MyArray
#ifndef _MYARRAY_H_
#define _MYARRAY_H_
#include <limits>
namespace PoEdu
{
template<typename T>
class MyArray
{
public:
MyArray();
MyArray(const MyArray &other);
MyArray(size_t n, const T &val);
MyArray(const T *first, const T *last);
MyArray &operator=(const MyArray &other);
~MyArray();
T *Begin() const;
T *End() const;
T *Rbegin() const;
T *Rend() const;
size_t Size() const;
size_t Max_size() const;
void Resize(size_t len, T str);
size_t Capacity() const;
bool Empty() const;
void Reserve(size_t len);
T &operator[](size_t index);
const T &operator[](size_t index) const;
T &At(size_t index);
const T &At(size_t index) const;
T &Front();
const T &Front() const;
T &Back();
const T &Back() const;
void Assign(size_t len, const T& other);
void Push_back(const T &val);
void Pop_back();
void Insert(size_t pos, size_t len, const T &other);
T *Erase(size_t pos);
T *Erase(size_t first, size_t last);
void Swap(MyArray &other);
void Clear();
private:
T *data_;
size_t size_;
size_t capacity_;
const size_t max_size_;
};
template<typename T>
MyArray<T>::MyArray() : max_size_(UINT_MAX / 4)
{
size_ = 0;
capacity_ = 5;
data_ = new T[capacity_];
}
template<typename T>
inline MyArray<T>::MyArray(const MyArray & other) : max_size_(UINT_MAX / 4)
{
if (&other != this)
{
size_ = other.size_;
capacity_ = other.capacity_;
data_ = new T[capacity_];
for (size_t i = 0; i < size_; ++i)
{
data_[i] = other.data_[i];
}
}
}
template<typename T>
MyArray<T>::MyArray(size_t n, const T & val) : max_size_(UINT_MAX / 4)
{
size_ = n;
capacity_ = 5;
while (capacity_ < size_)
{
capacity_ *= 2;
}
data_ = new T[size_];
for (size_t i = 0; i < size_; ++i)
{
data_[i] = val;
}
}
template<typename T>
MyArray<T>::MyArray(const T * first, const T * last) : max_size_(UINT_MAX / 4)
{
size_ = (last - first) / sizeof(T);
data_ = new T[capacity_];
T *pAddr = const_cast<T *>(first);
for (size_t i = 0; i < size_; ++i)
{
data_[i] = *pAddr;
pAddr += sizeof(T);
}
}
template<typename T>
MyArray<T> & MyArray<T>::operator=(const MyArray & other)
{
if (&other != this)
{
delete[] data_;
size_ = other.size_;
capacity_ = other.capacity_;
data_ = new T[capacity_];
for (size_t i = 0; i < size_; ++i)
{
data_[i] = other.data_[i];
}
}
return *this;
}
template<typename T>
MyArray<T>::~MyArray()
{
delete[] data_;
}
template<typename T>
T * MyArray<T>::Begin() const
{
return data_;
}
template<typename T>
T * MyArray<T>::End() const
{
return &(data_[size_]);
}
template<typename T>
T * MyArray<T>::Rbegin() const
{
return End();
}
template<typename T>
T * MyArray<T>::Rend() const
{
return Begin();
}
template<typename T>
size_t MyArray<T>::Size() const
{
return size_;
}
template<typename T>
size_t MyArray<T>::Max_size() const
{
return size_;
}
template<typename T>
void MyArray<T>::Resize(size_t len, T str)
{
if (len < size_)
{
for (size_t index = size_ - 1; index >= len; --index)
{
Pop_back();
}
}
else
{
for (size_t index = size_; index < len; ++index)
{
Push_back(str);
}
}
}
template<typename T>
size_t MyArray<T>::Capacity() const
{
return capacity_;
}
template<typename T>
bool MyArray<T>::Empty() const
{
return !size_;
}
template<typename T>
void MyArray<T>::Reserve(size_t len)
{
if (len > size_)
