JS [A]
JavaScript
JavaScript 1: JS Intro
- DOM: Document Object Model : HTML as nodes
- BOM: Browser Object Model : Browser
- ECMA: Nombas, ScriptEase, Flash, ActionScript
<meta charset="gb2312"></meta>
<script type="text/javascript">
<!--
//js script
//-->
document.write ("hello world!");
</script>
- example 1
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>JS1</title>
<script >
document.write("name: Bob<br>");
document.write("age: 22<br>");
document.write("address: Moon<br>");
</script>
</head>
<body>
</body>
</html>
- import JS: inside <> ex: < body>; inside < script>; imported script < script src="…/xx.js">< /script>
- alert
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>js2</title>
</head>
<body onload="alert('HELLO')">
<script>
alert("HELLOO!");
</script>
<script src="demo1.js"></script>
</body>
</html>
<!--demo1.js-->
alert("HELLOOO!!");
JavaScript 2: Grammar I
- var, alert, typeof, parse
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
</body>
<script>
var a="my name is variable";//variable
var $A="MY NAME IS VARIABLE"; //var case sensitive _ $
$A="EDITED VAR";
//var declare use comma
var b="b", c="c", d="d";
var num=100;
var bool=true;
alert(b+num+c+d+bool); //also popup window
alert(a);
alert($A);
alert("hello"); //comment
prompt("enter name: ", "Name"); /*comment*/
confirm("Yes?");
var u;
alert(u);//undefined
var e=true;
var f=typeof e;
alert(f); //boolean=null, undefined is false
alert(typeof f); //string
var p="1"+2*5; //110
alert(p);
var p2=parseInt("1")+2*5 //11
alert(p2);
var p3="11.99abcde123"; //11 parsed only
alert(parseInt(p3)+22); //33
alert(parseFloat(p3)+22); //33.99
alert(Number(p)+Number(p2));//11+110=121; Nan=not a number
alert(p.toString()+p2.toString());//11110
</script>
</html>
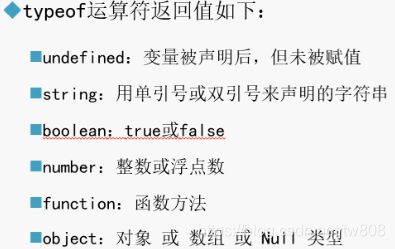
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
</body>
<script>
var a=1+2*3; //7
var b=a/2; //3.5
var c=a%2; //1
c+=1; //2
alert(c++); //2
alert(c); //3
alert(++c); //4
var bob=10;
var mary=11;
var bobAge=bob>10;
var maryAge=mary>10;
document.write ("bobAge: "+bobAge+ "<br>"); //false
document.write ("maryAge: "+maryAge+"<br>"); //true
document.write ("bob === mary "+(bob === mary)+"<br>"); //true
document.write ("bob !== mary "+(bob !== mary)+"<br>"); //true
if (maryAge){
document.write("maryAge pass 10"+"<br>");
}
if (maryAge || bobAge){
document.write("ok age for mary and bob"+"<br>")
}
var digit=12345;
document.write("num="+digit+"<br>")
var d1=digit%10;
document.write("d1: "+d1+"/1="+parseInt(d1/1)+"<br>");
var d2=digit%100;
document.write("d2: "+d2+"/10="+parseInt(d2/10)+"<br>");
var d3=digit%1000;
document.write("d3: "+d3+"/100="+parseInt(d3/100)+"<br>");
var d4=digit%10000;
document.write("d4: "+d4+"/1000="+parseInt(d4/1000)+"<br>");
var d5=digit%100000;
document.write("d5: "+d5+"/10000="+parseInt(d5/10000)+"<br>");
document.write("num="+parseInt(d5/10000).toString()+
parseInt(d4/1000).toString()+parseInt(d3/100).toString()
+parseInt(d2/10).toString()+parseInt(d1).toString()+"<br>");
</script>
</html>
- if…else if
- ? :
- switch(){ case: …break; default}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
</body>
<script>
var bool=false;
if (bool==true){
document.write("true"+"<br>");
}else{
document.write("false"+"<br>");
}
//? :
bool==true?document.write("true"+"<br>"):document.write("false"+"<br>");
//switch
var a=prompt("enter num: ");
switch(a){
case "1": alert(a);break;
case "50": alert(a); break;
case "100": alert(a); break;
default: alert("RANDOM!");}
</script>
</html>
- basic calculations
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
</body>
<script>
var num1=prompt("Enter num1: ");
var num2=prompt("Enter num2: ");
var sym=prompt("Enter symbol: ");
switch(sym){
case"+": alert(parseInt(num1)+parseInt(num2)); break;
case"-": alert(parseInt(num1)-parseInt(num2)); break;
case"*": alert(parseInt(num1)*parseInt(num2)); break;
case"/": alert(parseInt(num1)/parseInt(num2)); break;
case"%": alert(parseInt(num1)%parseInt(num2)); break;
}
</script>
</html>
- while, for, do,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
</body>
<script>
var age=1;
var middle=age<50;
while(middle){ //or do ...while
age++;
middle=age<40;
if (age==21){
continue; //skipped
}
if (age==31){
break; //get out of loop
}
document.write("Not 50 @ "+age+"<br>");
}
for(var a=1; a<50; a++){
document.write("Not 50 @ "+a+"<br>");
}
var b=[5,4,3,2,1];
for( var v in b) {
document.write(v + "<br>");
}
</script>
</html>
JavaScript 3: Grammar II
- function
- parseInt(); parseFloat(); isNan(); eval()
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
</body>
<script>
//NaN not a number
document.write(isNaN(12345)+"<br>");//false
document.write(isNaN("ABC")+"<br>");//true
//eval()
var str="alert(123)";
eval(str);
</script>
</html>
- function
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
</body>
<script>
function abc(i){
document.write(123+i+"<br>");
}
abc("a");
abc(1);
abc("ab");
//square
function sqr(num){
return num*num;
}
document.write("5^2 ="+sqr(5)+"<br>");
document.write("23^2 ="+sqr(23)+"<br>");
//hello
function hello(name){
alert(name+" says hello!");
}
hello("Mary");
hello("Bob");
//anonymous function
(function(){
alert(12345);
})()
// function as var
var fn=function(baby){
alert(baby()+"Baby"); //5Baby baby as return value
//if use baby return the whole function below as "function()..."
}
// function2
fn(function(){
alert("abc"); //abc
return 5; //
});
//function3
function words(w){
if (w=="ugly"){
return; //out of function, break
}
alert(w+w+w);
}
words("ugly");
words("beautiful");
</script>
</html>
- event (incident)
- onload
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body onload="fn()">
</body>
<script>
function fn(){
alert(888);
}
window.onload=function(){
alert(321);
}
</script>
</html>
- onfocus, onblur, onchange
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<form action="" id="form1">
<input type="text" id="hello">
<input type="text" onfocus="Hello()" >
<input type="text" onblur="Noo()" >
<input type="text" onchange="Change()" >
<input type="submit">
</form>
</body>
<script>
document.getElementById("hello").onfocus=function(){
console.log("Boo");
}
function Hello(){
//document.write("hello!");
console.log("hello!");
}
function Noo(){
//document.write("hello!");
console.log("Noo!");
}
function Change(){
//document.write("hello!");
console.log("CHANGE");
}
var form1=document.getElementById("form1");
form1.onsubmit=function(){
alert("SUBMIT");
return false;
}
</script>
</html>
- onmouseover, onmouseout, onclick, ondbclick
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1 id="h1">HELLO LOG1</h1>
<h2 id="h2" onmouseover="console.log('log h2')"
onclick="alert('h2 clicked!')">HELLO LOG2</h2>
</body>
<script>
var h1=document.getElementById("h1");
h1.onmouseover=function(){
console.log("log h1");
}
h1.onmouseout=function(){
console.log("log out h1");
}
h1.ondblclick=function(){
alert("h1 dbclicked!");
}
</script>
</html>
- onload, onsubmit
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>event1</title>
</head>
<script>
onload=function() {
var form1 = document.getElementById("form1");
form1.onsubmit = function () {
var isSubmit = confirm("submitted?")
if (isSubmit) {
return true;
}
return false;
}
}
</script>
<body>
<form action="http://bing.com" id="form1">
Title: <input type="text"><br>
Message: <textarea name="" id="" cols="30" rows="10"></textarea><br>
<input type="submit">
</form>
</body>
</html>
- debug use
- try…catch
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
</body>
<script>
try {
//alert(bbb);
console.log(123456789);
console.warn("PROBLEM!");
var a=9;
var height=20;
var result=height*a;
function sum(a, b) {
return a + b;
}
alert(sum(123, 456));
}catch(err){
console.log(err.message)
}
alert("result: "+result);
</script>
</html>
- triangles
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script type="text/javascript">
var t=prompt("enter num: ","");
if (t<=5){
console.log("less than 5");
for(var i=t; i>0; i--){
for(var j=0; j<i; j++) {
document.write("* ");
}
document.write("<br/>");
}
}
else if (t>5){
for(var i=t; i>0; i--){
for(var j=0; j<i; j++) {
document.write("* ");
}
document.write("<br/>");
}
for(var i=1; i<=t; i++){
for(var j=i; j>0; j--) {
document.write("* ");
}
document.write("<br/>");
}
}
</script>
</head>
<body style="text-align: center">
</body>
</html>
JavaScript 4: BOM & DOM
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body >
</body>
<script>
var age=44;
var height=155;
var weigth=129;
// var chat=function(){
// console.log("chatting");
// }
var person={age:44, height: 155, weight: 129,
chat:function(){console.log("chatting")},
run:function(){console.log("running")}};
alert(person.age);
person.chat();
person.run();
</script>
</html>
- Document Object Model
- Browser Object model: window, History, Location, screen, status
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<button>button</button>
</body>
<script>
var btn=document.querySelector("button");
btn.onclick=function(){
// window.history.back();
// window.history.forward();
window.history.go(-2); //back 2 pages
}
</script>
</html>
- window.ocation
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<button>link</button>
</body>
<script>
var btn=document.querySelector("button");
btn.onclick=function () {
window.location="http://www.bing.com";
alert(window.location);
}
</script>
</html>
- ping : host: 202.89.233.101
C:\Users\Administrator>ping www.bing.com
正在 Ping cn-0001.cn-msedge.net [202.89.233.101] 具有 32 字节的数据:
来自 202.89.233.101 的回复: 字节=32 时间=27ms TTL=116
来自 202.89.233.101 的回复: 字节=32 时间=28ms TTL=116
来自 202.89.233.101 的回复: 字节=32 时间=28ms TTL=116
来自 202.89.233.101 的回复: 字节=32 时间=27ms TTL=116
202.89.233.101 的 Ping 统计信息:
数据包: 已发送 = 4,已接收 = 4,丢失 = 0 (0% 丢失),
往返行程的估计时间(以毫秒为单位):
最短 = 27ms,最长 = 28ms,平均 = 27ms
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<style>
div{border:solid red; height:100px;}
</style>
<body>
<form action="" method="get">
<input type="text" name="username">
<input type="submit">
</form>
<a href="#abc">link</a>
<a href="#cba">link</a>
<div id="a"></div>
<div id="b"></div>
<div id="c"></div>
<div id="d"></div>
</body>
<script>
alert(window.location.hash);
alert(location.hash);
alert(location.href);
var btn=document.querySelector("a");
btn.onmouseover=function(){
location.replace("location2.html?#cba");
}
</script>
</html>
- window.document
- screen
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>document</title>
</head>
<body>
<input type="text" id="iphone">1<br>
<input type="text" id="iphone">2<br>
<input type="text" id="samsung" name="mobile">3 <br>
<input type="text" id="android" name="mobile">4<br>
<input type="text" id="huawei" >5<br>
<input type="text" id="xiaomi" class="smartphone">6<br>
</body>
</html>
<script>
document.title="Replace Title";
console.log(document);
document.write("<h1>Header1</h1>")
var abc=document.getElementById("iphone");
abc.value="IOS"; //1
var input= document.getElementsByTagName("input");
input[4].value="default"; //input[4]: 5
var input= document.getElementsByName("mobile");
input[1].value="default2"; //mobile[1]:4
var phone=document.getElementsByClassName("smartphone");
phone[0].value="xiaomi1"; //6
var arr=document.querySelectorAll("#iphone");
arr[1].value="Iphone"//2
document.querySelector("[name=mobile]").value="abc"; //3
</script>
- Document: InnerHTML
- BOM:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
span{
font-size:30px;
font-weight:bold;
height: 50px;
padding: 45px;
}
[type=text]{width:150px};
</style>
</head>
<script>
window.onload=function(){
//first button change word for title
var btn=document.querySelector("input");
function changeLayer(){
document.querySelector("span").innerHTML="SEARCH";
}
btn.onclick=changeLayer;
//Display
var btn2=document.querySelector("#display");
function show(){
var input=document.querySelectorAll("input");
var str="";
for(var i=0; i<input.length; i++){
str=str+ input[i].value+"<br>";
}
var stage=document.querySelector("#stage");
stage.innerHTML=str;
}
btn2.onclick=show;
//Default
var btn3=document.querySelector("#default");
function put(){
var input=document.querySelectorAll("input");
for(var i=0; i<input.length; i++){
if(input[i].type=="text") {
switch (i){
case 1:
document.querySelectorAll("input")[i].value="Spring";
break;
case 2:
document.querySelectorAll("input")[i].value="Summer";
break;
case 3:
document.querySelectorAll("input")[i].value="Autumn";
break;
case 4:
document.querySelectorAll("input")[i].value="Winter";
break;
default:
break;
}
}
}
}
btn3.onclick=put;
}
</script>
<body>
<span>ENTRY</span>
<input type="button" value="Change">
<br><br>
<input type="text" value="Spring">
<input type="text" value="Summer">
<input type="text" value="Autumn">
<input type="text" value="Winter">
<br><br>
<input type="button" value="Display" id="display">
<input type="button" value="Default" id="default">
<div id="stage"></div>
<!--inner html inserted inside div-->
