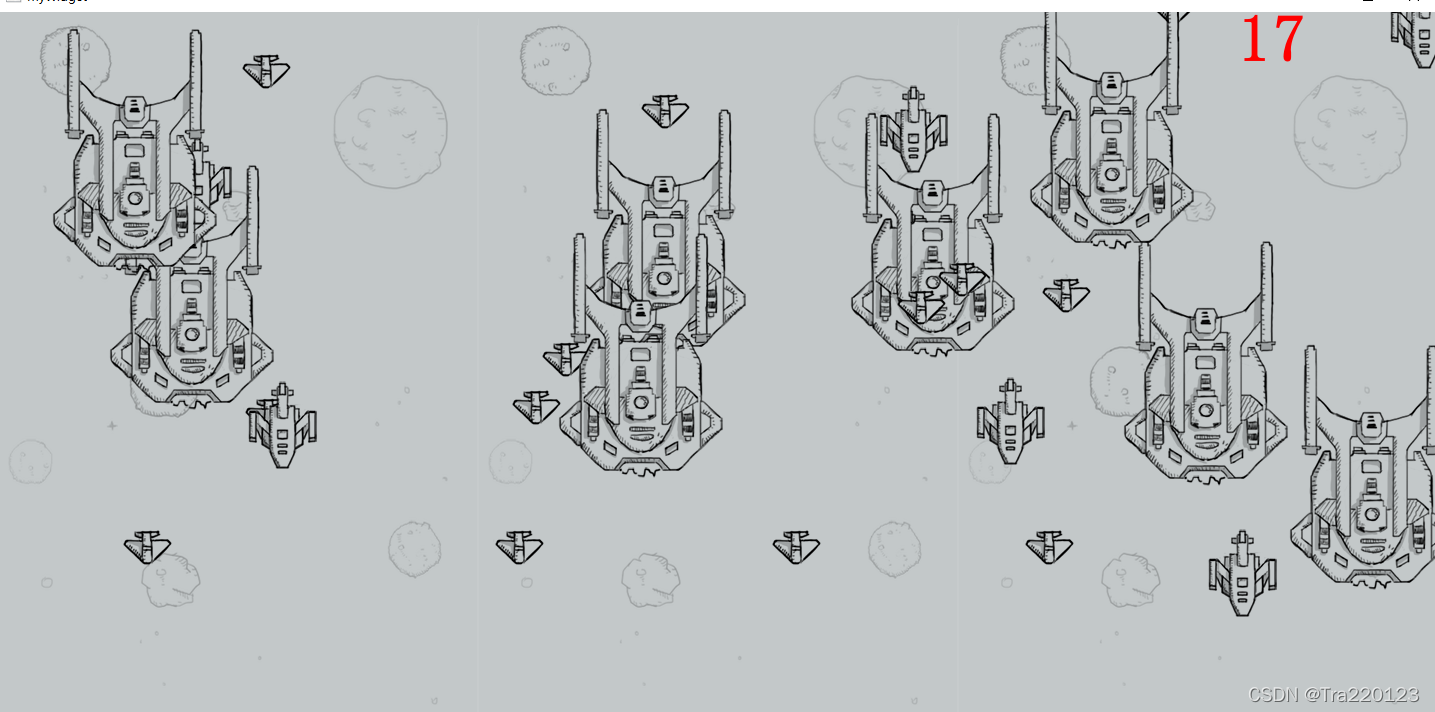
Headers:
1.mywidget.h
#ifndef MYWIDGET_H
#define MYWIDGET_H
#include <QWidget>
#include "uipicture.h"
QT_BEGIN_NAMESPACE
namespace Ui { class MyWidget; }
QT_END_NAMESPACE
class MyWidget : public QWidget
{
Q_OBJECT
public slots:
void refreshPaint();
public:
MyWidget(QWidget *parent = nullptr);
~MyWidget();
void paintEvent(QPaintEvent *event) override;
void keyPressEvent(QKeyEvent *event) override;
void mousePressEvent(QMouseEvent *event) override;
UiPicture *uipic;
QTimer *refreshPaintTimer;
private:
Ui::MyWidget *ui;
};
#endif // MYWIDGET_H
2.objinsky.h
#ifndef OBJINSKY_H
#define OBJINSKY_H
#include <QString>
#include <QPoint>
#include <QPixmap>
class ObjInSky
{
private:
QPoint _pos;
QString _shape;
Qt::GlobalColor _color;
int _size;
public:
ObjInSky(const QPoint &pos, QString shape, Qt::GlobalColor color=Qt::white, int size=20);
virtual ~ObjInSky();
Qt::GlobalColor color() const;
//modified 20220317
QPoint pos() const;
void setPos(QPoint newPos);
const QString &shape() const;
void setShape(const QString &newShape);
void setColor(Qt::GlobalColor newColor);
int size() const;
void setSize(int newSize);
virtual bool drop();
virtual void reset2Top()=0;
virtual QPixmap getPixmap()=0;
virtual QPoint getSize()=0;
};
#endif // OBJINSKY_H
3.plane.h
#ifndef PLANE_H
#define PLANE_H
#include "objinsky.h"
#include <QPixmap>
class Plane : public ObjInSky
{
private:
QPixmap img;
int imgW;
int imgH;
public:
Plane(const QPoint &pos, QString shape="X", Qt::GlobalColor color=Qt::yellow, int size=20);
~Plane();
QPixmap getPixmap() override;
QPoint getSize() override;
// bool drop() override;
void reset2Top() override;
};
#endif // PLANE_H
4.uipicture.h
#ifndef UIPICTURE_H
#define UIPICTURE_H
#include <QPainter>
#include "objinsky.h"
class UiPicture
{
private:
QPainter *painter;
QVector<ObjInSky *> objarr;
public:
UiPicture(QPainter *p, int n);
void drawBackground();
void drawObjects();
void drawScore();
void drawAll();
virtual ~UiPicture();
void setPainter(QPainter *newPainter);
void refresh();
void judgeKey(QString key);
void judgeMouse(int x, int y);
int score;
};
#endif // UIPICTURE_H
5.util.h
#ifndef UTIL_H
#define UTIL_H
#include <QPoint>
#include <QDebug>
class Util
{
static int width;
static int height;
public:
Util()=delete;
static int getWidth();
static int getHeight();
static void setWidth(int newWidth);
static void setHeight(int newHeight);
static quint32 rand();
static QPoint randPos();
};
#endif // UTIL_H
Sources:
1.mywidget.cpp
#include "mywidget.h"
#include "ui_mywidget.h"
#include <QDebug>
#include <QPainter>
#include "star.h"
