using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.IO;
using System.Runtime.InteropServices;
using System.Data.SqlClient;
using System.Collections;
using System.Windows;
using System.Threading;
using System.Management;
namespace TF_ReadWrite_Tools.V1._00
{
public partial class Form1 : Form
{
private List<String>Dirve_s;//读取U盘盘符
private List<String> Client_Info;//客户信息
private List<ComboBox> TestItemDrive;//测试项盘符
private List<Label> TestItemResult;//测试项结果
private int Execut_Count = 0;//执行的数量
private int Execut_PassCount = 0;//执行的PASS数
private int Execut_Status = 0;//当前启动状态
public String Isn = String.Empty;//ISN条码
public Boolean GetUSB_Dive()
{
Boolean Flag=false;
try
{
Dirve_s = new List<string>();
DriveInfo[] allDrives = DriveInfo.GetDrives();
foreach (DriveInfo d in allDrives)
{
if (d.DriveType == DriveType.Removable)
Dirve_s.Add(d.Name);
}
Flag = true;
}
catch (Exception ex)
{
MessageBox.Show(ex.ToString());
Flag = false;
}
return Flag;
}
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
Client_Info=new List<String>();
BaseData GetClient_Info = new BaseData(@"SERVER2\SERVER2", @"E_Other_Product_Test_Data", "sa", "adminsystem", @"SELECT * FROM Mian_Yuan_Client_Information");
if (GetClient_Info.Get_DataBase_Args_Data(Client_Info, comboBox9))
{
TestItemDrive = new List<ComboBox>();
TestItemResult = new List<Label>();
TestItemDrive.Add(comboBox1);
TestItemDrive.Add(comboBox2);
TestItemDrive.Add(comboBox3);
TestItemDrive.Add(comboBox4);
TestItemDrive.Add(comboBox5);
TestItemDrive.Add(comboBox6);
TestItemDrive.Add(comboBox7);
TestItemDrive.Add(comboBox8);
TestItemResult.Add(label34);
TestItemResult.Add(label35);
TestItemResult.Add(label36);
TestItemResult.Add(label37);
TestItemResult.Add(label38);
TestItemResult.Add(label39);
TestItemResult.Add(label43);
TestItemResult.Add(label40);
comboBox9.Enabled = true;
comboBox9.Focus();
}
}
private void textBox4_KeyPress(object sender, KeyPressEventArgs e)
{
if (e.KeyChar == (char)Keys.Enter)
{
if (textBox4.Text=="test"||textBox4.Text=="TEST")
{
if (GetUSB_Dive())
{
foreach (ComboBox i in TestItemDrive)
i.Text = "";
Execut_Count = 0;
Execut_PassCount = 0;
//Execut_Status = 0;
Isn = comboBox10.Text + comboBox2.Text + DateTime.Now.ToString("yyyyMMyyHHmmss");
//textBox4.Text = comboBox10.Text + comboBox2.Text + DateTime.Now.ToString("yyyyMMyyHHmmss");
timer1.Enabled = true;
for (int n = 0; n < Convert.ToInt32(comboBox11.Text); n++)
{
if(Dirve_s.Count>=n+1)TestItemDrive[n].Text = Dirve_s[n];
Drive_W_R_D_Test();
//Thread Test = new Thread(Drive_W_R_D_Test);
//Test.Start();
}
textBox4.Focus();
textBox4.SelectAll();
}
}
}
}
public void Drive_W_R_D_Test()
{
try
{
if (Drive_File_Write(Dirve_s[Execut_Count] + "TEST.log"))
{
if (Drive_File_Red(Dirve_s[Execut_Count] + "TEST.log"))
{
if (Drive_File_Delete(Dirve_s[Execut_Count] + "TEST.log"))
{
TestItemResult[Execut_Count].Text = "测试.Pass";
TestItemResult[Execut_Count].ForeColor = Color.Green;
