SPL06-001与SPL06-007的区别及驱动程序
网上搜了好久,关于这两个芯片的资料很少,对比发现两个只相差SPI驱动,SPL06-001只有IIC方式驱动,SPL06-007多了一个SPI驱动方式 ,其它完全一样。
画封装的时候注意那个外壳上的气孔与底板的MARK点不是同一个位置,而且在对角位置上,千万不要搞错了。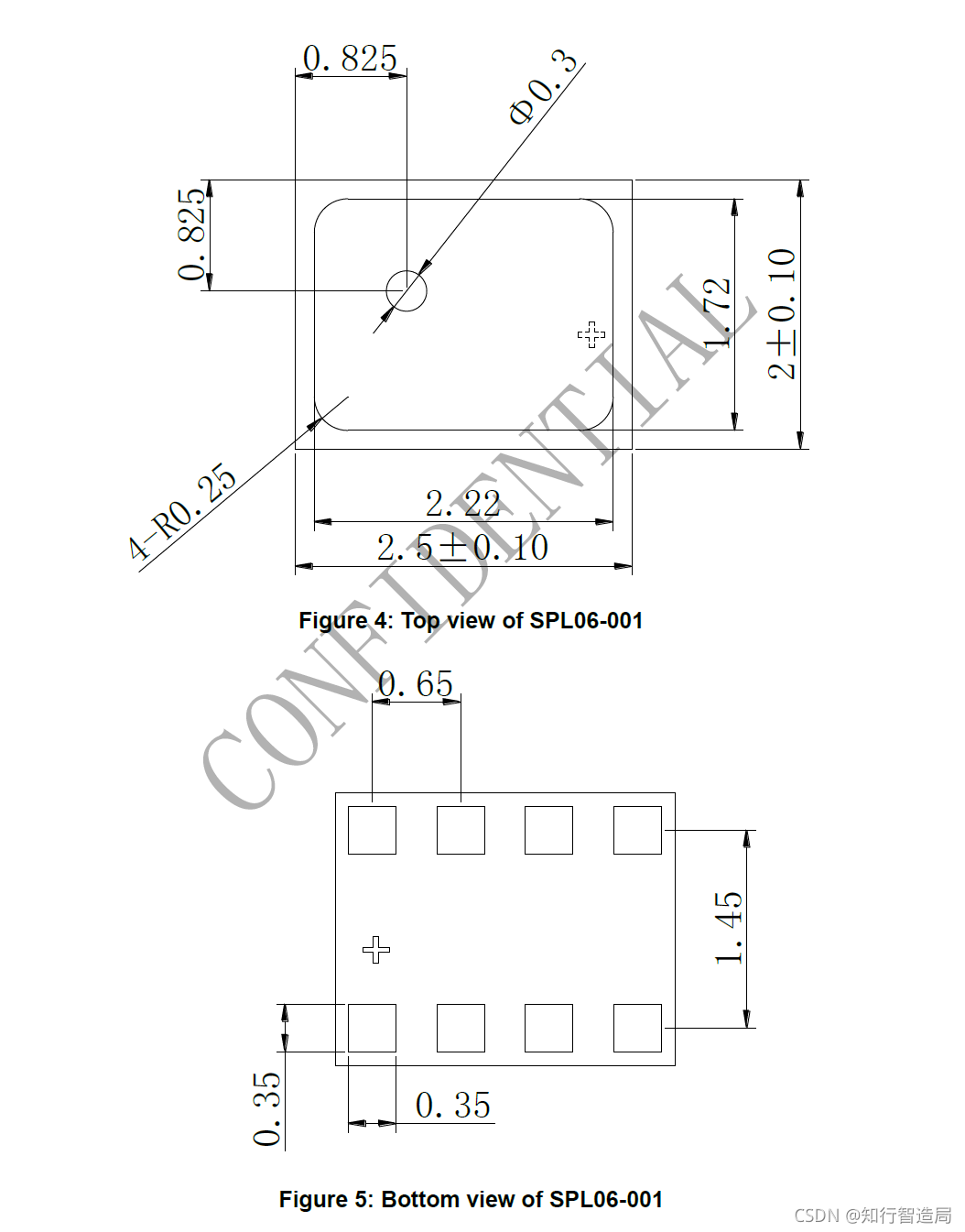
放置的位置注意不要靠近发热大的芯片周围,否则测量的就是板上的温度,高很多,有条件的可以在周围开隔离孔,
软件上如果是用IIC驱动两者是一样的,github有个库写的很好,直接使用
https://github.com/rv701/SPL06-007/blob/master/src/SPL06-007.cpp
SPL06-007.h文件
#include "Arduino.h"
void SPL_init();
uint8_t get_spl_id(); // Get ID Register 0x0D
uint8_t get_spl_prs_cfg(); // Get PRS_CFG Register 0x06
uint8_t get_spl_tmp_cfg(); // Get TMP_CFG Register 0x07
uint8_t get_spl_meas_cfg(); // Get MEAS_CFG Register 0x08
uint8_t get_spl_cfg_reg(); // Get CFG_REG Register 0x09
uint8_t get_spl_int_sts(); // Get INT_STS Register 0x0A
uint8_t get_spl_fifo_sts(); // Get FIFO_STS Register 0x0B
double get_altitude(double pressure, double seaLevelhPa); // get altitude in meters
double get_altitude_f(double pressure, double seaLevelhPa); // get altitude in feet
int32_t get_traw();
double get_traw_sc();
double get_temp_c();
double get_temp_f();
double get_temperature_scale_factor();
int32_t get_praw();
double get_praw_sc();
double get_pcomp();
double get_pressure_scale_factor();
double get_pressure();
int16_t get_c0();
int16_t get_c1();
int32_t get_c00();
int32_t get_c10();
int16_t get_c01();
int16_t get_c11();
int16_t get_c20();
int16_t get_c21();
int16_t get_c30();
void i2c_eeprom_write_uint8_t( uint8_t deviceaddress, uint8_t eeaddress, uint8_t data );
uint8_t i2c_eeprom_read_uint8_t( uint8_t deviceaddress, uint8_t eeaddress );
SPL06-007.cpp文件
`#include "SPL06-007.h"
#include "Wire.h"
uint8_t SPL_CHIP_ADDRESS = 0x76;
void SPL_init()
{
i2c_eeprom_write_uint8_t(SPL_CHIP_ADDRESS, 0X06, 0x03); // Pressure 8x oversampling
i2c_eeprom_write_uint8_t(SPL_CHIP_ADDRESS, 0X07, 0X83); // Temperature 8x oversampling
i2c_eeprom_write_uint8_t(SPL_CHIP_ADDRESS, 0X08, 0B0111); // continuous temp and pressure measurement
i2c_eeprom_write_uint8_t(SPL_CHIP_ADDRESS, 0X09, 0X00); // FIFO Pressure measurement
}
uint8_t get_spl_id()
{
return i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0x0D);
}
uint8_t get_spl_prs_cfg()
{
return i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0x06);
}
uint8_t get_spl_tmp_cfg()
{
return i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0x07);
}
uint8_t get_spl_meas_cfg()
{
return i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0x08);
}
uint8_t get_spl_cfg_reg()
{
return i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0x09);
