广点通sdk接入 _原生广告
1:导入相关架包,写入相关权限和配置
android-query-full.0.26.7.jar
GDTUnionSDK.4.8.513.jar
Volley.jar
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_COARSE_UPDATES" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<service
android:name="com.qq.e.comm.DownloadService"
android:exported="false" />
<activity
android:name="com.qq.e.ads.ADActivity" android:configChanges="keyboard|keyboardHidden|orientation|screenSize" />
2:include自定义广告布局nativelistitem.xml:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:descendantFocusability="blocksDescendants" >
<!-- 广告Logo -->
<ImageView
android:id="@+id/img_logo"
android:layout_width="64dp"
android:layout_height="64dp"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_margin="10dp" />
<!-- 广告标题 -->
<TextView
android:id="@+id/text_name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignTop="@id/img_logo"
android:layout_margin="5dp"
android:layout_toRightOf="@id/img_logo"
android:textColor="@android:color/black" />
<!-- 内容 -->
<TextView
android:id="@+id/text_desc"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/text_name"
android:layout_marginLeft="5dp"
android:layout_marginRight="15dp"
android:layout_toRightOf="@id/img_logo"
android:ellipsize="end"
android:singleLine="true"
android:textColor="#A0000000" />
<!-- 文本状态 -->
<TextView
android:id="@+id/text_status"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/text_desc"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"
android:layout_toRightOf="@id/img_logo"
android:textColor="#A0000000" />
<!-- 内容图片 -->
<ImageView
android:id="@+id/img_poster"
android:layout_width="match_parent"
android:layout_height="180dp"
android:layout_below="@id/text_status"
android:layout_margin="10dp"
android:minHeight="180dp"
android:scaleType="fitXY" />
<!-- 分割线 -->
<View
android:id="@+id/divider"
android:layout_width="match_parent"
android:layout_height="1dp"
android:layout_below="@id/img_poster"
android:layout_margin="10dp"
android:background="#30000000" />
<!-- 下载按钮 -->
<Button
android:id="@+id/btn_download"
android:layout_width="match_parent"
android:layout_height="40dp"
android:layout_below="@id/divider"
android:layout_margin="10dp"
android:background="#009688"
android:textColor="@android:color/white" />
</RelativeLayout>
3:主界面布局:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.qq.e.NewNativeAdActivity" >
<!-- 自定义广告布局类 -->
<include
android:id="@+id/nativeADContainer"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerInParent="true"
layout="@layout/nativelistitem" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/nativeADContainer"
android:layout_marginTop="10dp"
android:orientation="horizontal"
android:weightSum="2" >
<Button
android:id="@+id/loadNative"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="加载广告" />
<Button
android:id="@+id/showNative"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="展示广告" />
</LinearLayout>
</RelativeLayout>
4:详见Activity:
/**
* 自定义布局的原生广告
*
* @author Administrator
*
*/
public class NativeADActivity extends Activity implements NativeAdListener,
OnClickListener {
private NativeADDataRef adItem;
private NativeAD nativeAD;
private Button btn_download;
private Button loadNative, showNative;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_gdtnativead_demo);
