当我们使用Input时,我们可能会遇到一个问题,比如需要对用户输入的内容进行搜索时,当用户处于中文输入时,明明没有对内容进行确认,为什么会触发了onChange事件呢?
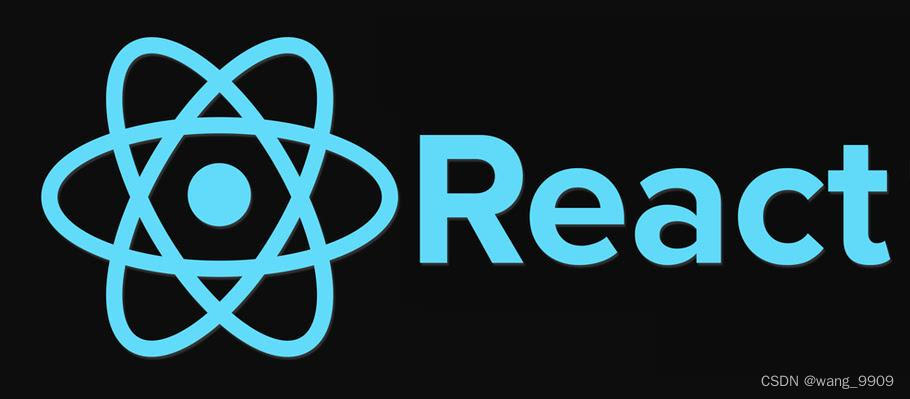
比如以下场景,中文一边输入另外一边onChange事件就已经被触发了,这样显然是不符合我们的需求的。无论我们对change事件如何做防抖和节流,当用户处于中文输入还没进行确认时,搜索请求就已经发送了。
const NewInput = () => {
const handleChange = (e: React.ChangeEvent<HTMLInputElement>) => {
console.log(e.target.value);
};
return <input onChange={handleChange} />;
};
export default NewInput;
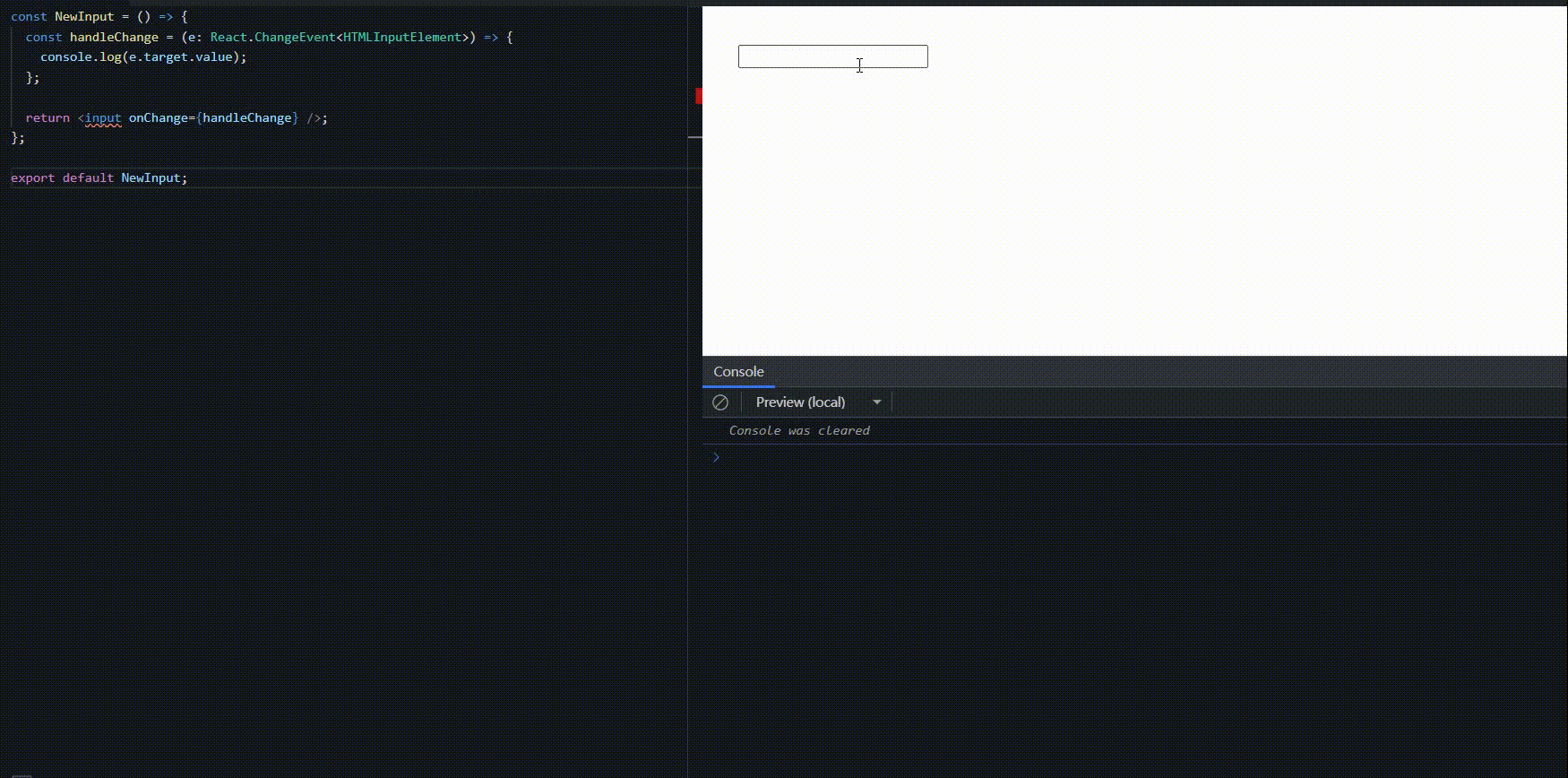
那有什么方法进行解决吗?
答案肯定是有的,不然这篇文章就不会写了。
首先,我们要知道input的事件有很多, 这里我们需要用到的方法是 onCompositionStart、 onCompositionUpdate以及 onCompositionEnd。这三个事件就可以让浏览器判断你的中文输入状态,分别代表着开始输入、正在输入中(输入更新)以及结束输入。
const NewInput = () => {
const handleChange = (e: React.ChangeEvent<HTMLInputElement>) => {
// console.log(e.target.value);
};
const Composition = (e: React.CompositionEvent<HTMLInputElement>) => {
console.log(e.type);
};
return (
<input
