1. 问题英文描述:
Given an array nums
, write a function to move all 0
's to the end of it while maintaining the relative order of the non-zero elements.
For example, given nums = [0, 1, 0, 3, 12]
, after calling your function, nums
should be [1, 3, 12, 0, 0]
.
Note:
- You must do this in-place without making a copy of the array.
- Minimize the total number of operations.
2. 问题中文描述:
给定一个数组nums,编写一个函数,使得数组中所有的0都移到后面,同时保持非零元素的相对位置。
例如: 给定数组 nums=[0,1,0,3,12],得到结果nums=[1,3,12,0,0]
注意:
1.不能复制数组,只能在该数组变量进行
2.使得操作数最小化
3.解题思路
1.C++有模板vector,可作为可变长数组,具有任意增加和删除数组元素的功能
2. 遍历数组nums,遇到零则删除,在数组末尾push 0,然后再从原位置继续遍历;如果没有遇到零,则迭代器+1.
4. 代码:
class Solution {
public:
void moveZeroes(vector<int>& nums) {
int len=nums.size();
vector<int>::iterator it=nums.begin();
for(int i=0;i<len;i++)
{
if(*it==0)
{
vector<int>::iterator it2=it;
nums.erase(it2);
nums.push_back(0);
}
else
{
it++;
}
}
}
};
5.运行结果
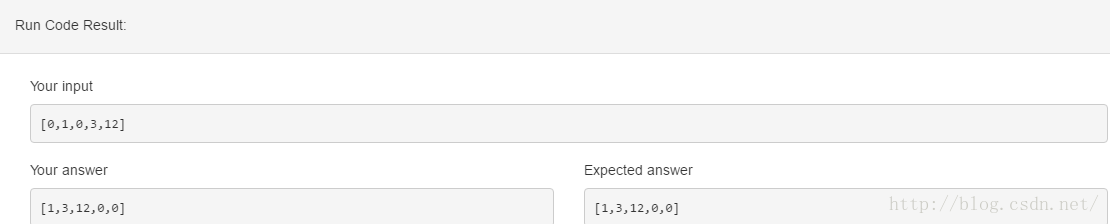
Given an array nums
, write a function to move all 0
's to the end of it while maintaining the relative order of the non-zero elements.
For example, given nums = [0, 1, 0, 3, 12]
, after calling your function, nums
should be [1, 3, 12, 0, 0]
.
Note:
- You must do this in-place without making a copy of the array.
- Minimize the total number of operations.
class Solution {
public:
void moveZeroes(vector<int>& nums) {
int len=nums.size();
vector<int>::iterator it=nums.begin();
for(int i=0;i<len;i++)
{
if(*it==0)
{
vector<int>::iterator it2=it;
nums.erase(it2);
nums.push_back(0);
}
else
{
it++;
}
}
}
};
5.运行结果