引言
- 针对环形文字识别,通过这几天调研,一般有两种方法,一是极坐标转换;二是薄板样条插值(TPS)法。
极坐标转换
方案一:
import numpy as np
def circle_to_rectangle(cir_img, start=0):
"""
对圆和椭圆的旋转文字进行极坐标到直角坐标转换
默认从正下方开始切,可以通过start设定偏移角度,单位为度
"""
h, w = cir_img.shape[:2]
x0, y0 = h // 2, w // 2
radius = (x0 + y0) // 2
rect_height = radius
rect_width = int(2 * np.pi * radius)
rect_img = np.zeros((rect_height, rect_width, 3), dtype="u1")
index_w = np.array(range(rect_width))
index_h = np.array(range(rect_height))
theta_array = 2 * np.pi * (index_w / rect_width + start / 360)
for j, theta in zip(index_w, theta_array):
x = x0 + (x0 - index_h) * np.cos(theta)
y = y0 + (y0 - index_h) * np.sin(theta)
x = np.where(x >= h, h - 1, x).astype(np.int16)
y = np.where(y >= w, w - 1, y).astype(np.int16)
rect_img[index_h, j, :] = cir_img[x, y, :]
rect_img = np.fliplr(rect_img)
return rect_img
方案二:
- 代码出处:cv-warpPolar-example
image = cv.imread('image.jpg')
h, w = image.shape[:2]
center = (round(w / 2), round( h / 2))
flags = cv.INTER_CUBIC + cv.WARP_FILL_OUTLIERS + cv.WARP_POLAR_LINEAR
radius = center[0]
img_res = cv.warpPolar(image, (300, 600), center, radius, flags)
img_res = img_res.transpose(1,0,2)[::-1]
img_res = cv2.cvtColor(img_res, cv2.COLOR_BGR2RGB)
plt.imshow(img_res)
plt.show()
- 示例图(
image.jpg
):
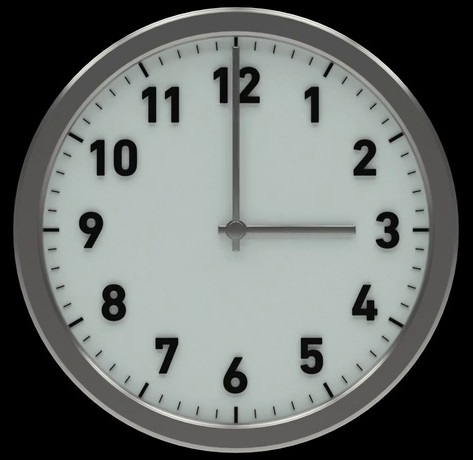
- 结果图
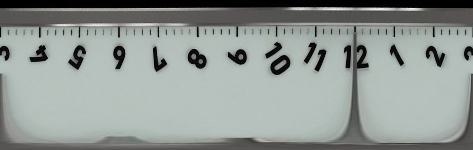
薄板样条插值法
- 代码示例:(出处:暂时找不到了,作者如果看到,请联系我)
import cv2
import numpy as np
def tps_cv2(source, img):
"""
source: list [(x0, y0), (x1, y1), (x2, y2), ...]
"""
if isinstance(source, list):
source = np.array(source)
tps = cv2.createThinPlateSplineShapeTransformer()
source_cv2 = source.reshape(1, -1, 2)
matches = list()
for i in range(len(source_cv2[0])):
matches.append(cv2.DMatch(i, i, 0))
new_points = []
N = len(source)
N = N // 2
h, w = img.shape[:2]
dx = int(w / (N - 1))
for i in range(N):
new_points.append((dx * i, 2))
for i in range(N - 1, -1, -1):
new_points.append((dx * i, h - 2))
target_cv2 = np.array(new_points, np.int32)
target_cv2 = target_cv2.reshape(1, -1, 2)
tps.estimateTransformation(target_cv2, source_cv2, matches)
new_img_cv2 = tps.warpImage(img)
return new_img_cv2
source = [[217, 135], [302, 89], [419, 56], [589, 58], [716, 88],
[815, 131], [789, 180], [697, 144], [578, 116], [426, 116],
[320, 149], [245, 183]]
img = cv2.imread('tps_img.jpeg')
new_img = tps_cv2(source, img)
cv2.imwrite('res_tps_img.jpeg', new_img)
- 示例图(
tps_img.jpeg
)
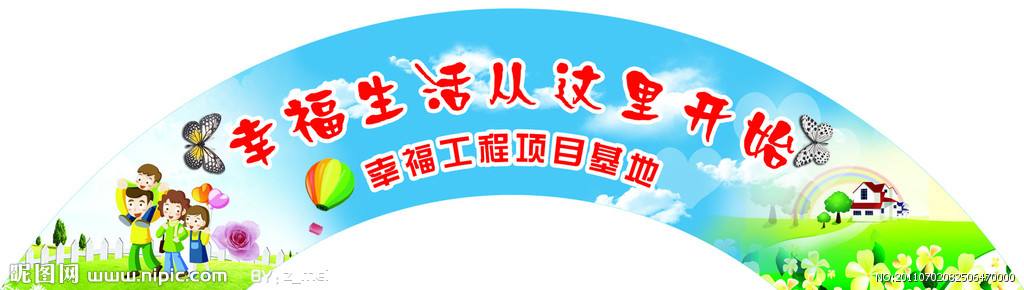
- 结果图(
res_tps_img.jpeg
)
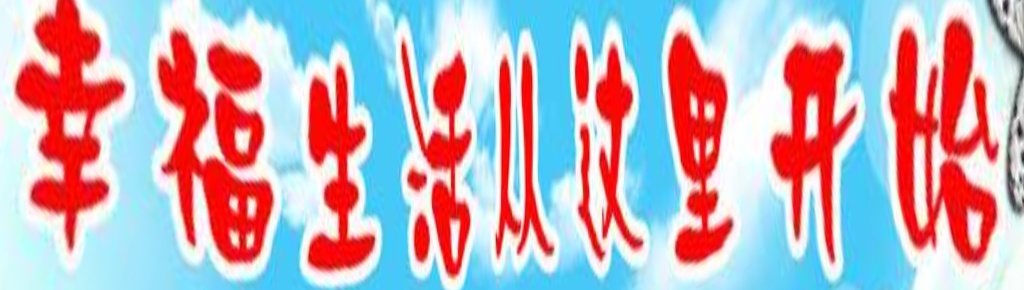