</body>
</html>
- checkbox
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<script>
onload=function(){
var btn=document.querySelector("#btn");
btn.onclick=function(){
var display=document.querySelector("#display");
var str="";
var box=document.querySelectorAll("[type=checkbox]");
for(var i=0; i<box.length; i++) {
if (box[i].checked == true) {
str = str + box[i].value + "<br>";
}
}
display.innerHTML=str;
}
}
</script>
<body>
<h3>Pick Hobbies</h3>
<ul>
<li><input type="checkbox" value="music">music</li>
<li><input type="checkbox" value="movie">movie</li>
<li><input type="checkbox" value="art">art</li>
<li><input type="checkbox" value="climb">climb</li>
<li><input type="checkbox" value="swim">swim</li>
<li><input type="checkbox" value="tennis">tennis</li>
<li><input type="checkbox" value="run">run</li>
<li><input type="checkbox" value="read">read</li>
<hr>
<li><input type="button" value="list " id="btn"></li>
</ul>
<div id="display"></div>
</body>
</html>
- window
- Open
- BOM:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Open</title>
</head>
<body>
<input type="button" id="btn" value="close">
</body>
<script>
win=window.open("http://www.bing.com","SEARCH","width=500, height=500," +
" location=0, status=0, menu=1, scroll=1");
//newWindow=window.open("url", "WindowName", "Attributes")
win.document.write("this is window 'win'");
var btn=document.getElementById("btn");
btn.onclick=function(){
win.close();
}
</script>
</html>
- Interval
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Interval</title>
</head>
<body>
<input type="text" id="name">
</body>
<script>
onload=function() {
var input = document.querySelector("input");
// change();
var interval=setInterval(function change() {
input.value += 1;
}, 2000);
var timeOut=setTimeout(function () {
input.value +="out";
clearInterval(interval);
}, 3000);
setTimeout(function (){
clearTimeout(timeOut)
},5000)
}
</script>
</html>
- counter
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>counter</title>
</head>
<script>
onload=function(){
var num=100;
var stage=document.querySelector("#stage");
function count(){
if(num>0){
num--;
}
stage.innerHTML=num;
return undefined;
}
var interval
//start
var start=document.querySelector("#start");
start.onclick=function(){
interval=setInterval(count,100);
}
//stop
var stop=document.querySelector("#stop");
stop.onclick=function(){
clearInterval(interval);
}
}
</script>
<body>
<button id="start">Start</button>
<button id="stop">Stop</button>
<h1 id="stage"></h1>
</body>
</html>
- DOM: Core, XML, HTML : Node based
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>node</title>
</head>
<body>
<ul>
<li></li>
<li></li>
<li></li>
</ul>
</body>
<script>
var a={name:"Bob", age:40};
console.log(a);
console.log(a.name);
var node1=document.getElementsByTagName("ul")[0];
console.log(node1.childNodes);
console.log(node1.firstChild);
console.log(node1.lastChild);
//history
console.log(window.history);
</script>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>node2</title>
</head>
<body>
<input type="text">
</body>
<script>
console.log(document.childNodes);
var h1=document.createElement("h1");
h1.innerHTML="Header 1";
var h2=document.createElement("h2");
h2.innerHTML="Header 2";
var body=document.getElementsByTagName("body")[0];
var h1c=h1.cloneNode(true);//deep clone
h1c.innerHTML="Header 1 clone";
body.appendChild(h1);
body.appendChild(h1c);
body.appendChild(h1.cloneNode(true));
body.appendChild(h1.cloneNode(true));
body.insertBefore(h2,h1);
body.removeChild(h1); //parent.removeChild
var input=document.querySelector("input");
// body.appendChild(input);
body.replaceChild(input, h1c);
</script>
</html>
- add button
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>add</title>
</head>
<script>
onload=function() {
var btn = document.querySelector("button");
btn.onclick=function(){
var li = document.createElement("LI");
document.querySelector("ul").appendChild(li);
li.innerHTML = "<input value='Insert Info'>";
}
}
</script>
<body>
<button>add query</button>
<ul></ul>
</body>
</html>
- table
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>table</title>
<style>
td{border:solid blue;
width: 300px;}
</style>
</head>
<body>
<table>
<tr>
<td>a</td>
<td>b</td>
</tr>
<tr>
<td>c</td>
<td>d</td>
</tr>
</table>
</body>
<script>
var table=document.querySelector("table");
console.log(table.childNodes);
console.log(table.firstElementChild);
table.insertRow(0).insertCell().innerHTML="1";
var tr=table.insertRow(0);
var td1=tr.insertCell().innerHTML="2";
var td2=tr.insertCell().innerHTML="3";
var td3=tr.insertCell().innerHTML="4";
tr.deleteCell(0);
tr.align="center";
alert(td3.cellIndex);
console.log(table.rows);
console.log(table.rows[0]);
console.log(table.rows[0].cells);
console.log(table.rows[0].cells[0]);
</script>
</html>
- table2
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Table</title>
<style>
table, tr, td{border:solid gray};
</style>
</head>
<body>
<button id="add">Add Line</button>
<button id="remove">Remove Line</button>
<table id="stage">
<tr>
<td>ID</td>
<td>NAME</td>
<td>AGE</td>
</tr>
</table>
</body>
<script>
var table
onload=function(){
var add=document.querySelector("#add");
add.onclick=function addNew(){
table=document.querySelector("#stage");
var tr=table.insertRow();
var td1=tr.insertCell();
td1.innerHTML="1";
var td2=tr.insertCell();
td2.innerHTML="2";
var td3=tr.insertCell();
td3.innerHTML="3";
}
var remove=document.querySelector("#remove");
remove.onclick=function removeRow(){
table.deleteRow(table.rows.length-1);
}
}
</script>
</html>
JavaScript 5: Internal Obj, Form, RegEx
- String
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>String</title>
</head>
<body>
</body>
<script>
var str="abc def c";
document.write(str+"<br>");// abc def c
document.write(str[2]+"<br>"); // c
document.write(str.indexOf("c")+"<br>"); // 2
document.write(str.indexOf("c",3)+"<br>"); // 8
document.write(str.substring(5)+"<br>"); //ef c
document.write(str.substring(0,5)+"<br>"); //abc d
document.write(str.split(" ")+"<br>"); // abc,def,c
document.write(typeof str+"<br>"); //string
document.write(str.toUpperCase()+"<br>"); //ABC DEF C
</script>
</html>
- math
- math
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Math</title>
</head>
<body>
</body>
<script>
var r=Math.random();
document.write(r+"<br>");
document.write(Math.floor(r*10)+"<br>");
document.write(Math.ceil(r*10)+"<br>");
document.write(Math.floor(r*100)+"<br>");
document.write(Math.ceil(r*100)+"<br>");
document.write(Math.sqrt(Math.ceil(r*100))+"<br>")
</script>
</html>
- date
- date
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
</body>
<script>
var d=new Date();
document.write("d "+d+"<br>");
document.write("d.getDate "+d.getDate()+"<br>");
document.write("d.getDay "+d.getDay()+"<br>");
document.write("d.getHours "+d.getHours()+"<br>");
document.write("d.getMinutes "+d.getMinutes()+"<br>");
document.write("d.getSeconds "+d.getSeconds()+"<br>");
document.write("d.getMonth "+d.getMonth()+"<br>");
document.write("d.getFullYear "+d.getFullYear()+"<br>");
document.write("d.getTime "+d.getTime()+"<br>"); //milliseconds
</script>
</html>
- clock
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>timer</title>
</head>
<script>
function show() {
var d = new Date();
// document.write("d "+d+"<br>");
var year = d.getFullYear();
var month = d.getMonth();
var date = d.getDate();
var hours = d.getHours();
var minutes = d.getMinutes();
var seconds = d.getSeconds();
var day = d.getDay();
var ampm = "AM";
if (hours> 12) {
hours = hours - 12;
ampm = "PM";
}
switch (day){
case 0: day="Sunday"; break;
case 1: day="Sunday"; break;
case 2: day="Sunday"; break;
case 3: day="Wednesday"; break;
case 4: day="Thursday"; break;
case 5: day="Friday"; break;
case 6: day="Saturday"; break;
}
var str = year + " year " + month + " month " +
date + " date " + hours + " hours " +
minutes + " minutes " + seconds + " seconds " +
ampm + " "+ day ;
//document.write(str+"<br>");
var clock=document.querySelector("#clock");
clock.innerHTML=str;
}
onload=function() {
setInterval(show, 1000); //1000 milliseconde= 1 seconde
}
</script>
<body>
<h1>Timer</h1>
<hr>
<h3 id="clock"></h3>
</body>
</html>
- Array
- array
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
</body>
<script>
var arr=[33,55,"abcd"];
document.write(arr.join("_")+"<br>");
for(var i=0; i<arr.length; i++){
document.write(arr[i]+"<br>");
}
for(var v in arr){
document.write(arr[v]+"<br>");
}
var arr1=[[1,2,3],["bb","aa"],[11,22,33]];
document.write(arr1.join("_")+"<br>");
arr1.push([0,7],[4,222]);
document.write(arr1.join("_")+"<br>");
document.write(arr1.sort()+"<br>");
for(var k in arr1){
for(var k2 in arr1[k]){
document.write(arr1[k][k2]+"<br>");
}
}
</script>
</html>
- triangle
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>triangle2</title>
<script type="text/javascript">
window.onload=function(){
var arr=[];
var txt;
for(var i=0; i<66; i++){
txt="x"
for(var j=0; j<i; j++){
txt+=" x";
}
arr.push(txt);
}
document.getElementById("text").innerHTML=(arr.join("<br/>"));
}
</script>
</head>
<body>
<p id="text" style="text-align: center"></p>
</body>
</html>
- average
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>average</title>
</head>
<script>
onload=function () {
var score=[[22,34,78,34,22,44],
[92,74,55,24,78,55],
[34,34,45,34,34,45]];
function avg(arr) {
var sum=0;
for(var i in arr){
sum+=arr[i];
}
var result=sum/arr.length;
return parseFloat(result, 2);
}
document.write("avg1="+avg(score[0])+"<br>");
document.write("avg2="+avg(score[1])+"<br>");
document.write("avg3="+avg(score[2])+"<br>");
}
</script>
<body>
</body>
</html>
- DOM control style
- style
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>style</title>
<style>
h1{
color: red;
}
.show{
display:block;
}
.hide{
display: table;
}
</style>
</head>
<script>
onload=function(){
var h1=document.querySelectorAll("h1");
h1[0].onclick=function () {
h1[0].style.color="#F0F000";
}
h1[1].onclick=function () {
h1[1].style.color="#F0F000";
}
var btn=document.querySelector("button");
btn.onclick=function () {
if(h1.classname=="hide") {
h1.classname = "hide";
}else{
h1.classname="show";
}
h1[0].style.backgroundColor="#F0F";
h1[1].style.backgroundColor="#F0F";
}
alert(h1.style.backgroundColor);
}
</script>
<body>
<button>display</button>
<h1 class="show" style="background-color: green">HELLO WORLD</h1>
<h1 class="hide" style="background-color: green">GOODBYE</h1>
</body>
</html>
- tabs: onmouseover, className
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>tabs</title>
<style>
.selected{
padding:5px;
border: 1px solid #0005ff;
background-color: #ff8c00;
}
.tab1,.tab2,.cont1,.cont2{
border: 1px solid #0005ff;
}
.cont1, .cont2{
height: 100px;
margin-top: 4px;
}
.cont2{
display: none;
background-color: #ffda00;
}
.tab1,.tab2{
padding:5px;
}
</style>
</head>
<script>
onload=function(){
var tab1=document.querySelector(".tab1");
var tab2=document.querySelector(".tab2");
var cont1=document.querySelector(".cont1");
var cont2=document.querySelector(".cont2");
tab1.onmouseover=function(){
cont2.style.display="none";
cont1.style.display="block";
tab1.className="selected";
tab2.className=("tab2");
}
tab2.onmouseover=function(){
cont1.style.display="none";
cont2.style.display="block";
tab2.className="selected";
tab1.className=("tab2");
}
}
</script>
<body>
<span class="tab1" >span 1</span>
<span class="tab2">span 2</span>
<div class="cont1">div 1</div>
<div class="cont2">div 2</div>
</body>
</html>
- menu drop down
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>dropdown</title>
<style>
ul {
border: 1px solid #aaaaaa;
background-color: #ddd;
}
li, dt {
float: left;
list-style: none;
width: 150px;
height: 40px;
line-height: 40px;
background-color: #ddd;
text-align: left;
}
dl {
margin: 0;
display: none;
}
dt {
border: 1px solid #aaaaaa;
}
.clear {
clear: both;
}
</style>
</head>
<script>
onload = function () {
var li = document.querySelectorAll("li");
for (var i = 0; i < li.length; i++) {
li[i].onmouseover = function () {
var dl = this.querySelector("dl");
if (dl) {
dl.style.display = "block";
}
}
li[i].onmouseout = function () {
var dl = this.querySelector("dl");
if (dl) {
dl.style.display = "none";
}
}
}
}
</script>
<body>
<ul>
<li>Menu</li>
<li>News
<dl>
<dt>World News</dt>
<dt>National News</dt>
</dl>
</li>
<li>Games
<dl>
<dt>RPG Games</dt>
<dt>Web Games</dt>
</dl>
</li>
<li>About</li>
<div class="clear"></div>
</ul>
</body>
</html>
-
form
-
DOM: onsubmit
-
GET : Form data are restricted to ASCII codes. Special care should be taken to encode and decode other types of characters when passing them through the URL in ASCII format. On the other hand, binary data, images and other files can all be submitted through METHOD=“POST”
- GET : All form data filled in is visible in the URL. Moreover, it is also stored in the user’s web browsing history/logs for the browser. These issues make GET less secure.
