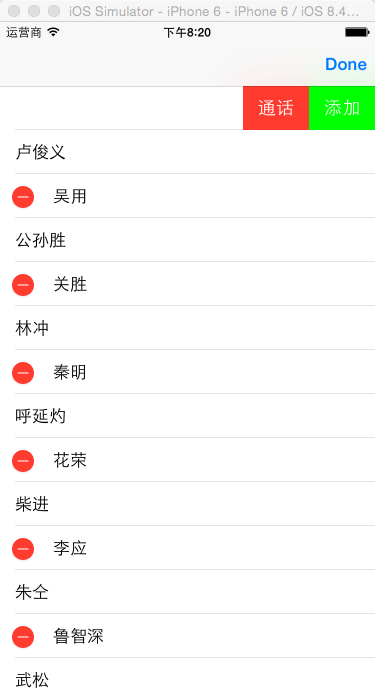
8.移动
- (void)tableView:(UITableView *)tableView moveRowAtIndexPath:(NSIndexPath *)sourceIndexPath toIndexPath:(NSIndexPath *)destinationIndexPath {
NSString *str = [self.arr[sourceIndexPath.row] retain];
[self.arr removeObjectAtIndex:sourceIndexPath.row];
[self.arr insertObject:str atIndex:destinationIndexPath.row];
[str release];
}
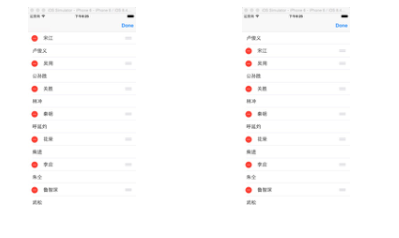
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
return self.arr.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
static NSString *reuse = @"reuse";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:reuse];
if (!cell) {
cell = [[[UITableViewCell alloc] initWithStyle:UITableViewCellStyleValue1 reuseIdentifier:reuse] autorelease];
}
cell.textLabel.text = self.arr[indexPath.row];
return cell;
}
9.单行修改数据,并刷新
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath {
[self.arr replaceObjectAtIndex:indexPath.row withObject:@"刘超"];
[tableView reloadRowsAtIndexPaths:@[indexPath] withRowAnimation:UITableViewRowAnimationLeft];
}
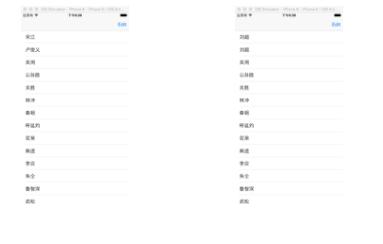