class gradapa(object):
def __init__(self, money):
self.money = money
print("gradapa money:",money)
def p(self):
print("this is gradapa")
class father(gradapa):
def __init__(self, money, job):
super().__init__(money*3)
self.job = job
print("father money:", money)
print("father job:",self.job)
def p(self):
print("this is father,我重写了父类的方法")
class mother(gradapa):
def __init__(self, money, job):
super().__init__(money*2)
self.job = job
print("mother money:", money)
print("mother job:",self.job)
def p(self):
print("this is mother,我重写了父类的方法")
return 100
def fc(obj):
obj.p()
gradapa1 = gradapa(9000)
father1 = father(5000, "工人")
mother1 = mother(1000, "老师")
fc(gradapa1)
fc(father1)
fc(mother1)
print(fc(mother1))
参考1
class A():
def __init__(self):
print('init A...')
print('end A...')
class B(A):
def __init__(self):
print('init B...')
A.__init__(self)
print('end B...')
class C(A):
def __init__(self):
print('init C...')
A.__init__(self)
print('end C...')
class D(B, C):
def __init__(self):
print('init D...')
B.__init__(self)
C.__init__(self)
print('end D...')
if __name__ == '__main__':
D()
参考2
a_list=[1,2,3]
b_list=['one','two','three']
result=zip(a_list,b_list)
print(set(result))
print(list(result))
