Description
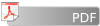
Killer Problem
Killer Problem |
You are given an array of N integers and Q queries. Each query is a closed interval [l, r]. You should find the minimum absolute difference between all pairs in that interval.
Input
First line contains an integer
T (
T
10).
T sets follow. Each set begins with an integer
N (
N
200000). In the next line there are
N integers
ai (
1
ai
104), the number in the
i-th cell of the array. Next line will contain
Q (
Q
104).
Q lines follow, each containing two integers
li,
ri (
1
li,
ri
N,
li < ri) describing the beginning and ending of of
i-th range. Total number of queries will be less than 15000.







Output
For the
i-th query of each test output the minimum
| aj–ak| for
li
j,
k
ri (
j
k) a single line.



Sample Input
1 10 1 2 4 7 11 10 8 5 1 10000 4 1 10 1 2 3 5 8 10
Sample Output
0 1 3 4
题意:给定一个整数数组,对每组的询问L,R,输出区间差最小是多少
思路:排序后处理,但是这样会超时,因为题目说和不超过15000,所以最差也就只有15000个数
#include <iostream>
#include <cstring>
#include <algorithm>
#include <cstdio>
using namespace std;
const int maxn = 200005;
const int inf = 0x3f3f3f3f;
int num[maxn];
int tmp[maxn];
int n, q;
void cal(int l, int r) {
if (r - l + 1 > 15000) {
printf("0\n");
return;
}
int cnt = 0;
for (int i = l; i <= r; i++)
tmp[cnt++] = num[i];
sort(tmp, tmp+cnt);
int Min = inf;
for (int i = 0; i < cnt-1; i++)
Min = min(Min, tmp[i+1]-tmp[i]);
printf("%d\n", Min);
}
int main() {
int t;
scanf("%d", &t);
while (t--) {
scanf("%d", &n);
for (int i = 1; i <= n; i++)
scanf("%d", &num[i]);
scanf("%d", &q);
int l, r;
while (q--) {
scanf("%d%d", &l, &r);
cal(l, r);
}
}
return 0;
}