"""Coordinator to help multiple threads stop when requested."""
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
import contextlib
import sys
import threading
import time
import six
from tensorflow.python.framework import errors
from tensorflow.python.platform import tf_logging as logging
from tensorflow.python.util import compat
class Coordinator(object):
"""A coordinator for threads.
线程协调器
This class implements a simple mechanism to coordinate the termination of a
set of threads.
这个类实现了一个简单的机制来协调一组线程。
#### Usage:
```python
# Create a coordinator. 创建一个协调器。
coord = Coordinator()
# Start a number of threads, passing the coordinator to each of them.
# 启动一些线程,将协调器传递给每个线程。
...start thread 1...(coord, ...)
...start thread N...(coord, ...)
# Wait for all the threads to terminate. 等待所有线程终止。
coord.join(threads)
```
Any of the threads can call `coord.request_stop()` to ask for all the threads to stop.
To cooperate with the requests, each thread must check for `coord.should_stop()` on a regular basis.
`coord.should_stop()` returns `True` as soon as `coord.request_stop()` has been called.
任何线程可以调用 `coord.request_stop()` 来要求所有线程停止。
为了配合请求,每个线程必须定期检查`coord.should_stop()`。
`coord.should_stop()` 一旦返回`True`, `coord.request_stop()`就会马上被调用。
A typical thread running with a coordinator will do something like:
与协调器运行的典型线程将执行以下操作:
```python
while not coord.should_stop():
...do some work...
```
#### Exception handling:
#### 异常处理:
A thread can report an exception to the coordinator as part of the `should_stop()` call.
The exception will be re-raised from the `coord.join()` call.
线程可以向协调器报告异常,作为 `should_stop()` 调用的一部分。
将从 `coord.join()` 调用中重新提出异常。
Thread code:
```python
try:
while not coord.should_stop():
...do some work...
except Exception as e:
coord.request_stop(e)
```
Main code:
```python
try:
...
coord = Coordinator()
# Start a number of threads, passing the coordinator to each of them.
...start thread 1...(coord, ...)
...start thread N...(coord, ...)
# Wait for all the threads to terminate.
coord.join(threads)
except Exception as e:
...exception that was passed to coord.request_stop()
```
To simplify the thread implementation, the Coordinator provides a context
handler `stop_on_exception()` that automatically requests a stop if
an exception is raised. Using the context handler the thread code
above can be written as:
为了简化线程实现,协调器提供了一个上下文处理程序 `stop_on_exception()` ,它会自动请求停止如果
提出异常。使用上下文处理程序,上面的线程代码可以写成:
```python
with coord.stop_on_exception():
while not coord.should_stop():
...do some work...
```
#### Grace period for stopping:
#### 宽限期
After a thread has called `coord.request_stop()` the other threads have a fixed time to stop,
this is called the 'stop grace period' and defaults to 2 minutes.
If any of the threads is still alive after the grace period expires `coord.join()`
raises a RuntimeException reporting the laggards.
一个线程调用 `coord.request_stop()` 后,其他线程有一个固定的时间停止,这被称为 '停止宽限期' ,默认为2分钟。
如果任何线程在宽限期到期后仍然存在,那么 `coord.join()` 引发一个 RuntimeException 来报告未停止的线程。
```python
try:
...
coord = Coordinator()
# Start a number of threads, passing the coordinator to each of them.
...start thread 1...(coord, ...)
...start thread N...(coord, ...)
# Wait for all the threads to terminate, give them 10s grace period
coord.join(threads, stop_grace_period_secs=10)
except RuntimeException:
...one of the threads took more than 10s to stop after request_stop()
...was called.
except Exception:
...exception that was passed to coord.request_stop()
```
"""
def __init__(self, clean_stop_exception_types=None):
"""Create a new Coo
coordinator.py
最新推荐文章于 2021-03-11 11:04:34 发布
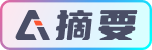