1、
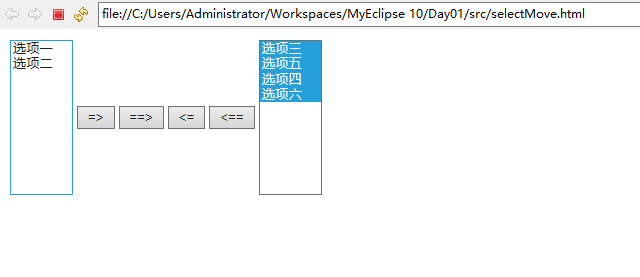
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> </head> <body> <table> <tr> <td><select name="select1" multiple="multiple" id="first" size="10"> <option>选项一</option> <option>选项二</option> <option>选项三</option> <option>选项四</option> <option>选项五</option> <option>选项六</option> </select></td> <td><input type="button" value="=>" id="moveRS"> <input type="button" value="==>" id="moveRA"> <input type="button" value="<=" id="moveLS"> <input type="button" value="<==" id="moveLA"></td> <td><select name="select2" multiple="multiple" id="second" size="10"> </select></td> </tr> </table> </body> <script type="text/javascript"> document.getElementById("moveRS").onclick = function() { var select1 = document.getElementById("first"); var select2 = document.getElementById("second"); var options = select1.options; for (var i = 0; i < options.length; i++) { if (options[i].selected) { select2.appendChild(options[i]); i--; } } } document.getElementById("moveRA").onclick = function() { var select1 = document.getElementById("first"); var select2 = document.getElementById("second"); var options = select1.options; for (var i = 0; i < options.length; i++) { select2.appendChild(options[i]); i--; } } document.getElementById("moveLS").onclick = function() { var select1 = document.getElementById("second"); var select2 = document.getElementById("first"); var options = select1.options; for (var i = 0; i < options.length; i++) { if (options[i].selected) { select2.appendChild(options[i]); i--; } } } document.getElementById("moveLA").onclick = function() { var select1 = document.getElementById("second"); var select2 = document.getElementById("first"); var options = select1.options; for (var i = 0; i < options.length; i++) { select2.appendChild(options[i]); i--; } } </script> </html>
2、计时器,开始和结束
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> <style type="text/css"> input { width: 400px; } </style> </head> <body> <input type="text" id="two"> <br /> <input type="button" value="开始" οnclick="setTime()"> <input type="button" value="结束" οnclick="endTime()"> <script type="text/javascript"> var id; function setTime() { if (!id) getTime(); } function getTime() { //获得input输入框 var two = document.getElementById("two"); //创建出当前时间的Date对象 var date = new Date(); //将时间添加到input中 two.value = date.toLocaleString(); //ID=调用自己的setTimeout(getTime,1000) id=setTimeout(getTime, 1000); } function endTime() { clearTimeout(id); id = undefined; } </script> </body> </html>
3、使用xml对dom对象进行操作
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> <style type="text/css"> #one { background-color: yellow; width: 300px; height: 200px; } #two { background-color: blue; width: 300px; height: 200px; } #three { background-color: green; width: 300px; height: 200px; } #four { background-color: purple; width: 300px; height: 200px; } </style> <script type="text/javascript"> //创建一个节点 function createNode() { var div_1 = document.getElementById("one"); var child = document.createElement("a"); child.setAttribute("href", "http://www.baidu.com"); child.innerHTML = "www.baidu.com"; div_1.appendChild(child); } //删除一个div function deleteNode() { var div_2 = document.getElementById("two"); var parent = div_2.parentNode; parent.removeChild(div_2); } //将div替换成图片 function updateNode() { var div_3 = document.getElementById("three"); var parent = div_3.parentNode; var img = document.createElement("img"); img.setAttribute("src", "2.jpg"); parent.replaceChild(img, div_3); } //复制div,并将复制后的div添加到末尾 function copyNode() { var div_4 = document.getElementById("four"); var cloneDiv = div_4.cloneNode(true); //true表示如果div中又子节点,那么连子节点也一起复制 //如果是false表示只复制div不复制子节点 var parent = div_4.parentNode; parent.appendChild(cloneDiv); } </script> </head> <body> <div id="one">div1</div> <br /> <div id="two">div2</div> <br /> <div id="three">div3</div> <br /> <div id="four">div4</div> <br /> <hr> <input type="button" value="增加div中的元素" οnclick="createNode()"> <input type="button" value="删除div" οnclick="deleteNode()"> <input type="button" value="修改div中的元素" οnclick="updateNode()"> <input type="button" value="复制div" οnclick="copyNode()"> </body> </html>
4、牛逼的表达验证,为什么JS这么这么牛逼呀!
