import java.util.Arrays;
import java.util.List;
public class GenerateColumns {
public static final String DOT = ".";
public static final String BLANK = " ";
public static final String COMMA = ",";
public static final String UNDERLINE = "_";
public static String originalColumns = "EMPNO,ENAME,JOB,MGR,HIREDATE,SAL,COMM,DEPTNO";
public static String newColumns = "E_EMPNO,E_ENAME,E_JOB,E_MGR,E_HIREDATE,E_SAL,E_COMM,E_DEPTNO";
public static void main(String[] args) {
String sql = generateSql("EMP", "E");
System.out.println(sql);
String xmlStr = generateMyBatisXml(newColumns);
System.out.println(xmlStr);
}
public static String generateMyBatisXml(String newColumns) {
String xmlStr = "";
String[] originalColumnArr = originalColumns.split(",");
String[] newColumnArr = newColumns.split(",");
List<String> newColumnList = Arrays.asList(newColumnArr);
for(int i = 0; i < newColumnList.size(); i++) {
String currentXmlStr = null;
if(i == 0) {
currentXmlStr = "<id column=\""
+ newColumnList.get(i).toUpperCase()
+ "\""
+ BLANK
+ "jdbcType=\"VARCHAR\""
+ BLANK
+ "property=\""
+ generatePropertyName(originalColumnArr[i])
+ "\"/>"
+ "\r\n";
}else {
currentXmlStr = "<result column=\""
+ newColumnList.get(i).toUpperCase()
+ "\""
+ BLANK
+ "jdbcType=\"VARCHAR\""
+ BLANK
+ "property=\""
+ generatePropertyName(originalColumnArr[i])
+ "\"/>"
+ "\r\n";
}
xmlStr += currentXmlStr;
}
return xmlStr;
}
public static String generatePropertyName(String columnName) {
String propertyName = "";
String[] columnNameArr = null;
if(columnName.indexOf(UNDERLINE) > 0) {
columnNameArr = columnName.split(UNDERLINE);
if(columnNameArr != null) {
for(int i = 0; i < columnNameArr.length; i++) {
String currentStr = columnNameArr[i];
if(i == 0) {
currentStr = currentStr.toLowerCase();
}else {
if(currentStr.length() > 0) {
currentStr = currentStr.toLowerCase();
currentStr = currentStr.substring(0, 1).toUpperCase() + currentStr.substring(1);
}
}
propertyName += currentStr;
}
}
}else {
propertyName = columnName.toLowerCase();
}
return propertyName;
}
public static String generateSql(String tableName, String tableAbbr){
String sql = "SELECT" + BLANK;
String[] originalColumnArr = originalColumns.split(COMMA);
List<String> originalColumnList = Arrays.asList(originalColumnArr);
String[] newColumnArr = newColumns.split(COMMA);
List<String> newColumnList = Arrays.asList(newColumnArr);
if(originalColumnList.size() == newColumnList.size()) {
for(int i = 0; i < originalColumnList.size(); i++) {
sql += tableAbbr.toUpperCase() + DOT
+ originalColumnList.get(i).toUpperCase()
+ BLANK
+ newColumnList.get(i).toUpperCase()
+ COMMA;
}
sql = sql.substring(0, sql.length() - 1);
sql += BLANK + "FROM" + BLANK + tableName.toUpperCase()
+ BLANK
+ tableAbbr.toUpperCase();
}
return sql;
}
}
以上是代码,运行结果展示如下:
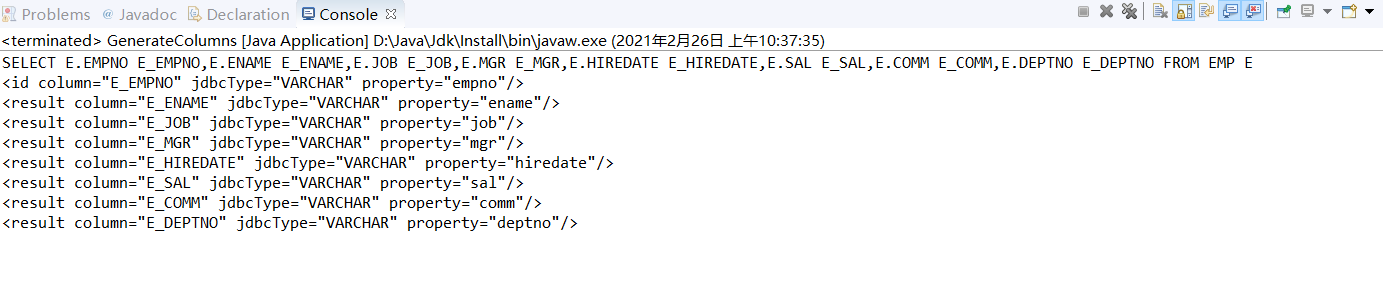
最后修改时间:2021年02月26日 10:38