目录
- 3.1 表达式和表达语句
- 3.2 ⚪的计算
- 3.3 温度转换(华氏度转化摄氏度)
- 3.4 getchar()输入putchar()输出
- 3.5 整型变量与字符型变量的关系
- 3.6 什么是算数运算?。。。
- 3.7 C++如何表示“真假”?系统如何判断量的“真假”?
- 3.8 逻辑表达式的值
- 3.9 比较大小
- 3.10 分段函数
- 3.11 分段成绩
- 3.12 逆序打印
- 3.13 奖金
- 3.14 四个数排序
- 3.15 最大公约数和最小公倍数
- 3.16 统计输入各类型字符数量
- 3.17 自定义多位数求和
- 3.18 阶乘求和
- 3.19 水仙花数
- 3.20 求1000以内的完数
- 3.21 分数序列求和
- 3.22 猴子吃桃
- 3.23 迭代法开根号
- 3.24 打印图形
- 3.25 比赛名单
3.1 表达式和表达语句
表达式末尾没有分号,而表达式语句有。表达式语句用于实现输入输出,赋值等操作。
在进行条件判断时应使用表达式,其他情况下使用表达式语句。
3.2 ⚪的计算
#include <iostream>
#include <iomanip>
using namespace std;
#define pi 3.1415926//cmath 未提供pi值
int main()
{
float r, h;
//r=1.5,h=3
cout << "Please enter radius:";
cin >> r;
cout << "Please enter height:";
cin >> h;
/*计算圆的周长*/
cout << "Circumference is:" << setiosflags(ios::fixed)<<setprecision(2)
<<2 * pi * r<<endl;
/*计算圆的表面积*/
cout << "Surface area of circu is:" << setiosflags(ios::fixed) << setprecision(2)
<< pi * r * r << endl;
/*计算球的表面积*/
cout << "Surface area of ball is:" << setiosflags(ios::fixed) << setprecision(2)
<< 4 * pi * r << endl;
/*计算球的体积*/
cout << "Volume of ball is:" << setiosflags(ios::fixed) << setprecision(2)
<< (4/3) * pi * r << endl;
/*计算圆柱的体积*/
cout << "Volume of column is:" << setiosflags(ios::fixed) << setprecision(2)
<< pi * r * r * h;
return 0;
}
3.3 温度转换(华氏度转化摄氏度)
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
double F;
cout << "Please enter Fahrenheit:";
cin >> F;
cout << "Celsius is:" << setiosflags(ios::fixed) << setprecision(2) <<
(5.0 / 9.0) * (F - 32);
return 0;
