QuertZ入门
QuertZ保留执行结果,留待下次调用
QuertZ耗时任务等待执行完成
QuertZ常用的定时策略
QuertZ监控日志
-QuertZ在任务执行过程中提供了两类监控日志。一类是监控定时任务运行的日志,一类是监控框架本身运行状况及问题的日志。
- 定时任务监控日志----JobListener,继承IJobListener接口,实现如下
public class CustomJobListener : IJobListener
{
public string Name => "任务运行状况监控日志";
/// <summary>
/// 任务被终止时,执行此操作
/// </summary>
/// <param name="context"></param>
/// <param name="cancellationToken"></param>
/// <returns></returns>
public async Task JobExecutionVetoed(IJobExecutionContext context, CancellationToken cancellationToken = default)
{
await Task.Run(() =>
{
Console.WriteLine("任务被终止,添加需要的处理逻辑");
});
}
/// <summary>
/// 任务即将被执行时,执行此操作
/// </summary>
/// <param name="context"></param>
/// <param name="cancellationToken"></param>
/// <returns></returns>
public async Task JobToBeExecuted(IJobExecutionContext context, CancellationToken cancellationToken = default)
{
await Task.Run(() =>
{
Console.WriteLine("任务即将被执行时,执行此操作");
});
}
/// <summary>
/// 任务执行以后,执行此操作
/// </summary>
/// <param name="context"></param>
/// <param name="jobException"></param>
/// <param name="cancellationToken"></param>
/// <returns></returns>
public async Task JobWasExecuted(IJobExecutionContext context, JobExecutionException jobException, CancellationToken cancellationToken = default)
{
await Task.Run(() =>
{
Console.WriteLine("任务执行以后,执行此操作");
});
}
}
- 定时任务监控日志----TriggerListener
public class CustomTriggerListener : ITriggerListener
{
public string Name => "时间策略监控日志";
/// <summary>
/// 时间策略完成时,执行此操作
/// </summary>
/// <param name="trigger"></param>
/// <param name="context"></param>
/// <param name="triggerInstructionCode"></param>
/// <param name="cancellationToken"></param>
/// <returns></returns>
public async Task TriggerComplete(ITrigger trigger, IJobExecutionContext context, SchedulerInstruction triggerInstructionCode, CancellationToken cancellationToken = default)
{
await Task.Run(() =>
{
Console.WriteLine($"TriggerComplete:{trigger.JobKey.Name}");
});
}
/// <summary>
/// Trigger被激发 它关联的job即将被运行
/// </summary>
/// <param name="trigger"></param>
/// <param name="context"></param>
/// <param name="cancellationToken"></param>
/// <returns></returns>
