Chandelier
Time Limit: 1000MS | Memory Limit: 65536K | |||
Total Submissions: 1003 | Accepted: 397 | Special Judge |
Description
Lamps-O-Matic company assembles very large chandeliers. A chandelier consists of multiple levels. On the first level crystal pendants are attached to the rings. Assembled rings and new pendants are attached to the rings of the next level, and so on. At the end there is a single large ring -- the complete chandelier with multiple smaller rings and pendants hanging from it.
A special-purpose robot assembles chandeliers. It has a supply of crystal pendants and empty rings, and a stack to store elements of a chandelier during assembly. Initially the stack is empty. Robot executes a list of commands to assemble a chandelier.
On command "a" robot takes a new crystal pendant and places it on the top of the stack. On command "1" to "9" robot takes the corresponding number of items from the top of the stack and consecutively attaches them to the new ring. The newly assembled ring is then placed on the top of the stack. At the end of the program there is a single item on the stack -- the complete chandelier.
Unfortunately, for some programs it turns out that the stack during their execution needs to store too many items at some moments. Your task is to optimize the given program, so that the overall design of the respective chandelier remains the same, but the maximal number of items on the stack during the execution is minimal. A pendant or any complex multi-level assembled ring count as a single item of the stack.
The design of a chandelier is considered to be the same if each ring contains the same items in the same order. Since rings are circular it does not matter what item is on the top of the stack when the robot receives a command to assemble a new ring, but the relative order of the items on the stack is important. For example, if the robot receives command "4" when items < i1, i2, i3, i4 > are on the top of the stack in this order (i1 being the topmost), then the same ring is also assembled if these items are arranged on the stack in the following ways: < i2, i3, i4, i1 >, or < i3, i4, i1, i2 >, or < i4, i1, i2, i3 >.
A special-purpose robot assembles chandeliers. It has a supply of crystal pendants and empty rings, and a stack to store elements of a chandelier during assembly. Initially the stack is empty. Robot executes a list of commands to assemble a chandelier.
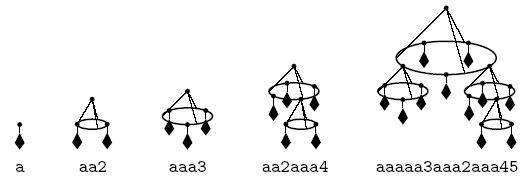
On command "a" robot takes a new crystal pendant and places it on the top of the stack. On command "1" to "9" robot takes the corresponding number of items from the top of the stack and consecutively attaches them to the new ring. The newly assembled ring is then placed on the top of the stack. At the end of the program there is a single item on the stack -- the complete chandelier.
Unfortunately, for some programs it turns out that the stack during their execution needs to store too many items at some moments. Your task is to optimize the given program, so that the overall design of the respective chandelier remains the same, but the maximal number of items on the stack during the execution is minimal. A pendant or any complex multi-level assembled ring count as a single item of the stack.
The design of a chandelier is considered to be the same if each ring contains the same items in the same order. Since rings are circular it does not matter what item is on the top of the stack when the robot receives a command to assemble a new ring, but the relative order of the items on the stack is important. For example, if the robot receives command "4" when items < i1, i2, i3, i4 > are on the top of the stack in this order (i1 being the topmost), then the same ring is also assembled if these items are arranged on the stack in the following ways: < i2, i3, i4, i1 >, or < i3, i4, i1, i2 >, or < i4, i1, i2, i3 >.
Input
The input contains a single line with a valid program for the robot. The program consists of at most 10 000 characters.
Output
On the first line of the output file write the minimal required stack capacity (number of items it can hold) to assemble the chandelier. On the second line write some program for the assembly robot that uses stack of this capacity and results in the same chandelier.
Sample Input
aaaaa3aaa2aaa45
Sample Output
6 aaa3aaa2aaa4aa5
题意:在一个栈中,每个单位空间可以存一个吊灯,然后数字代表把栈前面的几个组合起来,组合后的吊灯再放入栈中,不能改变吊灯的顺序,问你怎么组合能使需要的栈的大小最小。
思路:样例中一个灯有5的子灯,每个子灯所需要的栈的大小为 1 1 3 1 4 那么把这个序列按顺序加上0 1 2 3 4 时,需要的栈的大小为8,但是如果是1 2 3 4 0 的话,需要的栈的大小就是6。这么加是因为你如果你在装第三个灯的时候,你前面需要两个单位的栈去存放前两个灯,所以需要+2。然后这个问题用分差去做。
AC代码如下:
#include<cstdio>
#include<cstring>
#include<algorithm>
#include<vector>
using namespace std;
int left[10010],dp[10010][10010],dp2[10010][10010],num[10010],num2[10010],q[10010],h[10010],f;
char s[10010];
int solve(int m)
{
int i,j,k,ret;
for(i=1;i<=m;i++)
num2[i]=num[i]+i-1;
q[1]=num2[1];h[m]=num2[m];
for(i=2;i<=m;i++)
if(num2[i]>=q[i-1])
q[i]=num2[i];
else
q[i]=q[i-1];
for(i=m-1;i>=1;i--)
if(num2[i]>=h[i+1])
h[i]=num2[i];
else
h[i]=h[i+1];
ret=q[m];f=m;
for(i=1;i<m;i++)
if(max(q[i]+m-i,h[i+1]-i)<ret)
{
ret=max(q[i]+m-i,h[i+1]-i);
f=i;
}
return ret;
}
void dfs(int l,int r)
{
if(l==r)
return;
int i,j,k,pos=r,ret;
k=s[r]-'0';
for(j=k;j>=1;j--)
{
dfs(left[pos-1],pos-1);
pos=left[pos-1];
}
pos=r;
for(j=k;j>=1;j--)
{
num[j]=dp[left[pos-1]][pos-1];
pos=left[pos-1];
}
ret=solve(k);
dp[l][r]=ret;
dp2[l][r]=f;
}
void print(int l,int r)
{
if(l==r)
{
printf("a");
return;
}
int i,j,k,pos=r,m=dp2[l][r];
k=s[r]-'0';
for(j=k;j>=1;j--)
{
num[j]=pos-1;
pos=left[pos-1];
}
vector<int> vc;
vc.push_back(0);
for(i=1;i<=k;i++)
vc.push_back(num[i]);
for(i=m+1;i<=k;i++)
print(left[vc[i]],vc[i]);
for(i=1;i<=m;i++)
print(left[vc[i]],vc[i]);
printf("%c",s[r]);
}
int main()
{
int n,i,j,k,pos,ret=0;
scanf("%s",s+1);
n=strlen(s+1);
for(i=1;i<=n;i++)
{
if(s[i]=='a')
{
left[i]=i;
dp[i][i]=1;
}
else
{
k=s[i]-'0';
pos=i;
ret=0;
for(j=k;j>=1;j--)
pos=left[pos-1];
left[i]=pos;
}
}
dfs(1,n);
printf("%d\n",dp[1][n]);
print(1,n);
printf("\n");
}