银行家算法
1.运行环境:visual studio 2015
2.实验要求如下:
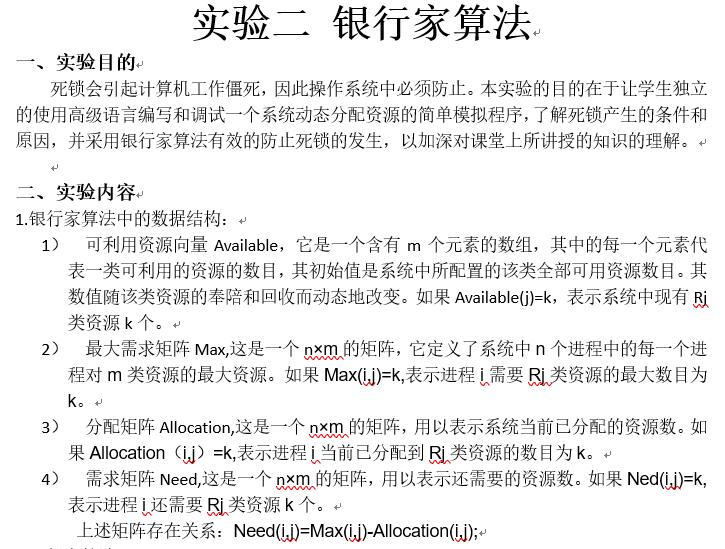
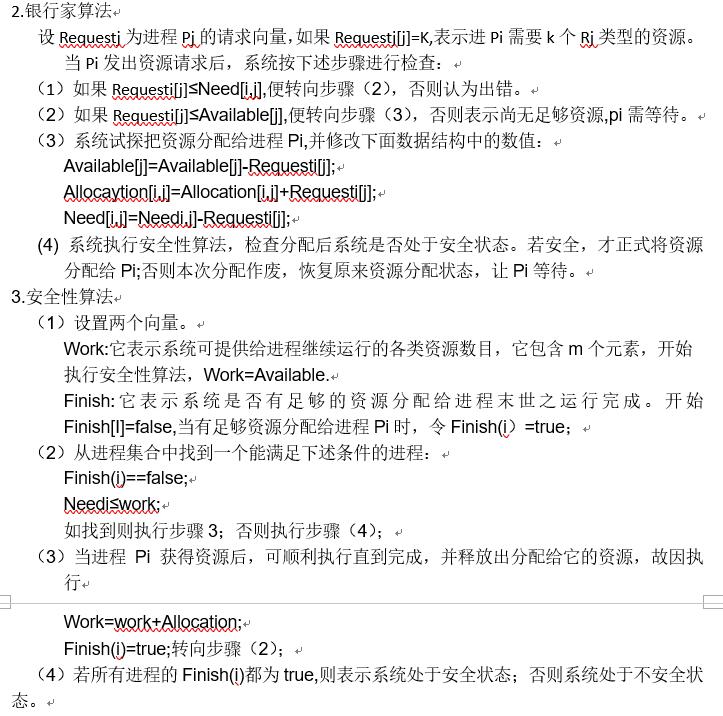
主函数
//******************************
//主函数。
// YinHangJia.cpp : 定义控制台应用程序的入口点。
//
#include "stdafx.h"
#include"ZiYuan.h"
#include"MaxNM.h"
#include<iostream>
using namespace std;
ZiYuan ziyuan;//电脑剩余可分配的资源
MaxNM JinCheng;//进程所需的资源和总需求资源。
int book[30];//标记
int n;//进程数量
int buzhou[30];
void dfs(int step)
{
if (JinCheng.isNeedFull())
{
cout << "**** 进程调度的方法: ";
for (int i = 0; i < JinCheng.GetN(); i++)
{
cout << buzhou[i]<<" ";
}
cout << endl;
return;
}
for (int i = 0; i < JinCheng.GetN(); i++)
{
if (book[i] == 0 && (!JinCheng.isPosNeedFull(i))&&JinCheng.IsPosSafe(i,ziyuan))
{
book[i] = step;
buzhou[step - 1] = i;
JinCheng.AllocationPosToOs(i,ziyuan);
dfs(step + 1);
book[i] = 0;
//JinCheng.AutoSetPosNeedData(i);
JinCheng.OsPosToAllocation(i, ziyuan);
}
}
}
int main()
{
/*
**输入资源数 m 以及 系统各类资源总数。
*/
for (int i = 0; i < 30; i++)
{
book[i] = 0;
}
int m;
std::cout << "输入设备类别数:_________\b\b\b\b";
std::cin >> m;
ziyuan.SetLen(m);
ziyuan.ZiYuanStart();
/*
**输入进程数 n。
*/
std::cout << "输入模拟的进程数量:__________\b\b\b\b\b";
std::cin >> n;
JinCheng.SetN(n);
JinCheng.SetM(m);
/*
**输入最大需求数。
**如果超出系统剩余则 提示错误,重新输入。
*/
loop1:
cin >> JinCheng;
if (JinCheng > ziyuan)
{
cout << "资源不够。输入太大。再输入一次。" << endl;
goto loop1;
}
/*
**初始化系统剩余数量。
*/
cin >> ziyuan;
/*
**输入每个进程 还需要的设备数。
**自动计算出 已经分配的设备数。
*/
JinCheng.StartNeedData();
JinCheng.AutoSetAllocation();
/*
**深搜出所有可能情况。
**并输出。
*/
dfs(1);
cout << endl<<endl<<"**************************" << endl;
cout << "模拟结束,给个高分吧" << endl;
cout << "开发人员列表:" << endl;
cout << "资源类: 姜 昊 152210708315" << endl;
cout << "MaxNM类: 吴陈杰 152210708327" << endl;
cout << " 主函数:吴海波 152210708328" << endl;
cout << "程序调试:王子源 152210708117" << endl;
cout << "**************************" << endl;
getchar();
getchar();
return 0;
}
资源类 ZiYuan .h 文件
//*****************************
