1. class
1.1 toString()和equals()
public class People{
private int age;
private String name;
public People(int age, String name){
this.age = age;
this.name = name;
}
public String toString(){
return name + " " + age;
}
public boolean equals(People anotherPeople){
return anotherPeople.age == this.age && anotherPeople.name = this.name;
}
}
public class Main{
pblic static void main(String[] args){
People p = new People(25,"Skylar");
System.out.println(p);
People p2 = new People(25, "Skylar");
System.out.println(p.equals(p2));
}
}
1.2 overload-constructor
- default
- 在没有其他任何constructor的情况下会自动补充。【建议都手动写】
- 有其他constructor fun就不会自动补充,会报错
public class People{
private int age;
private String name;
public People(){
}
}
public class Main{
pblic static void main(String[] args){
People p = new People();
System.out.println(p);
}
}
- 普通的
- 传入的variables来自instance variables。
- copy- constructor
- 把一个object的值,copy给另一个object。
public class People{
private int age;
private String name;
public People(People anotherPeople){
this.age = anotherPeople.age;
this.name = anotherPeople.name;
}
}
public class Main{
pblic static void main(String[] args){
People p = new People(25, "Skylar");
People p2 = p;
People p3 = new People(p);
}
}
1.3 static
- 当一个东西,属于整个class,就是static
- static method和static variable
- static variable:初始化过程跟instance variable一样。
- static method:建议调用时使用class name。
public class People{
private int age;
private String name;
private static int number = 0;
public People(int age, String name){
this.age = age;
this.name = name;
number++;
}
public People(People anotherPeople){
this.age = anotherPeople.age;
this.name = anotherPeople.name;
number++;
}
public People(){
number++;
}
public String toString(){
return name + " " + age;
}
public boolean equals(People anotherPeople){
return anotherPeople.age == this.age && anotherPeople.name = this.name;
}
public static void printClassInfo(){
System.out.println("Current number: " + number);
}
}
public class Main{
pblic static void main(String[] args){
People p = new People(25,"Skylar");
People.printClassInfo();
System.out.println(p);
People p2 = new People(25, "Skylar");
People.printClassInfo();
System.out.println(p.equals(p2));
}
}
2. Wrapper
- 包装类:为什么?怎么样?
- class的使用很灵活,基础数据类型不灵活
- boxing:将基础数据类型 = > wrapper class 的过程。
- unboxing: wrapper class => 基础数据类型 的过程。
【byte, short, int, long, float, double, char, boolean】
【Byte, Short, Integer, Float, Double, Character, Boolean】 - 注意⚠️:当使用Boolean时,要认为手动的去判断用户输入的是flase还是其他信息。然后再判断true/false。
- Character不能使用parse。【String是character的集合啦】
- substring(起, 终),不包含终。
- String => char:
- toUpperCase():转换成大写
- isUpperCase():回答是否为大写
- toLowerCase():转换成小写
- isLowerCase(): 回答是否为小写
- isWhitespace():回答是否有空格【3种:普通空格、\t 、\n】
- isLetter(): 回答是否是字母
- isDigit():回答是否是数字
- isLetterOrDigit():回答是否是字母 / 数字
public class Main{
public static void main(String[] args){
Integer.parseInt("10");
System.out.println(Boolean.parseBoolean("True"));
System.out.println(Boolean.parseBoolean("TRue"));
System.out.println(Boolean.parseBoolean("False"));
System.out.println(Boolean.parseBoolean("sfgdfg"));
String s = "helloworld!";
char x = s.charAt(5);/获取index有5时的内容。
Scanner sc = new Scanner(System.in);
String id = sc.nextLine();
String password = sc.nextLine();
boolean allNum = true;
for(int i = 0; i < id.length; i++){
if(!Character.isDigit(id.charAt(i))){
allNum = false;
}
}
String results = allNum ? "id合法": "id非法";
System.out.println(results);
boolean hasNum = false;
boolean hasUpper = false;
boolean hasLower = false;
for(int i = 0; i < password.length; i++){
if(Character.isUpperCase(password.charAt(i))){
hasUpper = true;
}else if(Character.isLowerCase(password.charAt(i))){
hasLower = true;
}else if(Character.isDigit(password.charAt(i))){
hasNum = true;
}
}
String results = hasNum && hasUpper && hasLower ? "密码合法": "密码不合法";
}
}
3. Maths
- java.lang: String, Wrapper, Math
- 绝大多数都是static
- abs、max、min
- pow(底数,指数)
- ceil、floor、sqrt
4. array
- array拷贝思路:
- 创建一个新的array
- copy旧array的内容
- 再将想添加的内容填入新的array
- 最后,将新array的地址赋给旧的人、array。
- 数组的另一种for loop:
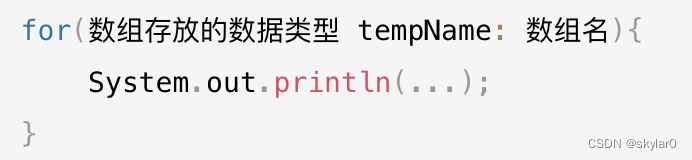
public class Main{
public static void main(String[] args){
People[] peoples = new People[10];
for(People p: peoples){
System.out.println(p);
}
}
}
5. arrayList
- 数组的局限性:长度固定。
=>. 想要一个像数组一样,能把相同数据类型放一起的,但长度可以自动修改的。=> array list.
- arraylist排序非常方便!
- arraylist会自动翻倍/缩放。【当位子不够了,原本4位,它会自动扩展到8位。删除同理缩放一倍】
- 缺点:访问速度比array慢一点。arraylist里的数据类型只能是class,无法存储基础数据类型。
import java.util.ArrayList;
public class Main{
public static void main(String[] args){
ArrayList<baseType> watches = new ArrayList<baseType>(初识容量大小);
ArrayList<String> watches = new ArrayList<>();
watches.add("AP");
watches.add("PP");
watches.add("Constantin");
System.out.println(watches);
System.out.println(watches.get(0));
watches.set(0, "Omega");
watches.remove(2);
watches.size();
Collections.sort(watches);
}
}