Android 用 OKhttp3 下载文件
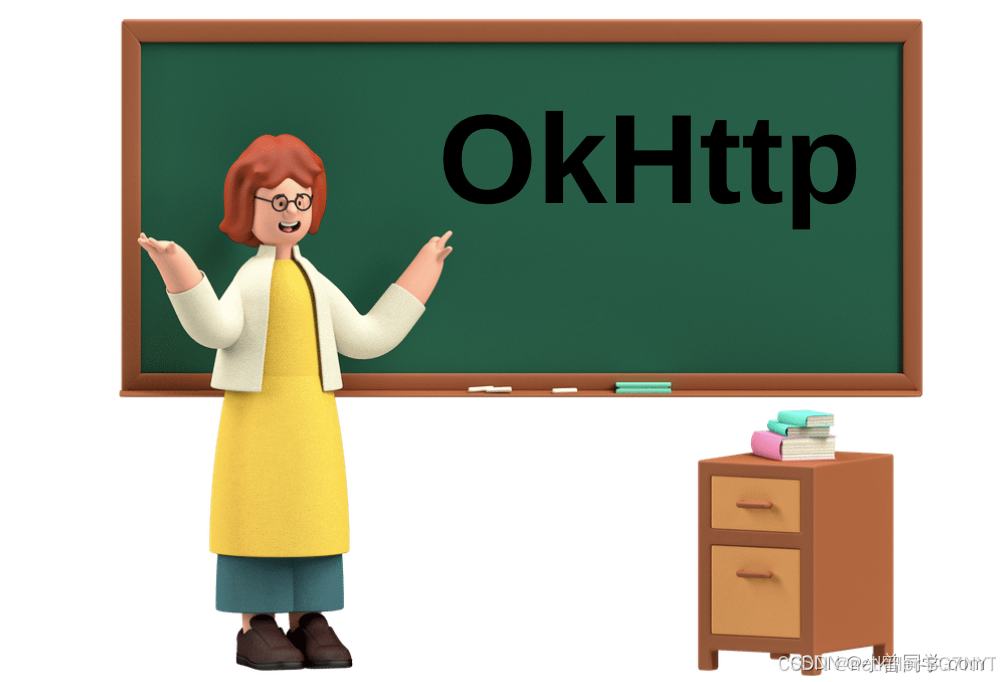
package cn.netkilller.conference.utils;
import android.os.Environment;
import android.util.Log;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class Download {
private static final String TAG = Download.class.getSimpleName();
public Download() {
}
public void downloadToDirectory(final String url, final String directory, final OnDownloadListener listener) throws IOException {
String filename = url.substring(url.lastIndexOf("/") + 1);
String savePath = isExistDir(directory);
File file = new File(savePath, filename);
download(url, file, listener);
}
public void downloadToFile(final String url, final String filename, final OnDownloadListener listener) throws IOException {
File file = new File(Environment.getExternalStorageDirectory() + "/Download", filename);
download(url, file, listener);
}
public void download(final String url, File file, final OnDownloadListener listener) {
OkHttpClient okHttpClient = new OkHttpClient();
Request request = new Request.Builder().url(url).build();
okHttpClient.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
listener.onFailure();
}
@Override
public void onResponse(Call call, Response response) throws IOException {
InputStream inputStream = null;
byte[] buf = new byte[2048];
int len = 0;
FileOutputStream fileOutputStream = null;
try {
inputStream = response.body().byteStream();
long total = response.body().contentLength();
Log.d(TAG, url + " => " + file.getAbsolutePath());
fileOutputStream = new FileOutputStream(file);
long sum = 0;
while ((len = inputStream.read(buf)) != -1) {
fileOutputStream.write(buf, 0, len);
sum += len;
int progress = (int) (sum * 1.0f / total * 100);
listener.onDownloading(progress);
}
fileOutputStream.flush();
listener.onSuccess(file.getAbsolutePath());
} catch (Exception e) {
listener.onFailure();
} finally {
try {
if (inputStream != null)
inputStream.close();
} catch (IOException e) {
}
try {
if (fileOutputStream != null)
fileOutputStream.close();
} catch (IOException e) {
}
}
}
});
}
private String isExistDir(String saveDir) throws IOException {
File downloadFile = new File(Environment.getExternalStorageDirectory(), saveDir);
if (!downloadFile.mkdirs()) {
downloadFile.createNewFile();
}
String savePath = downloadFile.getAbsolutePath();
return savePath;
}
public interface OnDownloadListener {
/**
* 下载成功
*/
void onSuccess(String filename);
/**
* @param progress 下载进度
*/
void onDownloading(int progress);
/**
* 下载失败
*/
void onFailure();
}
}