//系统中资源类 头文件。
//系统中 总计剩余的资源
#pragma once
#include<iostream>
#include<ostream>
#include<stdarg.h>
using namespace std;
class ZiYuan
{
public:
ZiYuan();
ZiYuan(int Len, ...);//初始化。
~ZiYuan();
private:
int Len;
int SheBei[30];
public:
int ZiYuanStart();//不断输入设备,再返回 Len。
int SetLen(int Len);//改变 Len。
void SetAll(int Len, ...);//改变所有的 设备数量
void ZenAll(int len, ...);//增加所有的 设备数量
int SetSheBei(int pos,int num);//改变 pos 个设备的数
int GetLen();//返回 Len
int GetSheBeiPos(int pos);//返回 pos 个设备的数量。
int ZenJiaPosX(int pos,int x);// 第 pos 个设备数 + x
friend ostream& operator << (ostream & os, const ZiYuan & data);//输出。
friend istream& operator>>(istream &in, const ZiYuan & data);
};
inline ostream & operator << (ostream & os, ZiYuan & data)
{
char ch = 'A';
for (int i = 0; i < data.GetLen(); i++)
{
os << "设备 "<<ch++<<" : "<<data.GetSheBeiPos(i) << " ";
if ((i+1) % 5 == 0)
{
os << endl;
}
}
os << endl;
return os;
}
inline istream& operator>>(istream &in,ZiYuan & data)
{
char ch = 'A';
int num;
for (int i = 0; i < data.GetLen(); i++)
{
std::cout << "设置设备 " << ch++ << " 的剩余量为:____\b\b\b ";
std::cin >> num;
data.SetSheBei(i, num);
}
cout << endl;
return in;
}
资源类 ZiYuan .CPP 文件
//******************************
//系统剩余资源类 cpp。
#include "stdafx.h"
#include "ZiYuan.h"
ZiYuan::ZiYuan()
{
Len = 0;
}
ZiYuan::ZiYuan(int Len,...)
{
this->Len = Len;
va_list ap;
va_start(ap, Len);
for (int i = 0; i < Len; i++)
{
this->SheBei[i] = va_arg(ap, int);
}
va_end(ap);
}
ZiYuan::~ZiYuan()
{
}
int ZiYuan::ZiYuanStart()
{
char ch = 'A';
cout << "系统剩余设备的初始化:" << endl;
for (int i = 0; i < Len; i++)
{
cout << "输入设备 " << ch++ << "的个数:______\b\b\b\b"<<endl;
cin >> SheBei[i];
}
return Len;
}
int ZiYuan::SetLen(int Len)
{
this->Len = Len;
return Len;
}
void ZiYuan::SetAll(int Len, ...)
{
va_list ap;
va_start(ap,Len);
for (int i = 0; i < Len; i++)
{
this->SheBei[i] = va_arg(ap, int);
}
va_end(ap);
}
void ZiYuan::ZenAll(int len, ...)