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> <style type="text/css"> table, td { border: 1px solid blue; } </style> </head> <body> <form name="form1" οnsubmit="return check()"> <table> <tr> <th colspan="2">表单提交</th> </tr> <tr> <td>用户名</td> <td><input type="text" name="username"></td> </tr> <tr> <td>密码</td> <td><input type="password" name="password"></td> </tr> <tr> <td>年龄</td> <td><input type="text" name="age"></td> </tr> <tr> <td>性别</td> <td><input type="radio" value="1" name="sex">男 <input type="radio" value="0" name="sex">女</td> </tr> <tr> <td>爱好</td> <td><input type="checkbox" value="吃饭" name="habit">吃饭 <input type="checkbox" value="睡觉" name="habit">睡觉 <input type="checkbox" value="打豆豆" name="habit">打豆豆</td> </tr> <tr> <td>学历</td> <td><select name="edu"> <option>---请选择学历----</option> <option>小学</option> <option>初中</option> <option>高中</option> <option>大学</option> </select></td> </tr> <tr> <td colspan="2"><input type="submit" value="提交"></td> </tr> </table> </form> </body> <script type="text/javascript"> function check() { var a = true; a = (checkUserName() & checkEdu() & checkPassword() & checkAge()) return a ? true : false; } //验证用户名 function checkUserName() { var username = document.form1.username.value; var regex = /^[a-zA-Z][a-zA-Z_0-9]{2,9}$/; if (regex.test(username)) { removeError(document.form1.username); return true; } else { addError(document.form1.username, "用户名只能以字母开头"); return false; } } //验证密码 function checkPassword() { var password = document.form1.password.value; var regex = /^.{6,10}$/; if (regex.test(password)) { removeError(document.form1.password); return true; } else { addError(document.form1.password, "请输入6-10位的密码") return false; } } //验证年龄 function checkAge() { var age = document.form1.age.value; var regex = /^\d{1,3}$/; if (regex.test(age)) { removeError(document.form1.age); return true; } else { addError(document.form1.age, "年龄输入的不对"); return false; } } //验证学历 function checkEdu() { var edu = document.form1.edu; if (edu.selectedIndex) { removeError(edu); return true; } else { addError(edu, "请选择学历"); return false; } } //添加错误提示标记 function addError(where, what) { if (where.parentNode.getElementsByTagName("font")[0]) { return; } var font = document.createElement("font"); font.setAttribute("color", "red"); font.innerHTML = what; where.parentNode.appendChild(font); } //删除错误标记 function removeError(where) { var font = where.parentNode.getElementsByTagName("font")[0]; if (font) { font.parentNode.removeChild(font); } } </script> </html>
5、对表格中的元素进行排序
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> <style type="text/css"> table,tr,td { border: 1px solid blue; } </style> <script type="text/javascript"> function dataSort() { //1获得表格中所有的行 var table = document.getElementsByTagName("table")[0]; var rows = table.rows; //2创建一个新的数组,将需要排序的行装入 var arr = []; for ( var i = 1; i < rows.length; i++) { arr[i - 1] = rows[i]; } //3对新的数组排序 arr.sort(abc); //4排序好的数组遍历插回表格中 for ( var i = 0; i < arr.length; i++) { table.appendChild(arr[i]); } } function abc(a, b) { return +a.cells[1].innerHTML - b.cells[1].innerHTML; } </script> </head> <body> <table> <tr> <td>姓名</td> <td><a href="javascript:void(0)" οnclick="dataSort()">年龄</a></td> <td>电话</td> </tr> <tr> <td>王金鉴</td> <td>11</td> <td>18341893452</td> </tr> <tr> <td>冯旭</td> <td>12</td> <td>18341893453</td> </tr> <tr> <td>李俊峰</td> <td>15</td> <td>18341893373</td> </tr> <tr> <td>赵维真</td> <td>22</td> <td>18341893451</td> </tr> <tr> <td>孟凯妮</td> <td>20</td> <td>18341893476</td> </tr> <tr> <td>邓一鸣</td> <td>19</td> <td>18341893321</td> </tr> </table> </body> </html>
