@这篇文档是由C++代码实现的线性表也就是链表
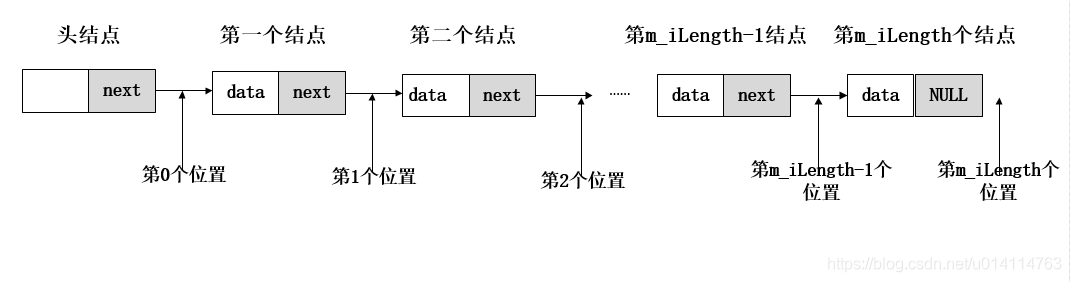
首先介绍一下链表的存储过程,如上图。每个结点分为data的数据域和next的指针域,单链表的第一个结点之前附设的一个结点称之为头结点。头结点的数据域可以不存储任何信息,也可存储如线性表的长度等类的附加信息,头结点的指针域存储指向第一个结点的指针。
本文是以Visual Studio中新建的C++win32的控制台应用程序实现的,其中建立了头文件和源文件以及实现main()的demo文件,分别是List.h和List.cpp、demo.cpp、Node.h、Node.cpp、Person.h、Person.cpp文件。其中List.h和List.cpp定义了链表的主要数据成员和成员函数,Node.h和Node.cpp一起定义了结点的数据成员,包含了数据域和指针域。Person.h和Person.cpp定义了Node.h中定义个数据域中存储的都是哪种类型的数据,包含了联系人的姓名和电话。最后实现一个电话本存储的功能。
List.h文件
#ifndef LIST_H
#define LIST_H
#include "Node.h"
class List
{
public:
List();
virtual ~List();
void ClearList();
bool ListEmpty();
int ListLength();
bool GetElement(int i,Node *pNode);
int LocateElement(Node *pNode);
bool PriorElement(Node *ce,Node *pe);
bool NextElementa(Node *ce, Node *ne);
bool ListInsert(int i ,Node *pNode);
bool ListDelete(int i ,Node *pNode);
void ListTraversal();
bool ListInsertHead(Node *pNode);
bool ListInsertTail(Node *pNode);
private:
Node *m_pList;
int m_iLength;
};
#endif
List.cpp文件
#include "List.h"
#include <iostream>
using namespace std;
List::List()
{
m_pList = new Node;
m_pList->next = NULL;
m_iLength = 0;
}
List::~List()
{
ClearList();
delete m_pList;
m_pList = NULL;
}
void List::ClearList()
{
Node *currentNode = m_pList->next;
while(currentNode != NULL)
{
Node *temp = currentNode->next;
delete currentNode;
currentNode = temp;
}
m_pList->next = NULL;
m_iLength = 0;
}
bool List::ListEmpty()
{
return m_iLength==0?true:false;
}
int List::ListLength()
{
return m_iLength;
}
bool List::GetElement(int i,Node *pNode)
{
if (i>=0 && i< m_iLength)
{
Node *currentNode = m_pList;
for (int k=0; k <=i; k++)
{
currentNode = currentNode->next;
}
pNode->data = currentNode->data;
return true;
}
else
{
return false;
}
}
int List::LocateElement(Node *pNode)
{
Node *currentnode = m_pList->next;
for (int i=0;i < m_iLength;i++)
{
if (currentnode->data == pNode->data)
{
return i;
}
currentnode = currentnode->next;
}
return -1;
}
bool List::PriorElement(Node *ce,Node *pe)
{
Node *currentNode = m_pList->next;
for (int i=0;i < m_iLength;i++)
{
if ((currentNode->next)->data == ce->data)
{
pe->data = currentNode->data;
return true;
}
currentNode = currentNode->next;
}
return false;
}
bool List::NextElementa(Node *ce, Node *ne)
{
Node *currentNode = m_pList->next;
for (int i=0;i < m_iLength-1;i++)
{
if (currentNode->data == ce->data)
{
ne->data = (currentNode->next)->data;
return true;
}
currentNode = currentNode->next;
}
return false;
}
bool List::ListInsert(int i ,Node *pNode)
{
if (i<0 || i>m_iLength)
{
return false;
}
else
{
Node *currentNode = m_pList;
for(int k=0; k<i;k++)
{
currentNode = currentNode->next;
}
Node *newNode2 = new Node;
if (newNode2 == NULL)
{
return false;
}
newNode2->data = pNode->data;
newNode2->next = currentNode->next;
currentNode->next = newNode2;
m_iLength++;
return true;
}
}
bool List::ListDelete(int i ,Node *pNode)
{
if (i<0 || i>=m_iLength)
{
return false;
}
else
{
Node *currentNode = m_pList;
for(int k=0; k<i; k++)
{
currentNode = currentNode->next;
}