onChange={handleChange}
onCompositionStart={Composition}
onCompositionEnd={Composition}
onCompositionUpdate={Composition}
/>
);
};
export default NewInput;
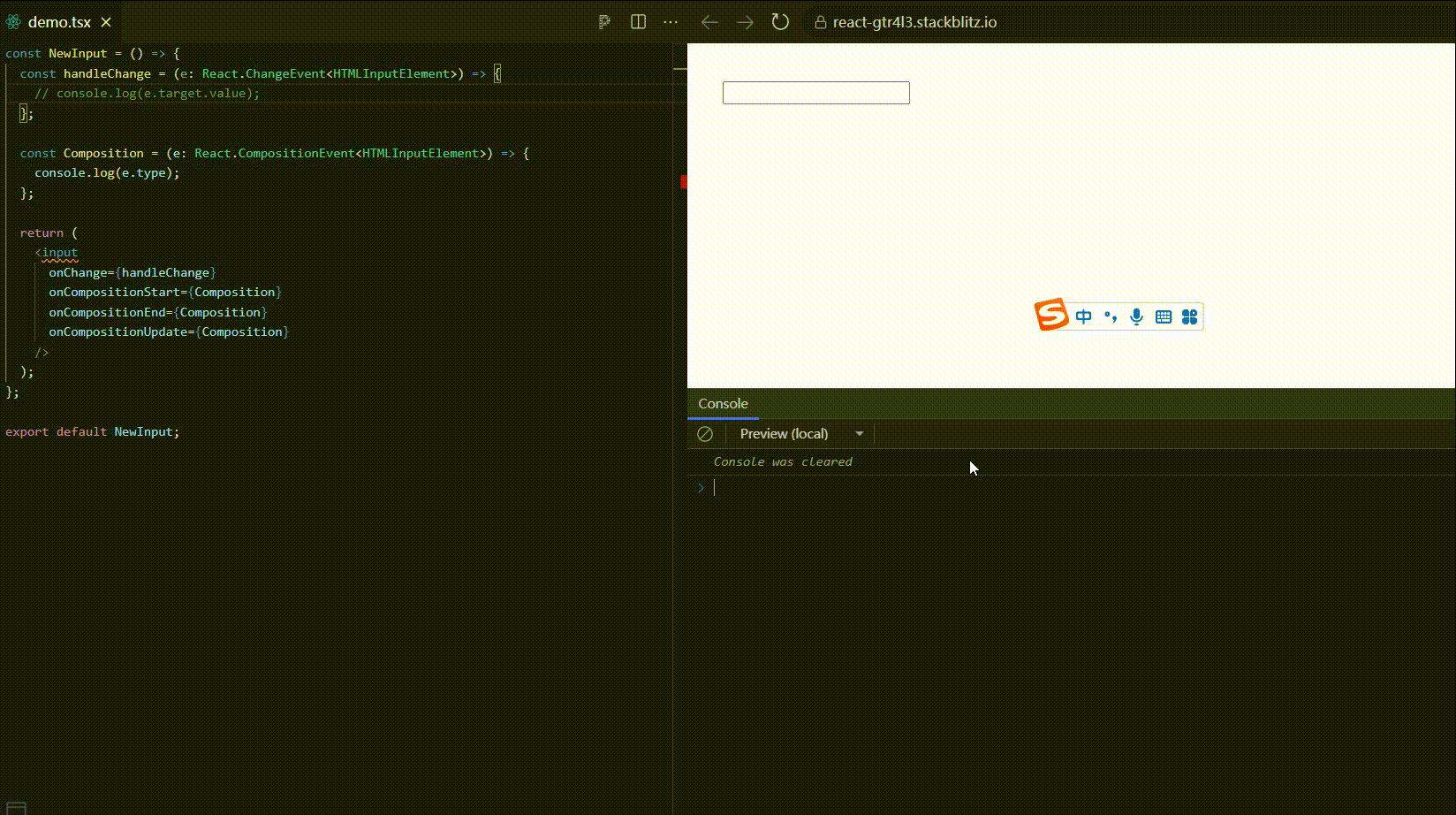
接下来,事情就要解决了,对input的onChange事件增加一个判断,当中文输入结束时才会触发搜索操作。
import { useRef } from 'react';
const NewInput = () => {
// 增加一个状态,记录中文输入状态
const compositionRef = useRef<boolean>(false);
const handleChange = (e: React.ChangeEvent<HTMLInputElement>) => {
if (!compositionRef.current) {
console.log(e.target.value);
}
};
const Composition = (e: React.CompositionEvent<HTMLInputElement>) => {
if (e.type === 'compositionend') {
compositionRef.current = false;
handleChange(e);
} else {
compositionRef.current = true;
}
};
return (
<input
onChange={handleChange}
onCompositionStart={Composition}
onCompositionEnd={Composition}
onCompositionUpdate={Composition}
/>
);
};
export default NewInput;
中文输入触发onChange异常的情况就算解决了。
