Queue<T>类
表示对象的先进先出集合。
队列在按接收顺序存储消息方面非常有用,以便于进行顺序处理。 存储在 Queue,<T> 中的对象在一端插入,从另一端移除。
Queue<T> 的容量是 Queue<T> 可以包含的元素数。 当向 Queue<T> 中添加元素时,将通过重新分配内部数组来根据需要自动增大容量。
可通过调用 TrimExcess 来减少容量。
Queue<T> 接受 null 作为引用类型的有效值并且允许有重复的元素。
命名控件:System.Collections.Generic
程序集:System(在System.dll中)
语法:public class Queue<T>:IEnumerable<T>, ICollection, IEnumerable
List<T>实现了IList<T>、 ICollection<T>、IEnumerable<T>、IList、ICollection、IEnumerable接口
因此可以看出与List<T>相比:
Queue<T>没有继承ICollection<T>接口,因为这个接口定义的Add()和Remove()方法不能用于队列;
Queue<T>没有继承IList<T>接口,所以不能使用索引器访问队列。
所以队列只允许在队列的尾部添加元素,从队列的头部获取元素。
常用的Queue<T>类的成员:
Count : 返回队列中元素的个数。
C#中Queue<T>类的使用以及部分方法的源码分析
最新推荐文章于 2024-08-20 15:24:31 发布
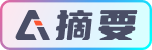