1,队列基本介绍
- 队列是一个有序列表,可以用数组或者链表来实现
- 队列遵循先入先出(FIFO)原则
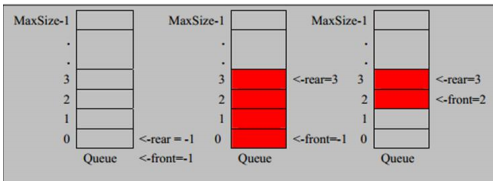
2,数组模拟队列
- 队列本身是有序列表,若使用数组的结构存储队列,则队列的声明如上图
- 因为队列的输入和输出是分别从两端处理,所以需要两个对应的索引数据
write
和read
,而write
和read
随着数据的输入和输出移动 - 在添加数据时,需要考虑的问题
- 数组是否已经初始化,如果没有初始化,初始化为默认长度,比如10
- 判断
写索引 + 写数据 > 读索引
,成立则数组越界,此处通过总数判断 - 写索引到索引末尾后,应该重置为0继续写入,如果此时读索引位置未变,即没有读事件,则数据越界
- 在取数据时,需要考虑的问题
- 写索引与读索引是否相等,相等则没有数据
- 读索引读取到末尾后,继续读0位置,实现循环处理
3,代码实现
package com.self.datastructure.queue;
import java.lang.annotation.Target;
import java.util.Scanner;
public class MyQueue {
private final int DEFAULT_COUNT = 8;
private int[] array;
private int capacity;
private int totalCount;
private int readIndex = 0;
private int writeIndex = 0;
public MyQueue(int count) {
initMyQueue(count);
}
public void initMyQueue(int count) {
count = count <= 0 ? DEFAULT_COUNT : count;
capacity = count;
array = new int[count];
}
public boolean isEmpty() {
return totalCount == 0;
}
public boolean isFull() {
return totalCount == capacity;
}
public boolean putData(int data) {
if (null == array) {
initMyQueue(DEFAULT_COUNT);
}
if (isFull()) {
throw new IndexOutOfBoundsException("数据满了...");
} else {
array[writeIndex++] = data;
totalCount++;
writeIndex = writeIndex % capacity;
}
return true;
}
public int readData() {
if (isEmpty()) {
throw new IndexOutOfBoundsException("没有数据...");
}
int data = array[readIndex++];
totalCount--;
readIndex = readIndex % capacity;
return data;
}
public void showDetails() {
System.out.print("totalCount: " + totalCount + "\t");
for (int i = readIndex; i < readIndex + getTotalCount(); i++) {
System.out.print(array[i % capacity] + ", ");
}
System.out.println();
}
public int getTotalCount() {
return totalCount;
}
}