pNode->data = (currentNode->next)->data;
currentNode->next = (currentNode->next)->next;
m_iLength--;
return true;
}
}
void List::ListTraversal()
{
if (ListEmpty())
{
cout << "该线性表为空!" <<endl;
}
else
{
Node *currentNode = m_pList->next;
for (int i=0;i < m_iLength;i++)
{
cout << currentNode->data <<endl;
currentNode = currentNode->next;
}
delete currentNode;
currentNode = NULL;
}
}
bool List::ListInsertHead(Node *pNode)
{
Node *temp = m_pList->next;
Node *newnode = new Node;
if (newnode == NULL)
{
return false;
}
newnode->data = pNode->data;
newnode->next = temp;
m_pList->next = newnode;
m_iLength++;
return true;
}
bool List::ListInsertTail(Node *pNode)
{
Node *currendnode = m_pList;
while(currendnode->next != NULL)
{
currendnode = currendnode->next;
}
Node *newnode1 = new Node;
if (newnode1 == NULL)
{
return false;
}
newnode1->data = pNode->data;
currendnode->next = newnode1;
newnode1->next = NULL;
m_iLength++;
return true;
}
Node.h文件
#ifndef NODE_H
#define NODE_H
#include "Person.h"
class Node
{
public:
Person data;
Node* next;
void printNode();
};
#endif
Node.cpp文件
#include "Node.h"
#include <iostream>
using namespace std;
void Node::printNode()
{
cout << data.name << endl;
cout << data.phone << endl;
}
Person.h文件
#ifndef PERSON_H
#define PERSON_H
#include <string>
#include <ostream>
using namespace std;
class Person
{
friend ostream &operator<<(ostream &out, Person &person);
public:
string name;
string phone;
Person &operator = (Person &person);
bool operator == (Person &person);
};
#endif
Person.h文件
#include "Person.h"
ostream &operator<<(ostream &out, Person &person)
{
out << person.name << "—" << person.phone;
return out;
}
Person &Person::operator = (Person &person)
{
this->name = person.name;
this->phone = person.phone;
return *this;
}
bool Person::operator == (Person &person)
{
if (this->name==person.name || this->phone==person.phone)
{
return true;
}
else
{
return false;
}
}
demo.cpp文件
#include "List.h"
#include "Node.h"
#include <iostream>
using namespace std;
int menu()
{
cout << "功能菜单:" << endl;
cout << "1.新建联系人" << endl;
cout << "2.删除联系人" << endl;
cout << "3.浏览通讯录" << endl;
cout << "4.退出通讯录" << endl;
cout << "请输入:";
int order = 0;
cin >> order;
return order;
}
void createPerson(List *pList)
{
Node node;
cout << "请输入联系人姓名:" << endl;
cin >> node.data.name;
cout << "请输入联系人电话:" << endl;
cin >> node.data.phone;
pList->ListInsertTail(&node);
}
void deletePerson(List *pList)
{
Node node;
cout << "请输入要删除的联系人姓名:" << endl;
cin >> node.data.name;
int i =pList->LocateElement(&node);
pList->ListDelete(i,&node);
cout << "删除联系人:" << node.data.name << endl;
}
int main(void)
{
int userOrder = 0;
List *pList = new List();
while(userOrder !=4)
{
userOrder = menu();
switch(userOrder)
{
case 1:
cout << "用户指令—新建联系人:" <<endl;
createPerson(pList);
break;
case 2:
cout << "用户指令—删除联系人:" <<endl;
deletePerson(pList);
break;
case 3:
cout << "用户指令—浏览通讯录:" <<endl;
pList->ListTraversal();
break;
case 4:
cout << "用户指令—退出通讯录:" <<endl;
break;
}
}
delete pList;
pList = NULL;
system("pause");
return 0;
}