-
GET : However, one advantage of form data being sent as part of the URL is that one can bookmark the URLs and directly use them and completely bypass the form-filling process.
-
GET : There is a limitation on how much form data can be sent because URL lengths are limited.
-
form
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>form</title>
</head>
<script>
onload = function () {
var verify = false;
//turn red on entering
var form = document.querySelector("#sub");
var input = document.querySelectorAll("input[type=text], input[type=password]");
for (var i in input) {
input[i].onfocus = function () {
this.style.outline = "red solid 1px";
verify=false;
}
}
function check(){
//turn green after entering
var username = document.querySelector("[name=username]");
username.onblur = function () {
var t = this.nextSibling;
if (this.value == "") {
t.innerHTML = "<span> Username empty</span>";
} else {
t.innerHTML = "";
this.style.outline = "green solid 1px";
verify=true;
}
}
var password = document.querySelector("[name=password]");
password.onblur = function () {
var t = this.nextSibling;
var reg = /\S{6,}/g;
if (!this.value.match(reg)) {
t.innerHTML = "<span> password too short (6)</span>";
} else {
t.innerHTML = "";
this.style.outline = "green solid 1px";
verify=true;
}
}
var nickname = document.querySelector("[name=nickname]");
nickname.onblur = function () {
var t = this.nextSibling;
if (this.value == "") {
t.innerHTML = "<span> Nickname empty</span>";
} else {
t.innerHTML = "";
this.style.outline = "green solid 1px";
verify=true;
}
}
var mobile = document.querySelector("[name=mobile]");
mobile.onblur = function () {
var t = this.nextSibling;
var reg = /^1[358]\d{9}$/g
if (!reg.test(this.value)) {
t.innerHTML = "<span> mobile incorrect</span>";
} else {
t.innerHTML = "";
this.style.outline = "green solid 1px";
verify=true;
}
}
var email = document.querySelector("[name=email]");
email.onblur = function () {
var t = this.nextSibling;
var reg = /^\w+@\w+(\.\w+){1,2}/g
if (!reg.test(this.value)) {
t.innerHTML = "<span> email incorrect</span>";
} else {
t.innerHTML = "";
this.style.outline = "green solid 1px";
verify=true;
}
}
}
form.onsubmit=function(){
check();
return verify;
}
}
</script>
<body>
<form action="http://www.bing.com" id="sub">
username <input type="text" name="username"><span></span><br>
password <input type="password" name="password"><span></span><br>
nickname <input type="text" name="nickname"><span></span><br>
mobile <input type="text" name="mobile"><span></span><br>
email <input type="text" name="email"><span></span><br>
<input type="submit">
</form>
</body>
</html>
- REGEX
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>regex</title>
</head>
<script>
var str="1. this is a new string : 12345_t";
var reg=/[tef]his/;
var reg2=new RegExp("[tef]his");
document.write(str.match(reg)+"<br>"); //this
document.write(str.match(reg2)+"<br>"); //this
var reg3 =/[ais]/;
var reg4 =/[ais]/g;
var reg5 =/[^ais]/g;
document.write(str.match(reg3)+"<br>"); //i
document.write(str.match(reg4)+"<br>"); //i,s,i,s,a,s,i
document.write(str.match(reg5)+"<br>"); //1,., ,t,h, , , ,n,e,w, ,t,r,n,g, ,:, ,1,2,3,4,5,_,t
var reg6 =/[0-9]/g;
var reg7 =/[a-zA-Z]/g;
document.write(str.match(reg6)+"<br>"); //1,1,2,3,4,5
document.write(str.match(reg7)+"<br>"); //t,h,i,s,i,s,a,n,e,w,s,t,r,i,n,g,t
var reg8=/[red|blue|green]/g;
var reg9=/(red)(blue)(green)/g;
var reg10=/red|blue|green/g;
var reg11=/[redbluegreen]/g;
var reg12=/[^redbluegreen]/g;
document.write(str.match(reg8)+"<br>"); //n,e,r,n,g
document.write(str.match(reg9)+"<br>"); //null
document.write(str.match(reg10)+"<br>"); //null
document.write(str.match(reg11)+"<br>"); //n,e,r,n,g
document.write(str.match(reg12)+"<br>"); //1,., ,t,h,i,s, ,i,s, ,a, ,w, ,s,t,i, ,:, ,1,2,3,4,5,_,t
var reg13=/t$/g;
var reg14=/ /g;
var reg15=/\s/g;
var reg16=/\S/g;
var reg17=/\d/g;
var reg18=/\D/g;
var reg19=/\w/g;
var reg20=/\W/g;
var reg21=/./g;
var reg22=/\_/g;
document.write(str.match(reg13)+"<br>"); //t
document.write(str.match(reg14)+"<br>"); //, , , , , , ,
document.write(str.match(reg15)+"<br>"); //, , , , , , ,
document.write(str.match(reg16)+"<br>"); //1,.,t,h,i,s,i,s,a,n,e,w,s,t,r,i,n,g,:,1,2,3,4,5,_,t
document.write(str.match(reg17)+"<br>"); //1,1,2,3,4,5
document.write(str.match(reg18)+"<br>"); //., ,t,h,i,s, ,i,s, ,a, ,n,e,w, ,s,t,r,i,n,g, ,:, ,_,t
document.write(str.match(reg19)+"<br>"); //1,t,h,i,s,i,s,a,n,e,w,s,t,r,i,n,g,1,2,3,4,5,_,t
document.write(str.match(reg20)+"<br>"); //., , , , , , ,:,
document.write(str.match(reg21)+"<br>"); //1,., ,t,h,i,s, ,i,s, ,a, ,n,e,w, ,s,t,r,i,n,g, ,:, ,1,2,3,4,5,_,t
document.write(str.match(reg22)+"<br>"); //_
</script>
<body>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>regex2</title>
</head>
<body>
</body>
<script>
var str="this333 is333 a333 new333 string333 12333345 red333 t_-[]'()'{}333"
var reg=/3/g;
var reg2=/3{2}/g;
var reg3=/\w{4}/g;
var reg4=/\w{4}\d{2}/g;
var reg5=/\w{4}\d{0,}/g;
var reg6=/\w\d*/g;
var reg7=/\w\d+/g;
var reg8=/\w\d?/g;
document.write(str.match(reg)+"<br>");
//3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3
document.write(str.match(reg2)+"<br>");
//33,33,33,33,33,33,33,33,33
document.write(str.match(reg3)+"<br>");
//this,is33,a333,new3,stri,ng33,1233,3345,red3
document.write(str.match(reg4)+"<br>");
//this33,new333,ring33,123333,red333
document.write(str.match(reg5)+"<br>");
//this333,is333,a333,new333,stri,ng333,12333345,red333
document.write(str.match(reg6)+"<br>");
//t,h,i,s333,i,s333,a333,n,e,w333,s,t,r,i,n,g333,12333345,r,e,d333,t,_,333
document.write(str.match(reg7)+"<br>");
//s333,s333,a333,w333,g333,12333345,d333,333
document.write(str.match(reg8)+"<br>");
//t,h,i,s3,33,i,s3,33,a3,33,n,e,w3,33,s,t,r,i,n,g3,33,12,33,33,45,r,e,d3,33,t,_,33,3
var reg9=/[a-zA-Z]/g;
var reg10=/^[a-zA-Z][\da-zA-Z]{2,12}/g;
document.write(str.match(reg9)+"<br>");
//t,h,i,s,i,s,a,n,e,w,s,t,r,i,n,g,r,e,d,t
document.write(str.match(reg10)+"<br>");
//this333,is333,a333,new333,string333,red333
document.write(reg10.test(str)+"<br>");
var str2="abc123@abc123.com";
var reg11=/^\w+@\w+(\.\w+){1,2}/g
document.write(str2.match(reg11)+"<br>");
</script>
</html>
- form
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>regex3</title>
</head>
<script>
onload=function(){
var verify=true;
var form=document.querySelector("#form");
form.onsubmit=function(){
check();
return verify;
}
function check() {
var mobile=document.querySelector("#mobile");
var reg=/1[358]\d{9}$/g
if(!reg.test(mobile.value)){
verify=false;
alert("Phone number incorrect");
}else{
verify=true;
}
}
}
</script>
<body>
<form action="http://www.bing.com" id="form">
Phone: <input type="text" id="mobile"><br>
<input type="submit">
</form>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>regex</title>
</head>
<body>
</body>
<script>
var str="I have a pen and a pineapple."
var reg=/\w{4}/;
var reg2=/\w{4}/g;
document.write(str.replace(reg,"####")+"<br>");
document.write(str.replace(reg2,"####")+"<br>");
</script>
</html>
JavaScript 6: Examples
- login check with regEx
- login.html
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title>当当网登录页面</title>
<link href="css/global.css" rel="stylesheet" type="text/css"/>
<link href="css/layout.css" rel="stylesheet" type="text/css"/>
<!--script type="text/javascript" src="js/login.js"></script-->
</head>
<body>
<div id="header">
<div class="login_header_left"><img src="images/logo.gif" alt="logo"></div>
<div class="login_header_mid"><img src="images/login_header.gif" alt="header"></div>
<div class="login_header_right"><a href="index.html" class="blue">首页</a> |
<a href="product.html" class="blue">商品展示页</a> | <a href="shopping.html" class="blue">购物车</a> |
<a href="register.html" class="blue">注册</a></div>
</div>
<div id="main">
<div class="login_main_left">
<div><img src="images/login_main_01.jpg" alt="中间图片">
<img src="images/login_main_02.jpg" alt="中间图片">
<img src="images/login_main_03.jpg" alt="中间图片">
</div>
<div class="login_main_end">
<dl class="login_green">
<dt>更多选择、更低价格</dt>
<dd>100万种商品包含图书、数码、美妆、运动健康等,价格低于地面店20%以上</dd>
</dl>
<div class="login_main_dotted"></div>
<dl class="login_green">
<dt>全场免运费、货到付款</dt>
<dd>免费送货上门、360个城市货到付款。另有网上支付、邮局汇款等多种支付方式</dd>
</dl>
<div class="login_main_dotted"></div>
<dl class="login_green">
<dt>真实、优质的商品评论</dt>
<dd>千万用户真实、优质的商品评论,给您多角度、全方位的购物参考</dd>
</dl>
</div>
</div>
<div class="login_main_mid">
<div class="login_content_top">请登录当当网</div>
<div class="login_content_line"></div>
<form action="" method="post" onSubmit="return checkLogin()" id="form">
<dl class="login_content">
<dt>Email地址或昵称:</dt>
<dd><input id="email" type="text" class="login_content_input" onFocus="emailFocus()"
onBlur="emailBlur()"></dd>
</dl>
<dl class="login_content">
<dt>密码:</dt>
<dd><input id="pwd" type="password" class="login_content_input" onFocus="pwdFocus()" onBlur="pwdBlur()">
</dd>
</dl>
<!--added script-->
<script>
onload = function () {
var form = document.querySelector("#form");
var pwd = document.querySelector("#pwd");
var input = document.querySelector("#email");
function emailFocus() {
input.classList.add("login_content_input_Focus");
}
function emailBlur() {
input.classList.remove("login_content_input_Focus");
}
function pwdFocus() {
input.classList.add("login_content_input_Focus");
}
function pwdBlur() {
input.classList.remove("login_content_input_Focus");
}
form.onsubmit = function () {
var verified = true;
var regEmail =/^\w+@\w+(\.\w+){1,2}$/;
var regName =/^\w{6,12}$/;
if ((!input.value.match(regEmail)) && (!input.value.match(regName))) {
alert("Email error");
verified = false;
}
var regPwd =/\S{6,12}/
if (!pwd.value.match(regPwd)) {
alert("Password error");
verified = false;
}
return verified;
}
}
</script>
<!--added script-->
<dl class="login_content">
<dt></dt>
<dd><input id="btn" value=" " type="submit" class="login_btn_out"
onmouseover="this.className='login_btn_over'" onmouseout="this.className='login_btn_out'">
</dd>
</dl>
</form>
<div class="login_content_dotted"></div>
<div class="login_content_end_bg">
<div class="login_content_end_bg_top">
<label class="login_content_bold">还不是当当网用户?</label>快捷方便的免费注册,让你立刻尽享当当网提供的条项优惠服务......