{
va_list ap;
va_start(ap, Len);
for (int i = 0; i < Len; i++)
{
this->SheBei[i] += (va_arg(ap, int));
}
va_end(ap);
}
int ZiYuan::SetSheBei(int pos,int num)
{
this->SheBei[pos] = num;
return num;
}
int ZiYuan::GetLen()
{
return Len;
}
int ZiYuan::GetSheBeiPos(int pos)
{
return SheBei[pos];
}
int ZiYuan::ZenJiaPosX(int pos, int x)
{
this->SheBei[pos] += x;
return this->SheBei[pos];
}
MaxNM .h 文件
//*****************************************
//need max 等矩阵的头文件
// 每个进程 需要的总资源
#pragma once
#include<iostream>
#include<ostream>
#include<istream>
#include"ZiYuan.h"
using namespace std;
//class ZiYuan;
class MaxNM
{
public:
MaxNM();
MaxNM(int n,int m);//n 个进程,每个有 m 个设备。
~MaxNM();
private:
int MaxN;
int MaxM;
int data[30][30];//每个进程需要的总资源。
int NeedData[30][30];//每个进程 还需要的资源
int Allocation[30][30];//当前已经分配的资源。
public:
int SetN(int n);//改变 进程数量为 n
int SetM(int m);//每个进程有 m 个设备。
int GetN();
int GetM();
int Setdata(int x, int y,int num);//设置 第 x 个进程 的第 y 个设备为 num。
int ZendataX(int x, int y, int num);//增加 第 x 个进程 的第 y 个设备的总需求数量。
int Getdata(int x, int y);//返回 第 x 个进程 的第 y 个设备总需求数量。
friend istream& operator >> (istream &os, MaxNM &a);
friend ostream& operator << (ostream &os, MaxNM &a);
bool operator<(ZiYuan a);//是否小于系统能分配的资源数量。
bool operator>(ZiYuan a);
int SetNeedData(int x, int y, int num);
int ZenNeedDataX(int x, int y, int num);
int GetNeedData(int x, int y);
void AutoSetPosNeedData(int pos);
void StartNeedData();//输入设备还需要的数量。
void printNeedData();//打印 debug。
bool isNeedFull();//所有 还需要的数 都为 0 则 true。
bool isPosNeedFull(int pos);//第 pos 个进程所需 设备都为 0 则 true。
int SetAllocation(int x, int y, int num);
int ZenAllocationX(int x, int y, int num);
int GetAllocation(int x, int y);
void AutoSetAllocation();//自动根据 NeedData 和 Data 填充 allocation。
void AllocationToOs(ZiYuan &a);
void AllocationPosToOs(int pos, ZiYuan &a);
void OsPosToAllocation(int pos, ZiYuan &a);
bool IsAllSafe(ZiYuan a);//所有进程是否安全。
bool IsPosSafe(int pos, ZiYuan a);//第pos个进程是否安全
};
inline istream& operator >> (istream &os, MaxNM &a)
{
for (int i = 0; i < a.MaxN; i++)
{
cout << "输入进程:" << i << " 总计需要的 "<<a.MaxM<<"个设备"<<endl;
for (int j = 0; j < a.MaxM; j++)
{
os >> a.data[i][j];
}
}
return os;
}
inline ostream& operator << (ostream &os, MaxNM &a)
{
os << "****************************" << endl;
for (int i = 0; i < a.MaxN; i++)
{
os << "进程 " << i << ": ";
char ch = 'A';
for (int j = 0; j < a.MaxM; j++)
{
os << " 设备"<<ch++<<" "<<a.data[i][j];
}
os << endl;
}
os << "****************************" << endl;
return os;
}
MaxNM .CPP 文件
//************************************
//need max 等矩阵的cpp
#include "stdafx.h"
#include "MaxNM.h"
MaxNM::MaxNM()
{
}
MaxNM::MaxNM(int n, int m)
{
this->MaxN = n;
this->MaxM = m;
}
MaxNM::~MaxNM()
{
}
int MaxNM::SetN(int n)
{
this->MaxN = n;
return n;
}
int MaxNM::SetM(int m)
{
this->MaxM = m;
return m;
}
int MaxNM::GetN()
{
return this->MaxN;
}
int MaxNM::GetM()
{
return this->MaxM;
}
int MaxNM::Setdata(int x, int y,int num)
{
this->data[x][y] = num;
return num;
}
int MaxNM::ZendataX(int x, int y, int num)
{
this->data[x][y] += num;
return this->data[x][y];