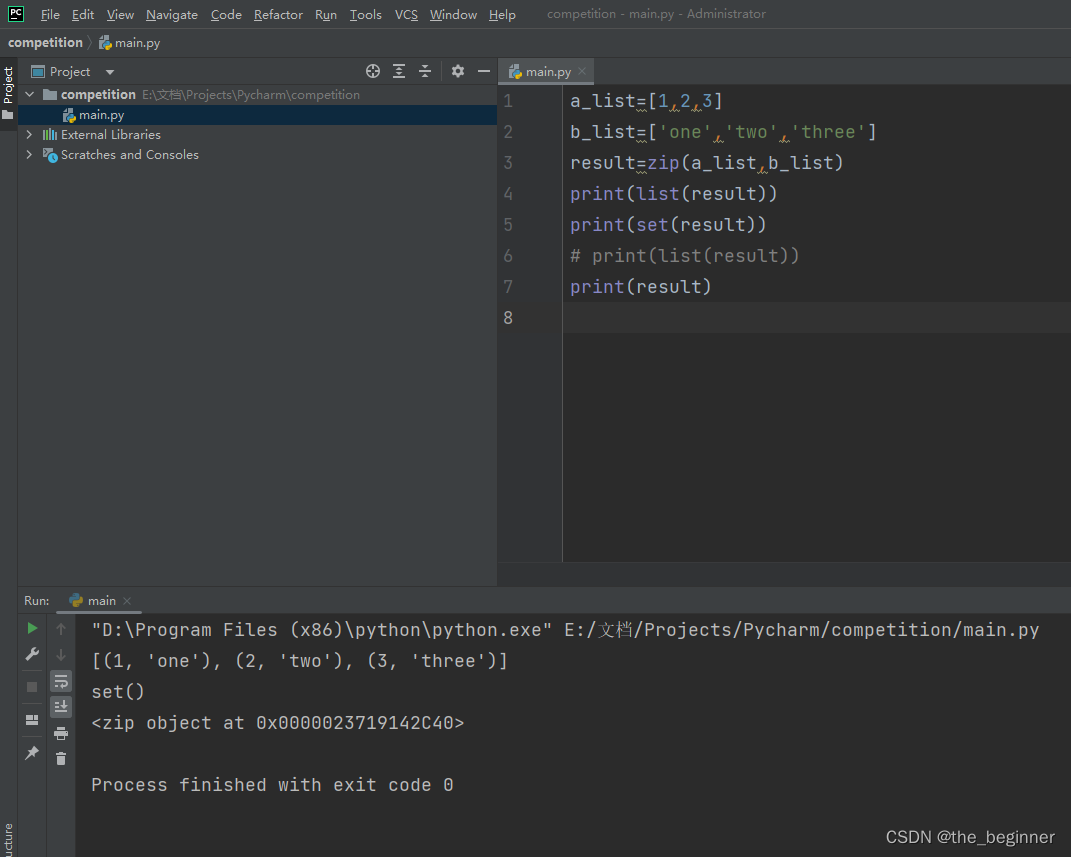
print([1,2,3]*3)
==优先级大于=
x=3 == 5,888,555,9999
print(x)
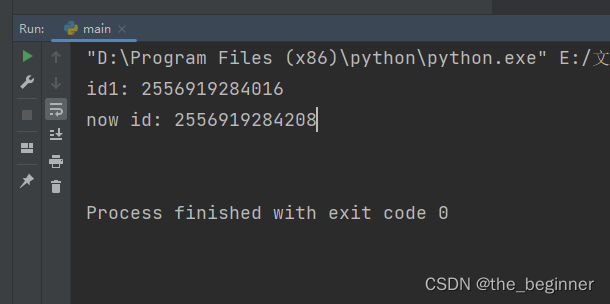
x=3
print("id1:",id(x))
x+=6
print("now id:",id(x))
print()
def add(n):
return lambda x:x+n
f=add(1)
print(f(2))
strs=' I like python '
one=strs.split(' ')
two=strs.split()
print(one)
print(two)
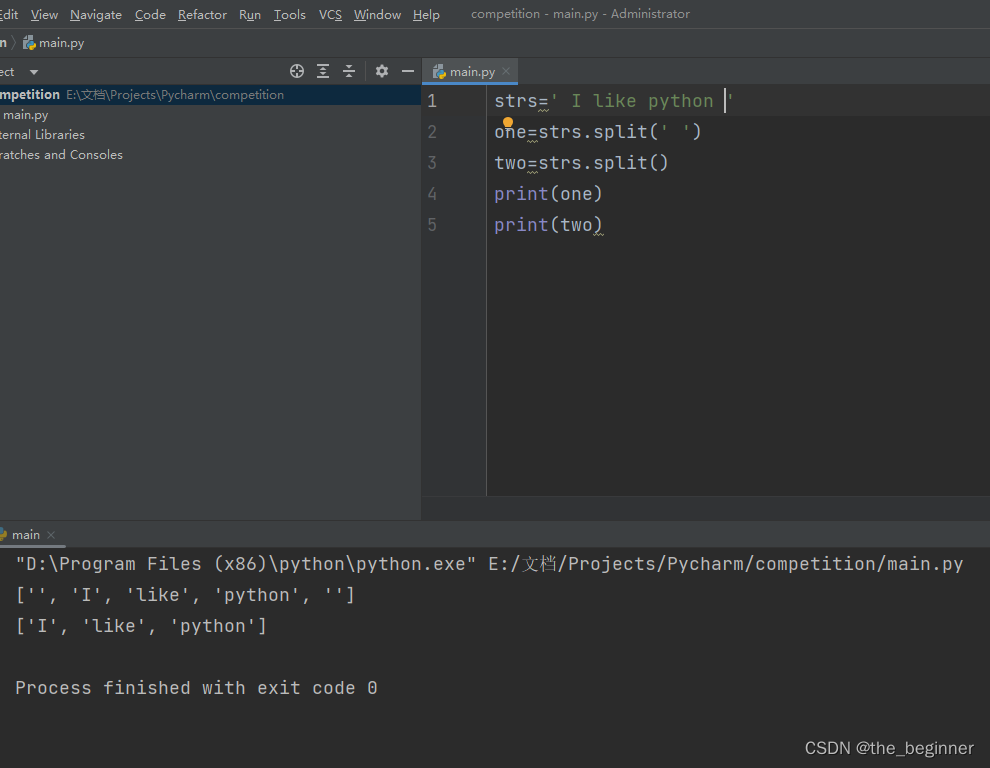
strs=' I like python '
one=strs.split(' ')
two=strs.split()
print(one)
print(two)
s= 'www.dod.com.cn'
print(s.split())
print(s.split('.'))
print(s.split('.',1))
print(s.split('.',2))
print(s.split('.',2)[1])
print(s.split('.',-1))
s1,s2,s3= s.split('.',2)
print(s1)
print(s3)
print(s2)
c = '''hello
world'''
print(c)
print(c.split('\n'))
print(c.split('\t'))
import os
print(os.path.split('/dodo/soft/python/'))
print(os.path.split('/dodo/soft/python'))
a= 'hello boy<[www.dodo.com.cn]>byebye'
print(a.split('['))
print(a.split('[')[1].split(']')[0])
print(a.split('[')[1].split(']')[0].split('.'))

x={i:str(i+3)for i in range(2)}
print(x)
print(sum(x))
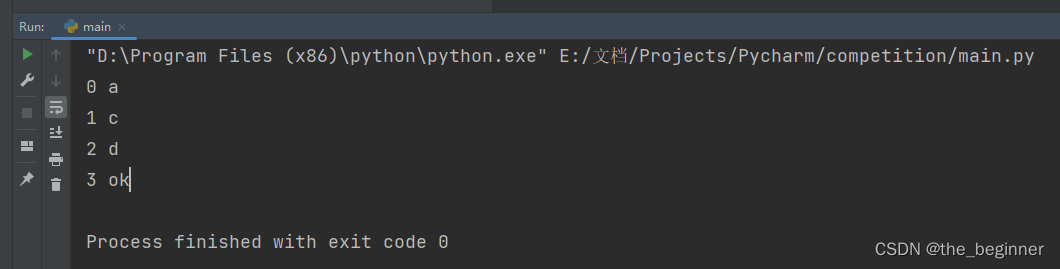
a = ['a', 'c', 'd', 'ok']
for index, item in enumerate(a):
print(index, item)

list1 = [1,2,4,"hello","xy","你好"]
a = list1.pop()
print(a,list1)