</div>
<div class="login_content_end_bg_end">
<input class="login_register_out" value=" " type="button"
onmouseover="this.className='login_register_over'"
onmouseout="this.className='login_register_out'" onClick="jump()">
</div>
</div>
</div>
<div class="login_main_right"><img src="images/login_main_04.jpg" alt="右侧图片"></div>
</div>
<div id="footer">
<iframe src="footer.html" height="50px" width="900px" frameborder="0"></iframe>
</div>
</body>
</html>
- form modification
- register.html
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>当当网注册页面</title>
<link href="css/global.css" rel="stylesheet" type="text/css" />
<link href="css/layout.css" rel="stylesheet" type="text/css" />
<script type="text/javascript" src="js/register.js"></script>
</head>
<body>
<div id="header">
<div id="register_header">
<div class="register_header_left"><img src="images/logo.gif" alt="logo"></div>
<div class="register_header_right"><a href="index.html" class="blue">首页</a> | <a href="product.html" class="blue">商品展示页</a> | <a href="shopping.html" class="blue">购物车</a> | <a href="login.html" class="blue">登录</a></div>
</div>
</div>
<div id="main">
<div class="register_content">
<div class="register_top_bg"></div>
<div class="register_mid_bg">
<ul>
<li class="register_mid_left">填写注册信息</li>
<li class="register_mid_mid">2. 邮箱验证</li>
<li class="register_mid_right">3. 完成注册</li>
</ul>
</div>
<div class="register_top_bg_mid">
<div class="register_top_bg_two_left"></div>
<div class="register_top_bg_two_right"></div>
<div class="register_title_bg"><img src="images/register_pic_02.gif" alt="欢迎注册"><br>您所提供的资料不会做其他用途,敬请安心填写。</div>
</div>
<div class="register_dotted_bg"></div>
<div class="register_message">
<form action="" method="post" id="myform" onSubmit="return checkRegister()">
<dl class="register_row">
<dt>Email地址:</dt>
<dd><input id="email" type="text" class="register_input" onFocus="emailFocus()" onBlur="emailBlur()"></dd>
<dd><div id="email_prompt"></div></dd>
<!--added script-->
</dl>
<dl class="register_row">
<dt>设置昵称:</dt>
<dd><input id="nickName" type="text" class="register_input" onFocus="nickNameFocus()" onBlur="nickNameBlur()"></dd>
<dd><div id="nickName_prompt"></div></dd>
<!--added script-->
</dl>
<dl class="register_row">
<dt>设定密码:</dt>
<dd><input id="pwd" type="password" class="register_input" onFocus="pwdFocus()" onBlur="pwdBlur()"></dd>
<dd><div id="pwd_prompt"></div></dd>
</dl>
<!--added script-->
<dl class="register_row">
<dt>再输入一次密码:</dt>
<dd><input id="repwd" type="password" class="register_input" onFocus="repwdFocus()" onBlur="repwdBlur()"></dd>
<dd><div id="repwd_prompt"></div></dd>
<!--added script-->
</dl>
<dl class="register_row">
<dt>性别:</dt>
<dd><input name="sex" id="man" type="radio" value="男"> <label for="man">男</label></dd>
<dd> <input name="sex" id="woman" type="radio" value="女"> <label for="woman">女</label></dd>
</dl>
<dl class="register_row">
<dt>所在地区:</dt>
<dd>
<select id="province" onChange="changeCity()" style="width:120px;">
<option>请选择省/城市</option>
</select>
</dd>
<dd>
<select id="city" style="width:130px;">
<option>请选择城市/地区</option>
</select>
</dd>
</dl>
<div class="registerBtn"><input id="registerBtn" type="image" src="images/register_btn_out.gif" onMouseOver="btn_over()" onMouseOut="btn_out()"></div>
</form>
</div>
</div>
</div>
<!--网站版权部分开始-->
<div id="footer">
<iframe src="footer.html" height="50px" width="900px" frameborder="0"></iframe>
</div>
</body>
</html>
- register.js
/**
* Created by Administrator on 2020/7/4.
*/
function emailFocus(){
console.log(11111);
var email=document.querySelector("#email");
var email_prompt=document.querySelector("#email_prompt");
email.classList.add("register_input_Focus");
email_prompt.innerHTML="Enter Email";
email_prompt.className="register_prompt"
}
function emailBlur(){
var email_prompt=document.querySelector("#email_prompt");
var email=document.querySelector("#email");
var reg=/\w+@\w+(\.\w+){1,2}/;
email.classList.add("register_input_Blur");
email.classList.remove("register_input_Focus");
if(reg.test(email.value)){
email_prompt.className="register_prompt_ok";
email_prompt.innerHTML="";
}else{
email_prompt.className="register_prompt_error";
email_prompt.innerHTML="Enter Correct Email";
}
}
var regionList=[];
regionList["ProvinceA"]=["CityA1", "CityA2", "CityA3"];
regionList["ProvinceB"]=["CityB1", "CityB2", "CityB3"];
onload=function(){
var selectProvince=document.querySelector("#province");
for(var i in regionList){
var element=document.createElement("OPTION");
element.value=i;
element.innerHTML=i;
selectProvince.appendChild(element);
}
}
function changeCity(){
var selectProvince=document.querySelector("#province");
var selectCity=document.querySelector("#city");
selectCity.innerHTML="<option>select City: Province</option>";
for(var i in regionList[selectProvince.value]){
var element=document.createElement("OPTION");
element.value=regionList[selectProvince.value][i];
element.innerHTML=regionList[selectProvince.value][i];
selectCity.appendChild(element);
}
}
function btn_over(){
var registerBtn=document.querySelector("#registerBtn");
registerBtn.setAttribute("src","images/register_btn_over.gif");
}
function btn_out(){
var registerBtn=document.querySelector("#registerBtn");
registerBtn.setAttribute("src","images/register_btn_out.gif");
}
- hide/show, deletion, calculations
- shopping.html
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>当当网购物车页面</title>
<link href="css/global.css" rel="stylesheet" type="text/css" />
<link href="css/layout.css" rel="stylesheet" type="text/css" />
<script type="text/javascript" src="js/shopping.js"></script>
</head>
<body>
<div id="header"><iframe src="header.html" height="155px" width="960px" frameborder="0"></iframe></div>
<!--中间部分开始-->
<div id="main">
<div> <img src="images/shopping_myshopping.gif" alt="shopping"> <a href="#">全场免运费活动中</a></div>
<!--为您推荐商品开始-->
<div class="shopping_commend">
<div class="shopping_commend_left">根据您挑选的商品,当当为您推荐</div>
<div class="shopping_commend_right"><img src="images/shopping_arrow_up.gif" alt="shopping" id="shopping_commend_arrow"
onClick="shopping_commend_show()"></div>
</div>
<div id="shopping_commend_sort" style="display:block">
<div class="shopping_commend_sort_left">
<ul>
<li class="shopping_commend_list_1">·<a href="#" class="blue">JavaScript DOM编程艺术</a></li>
<li class="shopping_commend_list_2">¥39.00</li>
<li class="shopping_commend_list_3">¥29.30</li>
<li class="shopping_commend_list_4"><a href="#" class="shopping_yellow">购买</a></li>
</ul>
<ul>
<li class="shopping_commend_list_1">·<a href="#" class="blue">解禁(当当网独家首发)</a></li>
<li class="shopping_commend_list_2">¥28.00</li>
<li class="shopping_commend_list_3">¥19.40</li>
<li class="shopping_commend_list_4"><a href="#" class="shopping_yellow">购买</a></li>
</ul>
<ul>
<li class="shopping_commend_list_1">·<a href="#" class="blue">地王之王(金融危机下房地产行...</a></li>
<li class="shopping_commend_list_2">¥32.80</li>
<li class="shopping_commend_list_3">¥25.10</li>
<li class="shopping_commend_list_4"><a href="#" class="shopping_yellow">购买</a></li>
</ul>
<ul>
<li class="shopping_commend_list_1">·<a href="#" class="blue">逃庄</a></li>
<li class="shopping_commend_list_2">¥36.00</li>
<li class="shopping_commend_list_3">¥27.70</li>
<li class="shopping_commend_list_4"><a href="#" class="shopping_yellow">购买</a></li>
</ul>
</div>
<div class="shopping_commend_sort_mid"></div>
<div class="shopping_commend_sort_left">
<ul>
<li class="shopping_commend_list_1">·<a href="#" class="blue">深入浅出MySQL数据库开发、优...</a></li>
<li class="shopping_commend_list_2">¥59.00</li>
<li class="shopping_commend_list_3">¥47.20</li>
<li class="shopping_commend_list_4"><a href="#" class="shopping_yellow">购买</a></li>
</ul>
<ul>
<li class="shopping_commend_list_1">·<a href="#" class="blue">大玩家(马未都、王刚推荐!央...</a></li>
<li class="shopping_commend_list_2">¥34.80</li>
<li class="shopping_commend_list_3">¥20.60</li>
<li class="shopping_commend_list_4"><a href="#" class="shopping_yellow">购买</a></li>
</ul>
<ul>
<li class="shopping_commend_list_1">·<a href="#" class="blue">都市风水师--官场风水小说:一...</a></li>
<li class="shopping_commend_list_2">¥39.80</li>
<li class="shopping_commend_list_3">¥30.50</li>
<li class="shopping_commend_list_4"><a href="#" class="shopping_yellow">购买</a></li>
</ul>
<ul>
<li class="shopping_commend_list_1">·<a href="#" class="blue">国戏(以麻将术语解读宦海沉浮...</a></li>
<li class="shopping_commend_list_2">¥25.00</li>
<li class="shopping_commend_list_3">¥17.30</li>
<li class="shopping_commend_list_4"><a href="#" class="shopping_yellow">购买</a></li>
</ul>
</div>
</div>
<div class="shopping_list_top">您已选购以下商品</div>
<div class="shopping_list_border">
<table width="100%" border="0" cellspacing="0" cellpadding="0">
<tr class="shopping_list_title">
<td class="shopping_list_title_1">商品名</td>
<td class="shopping_list_title_2">单品积分</td>
<td class="shopping_list_title_3">市场价</td>
<td class="shopping_list_title_4">当当价</td>
<td class="shopping_list_title_5">数量</td>
<td class="shopping_list_title_6">删除</td>
</tr>
</table>
<table width="100%" border="0" cellspacing="0" cellpadding="0" id="myTableProduct">
<tr class="shopping_product_list" id="shoppingProduct_01" onMouseOver="productOver('shoppingProduct_01')" onMouseOut="productOut('shoppingProduct_01')">
<td class="shopping_product_list_1"><a href="#" class="blue">私募(首部披露资本博弈秘密的金融...</a></td>
<td class="shopping_product_list_2"><label>189</label></td>
<td class="shopping_product_list_3">¥<label>32.00</label></td>
<td class="shopping_product_list_4">¥<label>18.90 </label>(59折)</td>
<td class="shopping_product_list_5"><input type="text" value="1" onblur="productCount()"></td>
