开发一个应用,基本会有很多个页面,就像我们开发Android原生应用的时候,多个Activity 多个Fragment之间跳转 ,Rn中是怎么跳转的呢?答案就是通过导航器Navigator。
例子代码:
/**
* Sample React Native App
* https://github.com/facebook/react-native
* @flow
*/
import React, { Component } from 'react';
import {
AppRegistry,
StyleSheet,
Text,
View,
TouchableHighlight,
Image,
Navigator
} from 'react-native';
class RnWidget extends Component {
render() {
let defaultName = 'firstPageName';
let defaultComponent = FirstPageComponent;
return (
<Navigator
styles = {styles.container}
initialRoute = {{name: defaultName,component : defaultComponent}}
configureScene = {
(route)=>{
return Navigator.SceneConfigs.FloatFromRight
}
}
renderScene = {(route,navigator)=>{
let Component = route.component;
return <Component{...route.params} navigator={navigator}/>
}}
/>
)
}
}
class FirstPageComponent extends Component{
clickJump(){
const{navigator} = this.props;
if(navigator){
navigator.push({
name : "SecondPageComponent",
component : SecondPageComponent
})
}
}
render(){
return(
<View style={styles.container}>
<Text>我是第一个界面</Text>
<TouchableHighlight
underlayColor="rgb(181, 136, 254)"
activeOpacity={0.5}
style={{ borderRadius: 8,padding: 8,marginTop:5,backgroundColor:"#0588fe"}}
onPress={this.clickJump.bind(this)}
>
<Text>点击进入第二个界面</Text>
</TouchableHighlight>
</View>
)
}
}
class SecondPageComponent extends Component{
clickJump(){
const{navigator} = this.props;
if(navigator){
navigator.pop();
}
}
render(){
return(
<View style={styles.container}>
<Text>我是第二个界面</Text>
<TouchableHighlight
underlayColor="rgb(181, 136, 254)"
activeOpacity={0.5}
style={{ borderRadius: 8,padding: 8,marginTop:5,backgroundColor:"#0588fe"}}
onPress={this.clickJump.bind(this)}
>
<Text>点击返回第一个界面</Text>
</TouchableHighlight>
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#ffffff',
},
});
AppRegistry.registerComponent('RnWidget', () => RnWidget);
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 90
- 91
- 92
- 93
- 94
- 95
- 96
- 97
- 98
- 99
- 100
- 101
- 102
- 103
- 104
- 105
- 106
- 107
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 90
- 91
- 92
- 93
- 94
- 95
- 96
- 97
- 98
- 99
- 100
- 101
- 102
- 103
- 104
- 105
- 106
- 107
首先要有一个程序的主入口:
class RnWidget extends Component
然后我们要有两个界面 第一个界面和第二个界面
class FirstPageComponent extends Component
class SecondPageComponent extends Component
(1)在程序的主入口里面初始化我们的导航器Navigator
initialRoute={{ name: defaultName, component: defaultComponent }}
这个指定了默认的页面,也就是启动app之后会看到界面的第一屏。 需要填写两个参数: name 跟 component。(注意这里填什么参数纯粹是自定义的,因为这个参数也是你自己发自己收,自己在renderScene方法中处理。我们这里示例用了两个参数,但其实真正使用的参数只有component)
configureScene={() => {
return Navigator.SceneConfigs.VerticalDownSwipeJump;
}} 这个是页面之间跳转时候的动画,具体有哪些?可查看下文档或者有源代码的: node_modules/React-native/Libraries/CustomComponents/Navigator/NavigatorSceneConfigs.js
renderScene={(route, navigator) => {
let Component = route.component;
return <Component {...route.params} navigator={navigator} />
}} />
);
这里是每个人最疑惑的,我们先看到回调里的两个参数:route, navigator。通过打印我们发现route里其实就是我们传递的name,component这两个货,navigator是一个Navigator的对象,为什么呢,因为它有push pop jump…等方法,这是我们等下用来跳转页面用的那个navigator对象。
(2) 在第一个界面和第二个界面里面分别有一个push的方法和pop的方法。
navigator.push 传入name和你想要跳的组件页面
navigator.pop(); 把当前页面pop掉
效果:
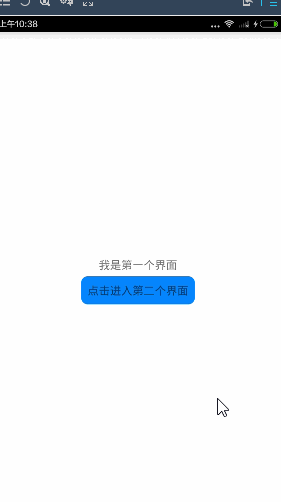