#include "util.h"
#include <QTimer>
#include <QKeyEvent>
MyWidget::MyWidget(QWidget *parent)
: QWidget(parent)
, ui(new Ui::MyWidget)
{
ui->setupUi(this);
setGeometry(100, 100, 1440, 700);
Util::setHeight(this->height());
Util::setWidth(this->width());
//Star *ps = new Star(QPoint(Util::getWidth(), Util::getHeight()));
Util::setHeight(this->height());
Util::setWidth(this->width());
uipic = new UiPicture(nullptr, 30);
refreshPaintTimer = new QTimer(this);
connect(refreshPaintTimer, &QTimer::timeout, this, &MyWidget::refreshPaint);
refreshPaintTimer->start(100);
}
MyWidget::~MyWidget()
{
refreshPaintTimer->stop();
delete refreshPaintTimer;
delete uipic;
delete ui;
}
void MyWidget::paintEvent(QPaintEvent *event)
{
Q_UNUSED(event);
Util::setHeight(this->height());
Util::setWidth(this->width());
QPainter p(this);
uipic->setPainter(&p);
uipic->drawAll();
//Star s(QPoint(50, 100));
//p.setPen(s.color());
//p.drawText(s.pos(), s.shape());
}
void MyWidget::refreshPaint()
{
uipic->refresh();
this->update();
}
void MyWidget::keyPressEvent(QKeyEvent *event)
{
//qDebug() << QString(event->key());
uipic->judgeKey(QString(event->key()));
}
void MyWidget::mousePressEvent(QMouseEvent *event)
{
uipic->judgeMouse(event->x(), event->y());
}
2.objinsky.cpp
#include "objinsky.h"
#include "util.h"
Qt::GlobalColor ObjInSky::color() const
{
return _color;
}
QPoint ObjInSky::pos() const
{
return _pos;
}
void ObjInSky::setPos(QPoint newPos)
{
_pos = newPos;
}
const QString &ObjInSky::shape() const
{
return _shape;
}
void ObjInSky::setShape(const QString &newShape)
{
_shape = newShape;
}
void ObjInSky::setColor(Qt::GlobalColor newColor)
{
_color = newColor;
}
int ObjInSky::size() const
{
return _size;
}
void ObjInSky::setSize(int newSize)
{
_size = newSize;
}
ObjInSky::ObjInSky(const QPoint &pos, QString shape, Qt::GlobalColor color, int size)
:_pos(pos), _shape(shape), _color(color), _size(size)
{
}
ObjInSky::~ObjInSky()
{
}
bool ObjInSky::drop()
{
setPos(QPoint(pos().x(), pos().y() + 4)); //10
if (pos().y() > Util::getHeight())
{
reset2Top();
return true;
}
return false;
}
3.plane.cpp
#include "plane.h"
#include "util.h"
Plane::Plane(const QPoint &pos, QString shape, Qt::GlobalColor color, int size)
:ObjInSky(pos, shape, color, size)
{
reset2Top();
}
QPixmap Plane::getPixmap()
{
return img;
}
QPoint Plane::getSize()
{
return QPoint(imgW, imgH);
}
Plane::~Plane()
{
}
void Plane::reset2Top()
{
setPos(QPoint(Util::rand() % Util::getWidth(), -250)); //根据图片长度解决图片从上闪现进来
switch (Util::rand() % 3)
{
case 0:
img = QPixmap(":/images/enemy1.png");
imgW = 57;
imgH = 43;
break;
case 1:
img = QPixmap(":/images/enemy2.png");
