带有增加与减少按钮的数量选择控件
用法:
<me.himanshusoni.quantityview.QuantityView
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/quantityView_default"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
app:qv_quantity="10" />
自定义
属性:
qv_dialog_changequantity.xml
attr.xml
string.xml
drawable:
qv_bg_selector.xml
qv_btn_selector.xml
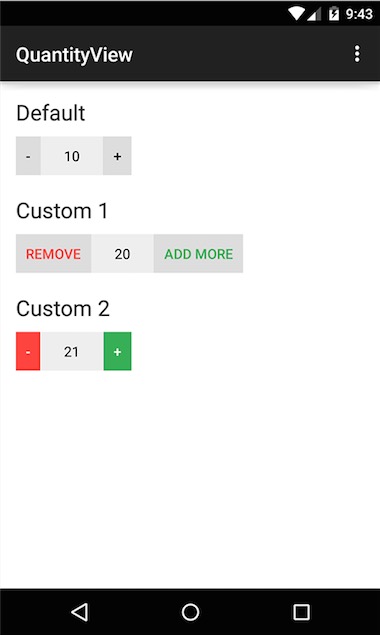
用法:
<me.himanshusoni.quantityview.QuantityView
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/quantityView_default"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
app:qv_quantity="10" />
自定义
属性:
- app:qv_addButtonBackground="color|drawable"
- app:qv_addButtonText="string"
- app:qv_addButtonTextColor="color"
- app:qv_removeButtonBackground="color|drawable"
- app:qv_removeButtonText="string"
- app:qv_removeButtonTextColor="color"
- app:qv_quantityBackground="color|drawable"
- app:qv_quantityTextColor="color"
- app:qv_quantity="integer"
- app:qv_quantityPadding="dimension"
- app:qv_maxQuantity="integer"
- app:qv_minQuantity="integer"
- import android.annotation.TargetApi;
- import android.content.Context;
- import android.content.DialogInterface;
- import android.content.res.TypedArray;
- import android.graphics.Color;
- import android.graphics.drawable.Drawable;
- import android.os.Build;
- import android.support.v4.content.ContextCompat;
- import android.support.v7.app.AlertDialog;
- import android.util.AttributeSet;
- import android.view.Gravity;
- import android.view.LayoutInflater;
- import android.view.View;
- import android.widget.Button;
- import android.widget.EditText;
- import android.widget.LinearLayout;
- import android.widget.TextView;
- /**
- * Quantity view to add and remove quantities
- */
- public class QuantityView extends LinearLayout implements View.OnClickListener {
- private Drawable quantityBackground, addButtonBackground, removeButtonBackground;
- private String addButtonText, removeButtonText;
- private int quantity, maxQuantity, minQuantity;
- private int quantityPadding;
- private int quantityTextColor, addButtonTextColor, removeButtonTextColor;
- private Button mButtonAdd, mButtonRemove;
- private TextView mTextViewQuantity;
- public interface OnQuantityChangeListener {
- void onQuantityChanged(int newQuantity, boolean programmatically);
- void onLimitReached();
- }
- private OnQuantityChangeListener onQuantityChangeListener;
- public QuantityView(Context context) {
- super(context);
- init(null, 0);
- }
- public QuantityView(Context context, AttributeSet attrs) {
- super(context, attrs);
- init(attrs, 0);
- }
- public QuantityView(Context context, AttributeSet attrs, int defStyle) {
- super(context, attrs, defStyle);
- init(attrs, defStyle);
- }
- @TargetApi(Build.VERSION_CODES.JELLY_BEAN)
- private void init(AttributeSet attrs, int defStyle) {
- final TypedArray a = getContext().obtainStyledAttributes(attrs, R.styleable.QuantityView, defStyle, 0);
- addButtonText = getResources().getString(R.string.qv_add);
- if (a.hasValue(R.styleable.QuantityView_qv_addButtonText)) {
- addButtonText = a.getString(R.styleable.QuantityView_qv_addButtonText);
- }
- addButtonBackground = ContextCompat.getDrawable(getContext(), R.drawable.qv_btn_selector);
- if (a.hasValue(R.styleable.QuantityView_qv_addButtonBackground)) {
- addButtonBackground = a.getDrawable(R.styleable.QuantityView_qv_addButtonBackground);
- }
- addButtonTextColor = a.getColor(R.styleable.QuantityView_qv_addButtonTextColor, Color.BLACK);
- removeButtonText = getResources().getString(R.string.qv_remove);
- if (a.hasValue(R.styleable.QuantityView_qv_removeButtonText)) {
- removeButtonText = a.getString(R.styleable.QuantityView_qv_removeButtonText);
- }
