双向数据绑定 v-model
需求1. 使input框的初始化值是value的值
需求2. 使input框输入的实时在p标签上输出
思想:
使用input.value控制input框的值
使用input事件,每次输入触发该事件,使用事件对象e.target.value传入输入的值
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<title>双向数据绑定</title>
</head>
<body>
<div id="app">
<input type="text" v-bind:value = "value" v-on:input = "input">
<p>{{ value }}</p>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
new Vue({
el : "#app",
data : {
value : "fanghuayong"
},
methods : {
input : function(e) {
this.value = e.target.value
console.log(this.value)
}
}
})
</script>
</body>
</html>
问题: 单向数据流
数据可以改变属性,但属性无法改变数据
v-bind的只是初始化了一下数据,使得数据绑定到了value属性上,但是没有v-on:input事件的话,在input框中输入的value值无法改变数据,所有需要借助v-on:input事件。
双向数据流: vue中提供一下更快捷的方法,v-model
<div id="app">
<input type="text" v-model="value">
<p>{{ value }}</p>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
new Vue({
el : "#app",
data : {
value : "fanghuayong"
},
})
</script>
v-model可以初始化value的属性, v-model改变value的值,就会改变数据的值,数据的值又可以改变value属性的值,成为双向数据绑定。
计算属性: computed字段
<div id="app">
<button v-on:click = "increase" style="background-color: green; color: #fff;">按钮+1</button>
<button v-on:click = "de" style="background-color: green; color: #fff;">按钮-1</button>
<p>{{ count }}{{ result() }}</p>
<button v-on:click = "count2++" style="background-color: yellow">按钮二+1</button>