public async Task TriggerFired(ITrigger trigger, IJobExecutionContext context, CancellationToken cancellationToken = default)
{
await Task.Run(() =>
{
Console.WriteLine($"TriggerFired:{trigger.JobKey.Name}");
});
}
/// <summary>
/// 当Trigger错过被激发时执行,比如当前时间有很多触发器都需要执行,但是线程池中的有效线程都在工作,
/// 那么有的触发器就有可能超时,错过这一轮的触发。
/// </summary>
/// <param name="trigger"></param>
/// <param name="cancellationToken"></param>
/// <returns></returns>
public async Task TriggerMisfired(ITrigger trigger, CancellationToken cancellationToken = default)
{
await Task.Run(() =>
{
Console.WriteLine($"TriggerMisfired:{trigger.JobKey.Name}");
});
}
/// <summary>
/// 是否中断任务,返回true任务被中断
/// </summary>
/// <param name="trigger"></param>
/// <param name="context"></param>
/// <param name="cancellationToken"></param>
/// <returns></returns>
public async Task<bool> VetoJobExecution(ITrigger trigger, IJobExecutionContext context, CancellationToken cancellationToken = default)
{
await Task.Run(() =>
{
Console.WriteLine("中断任务之前运行");
});
//此处中断任务后,将执行CustomJobListener的JobExecutionVetoed方法
return true;
}
}
- 定时任务监控日志----SchedulerListener
public class CustomSchedulerListener : ISchedulerListener
{
public async Task JobAdded(IJobDetail jobDetail, CancellationToken cancellationToken = default)
{
await Task.Run(() =>
{
Console.WriteLine($"{jobDetail.Key.Name} 添加进来了");
});
}
public async Task JobDeleted(JobKey jobKey, CancellationToken cancellationToken = default)
{
await Task.Run(() =>
{
Console.WriteLine($"{jobKey.Name} 删除了");
});
}
public Task JobInterrupted(JobKey jobKey, CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task JobPaused(JobKey jobKey, CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task JobResumed(JobKey jobKey, CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task JobScheduled(ITrigger trigger, CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task JobsPaused(string jobGroup, CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task JobsResumed(string jobGroup, CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task JobUnscheduled(TriggerKey triggerKey, CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task SchedulerError(string msg, SchedulerException cause, CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task