Execut_PassCount++;
Execut_Count++;
}
else
{
TestItemResult[Execut_Count].Text = "删除测试.FAIL";
TestItemResult[Execut_Count].ForeColor = Color.Red;
Execut_Count++;
}
}
else
{
TestItemResult[Execut_Count].Text = "读取测试.FAIL";
TestItemResult[Execut_Count].ForeColor = Color.Red;
Execut_Count++;
}
}
else
{
TestItemResult[Execut_Count].Text = "写入测试.FAIL";
TestItemResult[Execut_Count].ForeColor = Color.Red;
Execut_Count++;
}
}
catch (Exception ex)
{
TestItemResult[Execut_Count].Text = "侦测Device错误";
TestItemResult[Execut_Count].ForeColor = Color.Red;
Execut_Count++;
}
}
private void comboBox9_SelectedIndexChanged(object sender, EventArgs e)//客户名称
{
if (comboBox9.Text != null && comboBox9.Text != String.Empty)
{
int n=0;
comboBox10.Items.Clear();
foreach (String ss in Client_Info)
{
String[] Array_Str = ss.Split(new String[] { "," }, StringSplitOptions.RemoveEmptyEntries);
if (n == 0)
comboBox10.Items.Add(Array_Str[1]);
else
{
Boolean Is_Find = false;
foreach (String ii in comboBox10.Items)
{
if (ii == Array_Str[1])
{
Is_Find = true;
break;
}
}
if(!Is_Find)
comboBox10.Items.Add(Array_Str[1]);
}
n++;
}
if (n > 0)
{
comboBox9.Enabled = false;
comboBox10.Enabled = true;
comboBox10.Focus();
}
}
}
private void comboBox10_SelectedIndexChanged(object sender, EventArgs e)//产品型号
{
if (comboBox10.Text != null && comboBox10.Text != String.Empty)
{
int n = 0;
comboBox12.Items.Clear();
foreach (String s in Client_Info)
{
String[] Array_Str = s.Split(new String[] { "," }, StringSplitOptions.RemoveEmptyEntries);
if (n == 0)
comboBox12.Items.Add(Array_Str[2]);
else
{
Boolean IsFind = false;
foreach (String ss in comboBox12.Items)
{
if (ss == Array_Str[2])
{
IsFind = true;
break;
}
}
if(!IsFind)
comboBox12.Items.Add(Array_Str[2]);
}
n++;
}
if (n > 0)
{
comboBox10.Enabled = false;
comboBox12.Enabled = true;
comboBox12.Focus();
}
}
}
private void comboBox12_SelectedIndexChanged(object sender, EventArgs e)//机器编号
{
if (comboBox12.Text != null && comboBox12.Text != String.Empty)
{
comboBox12.Enabled = false;
textBox2.Enabled = true;
textBox2.Focus();
}
}
private void textBox2_KeyPress(object sender, KeyPressEventArgs e)//工号
{
if (e.KeyChar == (char)Keys.Enter)
{
if (textBox2.Text != null && textBox2.Text != String.Empty)
{
textBox2.Enabled = false;
comboBox11.Enabled = true;
comboBox11.Focus();
}
}
}
private void comboBox11_SelectedIndexChanged(object sender, EventArgs e)//绑码测试
{
foreach (String ii in Client_Info)
{
String []Array_Str=ii.Split(new String[] {","},StringSplitOptions.RemoveEmptyEntries);
if (Array_Str[0] == comboBox9.Text && Array_Str[1] == comboBox10.Text)
{
for (int n = 0; n < Convert.ToInt32(comboBox11.Text); n++)
{
TestItemResult[n].Text = "待检测...";
TestItemResult[n].BackColor = Color.Bisque;
}
textBox3.Text = Array_Str[3];
comboBox11.Enabled = false;
textBox4.Enabled = true;
textBox4.Focus();
}
}
}
public Boolean Drive_File_Delete(String Path_File_Name)//文本目录内容删除
