代码提交规范-ESLint+Prettier+husky+Commitlint
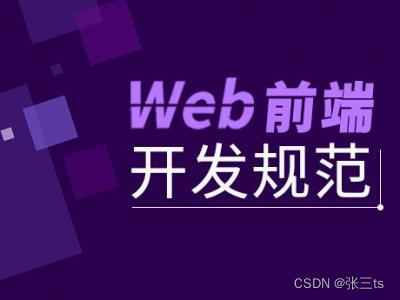
先看下思维导图分析
配置eslint (3步)
- 装包配置.eslintrc.js
- 配置忽略文件
- 运行
详细讲解
以react 项目为例
// 1.装包
yarn add eslint -D
// 2.生成配置文件(按指示一路回车即可)
// 相关选项可看下方结果
npx eslint --init
// 3.配置.eslintrc.js, 直接用下方的eslintrc替换自动生成的即可,可避免很多坑
// 4.使用eslint命令
// 在package的script中添加,fix表示可自动修复简单的问题。
"scripts": {
"lint": "eslint --fix src/**/*.{js,jsx,ts,tsx}",
}
// 运行 yarn lint 即可检查代码质量
- 配置文件 eslintrc文件
根目录/.eslintrc.js
module.exports = {
"env": {
"browser": true,
"es2021": true,
"node": true, // 解决‘module‘ is not defined报错。
},
"extends": [
"eslint:recommended",
"plugin:@typescript-eslint/recommended",
"plugin:react/recommended"
],
"overrides": [
{
"env": {
"node": true
},
"files": [
".eslintrc.{js,cjs}"
],
"parserOptions": {
"sourceType": "script"
}
}
],
"parser": "@typescript-eslint/parser",
"parserOptions": {
"ecmaVersion": "latest",
"sourceType": "module"
},
"plugins": [
"@typescript-eslint",
"react"
],
"globals": {
"Atomics": "readonly",
"SharedArrayBuffer": "readonly",
"process": true, // 排除对process的报错
},
rules: {
semi: ['error', 'always'], // 该规则强制使用一致的分号
"react/prop-types": "off", // 处理解构的报错
'no-unused-vars': 'off', // 禁止未使用过的变量
'@typescript-eslint/no-unused-vars': 'off',
'no-debugger': process.env.NODE_ENV === 'production' ? 'error' : 'off', //生产环境禁用 debugger
'no-console': process.env.NODE_ENV === 'production' ? 'error' : 'off', //生产环境禁用 console
'default-case': ['warn', { commentPattern: '^no default$' }], //要求 Switch 语句中有 Default
'dot-location': ['warn', 'property'], // 强制在点号之前或之后换行
eqeqeq: ['error', 'allow-null'], //要求使用 === 和 !==
'new-parens': 'warn', //要求调用无参构造函数时带括号
'no-caller': 'error', // 禁用 caller 或 callee
'no-const-assign': 'error', //不允许改变用 const 声明的变量
'no-dupe-args': 'error', //禁止在 function 定义中出现重复的参数
'no-dupe-class-members': 'error', //不允许类成员中有重复的名称
'no-dupe-keys': 'warn', //禁止在对象字面量中出现重复的键
'no-extend-native': 'warn', //禁止扩展原生对象
'no-extra-bind': 'warn', //禁止不必要的函数绑定
'no-fallthrough': 'error', //禁止 case 语句落空
'no-func-assign': 'warn', //禁止对 function 声明重新赋值
'no-implied-eval': 'error', //禁用隐式的 eval()
'no-label-var': 'error', //禁用与变量同名的标签
'no-loop-func': 'error', //禁止循环中存在函数
'no-mixed-operators': [
'warn',
{
groups: [
['&', '|', '^', '~', '<<', '>>', '>>>'],
['==', '!=', '===', '!==', '>', '>=', '<', '<='],
['&&', '||'],
['in', 'instanceof'],
],
allowSamePrecedence: false,
},
], //禁止混合使用不同的操作符
'no-multi-str': 'warn', //禁止多行字符串 (需要多行时用\n)
'no-native-reassign': 'warn', //禁止重新分配本地对象
'no-obj-calls': 'warn', //禁止将全局对象当作函数进行调用
'no-redeclare': 'error', //禁止重新声明变量
'no-script-url': 'warn', //禁用 Script URL
'no-shadow-restricted-names': 'warn', //关键字不能被遮蔽
'no-sparse-arrays': 'warn', //禁用稀疏数组
'no-this-before-super': 'warn', //在构造函数中禁止在调用 super()之前使用 this 或 super
'no-undef': 'error', //禁用未声明的变量
'no-unexpected-multiline': 'warn', //禁止使用令人困惑的多行表达式
'no-use-before-define': [
'off',
{
functions: false,
classes: false,
variables: false,
},
], //禁止定义前使用
'no-with': 'error', //禁用 with 语句
radix: 'error', //禁用函数内没有 yield 的 generator 函数
'rest-spread-spacing': ['warn', 'never'], //强制限制扩展运算符及其表达式之间的空格
'react/jsx-no-undef': 'error', //在 JSX 中禁止未声明的变量
'react/no-direct-mutation-state': 'error', //禁止 this.state 的直接变化
'react/jsx-uses-react': 'off', //防止 React 被错误地标记为未使用
'react/react-in-jsx-scope': 'off',
'no-alert': 0, //禁止使用alert confirm prompt
'no-duplicate-case': 2, //switch中的case标签不能重复
'no-eq-null': 2, //禁止对null使用==或!=运算符
'no-inner-declarations': [2, 'functions'], //禁止在块语句中使用声明(变量或函数)
'no-iterator': 2, //禁止使用__iterator__ 属性
'no-negated-in-lhs': 2, //in 操作符的左边不能有!
