import numpy as np import pandas as pd # 必须是偶数 用3×3或者5×5去卷积 # 不够偶数的用0去填满 # 卷积层 输出层有w b 池化层没有 # 卷积层为3×3=9,9+1=10,增加1个偏置 # 输出层和卷积层有关系,以3×3为例,卷积层为原来的偶数(n-2)**2 # 池化层则为(n-2)**2/4 # 输出层w为结果池化层的结果,b为偏置 # 输出有几个,输出w,b有几组 # 卷积网络先要确定数据维度,必须为n×n的形式,在确定用3×3卷积,有几个卷积 # X的shape[0]=shape[1]记为n,卷积层数量记为jjs,输出结果记为jgs def sigmoid(s): """激活函数 """ if s >= 0: return 1 / (1 + np.exp(-s)) else: return np.exp(s) / (1 + np.exp(s)) def np_sigmoid(nps): vfunc = np.vectorize(sigmoid) return vfunc(nps) def getxy(): datax_pd = pd.read_excel('cnnbp.xlsx') x_xh = 11 x_xhl = [] for i in range(96): x_xhl.append(x_xh) x_xh = x_xh + 6 x_d = [] x_x1 = 1 x_x = [] y_x = [] for label, value in datax_pd.items(): if x_x1 == 6: for j in range(3, 9): x_x.append(value[j]) x_x1 = 1 if x_x1 == 5: for j in range(3, 9): x_x.append(value[j]) x_x1 = 6 if x_x1 == 4: for j in range(3, 9): x_x.append(value[j]) x_x1 = 5 if x_x1 == 3: for j in range(3, 9): x_x.append(value[j]) x_x1 = 4 if x_x1 == 2: for j in range(3, 9): x_x.append(value[j]) x_x1 = 3 for x_xh in x_xhl: if x_x1 == 1 and label.endswith(str(x_xh)): for j in range(3, 9): x_x.append(value[j]) y_x.append([value[9], value[10], value[11]]) x_x1 = 2 break if len(x_x) > 37: pass if len(x_x) == 36: x_d.append(x_x) x_x = [] x_d_zl = [] for x_d1 in x_d: x_d_xl = [] for i in range(6): for j in range(6): x_d_xl.append(x_d1[i + j * 6]) x_d_zl.append(x_d_xl) datax = np.asarray(x_d_zl) datay = np.asarray(y_x) return datax, datay def netarg(): bparg = pd.read_excel('bparg.xlsx') jjcwb1 = [] jjcwb2 = [] sccwb1 = [] for index, row in bparg.iterrows(): if index <= 8: jjcwb1.append(row[1]) jjcwb1.append(row[2]) jjcwb1.append(row[3]) for index, row in bparg.iterrows(): if index == 9: jjcwb2.append(row[1]) jjcwb2.append(row[2]) jjcwb2.append(row[3]) jjcwb = [] for i1 in range(3): jjcwb.append([[jjcwb1[9*i1], jjcwb1[9*i1+1], jjcwb1[9*i1+2], jjcwb1[9*i1+3], jjcwb1[9*i1+4], jjcwb1[9*i1+5], jjcwb1[9*i1+6], jjcwb1[9*i1+7], jjcwb1[9*i1+8]], jjcwb2[i1]]) for index, row in bparg.iterrows(): if 27 >= index >= 10: sccwb1.append(row[1]) sccwb1.append(row[2]) sccwb2 = [] for index, row in bparg.iterrows(): if index == 28: sccwb2.append(row[1]) sccwb2.append(row[2]) sccwb2.append(row[3]) sccwb = [] for i1 in range(3): sccwb.append([[sccwb1[12 * i1], sccwb1[12 * i1 + 1], sccwb1[12 * i1 + 2], sccwb1[12 * i1 + 3], sccwb1[12 * i1 + 4], sccwb1[12 * i1 + 5], sccwb1[12 * i1 + 6], sccwb1[12 * i1 + 7], sccwb1[12 * i1 + 8], sccwb1[12 * i1 + 9], sccwb1[12 * i1 + 10], sccwb1[12 * i1 + 11]], sccwb2[i1]]) jjc_wbqjnp1 = np.asarray(jjcwb, dtype=object) scc_wbqjnp1 = np.asarray(sccwb, dtype=object) return jjc_wbqjnp1, scc_wbqjnp1 def dancijisuan(jjc_wbqj1, scc_wbqj1, datax, datay): jjc_wbqjnpdc = np.asarray(jjc_wbqj1, dtype=object) scc_wbqjnpdc = np.asarray(scc_wbqj1, dtype=object) jisuany = [] wc = [] jjcad = [] jjcad1 = [] sscqa = [] for i in range(datax.shape[0]): outc = [] jjcax = [] jjcax1 = [] for j in range(len(jjc_wbqjnpdc)): jjc_sr = datax[i].reshape(6, 6) jjc_z = [] for i0 in range(jjc_sr.shape[0] - 2): for j0 in range(jjc_sr.shape[1] - 2): x_1 = np.asarray([jjc_sr[i0, j0], jjc_sr[i0, j0 + 1], jjc_sr[i0, j0 + 2]]) x_2 = np.asarray([jjc_sr[i0 + 1, j0], jjc_sr[i0 + 1, j0 + 1], jjc_sr[i0 + 1, j0 + 2]]) x_3 = np.asarray([jjc_sr[i0 + 2, j0], jjc_sr[i0 + 2, j0 + 1], jjc_sr[i0 + 2, j0 + 2]]) xij = np.asarray([x_1, x_2, x_3]) jjc_z.append(xij) jjc_z = np.asarray(jjc_z) b = jjc_wbqjnpdc[j][1] a = [] for i1 in range(jjc_z.shape[0]): w_f11 = np.asarray(jjc_wbqjnpdc[j][0]) w_f12 = w_f11.flatten() w_f1 = w_f12.reshape(3, 3) a1 = np.sum(w_f1 * jjc_z[i1]) + b a.append(a1) a = np.asarray(a).reshape(4, 4) a = np_sigmoid(a) ax = a.ravel() * (1 - a.ravel()) jjcax.append(ax) jjcax1.append(a) p = [] for i2 in range(a.shape[0]): for j2 in range(a.shape[1]): if i2 % 2 == 0 and j2 % 2 == 0: p_1 = np.asarray([a[i2, j2], a[i2, j2 + 1]]) p_2 = np.asarray([a[i2 + 1, j2], a[i2 + 1, j2 + 1]]) pij = np.max([p_1, p_2]) p.append(pij) outc.append(p) outc = np.asarray(outc).ravel() sscqa.append(outc) outr = [] for outi in range(len(scc_wbqjnpdc)): sccw = scc_wbqjnpdc[outi][0] jjcdxx = [] jjcdxx.append([[sccw[0],sccw[0],sccw[0],sccw[0]], [sccw[1],sccw[1],sccw[1],sccw[1]], [sccw[2],sccw[2],sccw[2],sccw[2]], [sccw[3],sccw[3],sccw[3],sccw[3]]]) sccb = scc_wbqjnpdc[outi][1] sccr = np.sum(sccw * outc) + sccb outr.append(sccr) outr = np_sigmoid(outr) jisuany.append(outr) datay_c1 = np.sum((outr - datay[i])**2*0.5) datay_c2 = datay_c1 ** 2 * 0.5 wc.append(datay_c1) jjcad.append(jjcax) jjcad1.append(jjcax1) jisuany = np.asarray(jisuany) deltao1 = jisuany - datay deltao2 = jisuany deltao3 = 1 - jisuany deltao4 = deltao1 * deltao2 * deltao3 delta_o = deltao4 # 计算deltaf F1 11 12 21 22 对应 w11 jjcadjisuan = np.asarray(jjcad) jjcadjisuan1 = np.asarray(jjcad1) sccwhz = [] sccw1, sccw2, sccw3 = scc_wbqjnpdc[0][0], scc_wbqjnpdc[1][0], scc_wbqjnpdc[2][0] sccwx1 = [] for outi1 in range(len(sccw1)): sccwx = [sccw1[outi1], sccw2[outi1], sccw3[outi1]] sccwx1.append(sccwx) if (outi1+1) % 4 == 0: sccwhz.append(sccwx1) sccwx1 = [] sccwhznp = np.asarray(sccwhz) dwp = [] for i1 in range(datax.shape[0]): dp = [] d1 = delta_o[i1] for outi in range(len(scc_wbqjnpdc)): d31 = [] sccwnp = sccwhznp[outi] for sid in range(sccwnp.shape[0]): d2 = d1 * sccwnp[sid] d21 = np.sum(d2) for jd in range(2): d31.append(d21) d32 = np.asarray(d31).reshape(2, 4) d33 = [] for sid1 in range(d32.shape[0]): d33.append(d32[sid1]) d33.append(d32[sid1]) # -- d4 = jjcadjisuan[i1][outi] d5 = jjcadjisuan1[i1][outi] a1 = np.asarray(d5).reshape(4, 4) a2 = np.asarray(d4).reshape(4, 4) a3 = np.asarray(d33) jcp = [] for i2_jc in range(a1.shape[0]): for j2_jc in range(a1.shape[1]): if i2_jc % 2 == 0 and j2_jc % 2 == 0: p_1 = np.asarray([a1[i2_jc, j2_jc], a1[i2_jc, j2_jc + 1]]) p_2 = np.asarray([a1[i2_jc + 1, j2_jc], a1[i2_jc + 1, j2_jc + 1]]) pij = np.max([p_1, p_2]) p_131 = np.asarray([p_1, p_2]).ravel() p_1211 = [1 if x == pij else 0 for x in p_131] p_121 = 1 if a1[i2_jc, j2_jc] == pij else 0 p_3 = np.asarray([a2[i2_jc, j2_jc], a2[i2_jc, j2_jc + 1]]) p_4 = np.asarray([a2[i2_jc + 1, j2_jc], a2[i2_jc + 1, j2_jc + 1]]) p_13 = np.asarray([p_3, p_4]).ravel() p_14 = p_1211 * p_13 p_5 = np.asarray([a3[i2_jc, j2_jc], a3[i2_jc, j2_jc + 1]]) p_6 = np.asarray([a3[i2_jc + 1, j2_jc], a3[i2_jc + 1, j2_jc + 1]]) p_15 = np.asarray([p_5, p_6]).ravel() p_16 = np.asarray(p_14 * p_15).reshape(2, 2) jcp.append(p_16) jcp = np.asarray(jcp) dp.append(jcp) dwp.append(dp) delta_f = np.asarray(dwp) return jisuany, wc, sscqa, delta_o, delta_f def net(): # 3×3 jjc_wbqj = [[[-1.277300644,-0.454172162,0.358057716, 1.138250143,-2.397704576,-1.66424031, -0.794134078,0.8985833,0.675446205 ], -3.362863019 ], [[-1.274134977,2.338173328,2.301490473, 0.649226816,-0.339421188,-2.053992003, -1.021929466,-1.204455356,-1.899568354 ], -3.176354432 ], [[-1.869160636,2.044121491,-1.289733372, -1.710113666,-2.090869135,-2.945511246, 0.200874599,-1.322854945,0.206768688 ], -1.739194648 ]] scc_wbqj = [ [[-0.275747119,0.124448118, -0.961374728,0.717521785, -3.680378774,-0.594173531, 0.28047901,-0.782201884, -1.475452139,-2.009595842, -1.085433118,-0.188094434 ], 2.059747339], [[0.010226852,0.66050453, -1.591296391,2.189144834, 1.728139732,0.003480858, -0.250073861,1.897545127, 0.238156398,1.588690766, 2.246433757,-0.09260719 ], -2.746251954], [[-1.32191109,-0.217675096, 3.526685032,0.060919329, 0.61272745,0.217952344, -2.130273215,-1.678298588, 1.235675571,-0.486471873, -0.144296582,-1.234615501 ], -1.81779135388] ] jjc_wbqjnp = np.asarray(jjc_wbqj, dtype=object) scc_wbqjnp = np.asarray(scc_wbqj, dtype=object) return jjc_wbqjnp, scc_wbqjnp def updatenet(jjc_wbqj1, scc_wbqj1, sscqa, delta_o, delta_f, datax1, learning_rate): pianjjcw_zj = [] pianjjcb_zj = [] piansccw_zj = [] piansccb_zj = [] for i in range(datax1.shape[0]): pianjjcw = [] pianjjcb = [] for j in range(len(jjc_wbqj1)): jjc_sr = datax1[i].reshape(6, 6) jjc_z = [] for i0 in range(jjc_sr.shape[0] - 3): for j0 in range(jjc_sr.shape[1] - 3): x_1 = np.asarray([jjc_sr[i0, j0], jjc_sr[i0, j0 + 1], jjc_sr[i0+1, j0], jjc_sr[i0+1, j0+1]]) x_2 = np.asarray([jjc_sr[i0, j0 + 2], jjc_sr[i0, j0 + 3], jjc_sr[i0 + 1, j0 + 2], jjc_sr[i0 + 1, j0 + 3]]) x_3 = np.asarray([jjc_sr[i0 + 2, j0], jjc_sr[i0 + 2, j0 + 1], jjc_sr[i0 + 3, j0], jjc_sr[i0 + 3, j0 + 1]]) x_4 = np.asarray([jjc_sr[i0 + 2, j0+2], jjc_sr[i0 + 2, j0 + 3], jjc_sr[i0 + 3, j0 + 2], jjc_sr[i0 + 3, j0 + 3]]) xij = np.asarray([x_1, x_2, x_3, x_4]) jjc_z.append(xij) jjc_z = np.asarray(jjc_z) deltaf1 = delta_f[i][j].ravel() deltaf2 = [] for i1 in range(jjc_z.shape[0]): x11 = np.asarray(jjc_z[i1]).ravel() x12 = x11 * deltaf1 x13 = np.sum(x12) deltaf2.append(x13) pianjjcw.append(deltaf2) pianjjcb1 = np.sum(delta_f[i][j]) pianjjcb.append(pianjjcb1) pianjjcw_zj.append(pianjjcw) pianjjcb_zj.append(pianjjcb) piansccw = [] piansccb = [] for j1 in range(len(scc_wbqj1)): sscqa1 = sscqa[i]*delta_o[i][j1] piansccw.append(sscqa1) piansccb.append(delta_o[i][j1]) piansccw_zj.append(piansccw) piansccb_zj.append(piansccb) pianjjcw_zj1 = np.asarray(pianjjcw_zj) pianjjcb_zj1 = np.asarray(pianjjcb_zj) piansccw_zj1 = np.asarray(piansccw_zj) piansccb_zj1 = np.asarray(piansccb_zj) pianjjcw_zj2 = np.sum(pianjjcw_zj1, axis=0) pianjjcb_zj2 = np.sum(pianjjcb_zj1, axis=0) piansccw_zj2 = np.sum(piansccw_zj1, axis=0) piansccb_zj2 = np.sum(piansccb_zj1, axis=0) pianjjcw_zj3 = pianjjcw_zj2 * learning_rate pianjjcb_zj3 = pianjjcb_zj2 * learning_rate piansccw_zj3 = piansccw_zj2 * learning_rate piansccb_zj3 = piansccb_zj2 * learning_rate jjc_wb1 = [[jjc_wb[0][0] - pianjjcw_zj3[0], jjc_wb[0][1] - pianjjcb_zj3[0]], [jjc_wb[1][0] - pianjjcw_zj3[1], jjc_wb[1][1] - pianjjcb_zj3[1]], [jjc_wb[2][0] - pianjjcw_zj3[2], jjc_wb[2][1] - pianjjcb_zj3[2]]] ssc_wb1 = [[scc_wb[0][0] - piansccw_zj3[0], scc_wb[0][1] - piansccb_zj3[0]], [scc_wb[1][0] - piansccw_zj3[1], scc_wb[1][1] - piansccb_zj3[1]], [scc_wb[2][0] - piansccw_zj3[2], scc_wb[2][1] - piansccb_zj3[2]]] return jjc_wb1, ssc_wb1 jjc_wb, scc_wb = netarg() for _ in range(50): dx, dy = getxy() jisuany, wc, sscqa, deltao, deltaf = dancijisuan(jjc_wb, scc_wb, dx, dy) c = np.sum(wc) learning_rate = 0.2 jjc_wb, scc_wb = updatenet(jjc_wb, scc_wb, sscqa, deltao, deltaf, dx, learning_rate) print('错误值之和', c)
《深度学习的数学》涌井良幸等著第五章CNN的python实现
最新推荐文章于 2024-07-23 14:36:35 发布
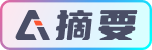