个人博客地址:https://travis1024.github.io/
简单数据结构-堆栈模拟
1. 问题描述
编写一个程序模拟堆栈,要求能够模拟、入栈、出栈、返回栈顶元素等基本操作。栈中元素可用整数代替。不能使用C++模板库预定义的类型。程序运行中可输入多组入栈、出栈操作,每次操作后展示栈中元素。
2. 问题分析
- 考虑可以模拟栈执行及功能实现的数据结构;
2) 栈主要功能元素出栈及入栈的实现方法;
3. 处理方法设计(算法步骤)
- 使用结构体模拟堆栈中元素的存储,其中array模拟线性的堆栈,top指向array中现有元素的位置,当有元素入栈的时候,top的位置首先加入一个元素,然后top++,也就是说top始终指向栈顶元素的上一个位置,栈中的元素个数就是top+1,当有元素出栈的时候,top–;
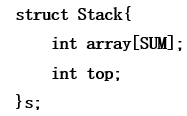
- 首先对结构体中的top进行初始化为-1;随后进行功能的选择;
4.测试数据设计
- 首先向堆栈中依次push 5个元素[1,2,3,4,5];
- 然后依次执行:pop栈顶元素,查看栈顶元素,栈中元素的数量,判断栈是否为空,判断栈是否已满,打印栈中的元素;
5.测试结果分析
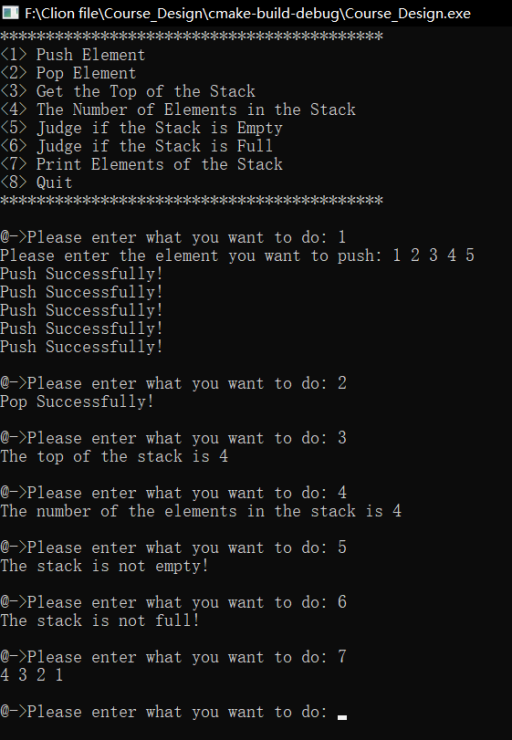
6.程序结构
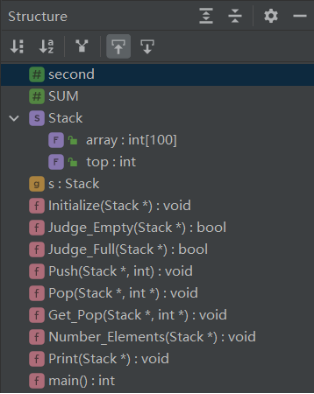
7.源代码
#include <iostream>
#include <sstream>
#include <stdio.h>
#define SUM 100
using namespace std;
struct Stack{
int array[SUM];
int top;
}s;
void Initialize(Stack * s){
s->top=-1;
}
bool Judge_Empty(Stack * s){
if(s->top==-1) return true;
else return false;
}
bool Judge_Full(Stack * s){
if(s->top==SUM-1) return true;
else return false;
}
void Push(Stack * s,int a){
if(Judge_Full(s))
cout<<"Failure to Push!"<<endl;
else{
s->top++;
s->array[s->top]=a;
cout<<"Push Successfully!"<<endl;
}
}
void Pop(Stack * s,int *a){
if(Judge_Empty(s))
cout<<"Failure to Pop!"<<endl;
else{
*a=s->array[s->top];
s->top--;
cout<<"Pop Successfully!"<<endl;
}
}
void Get_Pop(Stack * s,int *a){
if(Judge_Empty(s))
cout<<"Failure!"<<endl;
else{
*a=s->array[s->top];
cout<<"The top of the stack is "<<*a<<endl;
}
}
void Number_Elements(Stack * s){
cout<<"The number of the elements in the stack is "<<s->top+1<<endl;
}
void Print(Stack * s){
int now=s->top;
if(Judge_Empty(s)) cout<<"The stack is empty!"<<endl;
while(!Judge_Empty(s)){
int i=s->array[s->top];
s->top--;
cout<<i<<" ";
}
cout<<endl;
s->top=now;
}
int main(){
int a,index,t;
Initialize(&s);
cout<<"******************************************"<<endl;
cout<<"<1> Push Element"<<endl;
cout<<"<2> Pop Element"<<endl;
cout<<"<3> Get the Top of the Stack"<<endl;
cout<<"<4> The Number of Elements in the Stack"<<endl;
cout<<"<5> Judge if the Stack is Empty"<<endl;
cout<<"<6> Judge if the Stack is Full"<<endl;
cout<<"<7> Print Elements of the Stack"<<endl;
cout<<"<8> Quit"<<endl;
cout<<"******************************************"<<endl;
while (index!=8){
cout<<endl;
cout<<"@->Please enter what you want to do: ";
cin>>index;
switch (index) {
case 1:{
cout<<"Please enter the element you want to push: ";
while(cin>>t){
Push(&s,t);
char ch=getchar();
if(ch=='\n') break;
}
break;
}
case 2:Pop(&s,&a);break;
case 3:Get_Pop(&s,&a);break;
case 4:Number_Elements(&s);break;
case 5:{
bool PD=Judge_Empty(&s);
if(PD) cout<<"The stack is empty!"<<endl;
else cout<<"The stack is not empty!"<<endl;
break;
}
case 6:{
bool PD=Judge_Full(&s);
if(PD) cout<<"The stack is full!"<<endl;
else cout<<"The stack is not full!"<<endl;
break;
}
case 7:Print(&s);break;
case 8:return 0;
default:cout<<"Input Error!"<<endl;
}
}
return 0;
}