SchedulerInStandbyMode(CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task SchedulerShutdown(CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task SchedulerShuttingdown(CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task SchedulerStarted(CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task SchedulerStarting(CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task SchedulingDataCleared(CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task TriggerFinalized(ITrigger trigger, CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task TriggerPaused(TriggerKey triggerKey, CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task TriggerResumed(TriggerKey triggerKey, CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task TriggersPaused(string triggerGroup, CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
public Task TriggersResumed(string triggerGroup, CancellationToken cancellationToken = default)
{
throw new NotImplementedException();
}
}
- 定时任务监控添加
#region 创建单元----时间轴
StdSchedulerFactory factory = new StdSchedulerFactory();
IScheduler scheduler = await factory.GetScheduler();
await scheduler.Start();
#endregion
#region 添加监控日志
scheduler.ListenerManager.AddJobListener(new CustomJobListener());
scheduler.ListenerManager.AddTriggerListener(new CustomTriggerListener());
scheduler.ListenerManager.AddSchedulerListener(new CustomSchedulerListener());
#endregion
- 框架本身监控日志----LogProvider,展示框架运行信息
public class CustomLogProviderListener : ILogProvider
{
public Logger GetLogger(string name)
{
return new Logger((level, func, exception, parameters) =>
{
if (level >= LogLevel.Info && func != null)
{
Console.WriteLine($"[{DateTime.Now.ToLongTimeString()}] [{level}] [{func()}] {string.Join(":", parameters.Select(t => t == null ? "" : t.ToString()))} 自定义日志{name}");
}
return true;
});
}
public IDisposable OpenMappedContext(string key, object value, bool destructure = false)
{
throw new NotImplementedException();
}
public IDisposable OpenNestedContext(string message)
{
throw new NotImplementedException();
}
}
QuertZ系列文章只讲解了基础入门知识,后续在用的过程中,继续补充和深入。
谢谢打赏
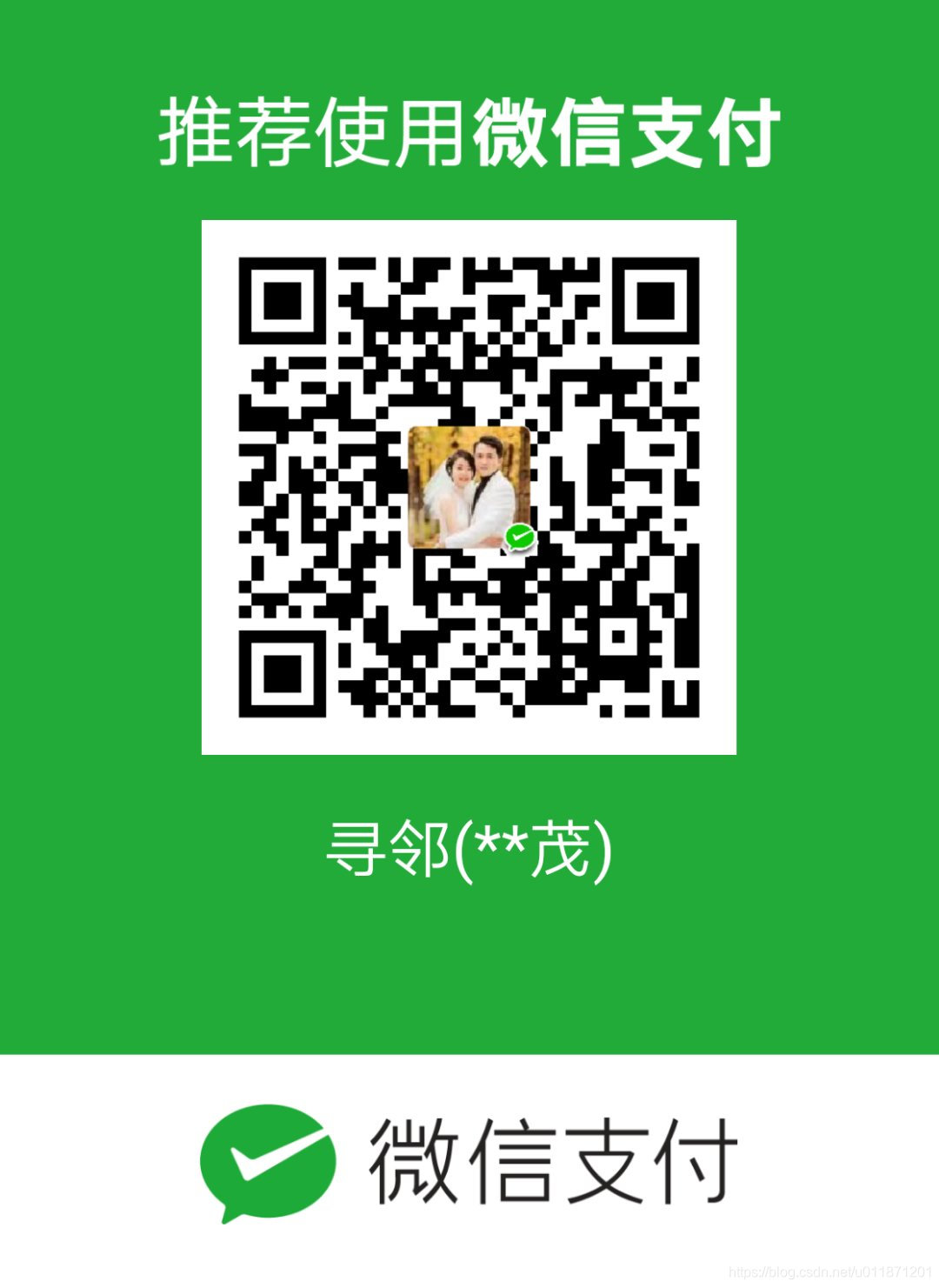
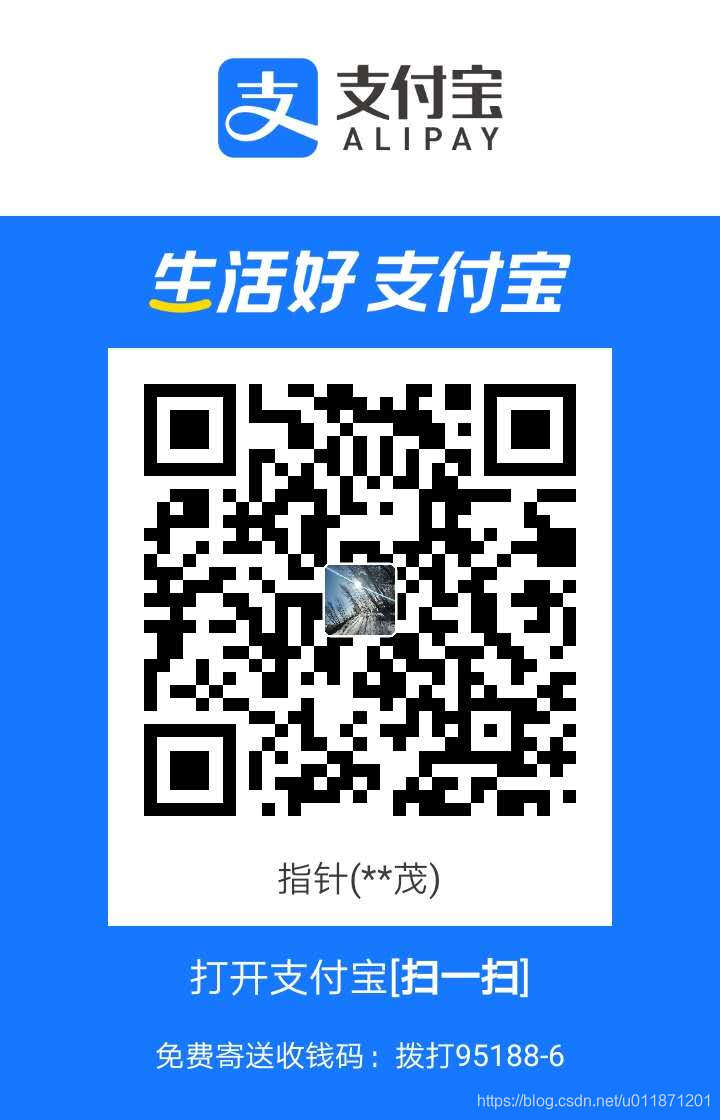