知识点目录
知识点回顾
8.1 将程序运行到手机上
前面的章节我们都是使用模拟器来运行程序的,但这章的Demo都需要在真正的Android手机上运行。
打开开发者选项:
从Android4.2系统开始,开发者选项是默认隐藏的,需要先进入到“关于手机”界面,然后对连续点击版本号,直到让开发者选项显示出来。
手机连接电脑
打开开发者选项后,我们还需要通过数据线把手机连接到电脑上。然后进入到设置—>开发者选项界面,并在这个界面中勾选中USB调试选项。
如果是Windows操作系统,还需要在电脑上安装手机驱动。一般借助360手机助手或豌豆荚等工具进行快速安装,安装完成后就可以看到手机已经连接到电脑上了:
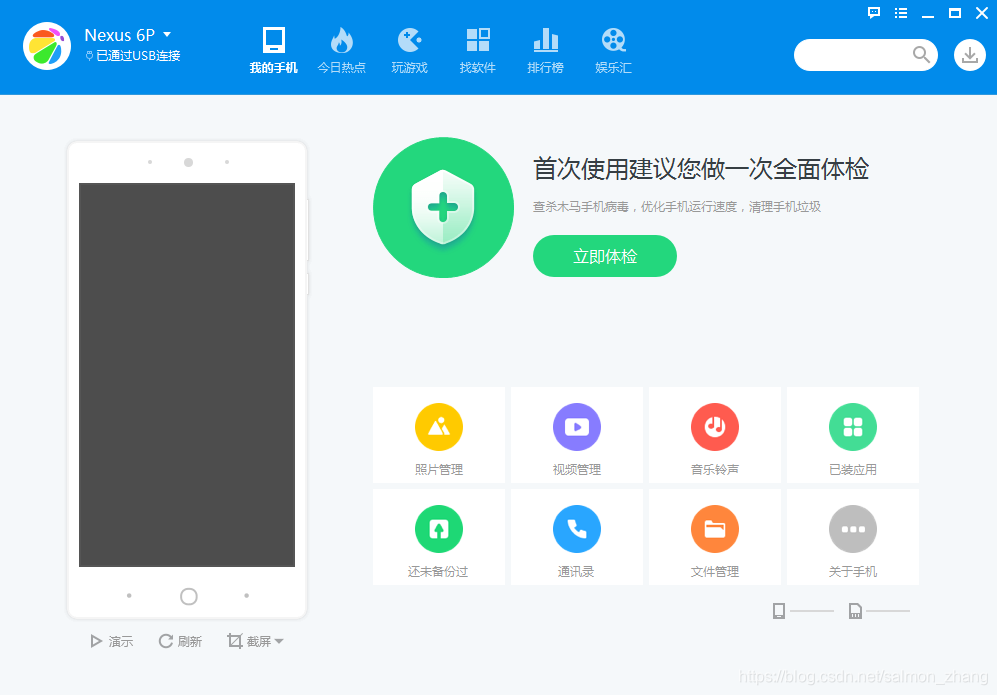
8.2 使用通知
通知的主要的作用是:当应用程序不是在前台运行时,向用户发出一些提示信息。
发出一条通知后,手机最上方的状态栏会显示一个通知的图标,下拉状态栏后可以看到通知的详细内容。
8.2.1 通知的基本使用
通知可以在活动、广播接收器或服务里创建。但无论在哪创建使用步骤都是一样的。
-
获取NotificationManager来对通知进行管理
NotificationManager manager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
-
创建Notification对象
Notification notification = new NotificationCompat.Builder(this) .setContentTitle("This is content title") .setContentText("This is content text") .setWhen(System.currentTimeMillis()) .setSmallIcon(R.mipmap.ic_launcher) .setLargeIcon(BitmapFactory.decodeResource(getResources(), R.mipmap.ic_launcher)) .build();
几乎每个Android版本都会对通知这部分进行修改,为了更好的兼容性,我们使用support库中提供的兼容API。support-v4库中提供了一个NotificationCompat类,使用这个类的构造器来创建Notification对象,就可以保证我们的程序在所有Android系统版本上都能正常工作。
-
setContentTitle()方法指定通知的标题内容
-
setContentText()方法指定通知的正文内容
-
setWhen()方法指定通知被创建的时间,以毫秒为单位,当下拉系统状态栏时,这里指定的时间会显示在相应的通知上
-
setSmallIcon()方法设置通知的小图标,只能使用纯alpha图层的图片进行设置,小图标会显示在系统状态栏上
-
setLargeIcon()方法设置通知的大图标,下拉系统状态栏时,就可以看到设置的大图标
-
调用NotificationManager的notify()方法
manager.notify(1,notification);
参数一:id,要保证为每个通知所指定的id都是不同的
参数二:创建的Notification对象
示例代码:
-
定义一个notification触发点
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:id="@+id/send_notice" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAllCaps="false" android:text="Send notice"/> </LinearLayout>
-
创建通知
public class MainActivity extends AppCompatActivity implements View.OnClickListener { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Button sendNotice = (Button) findViewById(R.id.send_notice); sendNotice.setOnClickListener(this); } @Override public void onClick(View v) { switch (v.getId()) { case R.id.send_notice: String id = "my_channel_01"; String name = "我是渠道名字"; NotificationManager