<td class="shopping_product_list_6"><a href="javascript:deleteProduct('shoppingProduct_01')" class="blue">删除</a></td>
</tr>
<tr class="shopping_product_list" id="shoppingProduct_02" onMouseOver="productOver('shoppingProduct_02')" onMouseOut="productOut('shoppingProduct_02')">
<td class="shopping_product_list_1"><a href="#" class="blue"> 小团圆(张爱玲最神秘小说遗稿)</a></td>
<td class="shopping_product_list_2"><label>173</label></td>
<td class="shopping_product_list_3">¥<label>28.00</label></td>
<td class="shopping_product_list_4">¥<label>17.30</label>(62折)</td>
<td class="shopping_product_list_5"><input type="text" value="1" onBlur="productCount()"></td>
<td class="shopping_product_list_6"><a href="javascript:deleteProduct('shoppingProduct_02')" class="blue">删除</a></td>
</tr>
<tr class="shopping_product_list" id="shoppingProduct_03" onMouseOver="productOver('shoppingProduct_03')" onMouseOut="productOut('shoppingProduct_03')">
<td class="shopping_product_list_1"><a href="#" class="blue">不抱怨的世界(畅销全球80国的世界...</a></td>
<td class="shopping_product_list_2"><label>154</label></td>
<td class="shopping_product_list_3">¥<label>24.80</label></td>
<td class="shopping_product_list_4">¥<label>15.40</label> (62折)</td>
<td class="shopping_product_list_5"><input type="text" value="2" onBlur="productCount()"></td>
<td class="shopping_product_list_6"><a href="javascript:deleteProduct('shoppingProduct_03')" class="blue">删除</a></td>
</tr>
<tr class="shopping_product_list" id="shoppingProduct_04" onMouseOver="productOver('shoppingProduct_04')" onMouseOut="productOut('shoppingProduct_04')">
<td class="shopping_product_list_1"><a href="#" class="blue">福玛特双桶洗衣机XPB20-07S</a></td>
<td class="shopping_product_list_2"><label>358</label></td>
<td class="shopping_product_list_3">¥<label>458.00</label></td>
<td class="shopping_product_list_4">¥<label>358.00</label> (78折)</td>
<td class="shopping_product_list_5"><input type="text" value="1" onBlur="productCount()"></td>
<td class="shopping_product_list_6"><a href="javascript:deleteProduct('shoppingProduct_04')" class="blue">删除</a></td>
</tr>
<tr class="shopping_product_list" id="shoppingProduct_05" onMouseOver="productOver('shoppingProduct_05')" onMouseOut="productOut('shoppingProduct_05')">
<td class="shopping_product_list_1"><a href="#" class="blue">PHP和MySQL Web开发 (原书第4版)</a></td>
<td class="shopping_product_list_2"><label>712</label></td>
<td class="shopping_product_list_3">¥<label>95.00</label></td>
<td class="shopping_product_list_4">¥<label>71.20</label> (75折)</td>
<td class="shopping_product_list_5"><input type="text" value="1" onBlur="productCount()"></td>
<td class="shopping_product_list_6"><a href="javascript:deleteProduct('shoppingProduct_05')" class="blue">删除</a></td>
</tr>
<tr class="shopping_product_list" id="shoppingProduct_06" onMouseOver="productOver('shoppingProduct_06')" onMouseOut="productOut('shoppingProduct_06')">
<td class="shopping_product_list_1"><a href="#" class="blue">法布尔昆虫记</a>(再买¥68.30即可参加“满199元减10元现金”活动)</td>
<td class="shopping_product_list_2"><label>10</label></td>
<td class="shopping_product_list_3">¥<label>198.00</label></td>
<td class="shopping_product_list_4">¥<label>130.70</label> (66折)</td>
<td class="shopping_product_list_5"><input type="text" value="1" onBlur="productCount()"></td>
<td class="shopping_product_list_6"><a href="javascript:deleteProduct('shoppingProduct_06')" class="blue">删除</a></td>
</tr>
</table>
<div class="shopping_list_end">
<ul>
<li class="shopping_list_end_1"><input name="" type="image" src="images/shopping_balance.gif"></li>
<li class="shopping_list_end_2">¥<label id="product_total"></label></li>
<li class="shopping_list_end_3">商品金额总计:</li>
<li class="shopping_list_end_4">您共节省金额:¥<label class="shopping_list_end_yellow" id="product_save"></label><br/>
可获商品积分:<label class="shopping_list_end_yellow" id="product_integral"></label>
</li>
</ul>
</div>
</div>
</div>
<!--网站版权部分开始-->
<div id="footer">
<iframe src="footer.html" height="50px" width="900px" frameborder="0"></iframe></div>
</body>
</html>
- shopping.js
/**
* Created by Administrator on 2020/7/12.
*/
///retract top items
function shopping_commend_show(){
var arrowImg=document.querySelector(".shopping_commend_right img");
var shopping_commend_sort=document.querySelector("#shopping_commend_sort");
//var imgPath=arrowImg.getAttribute("src");
//if (imgPath=="images/shopping_arrow_up.gif")
if(shopping_commend_sort.style.display=="none"){
shopping_commend_sort.style.display="block";
arrowImg.setAttribute("src","images/shopping_arrow_down.gif")
}else{
shopping_commend_sort.style.display="none";
arrowImg.setAttribute("src","images/shopping_arrow_up.gif")
}
}
//delete item
onload=function(){
var shopping_product_list=document.querySelectorAll(".shopping_product_list");
for(var i=0; i<shopping_product_list.length; i++) {
shopping_product_list[i].onmouseover = function () {
this.style.backgroundColor = "#fff";
}
shopping_product_list[i].onmouseout = function () {
this.style.backgroundColor = "#fefbf2";
}
var a = shopping_product_list[i].querySelector(".shopping_product_list_6 a");
a.onclick = function () {
var flag = confirm("Delete?");
if (!flag) {
return;
}
//var target= document.querySelector("#"+id);
//target.parentNode.removeChild(target);
var tr = this.parentNode.parentNode;
tr.parentNode.removeChild(tr);
countTotalPrice();
}
shopping_product_list[i].querySelector("input").onchange=function(){
countTotalPrice();
}
}
countTotalPrice();
}
price
function countTotalPrice(){
var totalPrice=0;
var totalScores=0;
var totalSaved=0;
var shopping_product_list=document.querySelectorAll(".shopping_product_list");
for(var i=0; i<shopping_product_list.length; i++){
var score=shopping_product_list[i].querySelector(".shopping_product_list_2 label").innerText;
var marketingPrice=shopping_product_list[i].querySelector(".shopping_product_list_3 label").innerText;
var dPrice=shopping_product_list[i].querySelector(".shopping_product_list_4 label").innerText;
var amount=shopping_product_list[i].querySelector(".shopping_product_list_5 input").value;
totalPrice+=parseFloat(dPrice)*amount;
totalScores+=parseFloat(score)*amount;
totalSaved+=(parseFloat(marketingPrice)-parseFloat(dPrice))*amount;
}
totalPrice=priceProcess(totalPrice);
totalScores=priceProcess(totalScores);
totalSaved=priceProcess(totalSaved);
var product_total=document.getElementById("product_total");
product_total.innerText=totalPrice;
var product_save=document.getElementById("product_save");
product_save.innerText=totalSaved;
var product_integral=document.getElementById("product_integral");
product_integral.innerText=totalScores;
}
function priceProcess(num){
return parseInt(num*100)/100;
}
- ad change, tabs, scroll
- index.html
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>当当网首页</title>
<link href="css/global.css" rel="stylesheet" type="text/css" />
<link href="css/layout.css" rel="stylesheet" type="text/css" />
<script type="text/javascript" src="js/index.js"></script>
</head>
<body>
<div id="right" class="right">
<div class="dd_close" id="dd_close">
<a href="###" id="shutAd">关闭</a></div>
<img src="images/dd_scroll.jpg" id="right1" /> </div>
<div id="header">
<iframe src="header.html" height="155px" width="960px" frameborder="0"></iframe>
</div>
<!--网站中间内容开始-->
<div id="main">
<div class="dd_index_top_adver"><img src="images/dd_index_top_adver.jpg" alt="通栏广告图片" /></div>
<!--左侧菜单开始-->
<div id="catList">
<!--推荐分类-->
<div class="book_sort">
<div class="book_sort_bg">推荐分类</div>
<div class="book_sort_bottom" style="border-bottom:0px;">外语 | 中小学教辅 |</div>
</div>
<!--图书商品分类开始-->
<div class="book_sort">
<div class="book_sort_bg"><img src="images/dd_book_cate_icon.gif" alt="图书" /> 图书商品分类</div>
<div class="book_cate">[小说]</div>
<div class="book_sort_bottom">悬疑 | 言情 | 职场 | 财经</div>
<div class="book_cate">[文艺]</div>
<div class="book_sort_bottom">文学 | 传记 | 艺术 | 摄影</div>
<div class="book_cate">[青春]</div>
<div class="book_sort_bottom">青春文学 | 动漫 | 幽默</div>
<div class="book_cate">[励志/成功]</div>
<div class="book_sort_bottom">修养 | 成功 | 职场 | 沟通</div>
<div class="book_cate">[少儿]</div>
<div class="book_sort_bottom">0-2 | 3-6 | 7-10 | 11-14<br />
文学 | 科普 | 图画书</div>
<div class="book_cate">[生活]</div>
<div class="book_sort_bottom">保健 | 家教 | 美丽装扮 | 育儿 | 美食 | 旅游 | 收藏 | 生活 | 体育 | 地图 | 个人理财</div>
<div class="book_cate">[个人社科]</div>
<div class="book_sort_bottom">文化 | 历史 | 哲学/宗教 | 古籍 | 政治/历史 | 法律 | 经济 | 社会科学 | 心理学</div>
<div class="book_cate">[管理]</div>
<div class="book_sort_bottom">管理 | 金融 | 营销 | 会计</div>
<div class="book_cate">[科技]</div>
<div class="book_sort_bottom">科普 | 建筑 | 医学 | 计算机 | 农林 | 自然科学 | 工业 | 通信</div>
<div class="book_cate">[教育]</div>
<div class="book_sort_bottom">教材 | 中小学教辅 | 外语</div>
<div class="book_cate">[工具书]</div>
<div class="book_cate">[图外原版书]</div>
<div class="book_cate">[期刊]</div>
</div>
<!--图书商品分类结束-->
</div>
<!--左侧菜单结束-->
<!--中间部分开始-->
<div id="content">
<!--轮换显示的横幅广告图片-->
<div class="scroll_top"></div>
<div class="scroll_mid"> <img src="images/dd_scroll_2.jpg" alt="轮换显示的图片广告" id="dd_scroll"/>
<div id="scroll_number">
<ul>
<li id="scroll_number_1" onmouseover="loopShow(1)">1</li>
<li id="scroll_number_2" onmouseover="loopShow(2)">2</li>
<li id="scroll_number_3" onmouseover="loopShow(3)">3</li>
<li id="scroll_number_4" onmouseover="loopShow(4)">4</li>
<li id="scroll_number_5" onmouseover="loopShow(5)">5</li>
<li id="scroll_number_6" onmouseover="loopShow(6)">6</li>
</ul>
</div>
</div>
<div class="scroll_end"></div>
<!--最新上架开始-->
<div class="book_sort">
<div class="book_new">
<div class="book_left">最新上架</div>
<div class="book_type" id="history">历史</div>