- removeButtonBackground = ContextCompat.getDrawable(getContext(), R.drawable.qv_btn_selector);
- if (a.hasValue(R.styleable.QuantityView_qv_removeButtonBackground)) {
- removeButtonBackground = a.getDrawable(R.styleable.QuantityView_qv_removeButtonBackground);
- }
- removeButtonTextColor = a.getColor(R.styleable.QuantityView_qv_removeButtonTextColor, Color.BLACK);
- quantity = a.getInt(R.styleable.QuantityView_qv_quantity, 0);
- maxQuantity = a.getInt(R.styleable.QuantityView_qv_maxQuantity, Integer.MAX_VALUE);
- minQuantity = a.getInt(R.styleable.QuantityView_qv_minQuantity, 0);
- quantityPadding = (int) a.getDimension(R.styleable.QuantityView_qv_quantityPadding, pxFromDp(24));
- quantityTextColor = a.getColor(R.styleable.QuantityView_qv_quantityTextColor, Color.BLACK);
- quantityBackground = ContextCompat.getDrawable(getContext(), R.drawable.qv_bg_selector);
- if (a.hasValue(R.styleable.QuantityView_qv_quantityBackground)) {
- quantityBackground = a.getDrawable(R.styleable.QuantityView_qv_quantityBackground);
- }
- a.recycle();
- int dp10 = pxFromDp(10);
- mButtonAdd = new Button(getContext());
- mButtonAdd.setGravity(Gravity.CENTER);
- mButtonAdd.setPadding(dp10, dp10, dp10, dp10);
- mButtonAdd.setMinimumHeight(0);
- mButtonAdd.setMinimumWidth(0);
- mButtonAdd.setMinHeight(0);
- mButtonAdd.setMinWidth(0);
- setAddButtonBackground(addButtonBackground);
- setAddButtonText(addButtonText);
- setAddButtonTextColor(addButtonTextColor);
- mButtonRemove = new Button(getContext());
- mButtonRemove.setGravity(Gravity.CENTER);
- mButtonRemove.setPadding(dp10, dp10, dp10, dp10);
- mButtonRemove.setMinimumHeight(0);
- mButtonRemove.setMinimumWidth(0);
- mButtonRemove.setMinHeight(0);
- mButtonRemove.setMinWidth(0);
- setRemoveButtonBackground(removeButtonBackground);
- setRemoveButtonText(removeButtonText);
- setRemoveButtonTextColor(removeButtonTextColor);
- mTextViewQuantity = new TextView(getContext());
- mTextViewQuantity.setGravity(Gravity.CENTER);
- setQuantityTextColor(quantityTextColor);
- setQuantity(quantity);
- setQuantityBackground(quantityBackground);
- setQuantityPadding(quantityPadding);
- setOrientation(HORIZONTAL);
- addView(mButtonRemove, LayoutParams.WRAP_CONTENT, LayoutParams.WRAP_CONTENT);
- addView(mTextViewQuantity, LayoutParams.WRAP_CONTENT, LayoutParams.MATCH_PARENT);
- addView(mButtonAdd, LayoutParams.WRAP_CONTENT, LayoutParams.WRAP_CONTENT);
- mButtonAdd.setOnClickListener(this);
- mButtonRemove.setOnClickListener(this);
- mTextViewQuantity.setOnClickListener(this);
- }
- @Override
- public void onClick(View v) {
- if (v == mButtonAdd) {
- if (quantity + 1 > maxQuantity) {
- if (onQuantityChangeListener != null) onQuantityChangeListener.onLimitReached();
- } else {
- quantity += 1;
- mTextViewQuantity.setText(String.valueOf(quantity));
- if (onQuantityChangeListener != null)
- onQuantityChangeListener.onQuantityChanged(quantity, false);
- }
- } else if (v == mButtonRemove) {
- if (quantity - 1 < minQuantity) {
- if (onQuantityChangeListener != null) onQuantityChangeListener.onLimitReached();
- } else {
- quantity -= 1;
- mTextViewQuantity.setText(String.valueOf(quantity));
- if (onQuantityChangeListener != null)
- onQuantityChangeListener.onQuantityChanged(quantity, false);
- }
- } else if (v == mTextViewQuantity) {
- AlertDialog.Builder builder = new AlertDialog.Builder(getContext());
- builder.setTitle("Change Quantity");
- View inflate = LayoutInflater.from(getContext()).inflate(R.layout.qv_dialog_changequantity, null, false);
- final EditText et = (EditText) inflate.findViewById(R.id.qv_et_change_qty);
- et.setText(String.valueOf(quantity));
- builder.setView(inflate);
- builder.setPositiveButton("Change", new DialogInterface.OnClickListener() {
- @Override