{
for (size_t index = size_; index < len; ++index)
{
Push_back("");
}
}
}
template<typename T>
T& MyArray<T>::operator[](size_t index)
{
return const_cast<T&>(static_cast<const MyArray &>(*this)[index]);
}
template<typename T>
const T & MyArray<T>::operator[](size_t index) const
{
return data_[index];
}
template<typename T>
T & MyArray<T>::At(size_t index)
{
return const_cast<T &>(static_cast<const MyArray &>(*this).At(index));
}
template<typename T>
const T & MyArray<T>::At(size_t index) const
{
size_t temp = index;
if (index >= size_)
{
std::cout << "超出范围" << std::endl;
temp = size_ - 1;
}
return data_[temp];
}
template<typename T>
T & MyArray<T>::Front()
{
return const_cast<T &>(static_cast<const MyArray &>(*this).Front());
}
template<typename T>
const T & MyArray<T>::Front() const
{
return At(0);
}
template<typename T>
T & MyArray<T>::Back()
{
return const_cast<T &>(static_cast<const MyArray &>(*this).Back());
}
template<typename T>
const T & MyArray<T>::Back() const
{
return At(size_ - 1);
}
template<typename T>
void MyArray<T>::Assign(size_t len, const T & other)
{
delete[] data_;
memset(data_, 0, capacity_ * sizeof(T));
if (len < size_)
{
for (size_t index = 0; index < len; ++index)
{
data_[index] = other;
}
}
else
{
size_ = len;
while (capacity_ < size_)
{
capacity_ *= 2;
}
data_ = new T[capacity_];
for (size_t index = 0; index < size_; ++index)
{
data_[index] = other;
}
}
}
template<typename T>
void MyArray<T>::Push_back(const T & val)
{
while (capacity_ < size_)
{
capacity_ += 5;
}
T *temp = new T[size_ + 1];
for (size_t i = 0; i < size_; ++i)
{
temp[i] = data_[i];
}
temp[size_] = val;
size_ += 1;
delete[] data_;
data_ = temp;
}
template<typename T>
void MyArray<T>::Pop_back()
{
T *temp = new T[capacity_];
memset(temp, 0, capacity_ * sizeof(T));
--size_;
for (size_t index = 0; index < size_; ++index)
{
temp[index] = data_[index];
}
delete[] data_;
data_ = temp;
}
template<typename T>
void MyArray<T>::Insert(size_t pos, size_t len, const T & other)
{
T *temp = new T[size_ + len];
for (size_t index = 0; index < pos; ++index)
{
temp[index] = data_[index];
}
for (size_t index = pos; index < pos + len; ++index)
{
temp[index] = other;
}
for (size_t index = pos + len; index < size_ + len; ++index)
{
temp[index] = data_[index - len];
}
size_ += len;
delete[] data_;
data_ = temp;
}
template<typename T>
T * MyArray<T>::Erase(size_t pos)
{
T *temp = new T[capacity_];
memset(temp, 0, capacity_ * sizeof(T));
for (size_t index = 0; index < pos; ++index)
{
temp[index] = data_[index];
}
for (size_t index = pos; index < size_ - 1; ++index)
{
temp[index] = data_[index + 1];
}
--size_;
delete[] data_;
data_ = temp;
return &(data_[pos]);
}
template<typename T>
T * MyArray<T>::Erase(size_t first, size_t last)
{
T *temp = new T[capacity_];
memset(temp, 0, capacity_ * sizeof(T));
for (size_t index = 0; index < first; ++index)
{
temp[index] = data_[index];
}
for (size_t index = first; index < size_ - (last - first); ++index)
{
temp[index] = data_[index + last - first];
}
size_ -= (last - first);
delete[] data_;
data_ = temp;
return &(data_[first]);
}
template<typename T>
void MyArray<T>::Swap(MyArray & other)
{
MyArray temp(other);