loadNative = (Button) findViewById(R.id.loadNative);
showNative = (Button) findViewById(R.id.showNative);
loadNative.setOnClickListener(this);
showNative.setOnClickListener(this);
}
/**
* 加载广告,初始化
*/
public void loadAD() {
MultiProcessFlag.setMultiProcess(true);
if (nativeAD == null) {
this.nativeAD = new NativeAD(this, Constants.APPID,
Constants.NativePosID, this);
}
int count = 1;
nativeAD.loadAD(count);
}
/**
* 展示广告,自定义广告布局
*/
public void showAD() {
ImageView img_logo = (ImageView) findViewById(R.id.nativeADContainer)
.findViewById(R.id.img_logo);
ImageView img_poster = (ImageView) findViewById(R.id.nativeADContainer)
.findViewById(R.id.img_poster);
TextView text_name = (TextView) findViewById(R.id.nativeADContainer)
.findViewById(R.id.text_name);
TextView text_desc = (TextView) findViewById(R.id.nativeADContainer)
.findViewById(R.id.text_desc);
btn_download = (Button) findViewById(R.id.nativeADContainer)
.findViewById(R.id.btn_download);
getImage(img_logo, adItem.getIconUrl());
getImage(img_poster, adItem.getImgUrl());
text_name.setText(adItem.getTitle());
text_desc.setText(adItem.getDesc());
adItem.onExposured(this.findViewById(R.id.nativeADContainer));
btn_download.setText(getADButtonText());
btn_download.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
adItem.onClicked(v);
}
});
}
/**
* App类广告安装、下载状态的更新(普链广告没有此状态,其值为-1) 返回的AppStatus含义如下: 0:未下载 1:已安装 2:已安装旧版本
* 4:下载中(可获取下载进度“0-100”) 8:下载完成 16:下载失败
*/
private String getADButtonText() {
if (adItem == null) {
return "……";
}
if (!adItem.isAPP()) {
return "查看详情";
}
switch (adItem.getAPPStatus()) {
case 0:
return "点击下载";
case 1:
return "点击启动";
case 2:
return "点击更新";
case 4:
return "下载中" + adItem.getProgress() + "%";
case 8:
return "点击安装";
case 16:
return "下载失败,点击重试";
default:
return "查看详情";
}
}
/**
* 加载成功时调用
*/
@Override
public void onADLoaded(List<NativeADDataRef> arg0) {
if (arg0.size() > 0) {
adItem = arg0.get(0);
showNative.setEnabled(true);
Toast.makeText(this, "原生广告加载成功", Toast.LENGTH_LONG).show();
} else {
Log.i("AD_DEMO", "NOADReturn");
}
}
/**
* 广告状态发送改变,更新下载按钮文字
*/
@Override
public void onADStatusChanged(NativeADDataRef arg0) {
btn_download.setText(getADButtonText());
}
/**
* 加载失败时调用
*/
@Override
public void onNoAD(int arg0) {
Log.e("tag: ", "" + arg0);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.loadNative:
loadAD();
break;
case R.id.showNative:
showAD();
break;
}
}
/**
* Volley框架获得图片
*
* @param iv
* @param url
*/
public void getImage(ImageView iv, String url) {
String imgUrl = url;
RequestQueue mRequestQueue = Volley.newRequestQueue(this);
final LruCache<String, Bitmap> mImageCache = new LruCache<String, Bitmap>(
20);
ImageLoader.ImageCache imageCache = new ImageLoader.ImageCache() {
@Override
public void putBitmap(String key, Bitmap value) {
mImageCache.put(key, value);
}
@Override
public Bitmap getBitmap(String key) {
return mImageCache.get(key);
}
};
ImageLoader mImageLoader = new ImageLoader(mRequestQueue, imageCache);
ImageLoader.ImageListener listener = ImageLoader
.getImageListener(iv, android.R.drawable.ic_menu_rotate,
android.R.drawable.ic_delete);
mImageLoader.get(imgUrl, listener);
}
}
5:效果图:
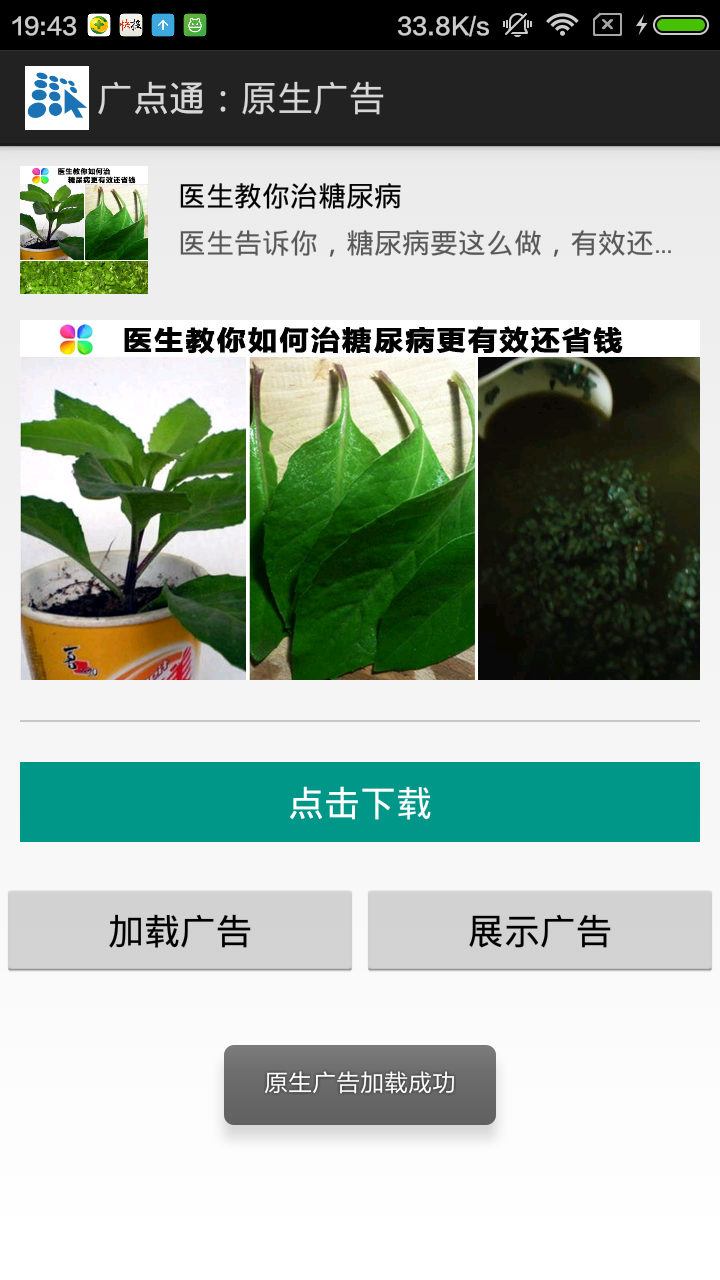