- public void onClick(DialogInterface dialog, int which) {
- String newQuantity = et.getText().toString();
- if (isNumber(newQuantity)) {
- int intNewQuantity = Integer.parseInt(newQuantity);
- setQuantity(intNewQuantity);
- }
- }
- }).setNegativeButton("Cancel", null);
- builder.show();
- }
- }
- public OnQuantityChangeListener getOnQuantityChangeListener() {
- return onQuantityChangeListener;
- }
- public void setOnQuantityChangeListener(OnQuantityChangeListener onQuantityChangeListener) {
- this.onQuantityChangeListener = onQuantityChangeListener;
- }
- public Drawable getQuantityBackground() {
- return quantityBackground;
- }
- public void setQuantityBackground(Drawable quantityBackground) {
- this.quantityBackground = quantityBackground;
- if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN) {
- mTextViewQuantity.setBackground(quantityBackground);
- } else {
- mTextViewQuantity.setBackgroundDrawable(quantityBackground);
- }
- }
- public Drawable getAddButtonBackground() {
- return addButtonBackground;
- }
- public void setAddButtonBackground(Drawable addButtonBackground) {
- this.addButtonBackground = addButtonBackground;
- if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN) {
- mButtonAdd.setBackground(addButtonBackground);
- } else {
- mButtonAdd.setBackgroundDrawable(addButtonBackground);
- }
- }
- public Drawable getRemoveButtonBackground() {
- return removeButtonBackground;
- }
- public void setRemoveButtonBackground(Drawable removeButtonBackground) {
- this.removeButtonBackground = removeButtonBackground;
- if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN) {
- mButtonRemove.setBackground(removeButtonBackground);
- } else {
- mButtonRemove.setBackgroundDrawable(removeButtonBackground);
- }
- }
- public String getAddButtonText() {
- return addButtonText;
- }
- public void setAddButtonText(String addButtonText) {
- this.addButtonText = addButtonText;
- mButtonAdd.setText(addButtonText);
- }
- public String getRemoveButtonText() {
- return removeButtonText;
- }
- public void setRemoveButtonText(String removeButtonText) {
- this.removeButtonText = removeButtonText;
- mButtonRemove.setText(removeButtonText);
- }
- public int getQuantity() {
- return quantity;
- }
- public void setQuantity(int quantity) {
- boolean limitReached = false;
- if (quantity > maxQuantity) {
- quantity = maxQuantity;
- limitReached = true;
- if (onQuantityChangeListener != null) onQuantityChangeListener.onLimitReached();
- }
- if (quantity < minQuantity) {
- quantity = minQuantity;
- limitReached = true;
- if (onQuantityChangeListener != null) onQuantityChangeListener.onLimitReached();
- }
- if (!limitReached && onQuantityChangeListener != null)
- onQuantityChangeListener.onQuantityChanged(quantity, true);
- this.quantity = quantity;
- mTextViewQuantity.setText(String.valueOf(this.quantity));
- }
- public int getMaxQuantity() {
- return maxQuantity;
- }
- public void setMaxQuantity(int maxQuantity) {
- this.maxQuantity = maxQuantity;
- }
- public int getMinQuantity() {
- return minQuantity;
- }
- public void setMinQuantity(int minQuantity) {
- this.minQuantity = minQuantity;
- }
- public int getQuantityPadding() {
- return quantityPadding;
- }
- public void setQuantityPadding(int quantityPadding) {
- this.quantityPadding = quantityPadding;
- mTextViewQuantity.setPadding(quantityPadding, 0, quantityPadding, 0);
- }
- public int getQuantityTextColor() {
- return quantityTextColor;
- }
- public void setQuantityTextColor(int quantityTextColor) {
- this.quantityTextColor = quantityTextColor;
- mTextViewQuantity.setTextColor(quantityTextColor);
- }
- public void setQuantityTextColorRes(int quantityTextColorRes) {