'no-octal-escape': 2, //禁止使用八进制转义序列
'no-plusplus': 0, //禁止使用++,--
'no-self-compare': 2, //不能比较自身
'no-undef-init': 2, //变量初始化时不能直接给它赋值为undefined
'no-unused-expressions': 'off', //禁止无用的表达式
'no-useless-call': 2, //禁止不必要的call和apply
'init-declarations': 0, //声明时必须赋初值
'prefer-const': 0, //首选const
'use-isnan': 2, //禁止比较时使用NaN,只能用isNaN()
'vars-on-top': 2, //var必须放在作用域顶部
"@typescript-eslint/no-explicit-any": ["off"]
},
// 处理react版本的报错React version not specified in eslint-plugin-react settings
"settings": {
react: {
version: 'detect',
},
},
}
设置忽略文件 .eslintignore
dist/*
node_modules/*
*.json
配置prettier(4步)
主要步骤
- 配置perttier
- 配置忽略文件
- 处理eslint的冲突
- 配置vscode的setting.json
1.安装配置prettier
yarn add prettier -D
配置.prettierrc.js文件
module.exports = {
printWidth: 100,
semi: true,
singleQuote: true,
tabWidth: 2,
};
判断是否生效直接使用命令 npx prettier --write [指定文件] ,查看文件是否根据 prettier 的规则格式化。
2.设置忽略文件 .prettierignore
node_modules/**
dist/**
public/**
doc/**
3.处理eslint冲突
安装eslint-config-prettier 解决prettier和eslint之间的冲突
yarn add eslint-config-prettier -D
4. 配置vscode 的settings.json
{
// eslint主要功能设定规范,捕获不规范的错误等 美化代码或者格式化代码功能交给prettier
"eslint.format.enable": false,
//打开保存格式化功能(保存代码的时候自动格式化)
"editor.formatOnSave": true,
//使用 prettier 作为默认格式化程序
"editor.defaultFormatter": "esbenp.prettier-vscode"
}
husky
参考:https://juejin.cn/post/7282744150843047991
简要步骤:
- 安装并初始化husky
- 安装并配置lint-staged
- 安装配置commitlint
安装并初始化husky(2步)
使用husky-init用于快速初始化husky项目。
yarn add husky -D
// 初始化husky。
// 1将prepare脚本添加到package 2、根目录创建.husky文件夹,包含pre-commit钩子
npx husky-init
知识点: prepare 脚本会在npm install(不带参数)之后自动执行
安装并配置lint-staged(3步)
作用:只处理git add .的文件
- 安装
yarn add lint-staged -D
- 配置package.json
{
"scripts": ....
// 设置lint-staged;提交时prettier代码格式化,eslint检查修复
"lint-staged": {
"src/**/*.{js,jsx,ts,tsx,json}": [
"prettier --write",
"eslint --fix"
]
},
}
- 修改.husky/pre-commit文件,使提交时能执行lint-staged钩子
#!/usr/bin/env sh
. "$(dirname -- "$0")/_/husky.sh"
pnpm exec lint-staged
// 删除 npm test
安装配置commitlint(4步)
-
安装包
// 校验提交注释是否规范(格式git commit : )
// 2.配置文件 .commitlintrc.js
yarn add @commitlint/cli @commitlint/config-conventional -D -
在项目根目录下添加commitlint.config.js配置文件
module.exports = {
extends: ['@commitlint/config-conventional'],
rules: {
// type 类型定义
'type-enum': [
2,
'always',
[
'feat', // 新功能 feature
'fix', // 修复 bug
'docs', // 文档注释
'style', // 代码格式(不影响代码运行的变动)
'refactor', // 重构(既不增加新功能,也不是修复bug)
'perf', // 性能优化
'test', // 增加测试
'chore', // 构建过程或辅助工具的变动
'revert', // 回退
'build', // 打包
],
],
// subject 大小写不做校验
// 自动部署的BUILD ROBOT的commit信息大写,以作区别
'subject-case': [0],
},
};
- 执行以下命令添加commitlint钩子
npx husky add .husky/commit-msg "npm run commitlint"
- 在package.json script中增加commitlint
"scripts": {
"commitlint": "commitlint --config commitlint.config.js -e -V"
},
大功告成