一.创建项目:
Asp.net Mvc4,基本 类型.
二.创建模型:
在Models目录下,创建类文件:Box.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.ComponentModel.DataAnnotations;
namespace MvcArray.Models
{
public class Box
{
[Display(Name="最大值:")]
public int iMax { set; get; }
[Display(Name = "最小值:")]
public int iMin { set; get; }
}
}
三.创建控制器:
HomeCtroller.cs代码如下:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using MvcArray.Models;
namespace MvcArray.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
int[] iArray = new int[100];
Random r = new Random();
for (int i = 0; i < 100; i++)
{
iArray[i] = r.Next(50, 1000);
}
int iMax, iMin;
iMax=iArray[0];
iMin = iArray[0];
for (int i = 0; i < 100; i++)
{
if (iArray[i] > iMax)
iMax=iArray[i] ;
if (iArray[i] < iMin)
iMin = iArray[i];
}
Box box = new Box();
box.iMin = iMin;
box.iMax = iMax;
ViewBag.box = box;
return View(iArray.ToList());
}
}
}
四.创建视图:
@model List<int>
@using MvcArray.Models
@{
ViewBag.Title = "Index";
var box = ViewBag.box as Box;
}
<h2>Index</h2>
@foreach (var i in Model)
{
<b>@i</b>
}
<h2>@box.iMax @box.iMin</h2>
五.测试预览.
[升级版]在上面基础上再演化
修改Box.cs文件,代码如下:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.ComponentModel.DataAnnotations;
namespace MvcArray.Models
{
public class Box
{
[Display(Name="最大值:")]
public int iMax { set; get; }
[Display(Name = "最小值:")]
public int iMin { set; get; }
[Display(Name = "平均值:")]
public float fAvg { set; get; }
[Display(Name = "最大值索引:")]
public int iMaxIndex { set; get; }
[Display(Name = "最小值索引:")]
public int iMinIndex { set; get; }
}
}
修改HomeController.cs文件如下:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using MvcArray.Models;
namespace MvcArray.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
int[] iArray = new int[100];
Random r = new Random();
for (int i = 0; i < 100; i++)
{
iArray[i] = r.Next(50, 1000);
}
Box box = new Box();
box.iMin = iArray.Min();
box.iMax = iArray.Max();
box.fAvg = (float)iArray.Average();
box.iMaxIndex = Array.IndexOf(iArray,box.iMax);
box.iMinIndex = Array.IndexOf(iArray, box.iMin);
ViewBag.iArray = iArray;
return View(box);
}
}
}
Index.cshtml修改如下:
@model MvcArray.Models.Box
@{
ViewBag.Title = "Asp.net Mvc4 前后端技术综合基本演练(1)";
var iArray = ViewBag.iArray as int[];
int iC = iArray.Count();
int iCC = 0;
}
<h2 style="color:Red;">提示:刷新界面,随机产生新的随机数组.</h2>
<table>