}
int MaxNM::Getdata(int x, int y)
{
return this->data[x][y];
}
bool MaxNM::operator<(ZiYuan a)
{
for (int i = 0; i < this->MaxN; i++)
{
for (int j = 0; j < MaxM; j++)
{
if (this->data[i][j] > a.GetSheBeiPos(j))
{
return false;
}
}
}
return true;
}
bool MaxNM::operator>(ZiYuan a)
{
for (int i = 0; i < this->MaxN; i++)
{
for (int j = 0; j < MaxM; j++)
{
if (this->data[i][j] > a.GetSheBeiPos(j))
{
return true;
}
}
}
return false;
}
int MaxNM::SetNeedData(int x, int y, int num)
{
NeedData[x][y] = num;
return num;
}
int MaxNM::ZenNeedDataX(int x, int y, int num)
{
NeedData[x][y] += num;
return NeedData[x][y];
}
int MaxNM::GetNeedData(int x, int y)
{
return NeedData[x][y];
}
void MaxNM::AutoSetPosNeedData(int pos)
{
for (int i = 0; i < this->MaxM; i++)
{
this->NeedData[pos][i] = this->data[pos][i] - this->Allocation[pos][i];
}
}
void MaxNM::StartNeedData()
{
cout << "初始化,进程还需要的设备数" << endl;
for (int i = 0; i < this->MaxN; i++)
{
cout << "初始化进程 " << i <<endl;
char ch = 'A';
for (int j = 0; j < this->MaxM; j++)
{
cout << "输入设备" << ch++ << "还需要的个数:_____\b\b\b";
cin >> this->NeedData[i][j];
}
cout << endl;
}
}
void MaxNM::printNeedData()
{
cout << "进程还需要的设备数:" << endl;
for (int i = 0; i < this->MaxN; i++)
{
cout << "进程 " << i <<": "<< endl;
char ch = 'A';
for (int j = 0; j < this->MaxM; j++)
{
cout << "设备" << ch++ << "还需要 ";
cout << this->NeedData[i][j] << " ";
}
cout << endl;
}
}
bool MaxNM::isNeedFull()
{
for (int i = 0; i < this->MaxN; i++)
{
for (int j = 0; j < this->MaxN; j++)
{
if (this->NeedData[i][j] > 0)
{
return false;
}
}
}
return true;
}
bool MaxNM::isPosNeedFull(int pos)
{
for (int i = 0; i < this->MaxM; i++)
{
if (NeedData[pos][i] > 0)
{
return false;
}
}
return true;
}
int MaxNM::SetAllocation(int x, int y, int num)
{
Allocation[x][y] = num;
return num;
}
int MaxNM::ZenAllocationX(int x, int y, int num)
{
Allocation[x][y] += num;
return Allocation[x][y];
}
int MaxNM::GetAllocation(int x, int y)
{
return Allocation[x][y];
}
void MaxNM::AutoSetAllocation()
{
for (int i = 0; i < this->MaxN; i++)
{
for (int j = 0; j < this->MaxM; j++)
{
int x=this->Allocation[i][j] = this->data[i][j] - NeedData[i][j];
}
}
}
void MaxNM::AllocationToOs(ZiYuan &a)
{
for (int i = 0; i < this->MaxN; i++)
{
for (int j = 0; j < this->MaxM; j++)
{
this->NeedData[i][j] = 0;
a.ZenJiaPosX(j,this->Allocation[i][j]);
}
}
}
void MaxNM::AllocationPosToOs(int pos,ZiYuan & a)
{
for (int j = 0; j < this->MaxM; j++)
{
this->NeedData[pos][j] = 0;
a.ZenJiaPosX(j, this->Allocation[pos][j]);
}
}
void MaxNM::OsPosToAllocation(int pos, ZiYuan & a)
{
for (int j = 0; j < this->MaxM; j++)
{
this->NeedData[pos][j] = this->data[pos][j] - this->Allocation[pos][j];
a.ZenJiaPosX(j, -(this->Allocation[pos][j]));
}
}
bool MaxNM::IsAllSafe(ZiYuan a)
{
for (int i = 0; i < this->MaxN; i++)
{
for (int j = 0; j <this->MaxM; j++)
{
if (this->NeedData[i][j] > a.GetSheBeiPos(j))
{
return false;
}
}
}
return true;
}
bool MaxNM::IsPosSafe(int pos, ZiYuan a)
{
for (int i = 0; i < this->MaxM; i++)
{
if (this->NeedData[pos][i] > a.GetSheBeiPos(i))
{
return false;
}
}
return true;
}