}
uint8_t get_spl_int_sts()
{
return i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0x0A);
}
uint8_t get_spl_fifo_sts()
{
return i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0x0B);
}
double get_altitude(double pressure, double seaLevelhPa) {
double altitude;
altitude = 44330 * (1.0 - pow(pressure / seaLevelhPa, 0.1903));
return altitude;
}
double get_altitude_f(double pressure, double seaLevelhPa)
{
double altitude;
altitude = 44330 * (1.0 - pow(pressure / seaLevelhPa, 0.1903));
return altitude * 3.281;
}
double get_traw_sc()
{
int32_t traw = get_traw();
return (double(traw)/get_temperature_scale_factor());
}
double get_temp_c()
{
int16_t c0,c1;
c0 = get_c0();
c1 = get_c1();
double traw_sc = get_traw_sc();
return (double(c0) * 0.5f) + (double(c1) * traw_sc);
}
double get_temp_f()
{
int16_t c0,c1;
c0 = get_c0();
c1 = get_c1();
double traw_sc = get_traw_sc();
return (((double(c0) * 0.5f) + (double(c1) * traw_sc)) * 9/5) + 32;
}
double get_temperature_scale_factor()
{
double k;
uint8_t tmp_Byte;
tmp_Byte = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X07); // MSB
//Serial.print("tmp_Byte: ");
//Serial.println(tmp_Byte);
//tmp_Byte = tmp_Byte >> 4; //Focus on bits 6-4
tmp_Byte = tmp_Byte & 0B00000111;
//Serial.print("tmp_Byte: ");
//Serial.println(tmp_Byte);
switch (tmp_Byte)
{
case 0B000:
k = 524288.0;
break;
case 0B001:
k = 1572864.0;
break;
case 0B010:
k = 3670016.0;
break;
case 0B011:
k = 7864320.0;
break;
case 0B100:
k = 253952.0;
break;
case 0B101:
k = 516096.0;
break;
case 0B110:
k = 1040384.0;
break;
case 0B111:
k = 2088960.0;
break;
}
return k;
}
int32_t get_traw()
{
int32_t tmp;
uint8_t tmp_MSB,tmp_LSB,tmp_XLSB;
tmp_MSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X03); // MSB
tmp_LSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X04); // LSB
tmp_XLSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X05); // XLSB
tmp = (tmp_MSB << 8) | tmp_LSB;
tmp = (tmp << 8) | tmp_XLSB;
if(tmp & (1 << 23))
tmp = tmp | 0XFF000000; // Set left bits to one for 2's complement conversion of negitive number
return tmp;
}
double get_praw_sc()
{
int32_t praw = get_praw();
return (double(praw)/get_pressure_scale_factor());
}
double get_pcomp()
{
int32_t c00,c10;
int16_t c01,c11,c20,c21,c30;
c00 = get_c00();
c10 = get_c10();
c01 = get_c01();
c11 = get_c11();
c20 = get_c20();
c21 = get_c21();
c30 = get_c30();
double traw_sc = get_traw_sc();
double praw_sc = get_praw_sc();