{
Boolean Flag = false;
try
{
if (!File.Exists(Path_File_Name))
return false;
File.Delete(Path_File_Name);
if (File.Exists(Path_File_Name))
return false;
Flag = true;
}
catch (Exception ex)
{
Flag = false;
}
return Flag;
}
public Boolean Drive_File_Write(String Path_File_Name)//文本目录内容写入
{
Boolean Flag = false;
FileStream fs = new FileStream(Path_File_Name,FileMode.Create,FileAccess.Write);
StreamWriter sw = new StreamWriter(fs);
try
{
String Temp = @"Data Write Pass,"+DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss");
sw.WriteLine(Temp);
Flag = true;
}
catch (Exception ex)
{
Flag = false;
}
finally
{
sw.Close();
fs.Close();
}
return Flag;
}
public Boolean Drive_File_Red(String Path_File_Name)//文本目录内容读取
{
Boolean Flag = false;
FileStream fs = new FileStream(Path_File_Name,FileMode.Open,FileAccess.Read);
StreamReader sr = new StreamReader(fs,Encoding.Default);
try
{
String Temp = String.Empty;
int n = 0;
while ((Temp = sr.ReadLine()) != null)
{
n++;
}
if (n > 0)
Flag = true;
}
catch (Exception ex)
{
Flag = false;
}
finally
{
sr.Close();
fs.Close();
}
return Flag;
}
private void timer1_Tick(object sender, EventArgs e)
{
if (Execut_Count == Convert.ToInt32(comboBox11.Text))
{
if (Execut_PassCount == Convert.ToInt32(comboBox11.Text))
{
BaseData Insert_TestData = new BaseData(@"SERVER2\SERVER2", @"E_Other_Product_Test_Data", "sa", "adminsystem", "usp_Insert_TestData");
if (Insert_TestData.Insert_TestData(Isn, comboBox9.Text, comboBox10.Text, textBox2.Text))
{
BaseData Update_Client_Info = new BaseData(@"SERVER2\SERVER2", @"E_Other_Product_Test_Data", "sa", "adminsystem", "Update_Client_Info");
if (Update_Client_Info.Update_TestTotal(comboBox9.Text, comboBox10.Text))
{
int n = Convert.ToInt32(textBox3.Text) + 1;
textBox3.Text = n.ToString();
label59.Text = "PASS";
label59.Location = new Point(button1.Location.X + (button1.Width / 2 - label59.Width / 2), button1.Location.Y + (button1.Height / 2 - label59.Height / 2));//设置居中
label59.ForeColor = Color.Green;
}
else
{
label59.Text = "更新完成订单数据Fail";
label59.Location = new Point(button1.Location.X + (button1.Width / 2 - label59.Width / 2), button1.Location.Y + (button1.Height / 2 - label59.Height / 2));//设置居中
label59.ForeColor = Color.Green;
}
}
else
{
label59.Text = "上传测试数据Fail";
label59.Location = new Point(button1.Location.X + (button1.Width / 2 - label59.Width / 2), button1.Location.Y + (button1.Height / 2 - label59.Height / 2));//设置居中
label59.ForeColor = Color.Green;
}
}
else
{
label59.Text = "FAIL";
label59.Location = new Point(button1.Location.X + (button1.Width / 2 - label59.Width / 2), button1.Location.Y + (button1.Height / 2 - label59.Height / 2));//设置居中
label59.ForeColor = Color.Red;
}
timer1.Enabled = false;
}
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data.SqlClient;
using System.Drawing;
using System.IO;
using System.Collections;
using System.Windows;
using System.Web;