<p>{{ count2 }}</p>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
new Vue({
el : "#app",
data : {
count : 0,
count2 : 0
},
methods : {
result : function() {
console.log("result")
return this.count > 5 ? ">5" : "<5"
},
increase : function() {
this.count++
// this.result = this.count > 5 ? ">5" : "<5"
},
de : function() {
this.count--
// this.result = this.count > 5 ? ">5" : "<5"
}
}
})
</script>
result函数是为了代码复用,因为按钮+1或者按钮-1都要使用到里面的函数,所以直接执行result()函数。
但是发现按钮2+1点击也会执行该函数,因为它不知道是谁需要它,只要有页面变动,为了最新的数据,它就会执行。
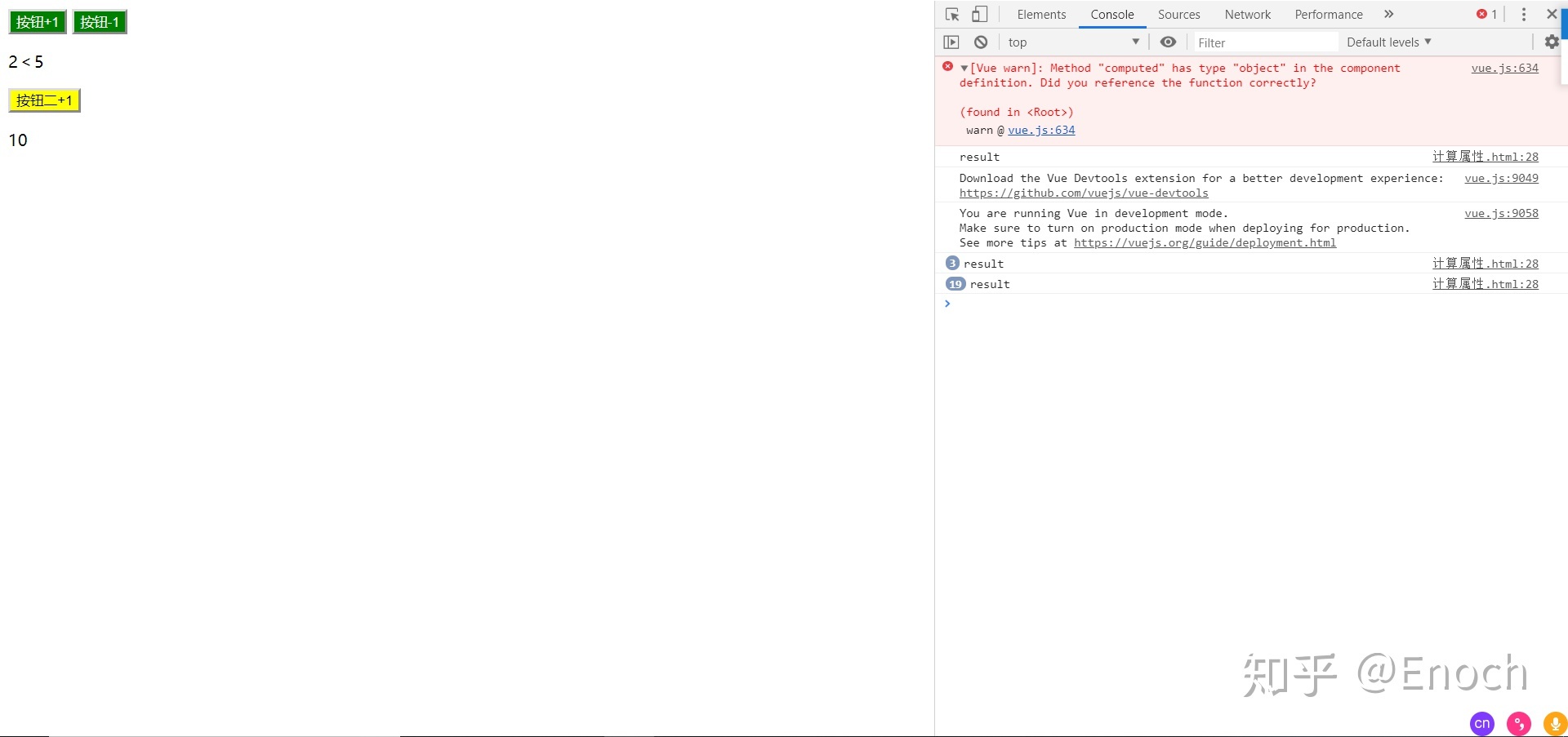
计算属性: 计算属性和函数属性有两点不同之处,
- 写法不同。函数属性: {{ result() }}是执行模式带括号,计算属性{{ output }}不需要带括号;
- 刷新作用域不同。函数属性只要页面dom节点有改动就刷新最新数据,适合凡事dom界面发生改变就会执行。计算属性会自动识别与它有关的,它才会刷新。
<div id="app">
<button v-on:click = "increase" style="background-color: green; color: #fff;">按钮+1</button>
<button v-on:click = "de" style="background-color: green; color: #fff;">按钮-1</button>
<p>{{ count }}{{ result() }}</p>
<button v-on:click = "count2++" style="background-color: yellow">按钮二+1</button>
<p>{{ count2 }}</p>
<p>函数属性:{{ result() }} | 计算属性:{{ output }}</p>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
new Vue({
el : "#app",
data : {
count : 0,
// result : "",
count2 : 0
},
computed : {
output : function() {
console.log("output")
return this.count > 5 ? ">5" : "<5"
}
},
methods : {
result : function() {
console.log("result")