manager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE); Notification notification = null; if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {//Android 8.0以上 Toast.makeText(this, "Build.VERSION.SDK_INT = " + Build.VERSION.SDK_INT, Toast.LENGTH_SHORT).show(); NotificationChannel channel = new NotificationChannel(id, name, NotificationManager.IMPORTANCE_DEFAULT); manager.createNotificationChannel(channel); notification = new NotificationCompat.Builder(this, id)//这里要传一个id进去 .setContentTitle("This is content title") .setContentText("heheda") .setWhen(System.currentTimeMillis()) .setSmallIcon(R.mipmap.ic_launcher) .setLargeIcon(BitmapFactory.decodeResource(getResources(), R.mipmap.ic_launcher)) .build(); } else {//Android8.0以下 Toast.makeText(this, "Build.VERSION.SDK_INT = " + Build.VERSION.SDK_INT, Toast.LENGTH_SHORT).show(); notification = new NotificationCompat.Builder(this) .setContentTitle("This is content title") .setContentText("hahaha") .setWhen(System.currentTimeMillis()) .setSmallIcon(R.mipmap.ic_launcher) .setLargeIcon(BitmapFactory.decodeResource(getResources(), R.mipmap.ic_launcher)) .build(); } manager.notify(1, notification); break; default: break; } } }
备注:在Android8.0以上发送通知的稍有不同,需要NotificationChannel。
PendingIntent
PendingIntent跟Intent有许多相似之处,都可以指明一个"意图",都可以启动活动、启动服务以及发送广播。不同的是Intent更加倾向于去立即执行某个动作,而PendingIntent更加倾向于在某个合适的时机去执行某个动作。所以,可以把PendingIntent理解为延迟执行的Intent
PendingIntent的基本用法:
- 获取PendingIntent实例
根据具体的需求来选择使用getActivity()方法、getBroadcast()方法或getService()方法。
Intent intent = new Intent(this, NotificationActivity.class);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intent, 0);
getActivity()方法、getBroadcast()方法或getService()方法接收的参数都是相同的:
参数一:上下文
参数二:请求码,一般传入0即可
参数三:Intent对象
参数四:用于确定PendingIntent的行为。有FLAG_ONE_SHOT、FLAG_NO_CREATE、FLAG_CANCEL_CURRENT和FLAG_UPDATE_CURRENT这4个值可选,通常传入0即可。
2.连缀在setContentIntent()方法中
notification = new NotificationCompat.Builder(this, id)//这里要传一个id进去
.setContentTitle("This is content title")
.setContentText("heheda")
.setWhen(System.currentTimeMillis())
.setSmallIcon(R.mipmap.ic