using System.Threading;
using System.Management;
using System.Net.NetworkInformation;
using System.Windows.Forms;
using System.Data;
namespace TF_ReadWrite_Tools.V1._00
{
public class BaseData:Form1
{
public String server;
public String database;
public String uid;
public String pwd;
public String Stored_Procedure;
public String sql_connect_str;
public SqlConnection sql_connect;
public SqlCommand cmd;
public BaseData(String server, String database, String uid, String pwd, String Stored_Procedure)
{
this.server = server;
this.database = database;
this.uid = uid;
this.pwd = pwd;
this.sql_connect_str = @"server=" + this.server + ";database=" + this.database + ";uid=" + this.uid + ";pwd=" + this.pwd;
this.Stored_Procedure = Stored_Procedure;
}
public Boolean Sql_Connect()
{
Boolean Flag = false;
try
{
this.sql_connect = new SqlConnection(this.sql_connect_str);
this.sql_connect.Open();
this.cmd = new SqlCommand(this.Stored_Procedure,this.sql_connect);
Flag = true;
}
catch (Exception ex)
{
MessageBox.Show(ex.ToString(),"系统提醒",MessageBoxButtons.OK,MessageBoxIcon.Error);
Flag = false;
}
return Flag;
}
public Boolean Update_TestTotal(String Client_Name, String Product_Name)//更新订单测试总数
{
Boolean Flag = false;
if (this.Sql_Connect())
{
this.cmd.CommandType = CommandType.StoredProcedure;
this.cmd.Parameters.Add("@Client_Name", Client_Name);
this.cmd.Parameters.Add("@Product_Name",Product_Name);
this.cmd.Parameters.Add("@rs",SqlDbType.Int).Direction=ParameterDirection.Output;
this.cmd.ExecuteScalar();
if ((int)this.cmd.Parameters["@rs"].Value == 0)
{
Flag = true;
}
else
{
Flag = false;
}
}
return Flag;
}
public Boolean Insert_TestData(String ISN, String Client_Name, String Product_Name, String Job_Number)//写入测试数据
{
Boolean Flag = false;
if (this.Sql_Connect())
{
this.cmd.CommandType = CommandType.StoredProcedure;
this.cmd.Parameters.Add("@ISN", ISN);
this.cmd.Parameters.Add("@Client_Name", Client_Name);
this.cmd.Parameters.Add("@Product_Name", Product_Name);
this.cmd.Parameters.Add("@Job_Number", Job_Number);
this.cmd.Parameters.Add("@rs", SqlDbType.Int).Direction = ParameterDirection.Output;
this.cmd.ExecuteScalar();
if ((int)this.cmd.Parameters["@rs"].Value == 0)
{
Flag = true;
}
else
{
Flag = false;
}
}
else
{
Flag = false;
}
return Flag;
}
public Boolean Get_DataBase_Args_Data(List<String> Args_List,ComboBox Item)//读取参数
{
Boolean Flag = false;
if (this.Sql_Connect())
{
SqlDataReader sdr = this.cmd.ExecuteReader();
//String Temp = String.Empty;
int n = 0;
Item.Items.Clear();
while (sdr.Read())
{
String Temp = String.Empty;
if (n == 0)
Item.Items.Add(sdr["Client_Name"].ToString());
else
{
Boolean IsFind = false;
foreach (String ss in Item.Items)
{
if (ss == sdr["Client_Name"].ToString())
{
IsFind = true;
break;
}
}
if(!IsFind)
Item.Items.Add(sdr["Client_Name"].ToString());
}
Temp = sdr["Client_Name"].ToString();
Temp= Temp+"," + sdr["Product_Name"].ToString();