return this.count > 5 ? ">5" : "<5"
},
increase : function() {
this.count++
// this.result = this.count > 5 ? ">5" : "<5"
},
de : function() {
this.count--
// this.result = this.count > 5 ? ">5" : "<5"
}
}
})
</script>
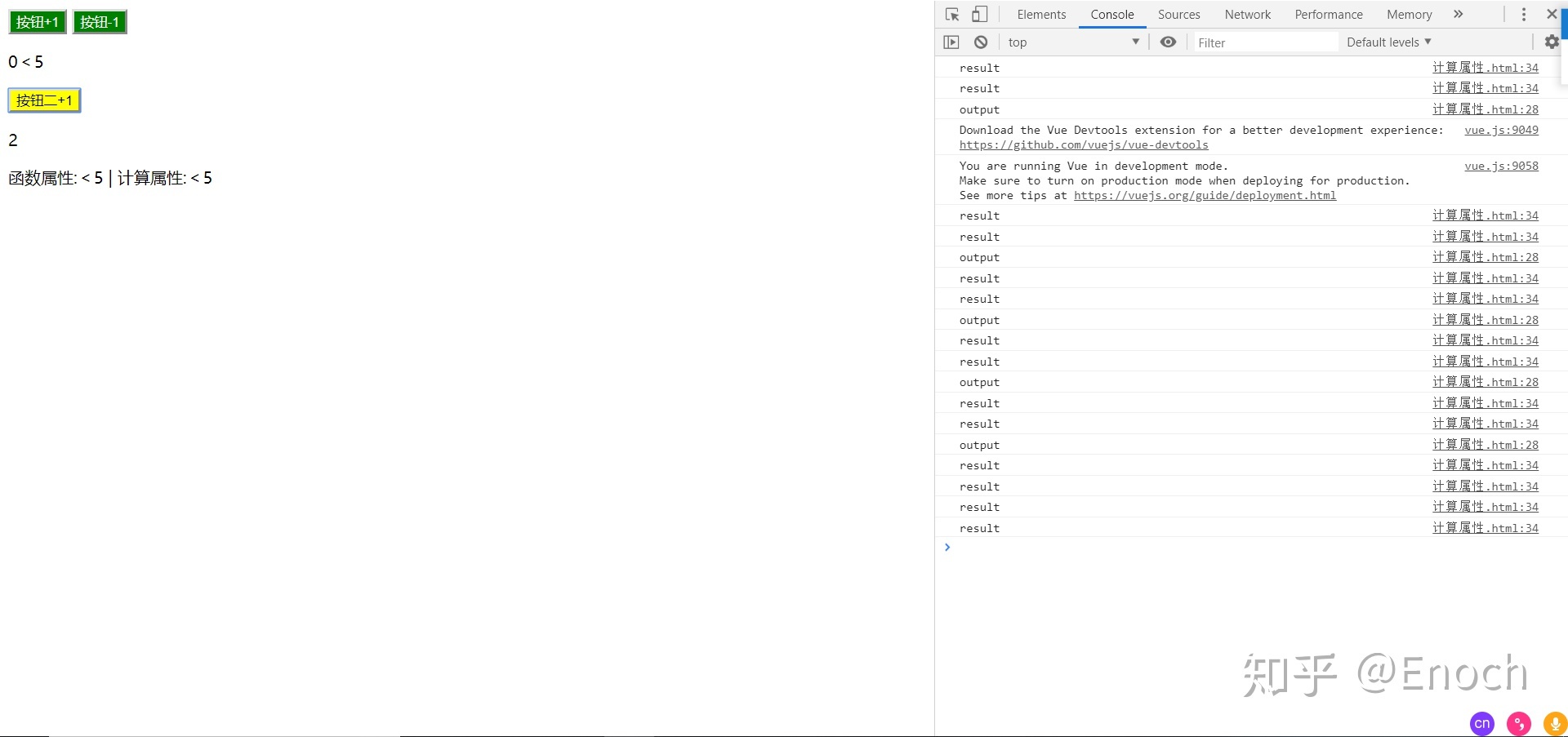
监听 watch字段
监听data中的变量,被监听的变量数据改变会触发该事件,并且会自动传入函数中。
new Vue({
el : "#app",
data : {
count : 0,
count2 : 0
},
watch : {
count : function(val) {
console.log(val)
}
},
computed : {
output : function() {
console.log("output")
return this.count > 5 ? ">5" : "<5"
}
},
methods : {
result : function() {
console.log("result")
return this.count > 5 ? ">5" : "<5"
},
increase : function() {
this.count++
// this.result = this.count > 5 ? ">5" : "<5"
},
de : function() {
this.count--
// this.result = this.count > 5 ? ">5" : "<5"
}
}
})
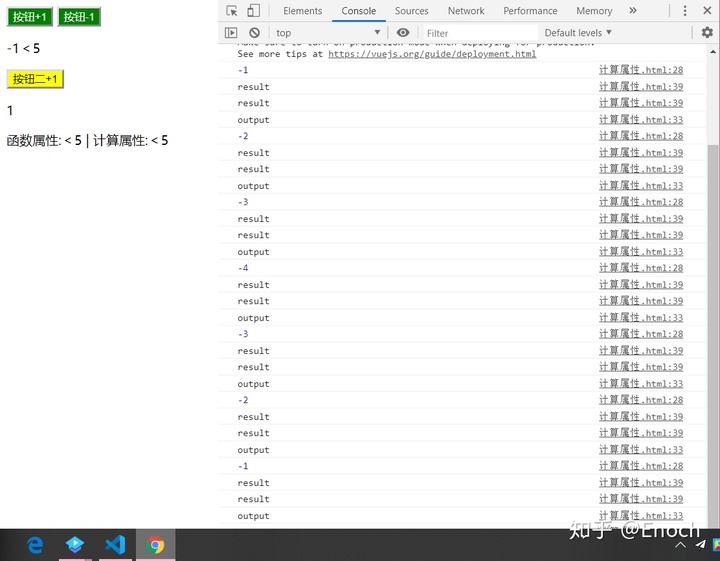
计算属性与watch属性(执行也是不需要括号())的区别:
- 计算属性需要返回一个值,watch属性不需要返回一个值,可以直接写逻辑操作
- 计算属性是同步代码,watch属性可以写异步代码(虽然我的异步写的不好,没有严格写,只是测试了一下)
// html代码
<p>函数属性:{{ result() }} | 计算属性:{{ output }}|watch属性: {{ output2 }}</p>
// js代码
data : {
count : 0,
count2 : 0,
output2 : ""
},
watch : {
count2 : function(val) {
console.log(val)
this.output2 = val > 5 ? "大于5" : "小于5";
var vm = this
// 设置2s后做什么
window.setTimeout(function(){
vm.count2 = 0;
console.log('被执行!')
},2000)
}
},
简写:
v-on:click == @click
v-bind:title == :title