既然问题解决了,那就再加个防抖节流操作吧。毕竟,都看到这里了,需求一步到位不是更好吗?
// 封装的具有解决中文输入异常问题的Input组件
import React, { useEffect, useRef } from 'react';
// props类型
interface InputProps extends React.InputHTMLAttributes<HTMLInputElement> {
value?: any;
onChange?: (e: any) => void;
}
export default (props: InputProps) => {
const { value, onChange, ...setProps } = props;
// 增加一个ref记录输入框的值,继承父组件的value
const inputRef = useRef<HTMLInputElement>(null);
const compositionRef = useRef<boolean>(false);
useEffect(() => {
if (inputRef.current) {
// 接受父组件传来的value
inputRef.current.value = value || '';
}
}, [value]);
const handleChange = (e: React.ChangeEvent<HTMLInputElement>) => {
if (!compositionRef.current) {
// 执行父组件的change事件
onChange && onChange(e);
}
};
const Composition = (e: React.CompositionEvent<HTMLInputElement>) => {
if (e.type === 'compositionend') {
compositionRef.current = false;
// 执行父组件的change事件
onChange && onChange(e);
} else {
compositionRef.current = true;
}
};
return (
<input
ref={inputRef}
onChange={handleChange}
onCompositionStart={Composition}
onCompositionEnd={Composition}
onCompositionUpdate={Composition}
{...setProps}
/>
);
};
// 父组件
import React, { useCallback, useState } from 'react';
import { createRoot } from 'react-dom/client';
import debounce from 'lodash-es/debounce';
import NewInput from './demo';
const App = () => {
const [value, setValue] = useState('');
const handleChange = (e) => {
setValue(e.target.value);
debounceSearch(e.target.value);
};
// 搜索操作进行防抖处理
const debounceSearch = useCallback(
debounce((e: string) => {
console.log(e);
// doSearch(e)
}, 800),
[]
);
return <NewInput value={value} onChange={handleChange} />;
};
createRoot(document.getElementById('container')).render(<App />);
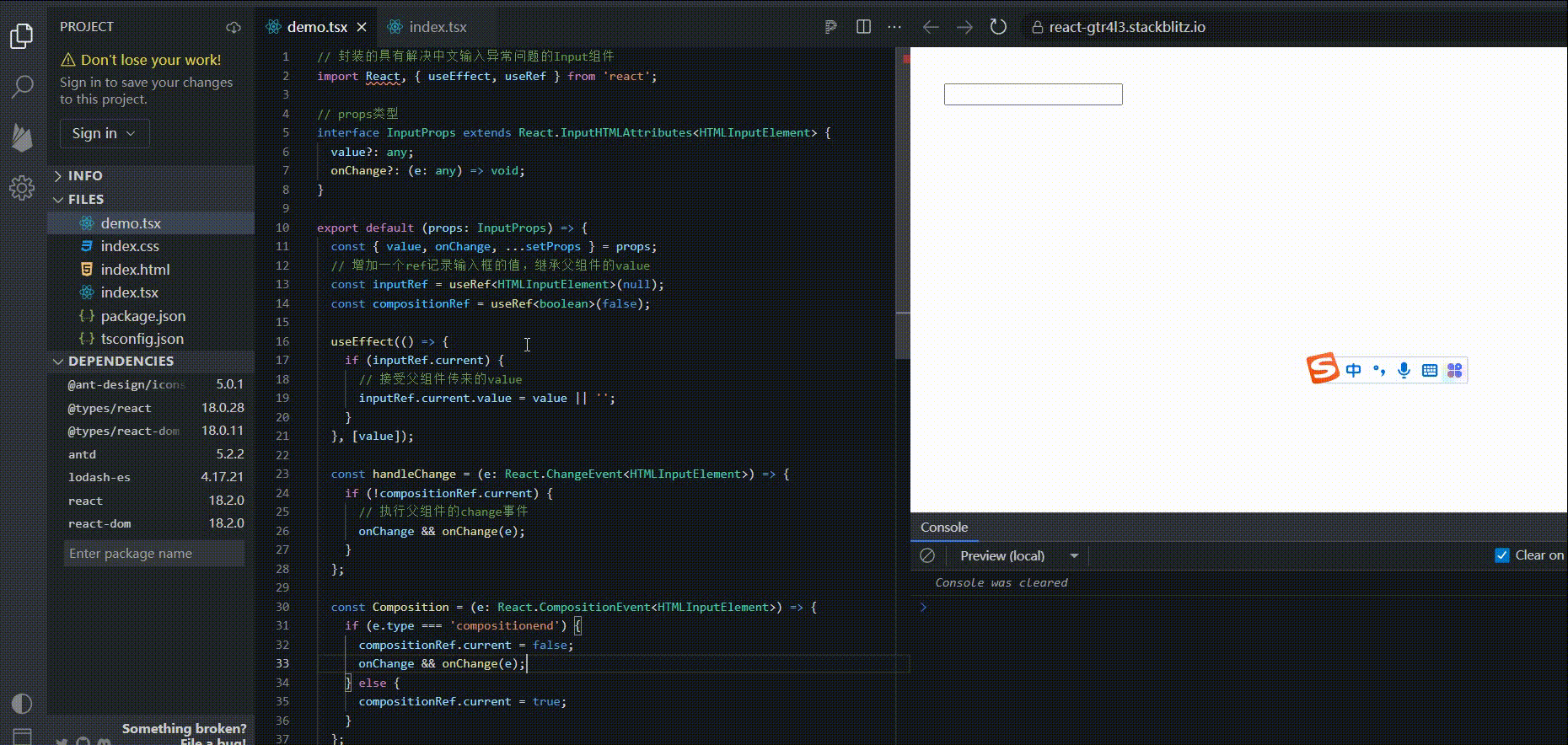
每天进步一点点,成长足迹看得见。