return double(c00) + praw_sc * (double(c10) + praw_sc * (double(c20) + praw_sc * double(c30))) + traw_sc * double(c01) + traw_sc * praw_sc * ( double(c11) + praw_sc * double(c21));
}
double get_pressure()
{
double pcomp = get_pcomp();
return pcomp / 100; // convert to mb
}
double get_pressure_scale_factor()
{
double k;
uint8_t tmp_Byte;
tmp_Byte = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X06); // MSB
tmp_Byte = tmp_Byte & 0B00000111; // Focus on 2-0 oversampling rate
switch (tmp_Byte) // oversampling rate
{
case 0B000:
k = 524288.0;
break;
case 0B001:
k = 1572864.0;
break;
case 0B010:
k = 3670016.0;
break;
case 0B011:
k = 7864320.0;
break;
case 0B100:
k = 253952.0;
break;
case 0B101:
k = 516096.0;
break;
case 0B110:
k = 1040384.0;
break;
case 0B111:
k = 2088960.0;
break;
}
return k;
}
int32_t get_praw()
{
int32_t tmp;
uint8_t tmp_MSB,tmp_LSB,tmp_XLSB;
tmp_MSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X00); // MSB
tmp_LSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X01); // LSB
tmp_XLSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X02); // XLSB
tmp = (tmp_MSB << 8) | tmp_LSB;
tmp = (tmp << 8) | tmp_XLSB;
if(tmp & (1 << 23))
tmp = tmp | 0XFF000000; // Set left bits to one for 2's complement conversion of negitive number
return tmp;
}
int16_t get_c0()
{
int16_t tmp;
uint8_t tmp_MSB,tmp_LSB;
tmp_MSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X10);
tmp_LSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X11);
tmp_LSB = tmp_LSB >> 4;
tmp = (tmp_MSB << 4) | tmp_LSB;
if(tmp & (1 << 11)) // Check for 2's complement negative number
tmp = tmp | 0XF000; // Set left bits to one for 2's complement conversion of negitive number
return tmp;
}
int16_t get_c1()
{
int16_t tmp;
uint8_t tmp_MSB,tmp_LSB;
tmp_MSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X11);
tmp_LSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X12);
tmp_MSB = tmp_MSB & 0XF;
tmp = (tmp_MSB << 8) | tmp_LSB;
if(tmp & (1 << 11)) // Check for 2's complement negative number
tmp = tmp | 0XF000; // Set left bits to one for 2's complement conversion of negitive number
return tmp;
}
int32_t get_c00()
{
int32_t tmp;
uint8_t tmp_MSB,tmp_LSB,tmp_XLSB;
tmp_MSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X13);
tmp_LSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X14);
tmp_XLSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X15);
tmp_XLSB = tmp_XLSB >> 4;
tmp = (tmp_MSB << 8) | tmp_LSB;
tmp = (tmp << 4) | tmp_XLSB;
tmp = (uint32_t)tmp_MSB << 12 | (uint32_t)tmp_LSB << 4 | (uint32_t)tmp_XLSB >> 4;