}
3.4 getchar()输入putchar()输出
3.5 整型变量与字符型变量的关系
3.6 什么是算数运算?。。。
3.7 C++如何表示“真假”?系统如何判断量的“真假”?
数值1代表“真”,0代表“假”。系统以0和非0来判定一个量是“真”或“假”。
3.8 逻辑表达式的值
- 0
- 1
- 1
- 0
- 1
3.9 比较大小
#include <iostream>
using namespace std;
int main()
{
int a, b, c,max;
cin >> a;
cin >> b;
cin >> c;
max = a;
if (max <= b)
{
max = b;
if (max <= c)
max = c;
cout << max;
}
else
cout << max;
return 0;
}
3.10 分段函数
#include <iostream>
using namespace std;
int main()
{
int x;
cin >> x;
if (x < 1)
cout << "y="<<x;
else if ((x >= 1) && x < 10)
cout << "y=" << 2 * x - 11;
else if ( x > 10)
cout << "y=" << 3 * x - 10;
else
cout << "Error"<<endl;
return 0;
}
3.11 分段成绩
#include <iostream>
using namespace std;
int main()
{
int x;
cout << "Please enter a score:";
cin >> x;
if ((x >= 90) && (x <= 100))
cout << "A";
else if ((x >= 80) && (x < 90))
cout << "B";
else if ((x >= 70) && (x < 80))
cout << "C";
else if ((x >= 60) && (x < 70))
cout << "D";
else if ((x < 60) && (x >= 0))
cout << "E";
else
cout << "Please make sure the score between 0-100"<<endl;
return 0;
}
3.12 逆序打印
#include <iostream>
using namespace std;
int main()
{
unsigned int x,d1,d2,d3,d4,d5,n;//n is a counter
n = 5;
cin >> x;
/*将各位剥离*/
d1 = x / 10000;
d2 = (x / 1000) % 10;
d3 = (x / 100) % 10;
d4 = (x / 10) % 10;
d5 = x % 10;
/*防止输入数字位数大于5*/
if (d1 > 10) {
cout << "Please enter numder under 99999!"<<endl;
return 0;
}
/*计算几位数*/
if (d1 > 0)
cout << n << '\n';
else {
n--;
if (d2 > 0)
cout << n << '\n';
else{
n--;
if (d3 > 0)
cout << n << '\n';
else{
n--;
if (d4 > 0)
cout << n << '\n';
else {
n--;
if (d5 >= 0)
cout << n << '\n';
}
}
}
}
/*打印各位数字*/
switch (n)
{
case(1):cout << d5;
break;
case(2):
cout << d4 << d5<<endl;
cout << d5 << d4;
break;
case(3):
cout << d3 << d4 << d5 << endl;
cout << d5 << d4 << d3;
break;
case(4):
cout << d2 << d3 << d4 << d5 << endl;
cout << d5 << d4 << d3 << d2;
break;
case(5):
cout << d1 << d2 << d3 << d4 << d5 << endl;
cout << d5 << d4 << d3 << d2 << d1;
break;
default:
break;
}
return 0;
}
3.13 奖金
Method-if
#include <iostream>
using namespace std;
int main()
{
double Profit, Bonus;
cout << "Please enter your Profit:";
cin >> Profit;
if (Profit <= 100000)
cout << "YOU WILL GET BONUS:" << Profit * 0.1;
else if ((Profit > 100000) && (Profit <= 200000))
cout << "YOU WILL GET BONUS:" << ((Profit - 100000) * 0.075) +
10000;
else if ((Profit > 200000) && (Profit <= 400000))
cout << "YOU WILL GET BONUS:" << ((Profit - 200000) * 0.05) +
100000 * 0.075 + 10000;
else if ((Profit > 400000) && (Profit <= 600000))
cout << "YOU WILL GET BONUS:" << ((Profit - 400000) * 0.03) +
200000 * 0.05 +100000 * 0.075 + 10000;
else if ((Profit > 600000) && (Profit <= 1000000))