- this.quantityTextColor = ContextCompat.getColor(getContext(), quantityTextColorRes);
- mTextViewQuantity.setTextColor(quantityTextColor);
- }
- public int getAddButtonTextColor() {
- return addButtonTextColor;
- }
- public void setAddButtonTextColor(int addButtonTextColor) {
- this.addButtonTextColor = addButtonTextColor;
- mButtonAdd.setTextColor(addButtonTextColor);
- }
- public void setAddButtonTextColorRes(int addButtonTextColorRes) {
- this.addButtonTextColor = ContextCompat.getColor(getContext(), addButtonTextColorRes);
- mButtonAdd.setTextColor(addButtonTextColor);
- }
- public int getRemoveButtonTextColor() {
- return removeButtonTextColor;
- }
- public void setRemoveButtonTextColor(int removeButtonTextColor) {
- this.removeButtonTextColor = removeButtonTextColor;
- mButtonRemove.setTextColor(removeButtonTextColor);
- }
- public void setRemoveButtonTextColorRes(int removeButtonTextColorRes) {
- this.removeButtonTextColor = ContextCompat.getColor(getContext(), removeButtonTextColorRes);
- mButtonRemove.setTextColor(removeButtonTextColor);
- }
- private int dpFromPx(final float px) {
- return (int) (px / getResources().getDisplayMetrics().density);
- }
- private int pxFromDp(final float dp) {
- return (int) (dp * getResources().getDisplayMetrics().density);
- }
- private boolean isNumber(String string) {
- try {
- Integer.parseInt(string);
- return true;
- } catch (Exception e) {
- return false;
- }
- }
- }
qv_dialog_changequantity.xml
- <?xml version="1.0" encoding="utf-8"?>
- <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:layout_width="match_parent"
- android:layout_height="match_parent"
- android:orientation="vertical">
- <EditText
- android:id="@+id/qv_et_change_qty"
- android:layout_width="match_parent"
- android:layout_height="wrap_content"
- android:layout_margin="10dp"
- android:inputType="number" />
- </LinearLayout>
attr.xml
- <resources>
- <declare-styleable name="QuantityView">
- <attr name="qv_addButtonText" format="string" />
- <attr name="qv_addButtonBackground" format="color|reference" />
- <attr name="qv_addButtonTextColor" format="color" />
- <attr name="qv_removeButtonText" format="string" />
- <attr name="qv_removeButtonBackground" format="color|reference" />
- <attr name="qv_removeButtonTextColor" format="color" />
- <attr name="qv_quantity" format="integer" />
- <attr name="qv_quantityBackground" format="color|reference" />
- <attr name="qv_quantityTextColor" format="color" />
- <attr name="qv_quantityPadding" format="dimension" />
- <attr name="qv_maxQuantity" format="integer" />
- <attr name="qv_minQuantity" format="integer" />
- </declare-styleable>
- </resources>
string.xml
- <string name="qv_add">+</string>
- <string name="qv_remove">-</string>
drawable:
qv_bg_selector.xml
- <?xml version="1.0" encoding="utf-8"?>
- <selector xmlns:android="http://schemas.android.com/apk/res/android">
- <item android:state_pressed="true">
- <shape android:shape="rectangle">
- <solid android:color="#ddd" />
- </shape>
- </item>
- <item android:state_selected="true">
- <shape android:shape="rectangle">
- <solid android:color="#ddd" />
- </shape>
- </item>
- <item>
- <shape android:shape="rectangle">
- <solid android:color="#eee" />
- </shape>
- </item>
- </selector>
qv_btn_selector.xml
- <?xml version="1.0" encoding="utf-8"?>
- <selector xmlns:android="http://schemas.android.com/apk/res/android">
- <item android:state_pressed="true">
- <shape android:shape="rectangle">
- <solid android:color="#ccc" />
- </shape>
- </item>
- <item android:state_selected="true">
- <shape android:shape="rectangle">
- <solid android:color="#ccc" />
- </shape>
- </item>
- <item>
- <shape android:shape="rectangle">
- <solid android:color="#ddd" />
- </shape>
- </item>
- </selector>