from ctypes import *
import socket
import struct
class IP(Structure):
_fields_ = [
("ihl", c_uint8, 4),
("version", c_uint8, 4),
("tos", c_uint8),
("len", c_uint16),
("id", c_uint16),
("offset", c_uint16),
("ttl", c_uint8),
("protocol_num", c_uint8),
("sum", c_uint16),
("src", c_uint32),
("dst", c_uint32)
]
def __new__(self, buffer=None):
return self.from_buffer_copy(buffer)
def __init__(self, buffer=None):
print(self.ihl.__hex__())
print(self.version.__hex__())
print(self.tos.__hex__())
print(self.len.__hex__())
print(self.id.__hex__())
print(self.offset.__hex__())
print(self.ttl.__hex__())
print(self.protocol_num.__hex__())
print(self.sum.__hex__())
print(socket.inet_ntoa(struct.pack('<L', self.src)))
print(socket.inet_ntoa(struct.pack('<L', self.dst)))
if __name__ == '__main__':
buffer = 'E\x00\x00T\xd2\xce@\x00@\x01i\xd8\x7f\x00\x00\x01\x7f\x00\x00\x01'
ip = IP(buffer)
$ python decode_ipheader.py
0x5
0x4
0x0
0x5400
0xced2
0x40
0x40
0x1
0xd869
127.0.0.1
127.0.0.1
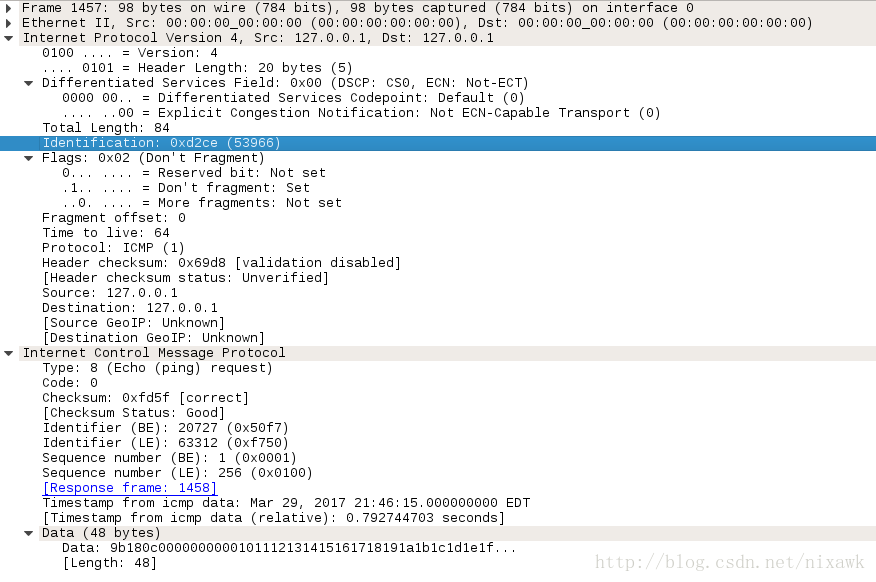
Sniffer
from ctypes import *
import socket
import struct
class IP(Structure):
_fields_ = [
("ihl", c_uint8, 4),
("version", c_uint8, 4),
("tos", c_uint8),
("len", c_uint16),
("id", c_uint16),
("offset", c_uint16),
("ttl", c_uint8),
("protocol_num", c_uint8),
("sum", c_uint16),
("src", c_uint32),
("dst", c_uint32)
]
def __new__(self, buffer=None):
return self.from_buffer_copy(buffer)
def __init__(self, buffer=None):
self.protocol_map = {1: "ICMP", 6: "TCP", 17: "UDP"}
self.src_address = socket.inet_ntoa(struct.pack("<L", self.src))
self.dst_address = socket.inet_ntoa(struct.pack("<L", self.dst))
try:
self.protocol = self.protocol_map[self.protocol_num]
except:
self.protocol = str(self.protocol_num)
if __name__ == '__main__':
if os.name == "nt":
socket_protocol = socket.IPPROTO_IP
else:
socket_protocol = socket.IPPROTO_ICMP
sniffer = socket.socket(socket.AF_INET, socket.SOCK_RAW, socket_protocol)
sniffer.bind((host, 0))
sniffer.setsockopt(socket.IPPROTO_IP, socket.IP_HDRINCL, 1)
if os.name == "nt":
sniffer.ioctl(socket.SIO_RCVALL, socket.RCVALL_ON)
try:
while True:
raw_buffer = sniffer.recvfrom(65535)[0]
ip_header = IP(raw_buffer[0:20])
print("Protocol: {} {} -> {}".format(
ip_header.protocol, ip_header.src_address, ip_header.dst_address
))
except KeyboardInterrupt:
if os.name == "nt":
sniffer.ioctl(socket.SIO_RCVALL, socket.RCVALL_OFF)
References
- BOOK: BLACK HAT PYTHON: Python Programming for Hackers and Pentesters