if(tmp & (1 << 19))
tmp = tmp | 0XFFF00000; // Set left bits to one for 2's complement conversion of negitive number
return tmp;
}
int32_t get_c10()
{
int32_t tmp;
uint8_t tmp_MSB,tmp_LSB,tmp_XLSB;
tmp_MSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X15); // 4 bits
tmp_LSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X16); // 8 bits
tmp_XLSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X17); // 8 bits
tmp_MSB = tmp_MSB & 0b00001111;
tmp = (tmp_MSB << 4) | tmp_LSB;
tmp = (tmp << 8) | tmp_XLSB;
tmp = (uint32_t)tmp_MSB << 16 | (uint32_t)tmp_LSB << 8 | (uint32_t)tmp_XLSB;
if(tmp & (1 << 19))
tmp = tmp | 0XFFF00000; // Set left bits to one for 2's complement conversion of negitive number
return tmp;
}
int16_t get_c01()
{
int16_t tmp;
uint8_t tmp_MSB,tmp_LSB;
tmp_MSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X18);
tmp_LSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X19);
tmp = (tmp_MSB << 8) | tmp_LSB;
return tmp;
}
int16_t get_c11()
{
int16_t tmp;
uint8_t tmp_MSB,tmp_LSB;
tmp_MSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X1A);
tmp_LSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X1B);
tmp = (tmp_MSB << 8) | tmp_LSB;
return tmp;
}
int16_t get_c20()
{
int16_t tmp;
uint8_t tmp_MSB,tmp_LSB;
tmp_MSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X1C);
tmp_LSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X1D);
tmp = (tmp_MSB << 8) | tmp_LSB;
return tmp;
}
int16_t get_c21()
{
int16_t tmp;
uint8_t tmp_MSB,tmp_LSB;
tmp_MSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X1E);
tmp_LSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X1F);
tmp = (tmp_MSB << 8) | tmp_LSB;
return tmp;
}
int16_t get_c30()
{
int16_t tmp;
uint8_t tmp_MSB,tmp_LSB;
tmp_MSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X20);
tmp_LSB = i2c_eeprom_read_uint8_t(SPL_CHIP_ADDRESS, 0X21);
tmp = (tmp_MSB << 8) | tmp_LSB;
return tmp;
Serial.print("tmp: ");
Serial.println(tmp);
}
void i2c_eeprom_write_uint8_t( uint8_t deviceaddress, uint8_t eeaddress, uint8_t data )
{
uint8_t rdata = data;
delay(5); // Make sure to delay log enough for EEPROM I2C refresh time
Wire.beginTransmission(deviceaddress);
Wire.write((uint8_t)(eeaddress));
Wire.write(rdata);
Wire.endTransmission();
}
uint8_t i2c_eeprom_read_uint8_t( uint8_t deviceaddress, uint8_t eeaddress )
{
uint8_t rdata = 0xFF;
Wire.beginTransmission(deviceaddress);
Wire.write(eeaddress);
Wire.endTransmission(false); // false to not release the line
Wire.requestFrom(deviceaddress,1);