<div class="book_type" id="family">家教</div>
<div class="book_type" id="culture">文化</div>
<div class="book_type" id="novel">小说</div>
<div class="book_right"><a href="#">更多>></a></div>
</div>
<div class="book_class" style="height:250px;">
<!--历史-->
<dl id="book_history">
<dt><img src="images/dd_history_1.jpg" alt="history"/></dt>
<dd><font class="book_title">《中国时代》(上)</font><br />
作者:师永刚,邹明 主编 <br />
出版社:作家出版社 <br />
<font class="book_publish">出版时间:2009年10月</font><br />
定价:¥39.00<br />
当当价:¥27.00 </dd>
<dt><img src="images/dd_history_2.jpg" alt="history"/></dt>
<dd><font class="book_title">中国历史的屈辱</font><br />
作者:王重旭 著 <br />
出版社:华夏出版社 <br />
<font class="book_publish">出版时间:2009年11月</font><br />
定价:¥26.00<br />
当当价:¥18.20 </dd>
<dt><img src="images/dd_history_3.jpg" alt="history"/></dt>
<dd><font class="book_title">《中国时代》(下)</font><br />
作者:师永刚,邹明 主编 <br />
出版社:作家出版社 <br />
<font class="book_publish"> 出版时间:2009年10月</font><br />
定价:¥38.00<br />
当当价:¥26.30 </dd>
<dt><img src="images/dd_history_4.jpg" alt="history"/></dt>
<dd><font class="book_title">大家国学十六讲</font><br />
作者:张荫麟,吕思勉 著 <br />
出版社:中国友谊出版公司 <br />
<font class="book_publish">出版时间:2009年10月</font><br />
定价:¥19.80<br />
当当价:¥13.70 </dd>
</dl>
<!--家教-->
<dl id="book_family" class="book_none">
<dt><img src="images/dd_family_1.jpg" alt="history"/></dt>
<dd><font class="book_title">嘿,我知道你</font><br />
作者:兰海 著 <br />
出版社:中国妇女出版社 <br />
<font class="book_publish">出版时间:2009年10月</font><br />
定价:¥28.80<br />
当当价:¥17.90 </dd>
<dt><img src="images/dd_family_2.jpg" alt="history"/></dt>
<dd><font class="book_title">择业要趁早</font><br />
作者:(美)列文<br />
出版社:海天出版社 <br />
<font class="book_publish">出版时间:2009年10月</font><br />
定价:¥28.00<br />
当当价:¥19.30 </dd>
<dt><img src="images/dd_family_3.jpg" alt="history"/></dt>
<dd><font class="book_title">爷爷奶奶的“孙子兵法”</font><br />
作者:伏建全 编著 <br />
出版社:地震出版社 <br />
<font class="book_publish"> 出版时间:2009年8月</font><br />
定价:¥28.00<br />
当当价:¥17.40 </dd>
<dt><img src="images/dd_family_4.jpg" alt="history"/></dt>
<dd><font class="book_title">1分钟读懂孩子心理</font><br />
作者:海韵 著 <br />
出版社:朝华出版社 <br />
<font class="book_publish">出版时间:2009年10月</font><br />
定价:¥28.00<br />
当当价:¥17.40 </dd>
</dl>
<!--文化-->
<dl id="book_culture" class="book_none">
<dt><img src="images/dd_culture_1.jpg" alt="history"/></dt>
<dd><font class="book_title">嘿,我知道你2</font><br />
作者:兰海 著 <br />
出版社:中国妇女出版社 <br />
<font class="book_publish">出版时间:2009年10月</font><br />
定价:¥28.80<br />
当当价:¥17.90 </dd>
<dt><img src="images/dd_culture_2.jpg" alt="history"/></dt>
<dd><font class="book_title">择业要趁早2</font><br />
作者:(美)列文 <br />
出版社:海天出版社 <br />
<font class="book_publish">出版时间:2009年10月</font><br />
定价:¥28.00<br />
当当价:¥19.30 </dd>
<dt><img src="images/dd_culture_3.jpg" alt="history"/></dt>
<dd><font class="book_title">爷爷奶奶的“孙子兵法”2</font><br />
作者:伏建全 编著 <br />
出版社:地震出版社 <br />
<font class="book_publish"> 出版时间:2009年8月</font><br />
定价:¥28.00<br />
当当价:¥17.40 </dd>
<dt><img src="images/dd_culture_4.jpg" alt="history"/></dt>
<dd><font class="book_title">1分钟读懂孩子心理2</font><br />
作者:海韵 著 <br />
出版社:朝华出版社 <br />
<font class="book_publish">出版时间:2009年10月</font><br />
定价:¥28.00<br />
当当价:¥17.40 </dd>
</dl>
<!--小说-->
<dl id="book_novel" class="book_none">
<dt><img src="images/dd_novel_1.jpg" alt="history"/></dt>
<dd><font class="book_title">嘿,我知道你3</font><br />
作者:兰海 著 <br />
出版社:中国妇女出版社 <br />
<font class="book_publish">出版时间:2009年10月</font><br />
定价:¥28.80<br />
当当价:¥17.90 </dd>
<dt><img src="images/dd_novel_2.jpg" alt="history"/></dt>
<dd><font class="book_title">择业要趁早3</font><br />
作者:(美)列文 <br />
出版社:海天出版社 <br />
<font class="book_publish">出版时间:2009年10月</font><br />
定价:¥28.00<br />
当当价:¥19.30 </dd>
<dt><img src="images/dd_novel_3.jpg" alt="history"/></dt>
<dd><font class="book_title">爷爷奶奶的“孙子兵法”3</font><br />
作者:伏建全 编著 <br />
出版社:地震出版社 <br />
<font class="book_publish"> 出版时间:2009年8月</font><br />
定价:¥28.00<br />
当当价:¥17.40 </dd>
<dt><img src="images/dd_novel_4.jpg" alt="history"/></dt>
<dd><font class="book_title">1分钟读懂孩子心理3</font><br />
作者:海韵 著 <br />
出版社:朝华出版社 <br />
<font class="book_publish">出版时间:2009年10月</font><br />
定价:¥28.00<br />
当当价:¥17.40 </dd>
</dl>
</div>
</div>
<!--重点关注-->
<div class="book_sort">
<div class="book_new">
<div class="book_left">重点关注</div>
</div>
<div class="book_class" style="height:380px;">
<dl id="book_focus">
<dt><img src="images/dd_focus_1.jpg" alt="focus"/></dt>
<dt><img src="images/dd_focus_2.jpg" alt="focus"/></dt>
<dt><img src="images/dd_focus_3.jpg" alt="focus"/></dt>
<dt><img src="images/dd_focus_4.jpg" alt="focus"/></dt>
<dd><a href="#" class="blue">郑玉巧育儿经·幼儿卷</a></dd>
<dd><a href="#" class="blue">蹦蹦和跳跳的故事(全10册)</a></dd>
<dd><a href="#" class="blue">人体自有大药(让每一个人都能变成...</a></dd>
<dd><a href="#" class="blue">特效穴位使用手册</a></dd>
<dd>定价:¥49.80<br />
当当价:¥32.00</dd>
<dd>定价:¥50.00<br />
当当价:¥33.00</dd>
<dd>定价:¥29.00<br />
当当价:¥19.40</dd>
<dd>定价:¥29.00<br />
当当价:¥19.40</dd>
<dt><img src="images/dd_focus_5.jpg" alt="focus"/></dt>
<dt><img src="images/dd_focus_6.jpg" alt="focus"/></dt>
<dt><img src="images/dd_focus_7.jpg" alt="focus"/></dt>
<dt><img src="images/dd_focus_8.jpg" alt="focus"/></dt>
<dd><a href="#" class="blue">《猫武士系列》(全6册)</a></dd>
<dd><a href="#" class="blue">求医不如求己养生救命大宝典</a></dd>
<dd><a href="#" class="blue">雅思词汇词根+联想记忆法</a></dd>
<dd><a href="#" class="blue">等待Nemo的日子</a></dd>
<dd>定价:¥120.00<br />
当当价:¥79.20</dd>
<dd>定价:¥198.00<br />
当当价:¥116.90</dd>
<dd>定价:¥28.00<br />
当当价:¥18.70</dd>
<dd>定价:¥26.00<br />
当当价:¥17.90</dd>
</dl>
</div>
</div>
</div>
<!--中间部分结束-->
<!--右侧部分开始-->
<div id="silder">
<!--书讯快递-->
<div class="book_sort">
<div class="book_sort_bg"><img src="images/dd_book_mess.gif" alt="mess" style=" vertical-align:text-bottom;"/>书讯快递</div>
<div class="book_class">
<div id="dome">
<div id="dome1">
<ul id="express">
<li>·2010考研英语大纲到货75折...</li>
<li>·权威定本四大名著(人民文...</li>
<li>·口述历史权威唐德刚先生国...</li>
<li>·袁伟民与体坛风云:实话实...</li>
<li>·我们台湾这些年:轰动两岸...</li>
<li>·畅销教辅推荐:精品套书50...</li>
<li>·2010版法律硕士联考大纲75...</li>
<li>·计算机新书畅销书75折抢购</li>
<li>·2009年孩子最喜欢的书>></li>
<li>·弗洛伊德作品精选集59折</li>
<li>·2010考研英语大纲到货75折...</li>
<li>·权威定本四大名著(人民文...</li>
<li>·口述历史权威唐德刚先生国...</li>
<li>·袁伟民与体坛风云:实话实...</li>
<li>·我们台湾这些年:轰动两岸...</li>
<li>·畅销教辅推荐:精品套书50...</li>
<li>·2010版法律硕士联考大纲75...</li>
<li>·计算机新书畅销书75折抢购</li>
<li>·2009年孩子最喜欢的书>></li>
<li>·弗洛伊德作品精选集59折</li>
<li>·2010考研英语大纲到货75折...</li>
<li>·权威定本四大名著(人民文...</li>
<li>·口述历史权威唐德刚先生国...</li>
<li>·袁伟民与体坛风云:实话实...</li>
<li>·我们台湾这些年:轰动两岸...</li>
<li>·畅销教辅推荐:精品套书50...</li>
<li>·2010版法律硕士联考大纲75...</li>
<li>·计算机新书畅销书75折抢购</li>
<li>·2009年孩子最喜欢的书>></li>
<li>·弗洛伊德作品精选集59折</li>
<li>·2010考研英语大纲到货75折...</li>
<li>·权威定本四大名著(人民文...</li>
<li>·口述历史权威唐德刚先生国...</li>
<li>·袁伟民与体坛风云:实话实...</li>
<li>·我们台湾这些年:轰动两岸...</li>
<li>·畅销教辅推荐:精品套书50...</li>
<li>·2010版法律硕士联考大纲75...</li>
<li>·计算机新书畅销书75折抢购</li>
<li>·2009年孩子最喜欢的书>></li>
<li>·弗洛伊德作品精选集59折</li>
</ul>
</div>
<div id="dome2"></div>
</div>
</div>
<div class="book_express_avder"> <img src="images/dd_book_right_adver1.jpg" alt="adver" style="margin-bottom:5px;" /> <img src="images/dd_book_right_adver2.gif" alt="adver" /> </div>
</div>
<!--近7日畅销榜-->
<div class="book_sort">
<div class="book_seven_title">近7日畅销榜 <img src="images/dd_bang.gif" alt="bang" style="vertical-align:top;"/></div>
<div class="book_seven_border">
<div class="book_seven_top">
<ul id="book_seven_cate">
<li>动漫</li>
<li>小说</li>
<li>外语</li>
<li>旅游</li>
<li>励志</li>
</ul>
</div>
<div class="book_seven_content">
<div class="book_seven_content_left">
<dl id="book_seven_number">
<dt><img src="images/dd_book_no_01.gif" alt="book"/></dt>
<dt><img src="images/dd_book_no_02.gif" alt="book"/></dt>
<dt><img src="images/dd_book_no_03.gif" alt="book"/></dt>
<dd><img src="images/dd_book_no_04.gif" alt="book"/></dd>
<dd><img src="images/dd_book_no_05.gif" alt="book"/></dd>
<dd><img src="images/dd_book_no_06.gif" alt="book"/></dd>
<dd><img src="images/dd_book_no_07.gif" alt="book"/></dd>
<dd><img src="images/dd_book_no_08.gif" alt="book"/></dd>
<!--<dd><img src="images/dd_book_no_09.gif" alt="book"/></dd>
<dd><img src="images/dd_book_no_10.gif" alt="book"/></dd>-->
</dl>
</div>
<div class="book_seven_content_right">
<!--励志开始-->
<div id="book_seven_hearten">
<dl>
<dt><img src="images/dd_seven_hearten_01.jpg" alt="hearten" /></dt>
<dd><a href="#" class="blue">不抱怨的世界</a><br />
作者:(美)鲍温<br />
出版社:陕西师范<br />
出版时间:2009年4月</dd>
<dt><img src="images/dd_seven_hearten_02.jpg" alt="hearten" /></dt>
<dd><a href="#" class="blue">遇见未知的自己</a><br />
作者:张德芬 <br />
出版社:华夏出版<br />
出版时间:2008年1月</dd>
<dt><img src="images/dd_seven_hearten_03.jpg" alt="hearten" /></dt>
<dd><a href="#" class="blue">活法</a><br />
作者:(日)稻盛<br />
出版社:东方出版<br />
出版时间:2005年3月</dd>
</dl>
<ul>
<li><a href="#" class="blue">高效能人士的七个习惯</a></li>
<li><a href="#" class="blue">被迫强大</a></li>
<li><a href="#" class="blue">遇见心想事成的自己</a></li>
<li><a href="#" class="blue">世界上最伟大的推销员</a></li>
<li><a href="#" class="blue">我的成功可以复制</a></li>
</ul>
</div>
<!--励志结束-->
</div>
</div>
</div>
</div>
</div>
<!--右侧部分结束-->
<script type="text/javascript" src="js/book.js"></script>
</div>
<!--网站版权部分开始-->
<div id="footer">
<div class="footer_top"><a href="#" target="_parent" class="footer_dull_red">公司简介</a> | <a href="#" target="_parent" class="footer_dull_red">诚证英才</a> | <a href="#" target="_parent" class="footer_dull_red">网站联盟</a> | <a href="#" target="_parent" class="footer_dull_red">百货招商</a> | <a href="#" target="_parent" class="footer_dull_red">交易条款</a></div>
<iframe src="footer.html" height="50px" width="900px" frameborder="0"></iframe>
</div>
</body>
</html>
- index.js
/**
* Created by Administrator on 2020/7/13.
