MainActivity.java
public class MainActivity extends AppCompatActivity {
ViewPager viewPager;
ArrayList<MyFragment> fragments;
ViewPagerAdapter adapter;
TabLayout tabLayout;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
viewPager = findViewById(R.id.viewPager);
tabLayout = findViewById(R.id.tablayout);
//初始化数据
fragments = new ArrayList<>();
fragments.add(new MyFragment("111",null,R.layout.first_fragment));
fragments.add(new MyFragment("222",null,R.layout.second_fragment));
//设置ViewPager适配器
adapter = new ViewPagerAdapter(getSupportFragmentManager(),fragments);
viewPager.setAdapter(adapter);
//关联ViewPager
tabLayout.setupWithViewPager(viewPager);
//设置固定的tab
tabLayout.setTabMode(TabLayout.MODE_FIXED);
}
}
activity_main
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.tabs.TabLayout
android:id="@+id/tablayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="50dp"
android:layout_marginRight="150dp"
app:layout_constraintTop_toTopOf="parent"
android:background="@color/purple_200"
app:tabIndicatorColor="#03A9F4"
app:tabIndicatorFullWidth="false"
app:tabIndicatorAnimationDuration="400"
app:tabSelectedTextColor="#FF0000"
app:tabTextColor="@color/white"/>
<androidx.viewpager.widget.ViewPager
android:id="@+id/viewPager"
android:layout_width="match_parent"
android:layout_height="300dp"
android:layout_marginLeft="50dp"
android:layout_marginRight="50dp"
android:background="@color/cardview_dark_background"
app:layout_constraintTop_toBottomOf="@id/tablayout"/>
</androidx.constraintlayout.widget.ConstraintLayout>
MyFragement.java
public class MyFragment extends Fragment {
private final String title;
private final String content;
private final int res;
Context mContext;
public MyFragment(String title, String content,int res){
super();
this.title = title;
this.content = content;
this.res = res;
}
public String getTitle() {
return title;
}
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
mContext = getActivity();
}
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
return inflater.inflate(res,container,false);
}
}
ViewPagerAdapter.java
public class ViewPagerAdapter extends FragmentPagerAdapter {
private final ArrayList<MyFragment> fragments;
public ViewPagerAdapter(FragmentManager fm, ArrayList<MyFragment> fragments) {
super(fm);
this.fragments = fragments;
}
@Override
public int getCount() {
return fragments.size();
}
@NonNull
@Override
public Fragment getItem(int position) {
return fragments.get(position);
}
/**
* 得到页面标题
* @param position The position of the title requested
* @return
*/
@Nullable
@Override
public CharSequence getPageTitle(int position) {
return fragments.get(position).getTitle();
}
}
first_fragment
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/teal_200">
</androidx.constraintlayout.widget.ConstraintLayout>
second_fragment
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/black">
</androidx.constraintlayout.widget.ConstraintLayout>
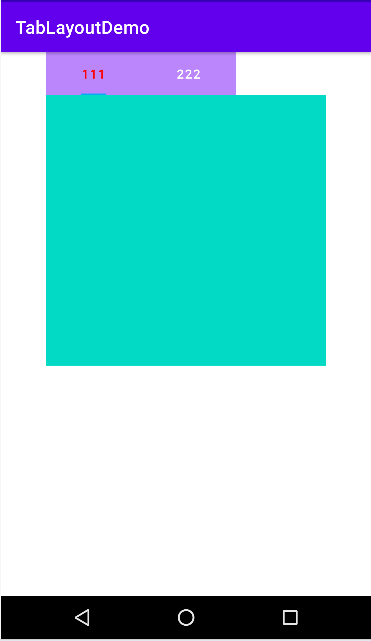
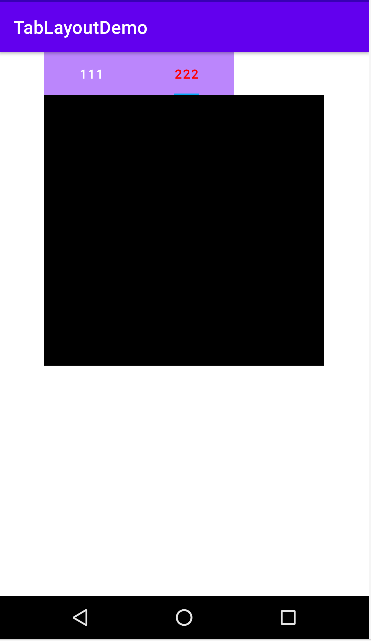