6、二级联动
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> </head> <body> <select id="province"> <option>----请选择省----</option> </select> <select id="city"> <option>----请选择市----</option> </select> </body> <script type="text/javascript"> var json = { "辽宁省" : [ "大连市", "阜新市", "沈阳市", "盘锦市", "营口市" ], "吉林省" : [ "长春市", "吉林市", "四平市" ] }; //遍历josn中的所有key,把key封装到option对象当中,把option对象添加到省的select中去 //1获得省的select对象 //2遍历json中的所有key //3key封装到option对象中 //添加 var province = document.getElementById("province"); var city = document.getElementById("city"); for ( var Key in json) { var option = document.createElement("option"); option.innerHTML = Key; province.appendChild(option); } //为province添加onchange事件 province.onchange = function() { //每次联动的时候需要清除city select中的数据 city.options.length = 1; //1.获得用户选择的省 var provinceKey = this.options[this.selectedIndex].innerHTML; //2.将选择的省去json中取得对应的市数组 var cities = json[provinceKey]; //3.遍历数组中的所有市,封装到option对象中 for (var i = 0; i < cities.length; i++) { var option = document.createElement("option"); //4、将option添加到city中 option.innerHTML = cities[i]; city.appendChild(option); } } </script> </html>
7、隔行变色
为什么我们这么牛逼什么玩意都会做,也是日了狗了
8、跨页面通讯,为什么我们这么牛逼,
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> </head> <frameset rows="30%,70%"> <frame src="top.html" /> <frameset cols="30%,*"> <frame src="left.html"/> <frame src="main.html"/> </frameset> </frameset> </html>
下边是top.html
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> </head> <body> <h1>标签页</h1> </body> </html>
下边是left.html
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> <script type="text/javascript"> function check() { var tr = document.createElement("tr"); var name = document.form1.name.value; var age = document.form1.age.value; var sex = document.form1.sex.value; tr.innerHTML = "<td>" + name + "</td><td>" + age + "</td><td>" + sex + "</td>" window.parent.frames[2].document.getElementsByTagName("table")[0] .appendChild(tr); } </script> </head> <body> <form name="form1" οnsubmit="check()"> 姓名:<input type="text" name="name"><br /> 年龄:<input type="text" name="age"><br /> 性别:<input type="text" name="sex"><input type="button" value="选择" onclick="window.open('select.html')"> <input type="submit" value="提交"> </form> </body> </html>
下边是main.html
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> </head> <body> <table> <tr> <td>姓名</td> <td><a href="javascript:void(0)" οnclick="dataSort()">年龄</a></td> <td>性别</td> </tr> <tr> <td>王金鉴</td> <td>11</td> <td>男</td> </tr> <tr> <td>冯旭</td> <td>12</td> <td>男</td> </tr> <tr> <td>李俊峰</td> <td>15</td> <td>男</td> </tr> <tr> <td>赵维真</td> <td>22</td> <td>男</td> </tr> <tr> <td>孟凯妮</td> <td>20</td> <td>女</td> </tr> <tr> <td>邓一鸣</td> <td>19</td> <td>男</td> </tr> </table> </body> </html>
9、流氓广告,鼠标走到哪里,广告就跟到哪里
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> <style type="text/css"> div { border: 1px solid red; } #one { width: 400px; height: 400px; } #two { background-color: pink; width: 50px; height: 50px; } </style> </head> <body> <div id="one"> <a href="隔行变色.html">这里有你想看的内容</a> <a href="selectMove.html">体育老师视频</a> </div> <div id="two"> <a href="formAdd.html" target="_blank" οnclick="remove(this)">流氓广告</a> </div> </body> <script type="text/javascript"> document.getElementById("one").onmousemove = function(event) { var x = event.clientX; var y = event.clientY; var div2 = document.getElementById("two"); div2.style.position = "absolute"; div2.style.top = (y - 25) + "px"; div2.style.left = (x - 25) + "px"; } function remove(a){ a.parentNode.style.display="none"; } </script> </html>