*/
var imgs=["images/dd_scroll_1.jpg",
"images/dd_scroll_2.jpg",
"images/dd_scroll_3.jpg",
"images/dd_scroll_4.jpg",
"images/dd_scroll_5.jpg",
"images/dd_scroll_6.jpg"];
var currentNumber=1;
var maxNumber=6;
///loop imgs
function loopShow(num){
if(num){
currentNumber=parseInt(num);
}
var img=document.querySelector("#dd_scroll");
img.setAttribute("src",imgs[currentNumber-1]);
///
var imgControllerBtns=document.querySelectorAll("#scroll_number li");
for(var i=0; i<imgControllerBtns.length;i++){
imgControllerBtns[i].className="scroll_number_out";
}
imgControllerBtns[currentNumber-1].className="scroll_number_over";
currentNumber++;
if(currentNumber>maxNumber){
currentNumber=1;
}
}
///tabs
onload=function() {
setInterval(loopShow,1000);
var slideDoorBtns=document.querySelectorAll(".book_sort .book_type");
var doors=document.querySelectorAll(".book_class dl");
for(var i=0;i<slideDoorBtns.length;i++){
slideDoorBtns[i].index=i;
slideDoorBtns[i].onmouseover=function () {
this.className="book_type_out";
for(var j=0;j<doors.length;j++) {
if (j == this.index) {
doors[j].className = "book_show";
} else {
doors[j].className = "book_none";
}
}
slideDoorBtns[i].onmouseout=function () {
this.className="book_type";
}
}
}
///scroll
var dome=document.querySelector("#dome");
var dome1=document.querySelector("#dome1");
var dome2=document.querySelector("#dome2");
dome2.innerHTML=dome1.innerHTML;
function moveUp(){
dome.scrollTop++;
if(dome.scrollTop>=dome1.offsetHeight){
dome.scrollTop=0;
}
}
var interval= setInterval(moveUp,10);
dome.onmouseover=function(){
clearInterval(interval);
}
dome.onmouseout=function(){
interval=setInterval(moveUp,10);
}
///float Ad
var btnShutAd=document.querySelector("#shutAd");
btnShutAd.onclick=function(){
var right=document.querySelector("#right");
right.style.display="none";
}
window.open("http://www.bing.com","height=500,width=300");
}
- css
- global.CSS
@charset "utf-8";
/* CSS Document */
body{
margin:0px;
padding:0px;
font-size:12px;
line-height:20px;
color:#333;
}
ul,li,ol,h1,dl,dd{
list-style:none;
margin:0px;
padding:0px;
}
a{
color:#333333;
text-decoration: none;
}
a:hover{
color:#333333;
text-decoration:underline;
}
img{
border:0px;
}
.blue{
color:#1965b3;
text-decoration:none;
}
.blue:hover{
color:#1965b3;
text-decoration:underline;
}
#header,#main,#footer{
width:960px;
margin:0px auto 0px auto;
clear:both;
float:none;
}
/*网页版权部分样式开始*/
.footer_top{
width:800px;
margin:0px auto 0px auto;
clear:both;
text-align:center;
}
.footer_top{
color:#9B2B0F;
}
.footer_dull_red,.footer_dull_red:hover{
color:#9B2B0F;
margin:0px 8px 0px 8px;
}
/*网页版权部分样式结束*/
- layout.CSS
@charset "utf-8";
/* CSS Document */
/*首页样式*/
/*右侧随鼠标滚动的广告图片*/
.right{
top:50px;
right:30px;
position:absolute;
z-index:3;
}
.dd_close{
width:35px;
height:18px;
text-align:center;
border:solid 1px #999;
background-color:#E0E0E0;
top:0px;
right:0px;
position:absolute;
}
/*通栏广告样式*/
.dd_index_top_adver{
margin:5px 0px 5px 0px;
clear:both;
}
#catList,#content,#silder{
float:left;
}
#catList{
width:180px;
margin-right:10px;
margin-top:10px;
}
#content{
width:540px;
margin-right:10px;
}
#silder{
width:220px;
margin-top:10px;
}
.book_sort{
border:solid 1px #999;
margin-bottom:10px;
}
.book_sort_bg{
background-color:#fff0d9;
padding-left:10px;
color:#882D00;
font-size:14px;
height:25px;
font-weight:bold;
line-height:30px;
}
.book_sort_bottom{
margin:0px 10px 0px 10px;
line-height:25px;
border-bottom:solid 1px #666;
}
.book_cate{
padding:10px 0px 0px 10px;
font-weight:bold;
}
.scroll_top{
background-image:url(../images/dd_scroll_top.gif);
width:540px;
height:51px;
background-repeat:no-repeat;
}
.scroll_mid{
background-color:#f2f2f3;
border-left:solid 1px #d6d5d6;
border-right:solid 1px #d6d5d6;
width:533px;
padding:5px 0px 5px 5px;
}
#dd_scroll{ /*FF*/
float:none;
}
*html #dd_scroll{
float:left; /*IE6*/
}
*+html #dd_scroll{
float:left; /*IE7*/
}
#scroll_number{
float:right;
padding-right:10px;
}
#scroll_number li{
width:13px;
height:13px;
text-align:center;
border:solid 1px #999;
margin-top:5px;
font-size:12px;
line-height:16px;
cursor:pointer;
}
.scroll_number_out{
}
.scroll_number_over{
background-color:#F96;
color:#FFF;
}
.scroll_end{
background-image:url(../images/dd_scroll_end.gif);
width:540px;
height:8px;
background-repeat:no-repeat;
margin-bottom:10px;
}
.book_new{
background-image:url(../images/dd_book_bg.jpg);
background-repeat:repeat-x;
height:25px;
line-height:30px;
clear:both;
}
.book_left{
margin:0px 50px 0px 10px;
color:#882D00;
font-size:14px;
font-weight:bold;
float:left;
}
.book_type{
float:left;
margin-left:3px;
background-image:url(../images/dd_book_bg1.jpg);
background-repeat:no-repeat;
width:40px;
height:23px;
margin-top:2px;
text-align:center;
cursor:pointer;
}
.book_type_out{
float:left;
margin-left:3px;
background-image:url(../images/dd_book_bg2.jpg);
background-repeat:no-repeat;
width:40px;
height:23px;
margin-top:2px;
text-align:center;
color:#882D00;
font-weight:bold;
cursor:pointer;
}
.book_right{
float:right;
margin-right:5px;
}
.book_class{
clear:both;
margin:0px 5px 0px 5px;
}
#dome{
overflow:hidden; /*溢出的部分不显示*/
height:250px;
padding:5px;
}
#book_history dt,#book_family dt,#book_novel dt,#book_culture dt{
float:left;
width:90px;
text-align:center;
}
#book_history dd,#book_family dd,#book_novel dd,#book_culture dd{
float:left;
width:170px;
margin:0px 0px 5px 0px;
}
.book_none{
display:none;
}
.book_show{
display:block;
}
.book_title{
color:#1965b3;
font-size:14px;
}
.book_publish{
color:#C00;
}
#book_focus dt{
width:125px;
margin:5px 0px 0px 7px;
float:left;
text-align:center;
height:90px;
display:inline;
}
#book_focus dd{
width:125px;
margin:5px 0px 0px 7px;
float:left;
height:40px;
display:inline;
}
#express li{
height:25px;
border-bottom:dashed 1px #999;
margin:0px 5px 0px 5px;
line-height:28px;
}
.book_express_avder{
margin:5px 0px 5px 0px;
text-align:center;
}
.book_seven_title{
background-color:#518700;
color:#ffffff;
font-size:14px;
height:25px;
padding-left:10px;
line-height:28px;
}
.book_seven_border{
background-color:#f2f4df;
margin:3px;
}
.book_seven_top{
background-image:url(../images/dd_seven_bg.jpg);
background-repeat:repeat-x;
height:18px;
clear:both;
}
.book_seven_content{
border:solid 1px #bdc1c4;
border-top:0px;
clear:both;
padding:3px;
height:375px;
}
#book_seven_cate li{
float:right;
background-image:url(../images/dd_seven_bg1.jpg);
background-repeat:no-repeat;
width:35px;
height:18px;
text-align:center;
cursor:pointer;
}
#book_seven_cate li:hover{
float:right;
background-image:url(../images/dd_seven_bg2.jpg);
background-repeat:no-repeat;
width:35px;
height:18px;
text-align:center;
color:#882D00;
}
.book_seven_content_left{
float:left;
width:22px;
}
.book_seven_content_right{
width:175px;
float:left;
}
#book_seven_number dt{
height:85px;
}
#book_seven_number dd{
height:25px;
}
#book_seven_hearten dt{
width:55px;
text-align:center;
float:left;
}
#book_seven_hearten dd{
width:120px;
float:left;
height:85px;
}
#book_seven_hearten ul li{
line-height:24px;
}
/*网页版权部分样式开始*/
.footer_top,.footer_end{
width:800px;
margin:0px auto 0px auto;
clear:both;
text-align:center;
}
.footer_top{
color:#9B2B0F;
}
.footer_dull_red,.footer_dull_red:hover{
color:#9B2B0F;
margin:0px 8px 0px 8px;
}
/*网页版权部分样式结束*/
/*当当网商品展示页样式开始*/
.current_place{
padding-left:10px;
clear:both;
height:30px;
}
#product_left{
width:180px;
float:left;
}
#product_catList{
border:solid 1px #d3d3d3;
background-color:#fff4d7;
}
.product_catList_top{
color:#FFF;
height:23px;
font-size:14px;
padding-left:10px;
background-image:url(../images/product_left_top_bg.gif);
background-repeat:repeat-x;
line-height:28px;
margin-bottom:10px;
}
#product_catList_class li{
height:25px;
padding-left:10px;
}
.product_catList_end{
border:solid 1px #d3d3d3;
margin:10px 0px 10px 0px;
text-align:center;
}
#product_storyList{
border:solid 1px #a1a1a1;
float:right;
width:770px;
margin-bottom:20px;
}
#product_storyList_top{
background-color:#f9d8a9;
border-bottom:solid 1px #a1a1a1;
height:30px;
}
#product_storyList_top li{
float:left;
line-height:28px;
padding-left:5px;
}
#product_storyList_top img{
padding-top:5px;}
.product_storyList_content{
margin:20px 10px 20px 10px;
}
.product_storyList_content_bottom{
border-bottom:1px solid #666;
clear:both;
margin-bottom:20px;
}
.product_storyList_content_left{
width:100px;
text-align:center;
float:left;
}
.product_storyList_content_right{
float:left;
width:640px;
}
.product_storyList_content_dash{
border-bottom:dashed 1px #666;
}
.blue_14{
color:#1965b3;
font-size:14px;
text-decoration:none;
}
.blue_14:hover{
color:#1965b3;
text-decoration:underline;
font-size:14px;
}
.product_content_dd dd{
float:right;
padding-right:10px;
}
.product_content_delete{
text-decoration:line-through;
}
#product_page li{
float:left;
}
.product_page{
width:16px;
height:15px;
text-decoration:none;
float:left;
display: block;
background-color:#FC6;
margin:0px 3px 1px 0px;
text-align:center;
}
.product_page:hover{
width:16px;
height:15px;
text-decoration:none;
float:left;
display: block;
background-color:#900;
margin:0px 3px 1px 0px;
color:#FFF;
}
/*购物车页面样式开始*/
.shopping_commend{
background-image:url(../images/shopping_commend_bg.gif);
background-repeat:repeat-x;
height:21px;
border:solid 1px #999;
}
.shopping_commend_left{
float:left;
padding-left:10px;
}
.shopping_commend_right{
float:right;
padding-right:10px;
margin-top:3px;
}
.shopping_commend_right img{
cursor:pointer;
}
#shopping_commend_sort{
border:solid 1px #999;
border-top:0;
padding:5px 20px 5px 20px;
height:120px;
}
.shopping_commend_sort_left{
float:left;
width:450px;
}
.shopping_commend_sort_mid{
float:left;
width:15px;
background-image:url(../images/shopping_dotted.gif);
background-repeat:repeat-y;
height:120px;
}
.shopping_commend_list_1,.shopping_commend_list_2,.shopping_commend_list_3,.shopping_commend_list_4{
float:left;
height:30px;
line-height:30px;
}
.shopping_commend_list_1{
width:240px;
}
.shopping_commend_list_2{
width:70px;
text-align:center;
text-decoration:line-through;
color:#999;
}
.shopping_commend_list_3{
width:70px;
text-align:center;
}
.shopping_commend_list_4{
text-align:center;
width:65px;
}
.shopping_yellow{
color:#ED610C;
}
.shopping_yellow:hover{
color:#ED610C;
text-decoration:underline;
}
.shopping_list_top{
clear:both;
font-size:14px;
font-weight:bold;
margin-top:20px;
}
.shopping_list_border{
border:solid 2px #999;
}
.shopping_list_title{
background-color:#d8e4c6;
height:25px;
}
.shopping_list_title li{
float:left;
line-height:28px;
}
.shopping_list_title_1{
width:420px;
padding-left:30px;
text-align:left;
}
.shopping_list_title_2{
width:120px;
text-align:center;
}
.shopping_list_title_3{
width:120px;
text-align:center;
}
.shopping_list_title_4{
width:120px;
text-align:center;
}
.shopping_list_title_5{
width:70px;
text-align:center;
}
.shopping_list_title_6{
width:70px;
text-align:center;
}
.shopping_product_list{
background-color:#fefbf2;