cout << "YOU WILL GET BONUS:" << ((Profit - 600000) * 0.015) +
200000 * 0.03 +200000 * 0.05 + 100000 * 0.075 + 10000;
else if (Profit > 1000000)
cout << "YOU WILL GET BONUS:" << ((Profit - 1000000) * 0.01) +
400000 * 0.015 + 200000 * 0.03 +200000 * 0.05 + 100000 * 0.075 + 10000;
else
cout <<"error"<<endl;
return 0;
}
Method-switch
#include <iostream>
using namespace std;
int main()
{
double Profit, Bonus;
int Level;
cout << "Please enter your Profit:";
cin >> Profit;
if (Profit <= 100000)
Level = 1;
else if ((Profit > 100000) && (Profit <= 200000))
Level = 2;
else if ((Profit > 200000) && (Profit <= 400000))
Level = 3;
else if ((Profit > 400000) && (Profit <= 600000))
Level = 4;
else if ((Profit > 600000) && (Profit <= 1000000))
Level = 5;
else if (Profit > 1000000)
Level = 6;
else
cout <<"error"<<endl;
switch (Level)
{
case(1):
cout << "YOU WILL GET BONUS:" << Profit * 0.1; break;
case(2):
cout << "YOU WILL GET BONUS:" << ((Profit - 100000) * 0.075) +
10000; break;
case(3):
cout << "YOU WILL GET BONUS:" << ((Profit - 200000) * 0.05) +
100000 * 0.075 + 10000; break;
case(4):
cout << "YOU WILL GET BONUS:" << ((Profit - 400000) * 0.03) +
200000 * 0.05 + 100000 * 0.075 + 10000; break;
case(5):
cout << "YOU WILL GET BONUS:" << ((Profit - 600000) * 0.015) +
200000 * 0.03 + 200000 * 0.05 + 100000 * 0.075 + 10000; break;
case(6):
cout << "YOU WILL GET BONUS:" << ((Profit - 1000000) * 0.01) +
400000 * 0.015 + 200000 * 0.03 +200000 * 0.05 + 100000 * 0.075 +
10000; break;
default:
break;
}
return 0;
}
3.14 四个数排序
#include <iostream>
using namespace std;
int main()
{
int n[4],temp,i,j;
cin >> n[0];
cin >> n[1];
cin >> n[2];
cin >> n[3];
for (j = 0; j < 4;j++) {
for (i = j; i < 3; i++) {
if (n[j] > n[i + 1]) {
temp = n[j];
n[j] = n[i + 1];
n[i + 1] = temp;
}
}
}
for (i = 0; i < 4; i++) {
cout << n[i]<<endl;
}
return 0;
}
3.15 最大公约数和最小公倍数
#include <iostream>
using namespace std;
int main()
{
int m, n,i,GCD,LCM;
cin >> m;
cin >> n;
GCD = 0;
for (i = 1; i < m + 1; i++) {
if (m % i == 0)
if (n % i == 0)
GCD = i;
}
LCM = (m * n) / GCD;
cout << "Greater common divisor(m&n):"<<GCD<<'\n';
cout << "Least common multiple(m&n):"<<LCM<<endl;
return 0;
}
3.16 统计输入各类型字符数量
#include <iostream>
using namespace std;
int main()
{
int Alphabet=0,Space=0,Number=0,Others=0;
char c;
while ((c = getchar()) != '\n') {//这个思路例3.15提到过
if ((c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z'))
Alphabet += 1;
else if (c == 32)
Space += 1;
else if (c >= '0' && c <= '9')
Number += 1;
else
Others += 1;
}
cout << "Alphabet:"<<Alphabet<<'\n';
cout << "Space:" << Space << '\n';
cout << "Number:" << Number << '\n';