other = *this;
*this = temp;
}
template<typename T>
void MyArray<T>::Clear()
{
delete[] data_;
}
}
#endif
#include "MyArray.h"
都在头文件中已经实现了
MyStack
#ifndef _MYSTACK_H_
#define _MYSTACK_H_
#include "MyArray.h"
namespace PoEdu
{
template <typename T, typename CON = MyArray<T> >
class MyStack
{
public:
MyStack() : c(){}
MyStack(const MyStack &other) : c(other){}
explicit MyStack(const CON &val) : c(val){}
MyStack& operator=(const MyStack& other)
{
c = other.c;
return (*this);
}
bool Empty() const
{
return c.Empty();
}
size_t Size() const
{
return c.Size();
}
T &Top()
{
return c.Back();
}
const T &Top() const
{
return c.Back();
}
void Push(const T& val)
{
c.Push_back(val);
}
void Emplace(const T& val)
{
c.Push_back(val);
}
void Pop()
{
c.Pop_back();
}
void Swap(MyStack &other) noexcept
{
c.Swap(other.c);
}
protected:
CON c;
};
}
#endif
Test
#include <iostream>
#include "MyStack.h"
int main()
{
using namespace PoEdu;
MyStack<int, MyArray<int> > Demo;
MyStack<int, MyArray<int> > Other;
std::cout << "Demo before Push.." << std::endl;
std::cout << "whether Empty: " << Demo.Empty() << std::endl;
std::cout << "Demo test Push, Top, Size, and Empty..." << std::endl;
Demo.Push(1);
std::cout << "Push element: " << 1 << std::endl;
std::cout << "Top elmemt: " << Demo.Top() << std::endl;
std::cout << "stack size: " << Demo.Size() << std::endl;
std::cout << "whether Empty: " << Demo.Empty() << std::endl;
Demo.Push(2);
std::cout << "Push element: " << 2 << std::endl;
std::cout << "Top elmemt: " << Demo.Top() << std::endl;
std::cout << "stack Size: " << Demo.Size() << std::endl;
std::cout << "whether Empty: " << Demo.Empty() << std::endl;
std::cout << std::endl;
std::cout << "Other before Emplace.." << std::endl;
std::cout << "whether Empty: " << Other.Empty() << std::endl;
std::cout << "Other test Emplace, Top, Size, and Empty..." << std::endl;
Other.Emplace(11);
std::cout << "Emplace element: " << 11 << std::endl;
std::cout << "top Elmemt: " << Other.Top() << std::endl;
std::cout << "stack Size: " << Other.Size() << std::endl;
std::cout << "whether Empty: " << Other.Empty() << std::endl;
Other.Emplace(12);
std::cout << "emplace Element: " << 12 << std::endl;
std::cout << "top Elmemt: " << Other.Top() << std::endl;
std::cout << "stack Size: " << Other.Size() << std::endl;
std::cout << "whether Empty: " << Other.Empty() << std::endl;
std::cout << std::endl;
std::cout << "test Swap, Top..." << std::endl;
Demo.Swap(Other);
std::cout << "Demo Top: " << Demo.Top() << std::endl;
std::cout << "Other top: " << Other.Top() << std::endl;
std::cout << std::endl;
std::cout << "Demo test Pop.." << std::endl;
std::cout << "Demo before Pop.." << std::endl;
std::cout << "Top elmemt: " << Demo.Top() << std::endl;
std::cout << "stack size: " << Demo.Size() << std::endl;
std::cout << "whether Empty: " << Demo.Empty() << std::endl;
Demo.Pop();
std::cout << "Top elmemt: " << Demo.Top() << std::endl;
std::cout << "stack Size: " << Demo.Size() << std::endl;
std::cout << "whether Empty: " << Demo.Empty() << std::endl;
Demo.Pop();
std::cout << "whether Empty: " << Demo.Empty() << std::endl;
std::cout << std::endl;
return 0;
}
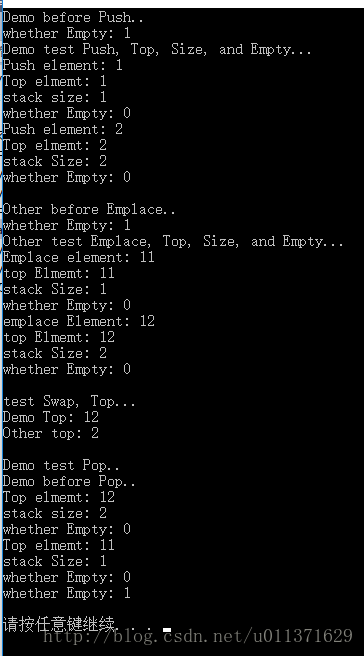