import re
import tkinter
import tkinter.messagebox
from pywin.scintilla.formatter import operators
window = tkinter.Tk()
window.geometry('480x400+400+100')
window.resizable(False, False)
window.configure(bg='silver')
window.attributes("-alpha", 0.95)
window.title('计算器')
def ButtonOperation(m):
"""按钮操作"""
theme = themeVar.get()
if theme.startswith('.'):
theme = '' + theme
if m in '0123456789':
theme += m
elif m == '.':
SegmentationPart = re.split(r'\+|-|\*|/|%|', theme)[-1]
if '.' in SegmentationPart:
tkinter.messagebox.showerror('错误', '重复出现小数点!')
return
else:
theme += m
elif m == 'Clear':
theme = ''
elif m in operators:
if theme.endswith(operators):
tkinter.messagebox.showerror('错误', '不允许存在连续运算符!')
return
else:
theme += m
elif m == 'Sqrt':
n = theme.split('.')
if all(map(lambda x: x.isdigit(), n)):
theme = eval(theme) ** 0.5
else:
tkinter.messagebox.showerror('错误', '算式错误!')
return
elif m == '=':
try:
theme = str(eval(theme))
except:
tkinter.messagebox.showerror('错误', '算式错误!')
return
themeVar.set(theme)
themeVar = tkinter.StringVar(window, '')
themeEntry = tkinter.Entry(window, textvariable=themeVar, font=('黑体', 18, 'bold', 'italic'), bg='LightGray',
justify='right', bd=3)
themeEntry['state'] = 'readonly'
themeEntry.place(x=10, y=10, width=460, height=40)
figure = list('1234567890.') + ['Sqrt']
index = 0
for row in range(4):
for col in range(3):
a = figure[index]
index += 1
m_figure = tkinter.Button(window, text=a, font=('黑体', 24, 'bold', 'italic'), justify='center',
bg='LightSlateGray',
activebackground='gray', activeforeground='white', bd=2.5,
command=lambda x=a: ButtonOperation(x))
m_figure.place(x=40 + col * 94, y=160 + row * 50, width=110, height=70)
operators = ('+', '-', '*', '/', '**', '//', '%')
for index, operator in enumerate(operators):
m_operator = tkinter.Button(window, text=operator, font=('黑体', 18, 'bold', 'italic'), anchor='center',
justify='center', bg='LightSkyBlue4',