<caption>Asp.net Mvc4 前后端技术综合基本演练(1)<br />随机产生数组,并求最大最小值等<br />作者:ExeSoft.cn</caption>
@for (int i = 0; i < 10; i++)
{
<tr>
@for (int j= 0; j < 10; j++)
{
if (Model.iMaxIndex== iCC)
{
<td class="red"><span>@iCC</span>@iArray[iCC]</td>
}
else if (Model.iMinIndex== iCC)
{
<td class="yellow"><span>@iCC</span>@iArray[iCC]</td>
}
else
{
<td><span>@iCC</span>@iArray[iCC]</td>
}
iCC++;
if (iCC > iC)
{
break;
}
}
</tr>
if (iCC > iC)
{
break;
}
}
</table>
<fieldset>
<legend>统计参数</legend>
<div class="display-label">
@Html.DisplayNameFor(model => model.iMax)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.iMax)
</div>
<div class="display-label">
@Html.DisplayNameFor(model => model.iMin)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.iMin)
</div>
<div class="display-label">
@Html.DisplayNameFor(model => model.fAvg)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.fAvg)
</div>
<div class="display-label">
@Html.DisplayNameFor(model => model.iMaxIndex)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.iMaxIndex)
</div>
<div class="display-label">
@Html.DisplayNameFor(model => model.iMinIndex)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.iMinIndex)
</div>
</fieldset>
Site.cs文件如下:
fieldset
{
box-sizing: border-box;
width:60%;
padding: 40px;
margin: 0 auto 0 auto;
box-shadow: 3px 3px 3px grey;
border:1px solid grey;
text-shadow:2px 2px 1px grey;
}
legend {
font-size: 2em;
font-weight: bold;
}
table
{
margin-bottom:30px;
padding:40px;
margin-left:auto;
margin-right:auto;
width:60%;
box-shadow: 3px 3px 3px grey;
border:1px solid grey;
}
td
{
position:relative;
padding:4px;
box-shadow: 2px 2px 1px grey;
}
caption{
text-shadow:2px 2px 1px grey;
font-size:xx-large;
margin-bottom:20px;
}
.red{
background:radial-gradient(circle 20px at center,white 0%,blue);
}
.yellow{
background:radial-gradient(circle 20px at center,white 0%,yellow);
}
span
{
display:block;
position:absolute;
top:2px;
right:5px;
color:red;
font-size:0.5em;
}
预览效果: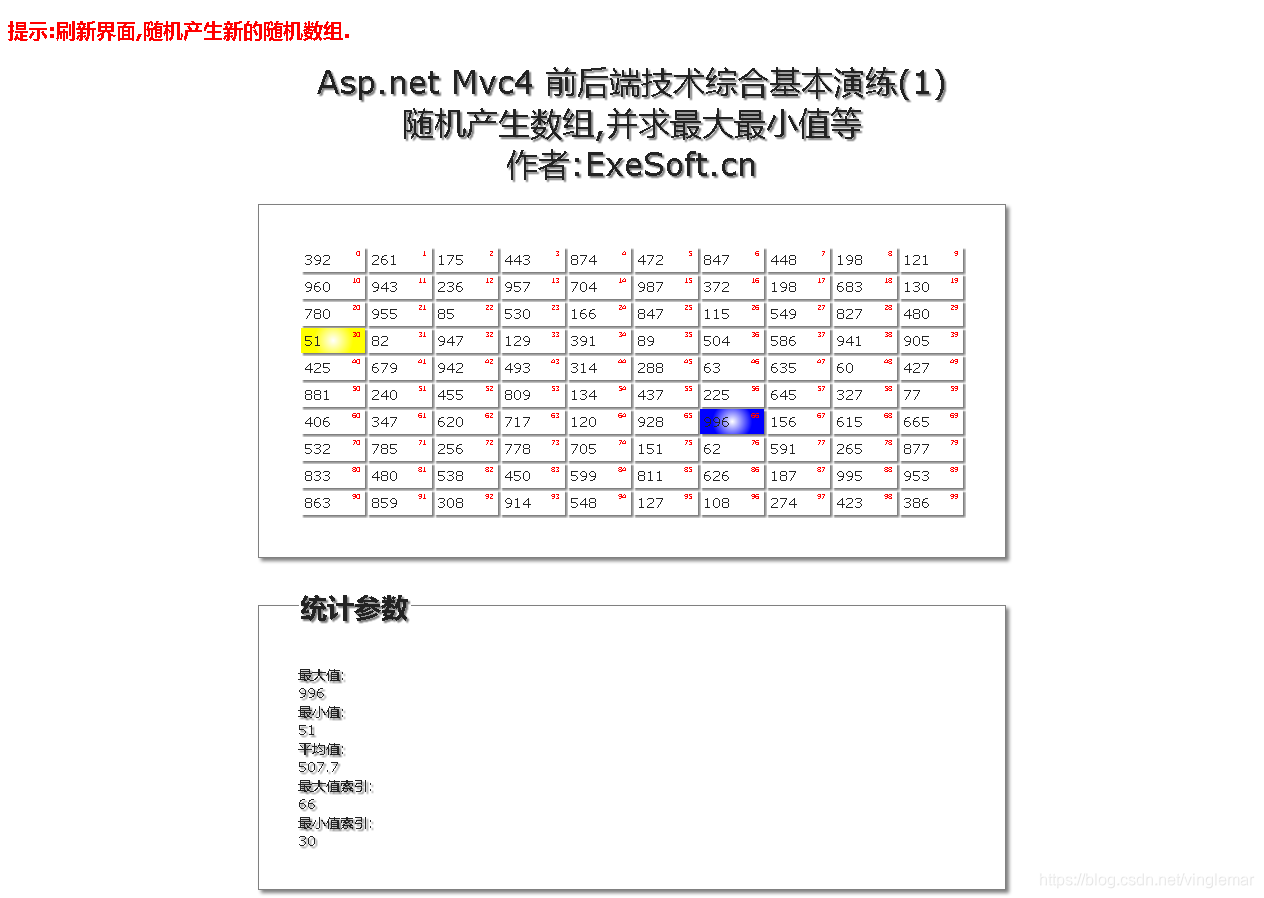
额外提示:
- 1.random.Next(): 返回非负的一个随机数
- 2.random.Next(MaxValue): 返回一个小于所指定最大值的非负随机数(注意:a.是小于最大值要生成的随机数的上界(随机数不能取该上界值);b.maxValue 类型:[System..::.Int32],maxValue 必须大于等于零。
- 3.Random.Next (minValue , maxValue): 返回一个指定范围内的随机数.一个大于等于 minValue 且小于 maxValue 的 32 位带符号整数,即:返回的值范围包括 minValue 但不包括maxValue。如果 minValue 等于 maxValue,则返回 minValue。