Temp=Temp+ "," + sdr["Machine_Number"].ToString();
Temp = Temp+"," + sdr["Product_Complete_The_Number"].ToString();
Args_List.Add(Temp);
n++;
}
Flag = true;
}
else
Flag = false;
return Flag;
}
}
}
USE E_Other_Product_Test_Data
GO
IF EXISTS(SELECT * FROM SYS.OBJECTS WHERE NAME='Mian_Yuan_Client_Information')
DROP TABLE Mian_Yuan_Client_Information
GO
CREATE TABLE Mian_Yuan_Client_Information
(
NO INT IDENTITY(1,1) NOT NULL,
Client_Name nvarchar(50) NOT NULL,
Product_Name varchar(50) NOT NULL,
Machine_Number varchar(10) NOT NULL,
Product_Complete_The_Number INT NOT NULL
)
GO
USE E_Other_Product_Test_Data
GO
ALTER TABLE Mian_Yuan_Client_Information
ADD CONSTRAINT PK_Product_Name PRIMARY KEY(Product_Name)
GO
USE E_Other_Product_Test_Data
GO
IF EXISTS(SELECT * FROM SYS.OBJECTS WHERE NAME='Mian_Yuan_Client_TestData')
drop table Mian_Yuan_Client_TestData
GO
CREATE TABLE Mian_Yuan_Client_TestData
(
NO INT IDENTITY(1,1)NOT NULL,
ISN VARCHAR(50) NOT NULL,---------------------------------------ISN
Client_Name nvarchar(50) NOT NULL,------------------------------客户名称
Product_Name varchar(50) NOT NULL,------------------------------产品名称
Job_Number varchar(50) NOT NULL,--------------------------------工号
Test_Result varchar(10) NOT NULL,-------------------------------测试结果
Date_Time varchar(50)NOT NULL-----------------------------------时间
)
GO
USE E_Other_Product_Test_Data
GO
ALTER TABLE Mian_Yuan_Client_TestData
ADD CONSTRAINT PK_ISN Primary KEY(ISN)
GO
USE E_Other_Product_Test_Data
GO
IF EXISTS(SELECT * FROM SYS.OBJECTS WHERE NAME='usp_Insert_TestData')
DROP PROC usp_Insert_TestData
GO
CREATE PROC usp_Insert_TestData
@ISN varchar(50),
@Client_Name varchar(50),
@Product_Name varchar(50),
@Job_Number varchar(50),
@rs int output
AS
DECLARE @Date_Time varchar(50)
BEGIN
SET @Date_Time=CONVERT(VARCHAR(50),GETDATE(),25)
SELECT @rs=count(*) from Mian_Yuan_Client_TestData where ISN=@ISN
if @rs>=1
BEGIN
UPDATE Mian_Yuan_Client_TestData SET Client_Name=@Client_Name,Product_Name=@Product_Name,Job_Number=@Job_Number,
Date_Time=@Date_Time where ISN=@ISN
IF @@ERROR<=0
BEGIN
SET @rs=0
return @rs
END
ELSE
BEGIN
SET @rs=1
return @rs
END
END
ELSE
BEGIN
INSERT INTO Mian_Yuan_Client_TestData VALUES(@ISN,@Client_Name,@Product_Name,@Job_Number,'PASS',@Date_Time)
IF @@ERROR<=0
BEGIN
SET @rs=0
return @rs
END
ELSE
BEGIN
SET @rs=1
return @rs
END
END
END
set @rs=1
return @rs
GO
USE E_Other_Product_Test_Data
GO
IF EXISTS(SELECT * FROM SYS.OBJECTS WHERE NAME='Update_Client_Info')
drop proc Update_Client_Info
GO
CREATE PROC Update_Client_Info
@Client_Name varchar(50),
@Product_Name varchar(50),
@rs int output
AS
BEGIN
UPDATE Mian_Yuan_Client_Information SET Product_Complete_The_Number=Product_Complete_The_Number+1 WHERE Client_Name=@Client_Name AND Product_Name=@Product_Name
IF @@ERROR<=0
BEGIN
SET @rs=0
RETURN @rs
END
ELSE
BEGIN
SET @rs=1
RETURN @rs
END
END
SET @rs=2
RETURN @rs
GO
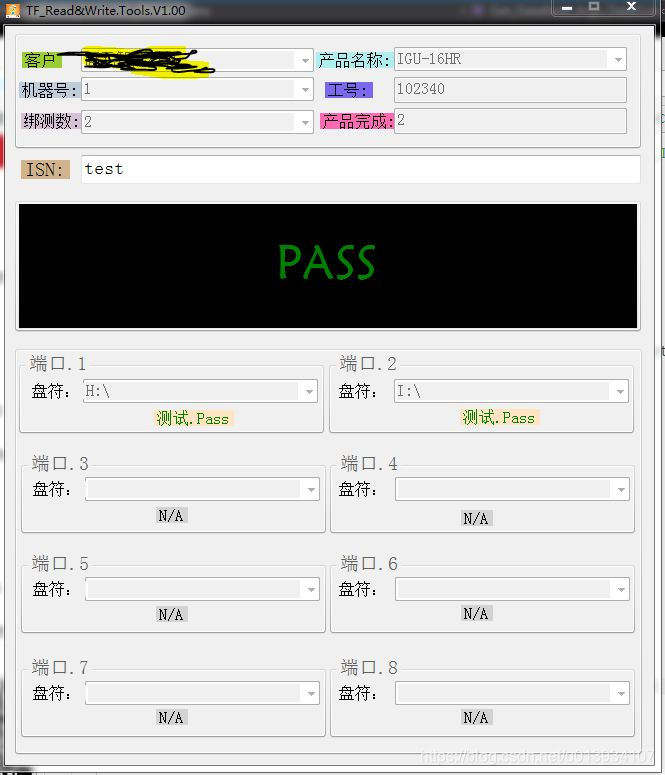