import React,{Component} from "react";
import {connect} from "react-redux";
import {
View,
Text,
StyleSheet,
Image,
ScrollView,
TouchableOpacity,
TextInput,
PanResponder,
Animated,
Easing
} from "react-native";
import Button from '../components/Button'
import Icon from "react-native-vector-icons/EvilIcons"
import { cs } from "../assets/css/commonStyle";
import { Toast } from "native-base";
class IndexD extends Component {
static navigationOptions = {
header:null,//<Text>哈哈哈</Text>,
title:"电影"
};
constructor(props){
super(props);
this.minHeaderH = 64
this.maxHeaderH = 300
this.headerH = this.maxHeaderH,
this.canAnimated = false,
this.canScroll = false,
this.headerStyles = [styles.topBox,{height: this.state.headerY}] //(this.canAnimated ? this.state.headerY : this.maxHeaderH)
//this.currentH = this.headerH //手势开始时的高度
}
state = {
isRefreshing: false,
loaded: 0,
headerY:new Animated.Value(300),
opacityVal:new Animated.Value(0),
length_Y:new Animated.Value(this.headerH),
length_X:new Animated.Value(0),
rowData: Array.from(new Array(10)).map(
(val, i) => ({text: i})),
}
componentWillMount(){
this._panResponder = PanResponder.create({
// 要求成为响应者:
onStartShouldSetPanResponder: (evt, gestureState) => {
console.log('onStartShouldSetPanResponder','是vfiy电脑用了响应者');
return true;
},
onStartShouldSetPanResponderCapture: (evt, gestureState) => {
//这个属性接收一个回调函数,函数原型是 function(evt): bool,在触摸事件开始(touchDown)的时候,
//RN 容器组件会回调此函数,询问组件是否要劫持事件响应者设置,自己接收事件处理,如果返回 true,表示需要劫持;
console.log('onStartShouldSetPanResponderCapture','');
return false
},
onMoveShouldSetPanResponder: (evt, gestureState) => {
console.log('onMoveShouldSetPanResponder')
return true;
},
onMoveShouldSetPanResponderCapture: (evt, gestureState) => {
//如果返回true 表示劫持 false给i劫持
if(this.headerH == 64){
console.log('onMoveShouldSetPanResponderCapture',' = 64 ,不劫持onMove移动动画');
return false
}else{
console.log('onMoveShouldSetPanResponderCapture',' != 64 ,劫持onMove移动动画');
return true
}
},
onPanResponderGrant: (evt, gestureState) => {
console.log('onPanResponderGrant','开始手势操作。给用户一些视觉反馈,让他们知道发生了什么事情!');
// 开始手势操作。给用户一些视觉反馈,让他们知道发生了什么事情!
this.currentH = this.headerH;
// gestureState.{x,y}0 现在会被设置为0
},
onPanResponderMove: (evt, gestureState) => {
// 最近一次的移动距离为gestureState.move{X,Y}
let BgOpacity = 1-((this.maxHeaderH-this.headerH)/(236));
console.log('BgOpacity',BgOpacity);
if(this.headerH>64 && gestureState != 0){
this.header.setNativeProps({
height:this.currentH+gestureState.dy,
})
}
// 从成为响应者开始时的累计手势移动距离为gestureState.d{x,y}
},
onPanResponderRelease: (evt, gestureState) => {
// 用户放开了所有的触摸点,且此时视图已经成为了响应者。
// 一般来说这意味着一个手势操作已经成功完成。
this.state.headerY.setValue(this.headerH);
console.log('------',this.headerH);
if(this.headerH>80 && this.headerH != 300){
this.canAnimated = true;
Animated.timing(this.state.headerY, {
toValue: 64,
duration: 600,
easing: Easing.linear,
}).start();
}
console.log("onPanResponderRelease");
},
onPanResponderTerminationRequest: (evt, gestureState) => {
console.log('onPanResponderTerminationRequest','如果回调函数返回为 true,则表示同意释放响应者角色 同时会回调onResponderTerminate函数,通知组件事件响应处理被终止了');
if(this.headerH == 64){
console.log('onPanResponderTerminationRequest',' !true ,如果回调函数返回为 true,则表示同意释放响应者角色');
return true
}else{
console.log('onPanResponderTerminationRequest',' false ,如果回调函数返回为 false,则表示不释放响应者角色');
return false
}
},
onPanResponderTerminate: (evt, gestureState) => {
// 另一个组件已经成为了新的响应者,所以当前手势将被取消。
console.log('onPanResponderTerminate','另一个组件已经成为了新的响应者,所以当前手势将被取消')
},
onShouldBlockNativeResponder: (evt, gestureState) => {
// 返回一个布尔值,决定当前组件是否应该阻止原生组件成为JS响应者
// 默认返回true。目前暂时只支持android。
return true;
},
});
}
_onScroll=(e)=>{
console.log(e.nativeEvent);
Animated.event(
{nativeEvent:{contentOffset:{Y:this.state.opacityVal}}}
);
}
//onlayout事件 高度改变了 其它也改变
heightChangeEvent(e,pThis){
this.headerH = e.nativeEvent.layout.height
let BgOpacity = 1-((this.maxHeaderH-this.headerH)/(236));
this.header.setNativeProps({
backgroundColor:'rgba(70,147,236,'+BgOpacity+')'