if (Wire.available()) rdata = Wire.read();
return rdata;
}
使用非常简单
#include <Wire.h>
void setup() {
Wire.begin(); // begin Wire(I2C)
Serial.begin(115200); // begin Serial
Serial.println("\nGoertek-SPL06-007 Demo\n");
SPL_init(); // Setup initial SPL chip registers
}
void loop() {
// ---- Register Values ----------------
Serial.print("ID: ");
Serial.println(get_spl_id());
Serial.print("PRS_CFG: ");
Serial.println(get_spl_prs_cfg(),BIN);
Serial.print("TMP_CFG: ");
Serial.println(get_spl_tmp_cfg(),BIN);
Serial.print("MEAS_CFG: ");
Serial.println(get_spl_meas_cfg(),BIN);
Serial.print("CFG_REG: ");
Serial.println(get_spl_cfg_reg(),BIN);
Serial.print("INT_STS: ");
Serial.println(get_spl_int_sts(),BIN);
Serial.print("FIFO_STS: ");
Serial.println(get_spl_fifo_sts(),BIN);
// ---- Coefficients ----------------
Serial.print("c0: ");
Serial.println(get_c0());
Serial.print("c1: ");
Serial.println(get_c1());
Serial.print("c00: ");
Serial.println(get_c00());
Serial.print("c10: ");
Serial.println(get_c10());
Serial.print("c01: ");
Serial.println(get_c01());
Serial.print("c11: ");
Serial.println(get_c11());
Serial.print("c20: ");
Serial.println(get_c20());
Serial.print("c21: ");
Serial.println(get_c21());
Serial.print("c30: ");
Serial.println(get_c30());
// ---- Temperature Values ----------------
Serial.print("traw: ");
Serial.println(get_traw());
Serial.print("traw_sc: ");
Serial.println(get_traw_sc(),3);
Serial.print("Temperature: ");
Serial.print(get_temp_c());
Serial.println(" C");
Serial.print("Temperature: ");
Serial.print(get_temp_f());
Serial.println(" F");
// ---- Pressure Values ----------------
Serial.print("praw: ");
Serial.println(get_praw());
Serial.print("praw_sc: ");
Serial.println(get_praw_sc(),3);
Serial.print("pcomp: ");
Serial.println(get_pcomp(),2);
Serial.print("Measured Air Pressure: ");
Serial.print(get_pressure(),2);
Serial.println(" mb");
// ---- Altitude Values ----------------
double local_pressure = 1011.3; // Look up local sea level pressure on google // Local pressure from airport website 8/22
Serial.print("Local Airport Sea Level Pressure: ");
Serial.print(local_pressure,2);
Serial.println(" mb");
Serial.print("altitude: ");
Serial.print(get_altitude(get_pressure(),local_pressure),1);
Serial.println(" m");
Serial.print("altitude: ");
Serial.print(get_altitude_f(get_pressure(),local_pressure),1); // convert from meters to feet
Serial.println(" ft");
Serial.println("\n");
delay(2000);
}
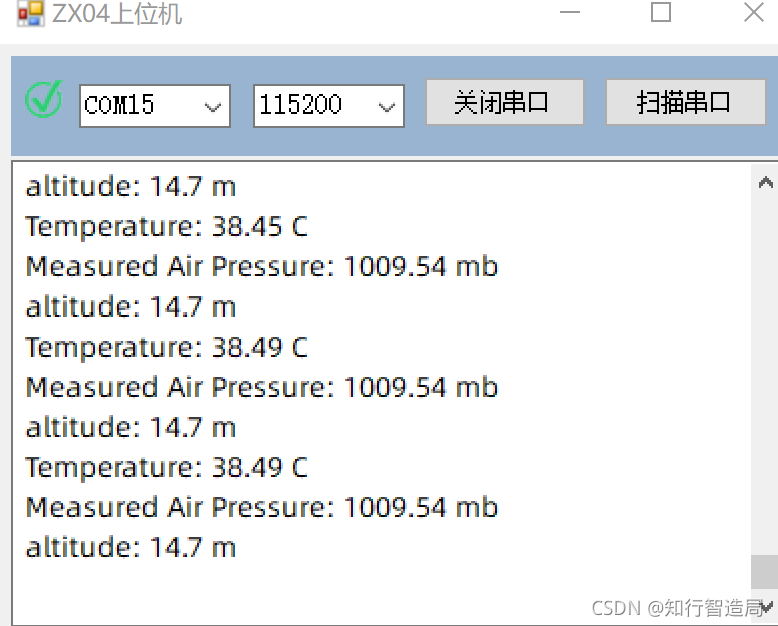