height:40px;
}
.shopping_product_list input{
width:30px;
height:15px;
border:solid 1px #666;
text-align:center;
}
.shopping_product_list td{
line-height:35px;
border-bottom:dashed 1px #CCC;
}
.shopping_product_list_1{
width:420px;
padding-left:30px;
text-align:left;
}
.shopping_product_list_2{
width:120px;
text-align:center;
color:#464646;
}
.shopping_product_list_3{
width:120px;
text-align:center;
color:#464646;
}
.shopping_product_list_4{
width:120px;
text-align:center;
color:#191919;
}
.shopping_product_list_5{
width:70px;
text-align:center;
}
.shopping_product_list_6{
width:70px;
text-align:center;
}
.shopping_list_end{
background-color:#cddbb8;
height:60px;
}
.shopping_list_end li{
float:right;
}
.shopping_list_end_1{
margin:10px 10px 0px 10px;
}
.shopping_list_end_2{
font-weight:bold;
color:#BD3E00;
font-size:14px;
margin:15px 10px 0px 0px;
}
.shopping_list_end_3{
font-weight:bold;
font-size:14px;
margin:15px 0px 0px 15px;
}
.shopping_list_end_4{
border-right:solid 1px #666666;
margin:10px 0px 0px 15px;
padding-right:10px;
}
.shopping_list_end_yellow{
color:#BD3E00;
}
/*注册页面样式*/
#register_header{
background-image:url(../images/register_head_bg.gif);
background-repeat:repeat-x;
height:48px;
border:solid 1px #CCC;
}
.register_header_left{
float:left;
margin:7px 0px 0px 40px;
display:inline;
}
.register_header_right{
float:right;
margin:25px 20px 0px 0px;
display:inline;
}
.register_content{
width:950px;
margin:15px auto 15px auto;
}
.register_top_bg{
background-image:url(../images/register_top_bg.gif);
background-repeat:no-repeat;
height:5px;
}
.register_mid_bg{
border:solid 1px #a3a3a3;
border-top:0px;
height:32px;
}
.register_mid_bg li{
float:left;
}
.register_mid_left{
background-image:url(../images/register_tag_left.gif);
height:32px;
width:207px;
background-repeat:no-repeat;
padding:0px 0px 0px 106px;
font-size:14px;
color:#501500;
font-weight:bold;
line-height:35px;
}
.register_mid_mid{
background-image:url(../images/register_tag_mid.gif);
height:32px;
width:204px;
background-repeat:no-repeat;
padding:0px 0px 0px 115px;
font-size:14px;
line-height:35px;
}
.register_mid_right{
background-image:url(../images/register_tag_right.gif);
height:32px;
width:316px;
background-repeat:no-repeat;
font-size:14px;
line-height:35px;
text-align:center;
}
.register_top_bg_two_left{
float:left;
background-image:url(../images/register_cont.gif);
width:1px;
height:406px;
background-repeat:no-repeat;
}
.register_top_bg_two_right{
float:right;
background-image:url(../images/register_cont.gif);
width:1px;
height:406px;
background-repeat:no-repeat;
}
.register_top_bg_mid{
background-image:url(../images/register_shadow.gif);
background-repeat:repeat-x;
}
.register_title_bg{
background-image:url(../images/register_pic_01.gif);
background-position:123px 30px;
background-repeat:no-repeat;
padding:42px 0px 0px 202px;
color:#C30;
}
.register_dotted_bg{
background-image:url(../images/register_dotted.gif);
background-repeat:repeat-x;
height:1px;
margin:0px 20px 20px 20px;
}
.register_message{
float:left;
}
.register_row{
clear:both;
}
.register_row dt{
float:left;
width:200px;
height:30px;
text-align:right;
font-size:14px;
}
.register_row dd{
float:left;
margin-right:5px;
display:inline;
}
.register_input{
width:200px;
height:18px;
border:solid 1px #999;
margin:0px 0px 8px 0px;
}
.registerBtn{
height:35px;
margin:10px 0px 10px 200px;
clear:both;
}
/*注册页面提示样式*/
.register_input_Blur{
background-color:#fef4d0;
width:200px;
height:18px;
border:solid 1px #999;
margin:0px 0px 8px 0px;
}
.register_input_Focus{
background-color:#f1ffde;
width:200px;
height:18px;
border:solid 1px #999;
margin:0px 0px 8px 0px;
}
.register_prompt{
font-size:12px;
color:#999;
}
.register_prompt_error{
font-size:12px;
color:#C8180B;
border:solid 1px #999;
background-color:#fef4d0;
padding:0px 5px 0px 5px;
height:18px;
}
.register_prompt_ok{
background-image:url(../images/register_write_ok.gif);
background-repeat:no-repeat;
width:15px;
height:11px;
margin:5px 0px 0px 5px;
}
/*登录页面样式*/
.login_header_left{
float:left;
margin:30px 10px 0px 100px;
}
.login_header_mid{
float:left;
margin:55px 0px 0px 0px;
}
.login_header_right{
float:right;
margin:50px 60px 0px 0px;
}
.login_main_left{
float:left;
}
.login_main_left img{
float:left;
border:0px;
}
.login_main_end{
margin:0px 0px 0px 30px;
width:540px;
}
.login_green{
margin:20px 0px 20px 0px;
float:left;
width:150px;
}
.login_green dt{
color:#519403;
font-weight:bold;
}
.login_green dd{
color:#666666;
line-height:25px;
}
.login_main_dotted{
float:left;
background-image:url(../images/shopping_dotted.gif);
width:1px;
height:90px;
margin:20px 15px 20px 15px;
}
.login_main_mid{
border:solid 1px #666;
float:left;
width:300px;
padding:10px 5px 10px 5px;
}
.login_main_right{
float:left;
width:74px;
}
.login_main_right img{
border:0px;
}
.login_content_top{
background-image:url(../images/login_icon_bg_01.gif);
background-position:-300px -30px;
background-repeat:no-repeat;
padding:10px 0px 0px 35px;
font-weight:bold;
font-size:14px;
color:#900;
}
.login_content_line{
background-image:url(../images/login_icon_bg_02.gif);
background-position:0px -195px;
background-repeat:repeat-x;
height:3px;
margin:0px 0px 30px 0px;
}
.login_content{
clear:both;
margin:10px 0px 0px 0px;
}
*html .login_content{/*IE6*/
display:inline;
}
*+html .login_content{/*IE7*/
display:inline;
}
.login_content dt{
font-size:14px;
height:30px;
text-align:right;
width:150px;
float:left;
}
.login_content dd{
float:left;
}
.login_content_input{
width:120px;
height:16px;
border:solid 1px #999;
}
.login_content_input_Focus{
background-color:#f1ffde;
width:120px;
height:16px;
border:solid 1px #999;
}
.login_btn_out{
background-image:url(../images/login_icon_bg_01.gif);
background-position:0px -30px;
background-repeat:no-repeat;
width:77px;
height:26px;
border:0px;
cursor:pointer;
}
.login_btn_over{
background-image:url(../images/login_icon_bg_01.gif);
background-position:-78px -30px;
background-repeat:no-repeat;
width:77px;
height:26px;
border:0px;
cursor:pointer;
}
.login_content_dotted{
background-image:url(../images/register_dotted.gif);
height:1px;
background-repeat:repeat-x;
margin:60px 0px 0px 0px;
overflow:hidden; /*使div的高度为1px*/
}
.login_content_end_bg{
background-image:url(../images/login_icon_bg_02.gif);
background-repeat:repeat-x;
background-position:0px 0px;
padding:10px 20px 10px 20px;
}
.login_content_end_bg_top{
clear:both;
}
.login_content_bold{
font-weight:bold;
color:#333;
}
.login_content_end_bg_end{
clear:both;
text-align:right;
padding:10px;
}
.login_register_out{
background-image:url(../images/login_icon_bg_01.gif);
background-position:0px -3px;
background-repeat:no-repeat;
width:144px;
height:26px;
border:0px;
cursor:pointer;
}
.login_register_over{
background-image:url(../images/login_icon_bg_01.gif);
background-position:-144px -3px;
background-repeat:no-repeat;
width:144px;
height:26px;
border:0px;
cursor:pointer;
}
- template.css
@charset "gb2312";
/* CSS Document */
/*网页头部导航样式开始*/
.header_top,.header_middle,.header_search{
margin-left:auto;
margin-right:auto;
width:955px;
clear:both;
}
.header_top{
border:solid 1px #999;
background-image:url(../images/dd_header_bg.gif);
background-repeat:repeat-x;
height:24px;
}
.header_top_left{
float:left;
width:260px;
padding-left:10px;
line-height:28px;
}
*html .header_top_left{ /*only IE6*/
line-height:24px;
}
.header_top_right{
float:right;
padding-right:10px;
width:400px;
text-align:right;
}
.header_top_right li{
float:right;
margin-left:5px;
margin-top:5px;
}
.logo,.menu_left,.menu_right{
float:left;
}
.logo{
width:130px;
padding-top:13px;
height:47px;
}
.menu_left{
height:28px;
padding-top:32px;
line-height:35px;
width:510px;
}
*html .menu_left{ /*only IE6*/
line-height:28px;
}
#menu_left_bold li{
float:left;
background-image:url(../images/dd_head_bg_mid.gif);
height:28px;
background-repeat:repeat-x;
padding:0px 3px 0px 3px;
}
.bold,.bold:hover{
font-weight:bold;
}
.menu_left_first{
background-image:url(../images/dd_head_bg_left.gif);
background-repeat:no-repeat;
background-position:0px 0px;
height:28px;
width:4px;
float:left;
}
.menu_left_end{
background-image:url(../images/dd_head_bg_right.gif);
background-repeat:no-repeat;
background-position:0px 0px;
height:28px;
width:4px;
float:left;
}
.menu_right{
padding-top:32px;
height:28px;
}
#menu_dull_red li{
float:left;
margin-left:5px;
text-align:center;
line-height:35px;
height:28px;
}
*html #menu_dull_red li{ /*only IE6*/
line-height:28px;
}
#menu_dull_red a,#menu_dull_red:hover{
color:#9B2B0F;
text-decoration:none;
font-weight:bold;
}
.menu_right_1{
background-image:url(../images/dd_header_1_a.jpg);
width:52px;
background-repeat:no-repeat;
}
.menu_right_2{
background-image:url(../images/dd_header_2_a.jpg);
width:39px;
background-repeat:no-repeat;
}
.menu_right_3{
background-image:url(../images/dd_header_3_a.jpg);
width:65px;
background-repeat:no-repeat;
}
.menu{
clear:both;
}
#menu_white{
float:left;
background-image:url(../images/dd_head_bg_mid.gif);
background-repeat:repeat-x;
background-position:0px -63px;
height:27px;
width:99%;
line-height:28px;
text-align:center;
color:#FFF;
}
.menu_mid_white,.menu_mid_white:hover{
color:#FFF;
padding:0px 4px 0px 4px;
}
.menu_first{
background-image:url(../images/dd_head_bg_left.gif);
background-repeat:no-repeat;
background-position:0px -31px;
height:27px;
width:4px;
float:left;
}
.menu_end{
background-image:url(../images/dd_head_bg_right.gif);
background-repeat:no-repeat;
background-position:0px -31px;
height:27px;
width:4px;
float:left;
}
.header_search{
padding-top:2px;
}
.header_serach_left,.header_serach_mid,.header_serach_right{
float:left;
height:35px;
}
.header_serach_left{
background-image:url(../images/dd_head_bg_left.gif);
background-repeat:no-repeat;
background-position:0px -58px;
width:4px;
}
.header_serach_mid{
background-image:url(../images/dd_head_bg_mid.gif);
background-repeat:repeat-x;
background-position:0px -28px;
width:99%;
}
.header_serach_right{
background-image:url(../images/dd_head_bg_right.gif);
background-repeat:no-repeat;
background-position:0px -58px;
width:4px;
}
#header_serach_mid_menu li{
float:left;
margin-top:6px;
padding:0px 5px 0px 5px;
line-height:25px;
}
.header_input_search{
margin-left:15px;
width:200px;
height:18px;
}
.header_secrch_btn{
}
/*网页头部导航样式结束*/
/*网页版权部分样式开始*/
.footer_top,.footer_end{
width:800px;
margin:0px auto 0px auto;
clear:both;
text-align:center;
}
.footer_top{
color:#9B2B0F;
}
.footer_dull_red,.footer_dull_red:hover{
color:#9B2B0F;
margin:0px 8px 0px 8px;
}
/*网页版权部分样式结束*/
/*导航部分下拉菜单样式*/
#dd_menu_top_down{
position:absolute;
width:80px;
text-align:left;
border:solid 1px #999;
border-top:0px;
display:none;
background-color:#FFF;
padding-left:10px;
}
*html #dd_menu_top_down{/*only IE6*/
right:30px;
top:25px;
}
*+html #dd_menu_top_down{/*only IE7*/
right:30px;
top:25px;
}
#dd_menu_top_down li{
float:none;
}