imgW = 69;
imgH = 99;
break;
case 2:
img = QPixmap(":/images/enemy3_n1.png");
imgW = 169;
imgH = 258;
break;
}
}
4.uipicture.cpp
#include "uipicture.h"
#include "util.h"
#include "star.h"
#include "letter.h"
#include "objinsky.h"
#include "plane.h"
void UiPicture::setPainter(QPainter *newPainter)
{
painter = newPainter;
}
UiPicture::UiPicture(QPainter *p, int n)
:painter(p), objarr(n), score(0)
{
//qDebug() << "uipic contructor";
for (int i = 0; i < n; i++)
{
objarr[i] = new Plane(QPoint(Util::randPos()));
}
//qDebug() << "uipic contructor over";
}
void UiPicture::drawBackground()
{
// for letter
//painter->setBrush(Qt::black);
//painter->drawRect(0, 0, Util::getWidth(), Util::getHeight());
QPixmap background(":/images/background.png");
painter->drawPixmap(0, 0, 480, 700, background);
painter->drawPixmap(480, 0, 480, 700, background);
painter->drawPixmap(960, 0, 480, 700, background);
}
void UiPicture::drawObjects()
{
//qDebug() << "drawObjects";
for (int i = 0; i < objarr.size(); i++)
{
painter->drawPixmap(objarr[i]->pos(), objarr[i]->getPixmap());
}
}
void UiPicture::drawScore()
{
painter->setPen(Qt::red);
painter->setFont(QFont("Times", 50, QFont::Bold));
painter->drawText(Util::getWidth()-200, 50, QString::number(score));
}
void UiPicture::drawAll()
{
//qDebug() << "drawall";
drawBackground();
drawObjects();
drawScore();
}
UiPicture::~UiPicture()
{
for (int i = 0; i < objarr.size(); i++)
{
delete objarr[i];
}
}
void UiPicture::refresh()
{
for (int i = 0; i < objarr.size(); i++)
{
score -= objarr[i]->drop();
}
}
void UiPicture::judgeKey(QString key)
{
int lowest = 0;
int where = -1;
for (int i = 0; i < objarr.size(); i++)
{
if (objarr[i]->shape() == key)
{
if (objarr[i]->pos().y() > lowest)
{
lowest = objarr[i]->pos().y();
where = i;
}
}
}
if (where > -1)
{
objarr[where]->reset2Top();
score++;
} else {
score--;
}
}
void UiPicture::judgeMouse(int x, int y)
{
for (int i = 0; i < objarr.size(); i++)
{
if (x > objarr[i]->pos().x() && x < objarr[i]->pos().x() + objarr[i]->getSize().x() &&
y > objarr[i]->pos().y() && y < objarr[i]->pos().y() + objarr[i]->getSize().y())
{
objarr[i]->reset2Top();
score++;
return;
}
}
score--;
}
5.util.cpp
#include "util.h"
#include <QRandomGenerator>
int Util::width=0;
int Util::height=0;
int Util::getWidth()
{
return width;
}
int Util::getHeight()
{
return height;
}
void Util::setWidth(int newWidth)
{
width = newWidth;
}
void Util::setHeight(int newHeight)
{
height = newHeight;
}
quint32 Util::rand()
{
return QRandomGenerator::global()->generate();
}
QPoint Util::randPos()
{
return QPoint(rand() % width, rand() % height);
}