})
this.headerBottom.setNativeProps({
opacity:BgOpacity
}),
this.headerTop.setNativeProps({
opacity:BgOpacity
})
console.log("headerH变化中。。。",this.headerH);
}
render(){
console.log("indexD screen this.props:",this.props);
const {navigate} = this.props.navigation;
// this.state.length_Y.interpolate({
// inputRange:[-200,0,200],
// outputRange: [0,200,400]
// })
return (
<View style={styles.body} {...this._panResponder.panHandlers}>
<Animated.View ref={(r)=>this.header=r }
onLayout={this.heightChangeEvent.bind(this)}
style={this.headerStyles}
>
<View style={styles.headerTop} ref={(r)=>this.headerTop=r}><Text style={{color:'#ffffff'}}>天气 签到</Text></View>
<View style={styles.headerMiddle}>
<TextInput
style={{height:40,width:'80%',backgroundColor:'#ffffff'}}
placeholder ="搜索 ..."// = android EditText hint
placeholderTextColor="#6435c9"// = hint color
underlineColorAndroid='transparent'// 下划线透明
ref={(r)=>this.headerMiddle=r}
// clearButtonMode="while-editing"
/>
</View>
<View style={styles.headerBottom} ref={(r)=>this.headerBottom=r}>
<Icon name="camera" size={24} color="#ff0000" ></Icon>
<Icon name="camera" size={24} color="#ff0000" ></Icon>
<Icon name="camera" size={24} color="#ff0000" ></Icon>
<Icon name="camera" size={24} color="#ff0000" ></Icon>
</View>
</Animated.View>
<ScrollView scrollEventThrottle = {10} scrollEnabled={false} >
<View style={styles.header}>
<View style={styles.content} >
<View style={{flexDirection:"row",justifyContent:"flex-start"}}>
<Image source={require('../assets/image/login.png')} style={styles.headerImage} />
<View style={styles.topText}>
<Text style={{color:cs.white}}>刘德华</Text>
<Text style={{color:cs.white}}>个人信息</Text>
</View>
</View>
<View style={{justifyContent:'center',alignContent:'center'}}><Text style={{color:cs.white}}>右边按钮</Text></View>
</View>
</View>
<View style={styles.buttonList}>
<TouchableOpacity onPress={()=>{this.buttonClick()}} style={styles.Li}>
<View style={styles.Lileft}>
<Icon name="sc-pinterest" size={24} color="#ff0000" style={styles.iconLeft} ></Icon>
<Text>我的钱包</Text>
</View>
<View style={styles.LiRight}>
<Text>信用卡</Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
<TouchableOpacity onPress={()=>{this.buttonClick()}} style={styles.Li}>
<View style={styles.Lileft}>
<Icon name="eye" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>余额</Text>
</View>
<View style={styles.LiRight}>
<Text>¥2000元</Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
<TouchableOpacity onPress={()=>{this.buttonClick()}} style={styles.Li}>
<View style={styles.Lileft}>
<Icon name="minus" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>抵用券</Text>
</View>
<View style={styles.LiRight}>
<Text>62</Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
<TouchableOpacity onPress={()=>{this.buttonClick()}} style={styles.Li}>
<View style={styles.Lileft}>
<Icon name="sc-google-plus" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>会员卡</Text>
</View>
<View style={styles.LiRight}>
<Text>1张</Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
<TouchableOpacity onPress={()=>{this.buttonClick()}} style={styles.Li}>
<View style={styles.Lileft}>
<Icon name="camera" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>我的评价</Text>
</View>
<View style={styles.LiRight}>
<Text></Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
<TouchableOpacity onPress={()=>{this.buttonClick()}} style={styles.Li}>
<View style={styles.Lileft}>
<Icon name="chevron-down" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>我的评价</Text>
</View>
<View style={styles.LiRight}>
<Text></Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
<TouchableOpacity onPress={()=>{this.buttonClick()}} style={styles.Li}>
<View style={styles.Lileft}>
<Icon name="comment" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>我的文章</Text>
</View>
<View style={styles.LiRight}>
<Text>23</Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
{
!this.props.isLoggedIn ?
