1.效果图
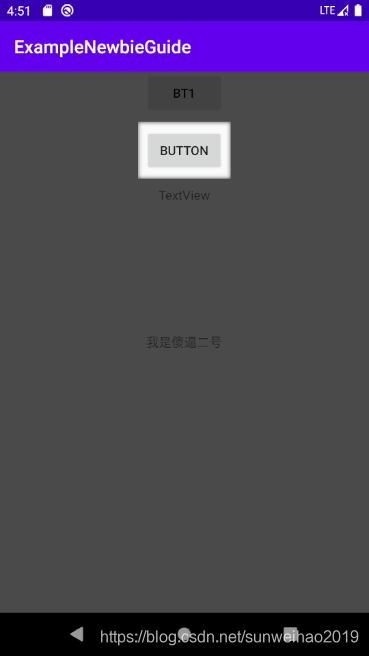
2.使用步骤
1.添加依赖
//新手使用引导
// 下面注释是为了忽略
//noinspection GradleCompatible
compileOnly 'com.android.support:appcompat-v7:25.3.1'
implementation 'com.github.huburt-Hu:NewbieGuide:v2.4.0'
2.在布局中使用
NewbieGuide.with(this)
.setLabel("page")
.setOnGuideChangedListener(new OnGuideChangedListener() {
@Override
public void onShowed(Controller controller) {
Log.e(TAG, "NewbieGuide onShowed: ");
}
@Override
public void onRemoved(Controller controller) {
Log.e(TAG, "NewbieGuide onRemoved: ");
}
})
.setOnPageChangedListener(new OnPageChangedListener() {
@Override
public void onPageChanged(int page) {
Log.e(TAG, "NewbieGuide onPageChanged: " + page);
}
})
.alwaysShow(true)
.addGuidePage(
GuidePage.newInstance()
.addHighLight(bt_content)
.addHighLight(bt_content, HighLight.Shape.RECTANGLE)
.setLayoutRes(R.layout.activity_text_style)
.setOnLayoutInflatedListener(new OnLayoutInflatedListener() {
@Override
public void onLayoutInflated(View view, Controller controller) {
TextView tv = view.findViewById(R.id.textView2);
tv.setText("我是动态设置的文本");
}
})
)
.addGuidePage(
GuidePage.newInstance()
.addHighLight(tvBottom, HighLight.Shape.RECTANGLE, 20)
.setLayoutRes(R.layout.view_guide_custom)
.setEverywhereCancelable(true)
)
.show();
3.全部代码
1.java
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
import com.app.hubert.guide.NewbieGuide;
import com.app.hubert.guide.core.Controller;
import com.app.hubert.guide.listener.OnGuideChangedListener;
import com.app.hubert.guide.listener.OnLayoutInflatedListener;
import com.app.hubert.guide.listener.OnPageChangedListener;
import com.app.hubert.guide.model.GuidePage;
import com.app.hubert.guide.model.HighLight;
public class MainActivity2 extends AppCompatActivity implements View.OnClickListener {
private Button bt_content;
private String TAG = MainActivity2.class.getSimpleName();
private Button tvBottom;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main2);
initView();
}
private void initView() {
bt_content = (Button) findViewById(R.id.bt_content);
bt_content.setOnClickListener(this);
tvBottom = (Button) findViewById(R.id.tvBottom);
tvBottom.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.bt_content:
NewbieGuide.with(this)
.setLabel("page")
.setOnGuideChangedListener(new OnGuideChangedListener() {
@Override
public void onShowed(Controller controller) {
Log.e(TAG, "NewbieGuide onShowed: ");
}
@Override
public void onRemoved(Controller controller) {
Log.e(TAG, "NewbieGuide onRemoved: ");
}
})
.setOnPageChangedListener(new OnPageChangedListener() {
@Override
public void onPageChanged(int page) {
Log.e(TAG, "NewbieGuide onPageChanged: " + page);
}
})
.alwaysShow(true)
.addGuidePage(
GuidePage.newInstance()
.addHighLight(bt_content)
.addHighLight(bt_content, HighLight.Shape.RECTANGLE)
.setLayoutRes(R.layout.activity_text_style)
.setOnLayoutInflatedListener(new OnLayoutInflatedListener() {
@Override
public void onLayoutInflated(View view, Controller controller) {
TextView tv = view.findViewById(R.id.textView2);
tv.setText("我是动态设置的文本");
}
})
)
.addGuidePage(
GuidePage.newInstance()
.addHighLight(tvBottom, HighLight.Shape.RECTANGLE, 20)
.setLayoutRes(R.layout.view_guide_custom)
.setEverywhereCancelable(true)
)
.show();
break;
case R.id.tvBottom:
break;
}
}
}
2.xml
1.activity_main2
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity2">
<Button
android:id="@+id/bt_content"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="bt1"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/tvBottom"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Button"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/bt_content" />
<TextView
android:id="@+id/iv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="TextView"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/tvBottom" />
</androidx.constraintlayout.widget.ConstraintLayout>
2.activity_text_style
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".TextStyleActivity3">
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="TextView"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
3.view_guide_custom
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="我是傻逼二号"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>