/*统计一个Byte中1的个数,算法尽可能高性能*/
#include <iostream>
using namespace std;
//方法1:对2取模得出最后一位,然后除以2实现右移1位,循环8次
int count1(unsigned char c)
{
int cnt=0;
for (int i=0;i!=8;++i)
{
cnt+=c%2;
c=c/2;
}
return cnt;
}
//方法2:对0x01执行&操作,统计最右边一位的1,然后右移1位,循环8次,o(n)复杂度
int count2(unsigned char c)
{
int cnt=0;
for (int i=0;i!=8;++i)
{
cnt+=(c&0x01);
c>>=1;
}
return cnt;
}
//方法3:c=c&(c-1),只统计c中1的个数,循环次数是字节中1的个数,效率比方法2高
int count3(unsigned char c)
{
int cnt=0;
while (c!=0)
{
c&=(c-1);
++cnt;
}
return cnt;
}
//方法4:查表法,建立一个含有256个整数的数组,每个整数值代表的是c所在的位置含有1的个数
//时间复杂度o(1),空间复杂度o(2^n)
int countTable[256]=
{
0,1,1,2,1,2,2,3,1,2,2,3,2,3,3,4,
1,2,2,3,2,3,3,4,2,3,3,4,3,4,4,5,
1,2,2,3,2,3,3,4,2,3,3,4,3,4,4,5,
2,3,3,4,3,4,4,5,3,4,4,5,4,5,
统计一个Byte中1的个数,算法尽可能高性能——C++实现
最新推荐文章于 2023-07-08 20:24:47 发布
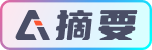