<TouchableOpacity onPress={()=>navigate("LoginActivity",{paramKey:"paramValue"})} style={[styles.Li]}>
<View style={styles.Lileft}>
<Icon name="credit-card" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>登录</Text>
</View>
<View style={styles.LiRight}>
<Text>23</Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
:
<TouchableOpacity onPress={()=>{this.buttonClick()}} style={[styles.Li,{height:300}]}>
<View style={styles.Lileft}>
<Icon name="credit-card" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>注销</Text>
</View>
<View style={styles.LiRight}>
<Text>{this.props.isLoggedIn ? "已经登录" : '未登录'}</Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
}
{
this.props.isLoggedIn ||
<TouchableOpacity onPress={()=>navigate("UserRegister",{paramKey:"paramValue"})} style={[styles.Li]}>
<View style={styles.Lileft}>
<Icon name="credit-card" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>注册</Text>
</View>
<View style={styles.LiRight}>
<Text>23</Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
}
<TouchableOpacity onPress={()=>{this.buttonClick()}} style={styles.Li}>
<View style={styles.Lileft}>
<Icon name="pencil" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>我的视频</Text>
</View>
<View style={styles.LiRight}>
<Text>23</Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
<TouchableOpacity onPress={()=>{this.buttonClick()}} style={styles.Li}>
<View style={styles.Lileft}>
<Icon name="calendar" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>我的视频</Text>
</View>
<View style={styles.LiRight}>
<Text>23</Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
<TouchableOpacity onPress={()=>{this.buttonClick()}} style={styles.Li}>
<View style={styles.Lileft}>
<Icon name="lock" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>清空缓存</Text>
</View>
<View style={styles.LiRight}>
<Text>23</Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
<TouchableOpacity onPress={()=>{this.buttonClick()}} style={styles.Li}>
<View style={styles.Lileft}>
<Icon name="calendar" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>我的视频</Text>
</View>
<View style={styles.LiRight}>
<Text>23</Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
<TouchableOpacity onPress={()=>{this.buttonClick()}} style={styles.Li}>
<View style={styles.Lileft}>
<Icon name="lock" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>清空缓存</Text>
</View>
<View style={styles.LiRight}>
<Text>23</Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
<TouchableOpacity onPress={()=>{this.buttonClick()}} style={styles.Li}>
<View style={styles.Lileft}>
<Icon name="calendar" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>我的视频</Text>
</View>
<View style={styles.LiRight}>
<Text>23</Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
<TouchableOpacity onPress={()=>{this.buttonClick()}} style={styles.Li}>
<View style={styles.Lileft}>
<Icon name="lock" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
<Text>清空缓存</Text>
</View>
<View style={styles.LiRight}>
<Text>23</Text>
<Icon name="chevron-right" size={24} color="#ff0000" style={styles.iconLeft}></Icon>
</View>
</TouchableOpacity>
</View>
</ScrollView>
</View>
//{/* <Image source={require('../assets/image/login.png')} style={styles.icons} /> */}
// <View style={styles.body}>
// <Text style={{fontSize:20,textAlign:"center",color:"red"}}>
// IndexD 当前登录状态:{this.props.isLoggedIn ? "已经登录" : '未登录'}
// </Text>
// <Button onPress={()=>navigate("LoginActivity",{paramKey:"paramValue"})}>
// <Text>去登录就行了</Text>
// </Button>
// </View>
)
}
buttonClick(){
alert("点点更健康。。。");
}
dologin(){
const {navigate} = this.props.navigation;
console.log("正在去登录");
}
}
const styles = StyleSheet.create({
body:{
flex: 1,
backgroundColor:'#333333'//cs.bgColor
},
topBox:{
width:'100%',
justifyContent:'space-around',
flexDirection:'column',
height:cs.navHeight,
alignContent:'center',
alignItems:'center',
zIndex:500,
height:300,
borderWidth: cs.smallLine,
opacity: 1,
backgroundColor: '#4693EC',
//...cs.border1
},
headerTop:{
},
headerMiddle:{
width:'100%',justifyContent: 'center',alignItems: 'center',
},
headerBottom:{
width:'100%',justifyContent:'space-around',flexDirection:'row'
},
header:{
backgroundColor:"#01C1AE",
paddingLeft: 15,
paddingRight: 15,
paddingTop:cs.statusBarH+25,
paddingBottom: 15,
justifyContent: 'center',
alignContent:"center",
},
content:{
flexDirection: 'row',justifyContent:'space-between',alignContent:"center"
},
headerImage:{
//marginTop: 45,
width:60,
height:60,
borderRadius: 30,
borderWidth: cs.smallLine,
borderColor: 'red',
marginRight: 10,
},
topText:{
justifyContent:"space-between",alignContent:"center"
},
buttonList:{
flex: 1,
flexDirection: 'column',
marginVertical: 20,
},
Li:{
backgroundColor: cs.white,
borderBottomWidth: cs.lineWidth,
borderColor: cs.lineColor,
justifyContent: 'space-between',
alignContent: 'center',
flexDirection: 'row',
paddingVertical: 18,
paddingHorizontal: 7,
},
Lileft:{
flexDirection: 'row',
justifyContent: 'flex-start',
},
LiRight:{
flexDirection: 'row',
justifyContent: 'flex-start',
},
icons:{
width:15,
height:15
},
iconLeft:{
marginRight:5
}
});
mapStateToProps = (state)=>{
return {
isLoggedIn:state.userStore.isLoggedIn
}
};
export default connect(mapStateToProps)(IndexD);
ract native PanResponder 滑动事件 手势动画 事件管理等
最新推荐文章于 2021-06-07 11:01:30 发布
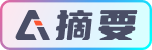