cout << "Others:" << Others << endl;
return 0;
}
/*Test Result
I am R2D2!
Alphabet:5
Space : 2
Number : 2
Others : 1*/
3.17 自定义多位数求和
#include <iostream>
using namespace std;
int main()
{
int a,i,n,Sn=0;
cin >> a;//数字
if(a>9)
return 0;
cin >> n;//最高位数
for (i = n; i > 0; i--) {
Sn += a * i;
a = a * 10;
}
cout << Sn << endl;
return 0;
}
3.18 阶乘求和
#include <iostream>
using namespace std;
int main()
{
int a=1,i,j,n,Sn=0;
cin >> n;//n=20
for (j = n; j > 0; j--) {
for (i = 1; i <= j; i++) {//注意循环次数
a = a* i;
}
Sn += a;
a = 1;
}
cout << Sn << endl;
return 0;
}
/*
20
268040729
*/
F9标记断点,F10单步执行,进行调试。
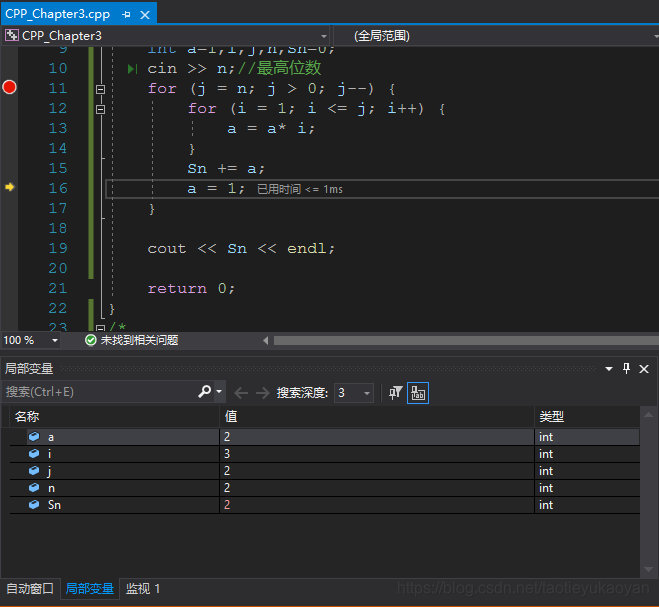
3.19 水仙花数
#include <iostream>
#include <cmath>
using namespace std;
int main()
{
int x,d1,d2,d3;
for (x = 100; x <= 999;x++) {
d1 = x / 100;
d2 = (x / 10) % 10;
d3 = x % 10;
if (x == (pow(d1,3) + pow(d2,3) + pow(d3,3))) {//pow由cmath提供,用于计算幂
cout << x <<'\t';
}
}
return 0;
}
3.20 求1000以内的完数
#include <iostream>
using namespace std;
int main()
{
int x,i,n=0;
for (x = 1; x <= 1000; x++) {
for (i = 1; i < x; i++) {
if (x % i == 0)
n += i;
}
if (x == n) {
cout << x << ",its factors are 1";
for (i = 2; i < x; i++) {
if (x % i == 0)
cout << "," << i;
}
cout << endl;
}
n = 0;
}
return 0;
}
/* Test results
6,its factors are 1,2,3
28,its factors are 1,2,4,7,14
496,its factors are 1,2,4,8,16,31,62,124,248
*/
3.21 分数序列求和
#include <iostream>
using namespace std;
int main()
{
int x=20, i;
double n = 1.0;
for (i = 1; i <= x; i++) {
n += ((n + i) / n);
}
cout << n << endl;
return 0;
}
/*33.898*/
3.22 猴子吃桃
#include <iostream>
using namespace std;
int main()
{
int i,n=1;
for (i = 1; i < 10; i++) {
n = n * 2 + 1;
}
cout << n << endl;
return 0;
}
/*1023*/
3.23 迭代法开根号
#include <iostream>
#include <cmath>
using namespace std;
int main()
{
double a=0,temp=a,x=1;
cin >> a;
while (fabs(temp-x) > 1e-5) {
temp = x;
x = (1.0 / 2.0) * (temp + (a / temp));
}
cout << x << endl;
return 0;
}
/*Test results
2
1.41421
3
1.73205
5
2.23607
*/
3.24 打印图形
#include <iostream>
using namespace std;
int main()
{
int i=0,n=0;
for (n = 1; n <= 4; n++) {
for (i = 1; i <= n + n - 1; i++) {
cout << "* ";
}
cout << '\n';
}
for (n = n-2; n > 0; n--) {
for (i = 1; i <= n + n - 1; i++) {
cout << "* ";
}
cout << '\n';
}
return 0;
}
3.25 比赛名单
#include <iostream>
using namespace std;
int main()
{
char i, j, k;
for (k = 'X'; k <= 'Z'; k++) {
for (i = 'X'; i <= 'Z'; i++) {
if (i != k) {//i与k不能相同
for (j = 'X'; j <= 'Z'; j++) {
if (i != j && j != k) {//j与i与k不能相同
if ((i != 'X') && (k != 'X') && (k != 'Z'))
cout << "A--" << i << '\n'
<< "B--" << j << '\n'
<< "C--" << k << endl;
}
}
}
}
}
return 0;
}
完全依靠条件判断得出结论