Radar Installation
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 55857 | Accepted: 12596 |
Description
Assume the coasting is an infinite straight line. Land is in one side of coasting, sea in the other. Each small island is a point locating in the sea side. And any radar installation, locating on the coasting, can only cover d distance, so an island in the sea can be covered by a radius installation, if the distance between them is at most d.
We use Cartesian coordinate system, defining the coasting is the x-axis. The sea side is above x-axis, and the land side below. Given the position of each island in the sea, and given the distance of the coverage of the radar installation, your task is to write a program to find the minimal number of radar installations to cover all the islands. Note that the position of an island is represented by its x-y coordinates.
Figure A Sample Input of Radar Installations
We use Cartesian coordinate system, defining the coasting is the x-axis. The sea side is above x-axis, and the land side below. Given the position of each island in the sea, and given the distance of the coverage of the radar installation, your task is to write a program to find the minimal number of radar installations to cover all the islands. Note that the position of an island is represented by its x-y coordinates.
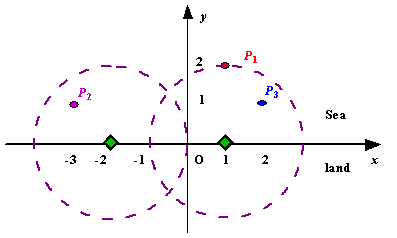
Figure A Sample Input of Radar Installations
Input
The input consists of several test cases. The first line of each case contains two integers n (1<=n<=1000) and d, where n is the number of islands in the sea and d is the distance of coverage of the radar installation. This is followed by n lines each containing two integers representing the coordinate of the position of each island. Then a blank line follows to separate the cases.
The input is terminated by a line containing pair of zeros
The input is terminated by a line containing pair of zeros
Output
For each test case output one line consisting of the test case number followed by the minimal number of radar installations needed. "-1" installation means no solution for that case.
Sample Input
3 2 1 2 -3 1 2 1 1 2 0 2 0 0
Sample Output
Case 1: 2 Case 2: 1
Source
题目大意:在直角坐标系中,x轴为海岸,x轴上方为海(岛屿在x轴上方),下方为陆地,告诉你岛屿的坐标,以及雷达的探测半径d,求最小数量的雷达,将岛屿覆盖,如果不能则输出-1;
显然在d<y(岛屿的纵坐标)时,不可能有雷达覆盖他,这是输出-1;
此题采用贪心策略: 以岛屿i坐标(p[i].x,p[i].y)为圆心,做半径为d的圆,交x轴两个交点,p[i].l记为左交点,p[i].r记为右交点,将岛屿按照p[i].l排序,按照排序后从左到右依次选择圆心所在位置(圆心可以不为整数),使得该圆能够覆盖足够多的点,首先选择第一个点的p[i].r作为圆心记为cr,然后判断,当(p[i].l>cr)时,显然,当前的圆覆盖不了此点,只能新建一个,ans++,否则当p[i].r<cr时,说明还有更优的选择,将当前cr更新为p[i].r;
此外此题还应注意一点:用qsort排序时,下面这样会导致精度丢失,导致WA
int cmp(const void *a,const void *b)
{
Point *p1=(Point *)a,*p2=(Point *)b;
if(p1->l!=p2->l)
return p1->l-p2->l;
return p1->r-p2->r;
}
应该这样写:
int cmp(const void *a,const void *b)
{
Point *p1=(Point *)a,*p2=(Point *)b;
if(p1->l>p2->l)
return 1;
if(p1->l<p2->l)
return -1;
if(p1->r>p2->r)
return 1;
if(p1->r<p2->r)
return -1;
return 0;
}
代码:
#include <iostream>
#include<stdio.h>
#include<math.h>
#include<string.h>
#include<stdlib.h>
#define N 1010
#define getx2(x) (x)*(x)
#define eps 1e-10
/* run this program using the console pauser or add your own getch, system("pause") or input loop */
using namespace std;
struct Point
{
double l,r;
int x,y;
}p[N];
void init(int n)
{
for(int i=0;i<=n;i++)
{
p[i].l=p[i].r=p[i].x=p[i].y=0;
}
}
int cmp(const void *a,const void *b)
{
Point *p1=(Point *)a,*p2=(Point *)b;
if(p1->l>p2->l)
return 1;
if(p1->l<p2->l)
return -1;
if(p1->r>p2->r)
return 1;
if(p1->r<p2->r)
return -1;
return 0;
}
int main(int argc, char** argv) {
int n,d,cnt=0,i;
bool flag;
while(~scanf("%d%d",&n,&d)&&(n||d))
{
flag=true;
init(n);
for(i=0;i<n;i++)
{
scanf("%d%d",&p[i].x,&p[i].y);
if(p[i].y>d)
{
flag=false;
continue;
}
p[i].l=p[i].x-sqrt(getx2(d)-getx2(p[i].y));
p[i].r=p[i].x+sqrt(getx2(d)-getx2(p[i].y));
}
if(!flag)
{
printf("Case %d: -1\n",++cnt);
continue;
}
qsort(p,n,sizeof(p[0]),cmp);
int ans=1;
double cr=p[0].r;
for(i=1;i<n;i++)
{
if(p[i].l>cr)
{
ans++,cr=p[i].r;
}
else if(p[i].r<cr)
cr=p[i].r;
}
printf("Case %d: %d\n",++cnt,ans);
}
return 0;
}