border=2.1, command=lambda x=operator: ButtonOperation(x))
m_operator.place(x=340, y=160 + index * 30, width=110, height=40)
m_Clear = tkinter.Button(window, text='Clear', bg='LightSkyBlue3', font=('黑体', 18, 'bold', 'italic'), anchor='center',
border=2.1, command=lambda: ButtonOperation('Clear'))
m_Clear.place(x=40, y=100, width=260, height=40)
m_EqualSign = tkinter.Button(window, text='=', font=('黑体', 18, 'bold', 'italic'), bg='orange', anchor='center',
border=2.1, command=lambda: ButtonOperation('='))
m_EqualSign.place(x=340, y=100, width=110, height=40)
window.mainloop()
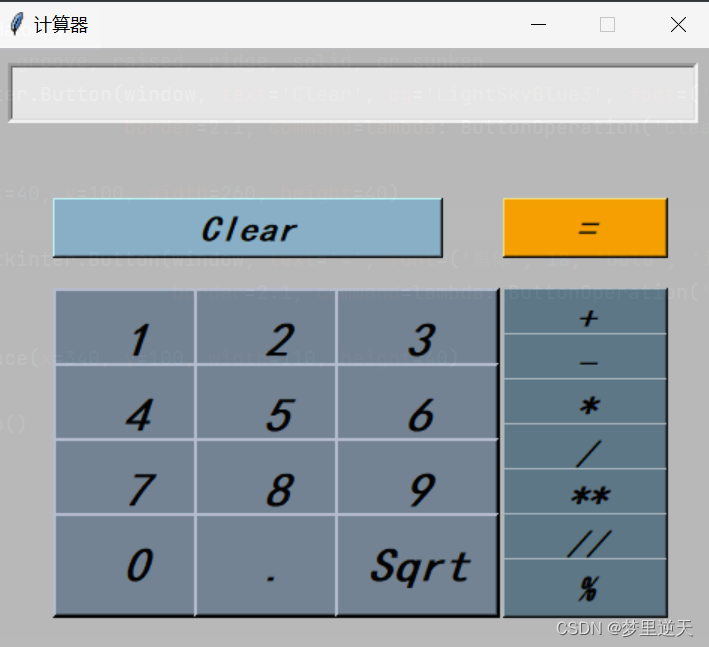
- python打包成exe文件
- 1.安装pyinstaller:pip install pyinstaller
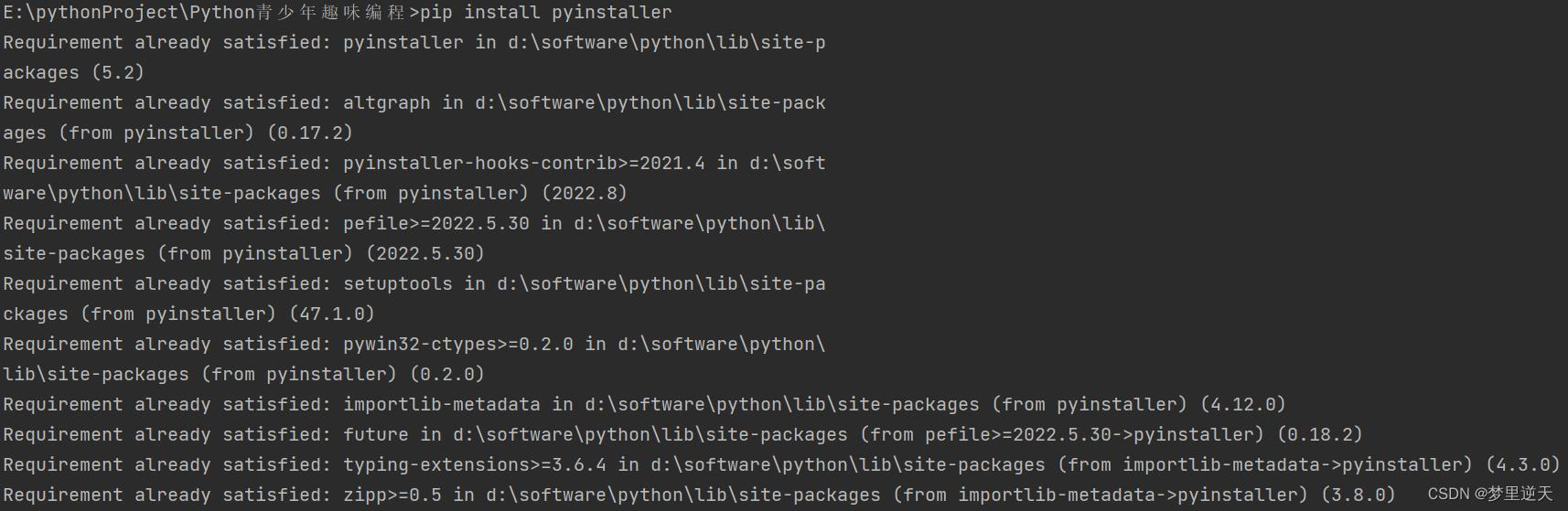
- 2.文件路径不能超过18个字符,最好直接放在磁盘下(如E:)
- 3.pyinstaller -F -w xx.py
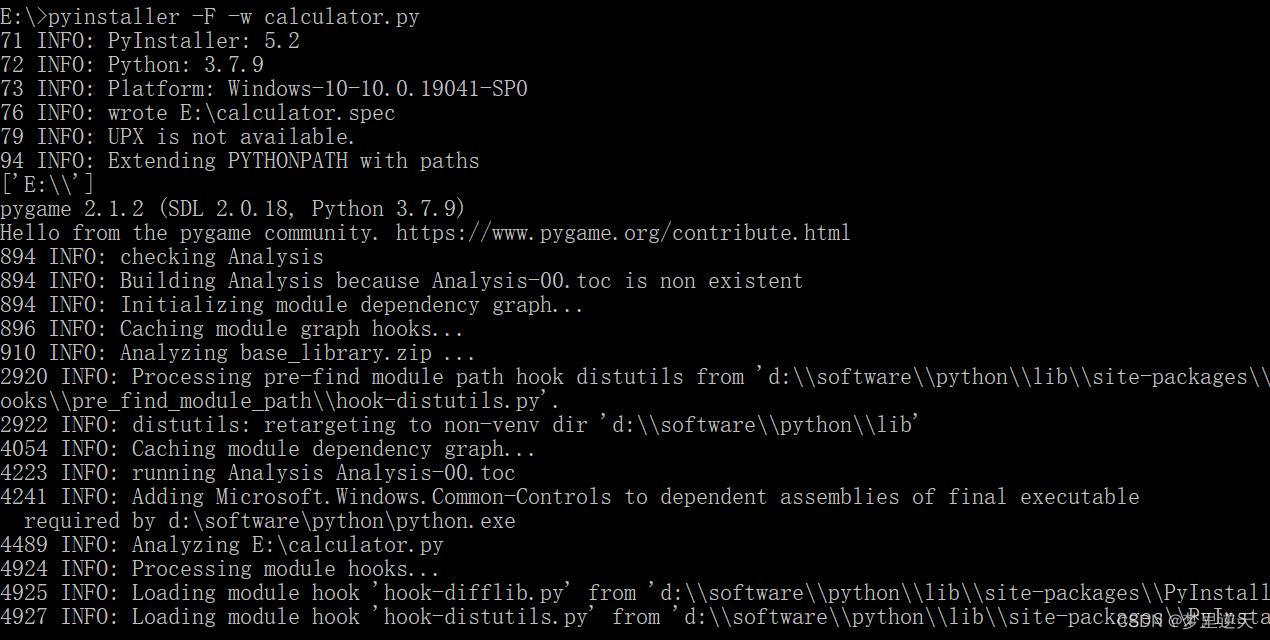
- 4.打包成功后,文件目录下会出现几个新的文件:dist + build + xx.spec,exe文件就在dist文件夹中。
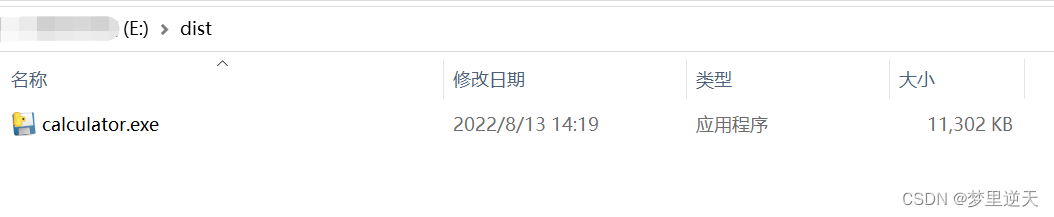
- 5.双击calculator.exe。
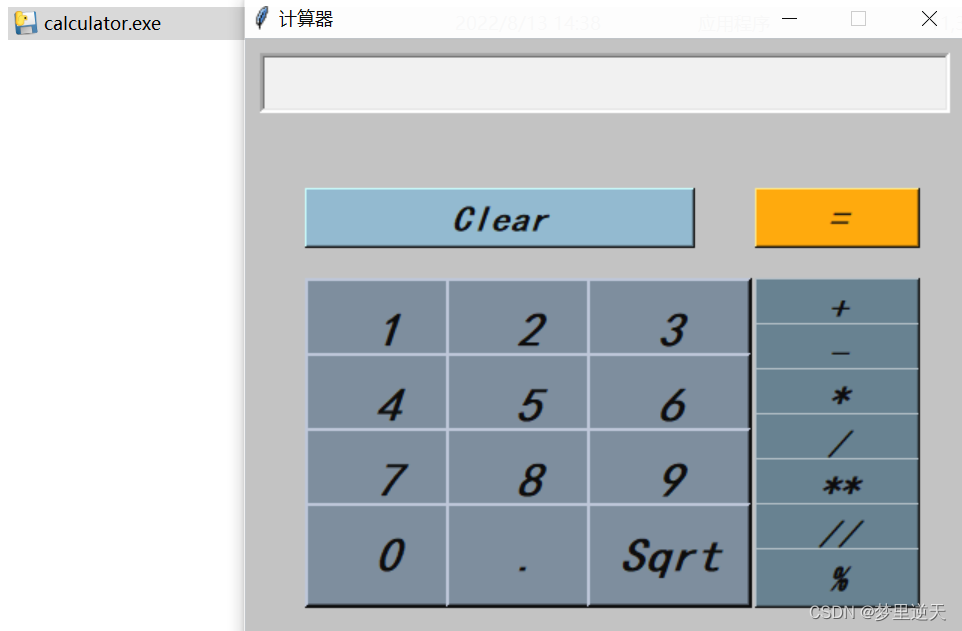