xamarin ios
为您的应用程序打开机遇之门 (OPENS A WORLD OF OPPORTUNITIES FOR YOUR APPS)
There have been questions on StackOverflow asking how to use Google Sheets to store data using Xamarin, as a quick and simple database. After finding no articles online, I decided to spend a few hours to figure it out. Using the steps below, you can use a Restful POST API call to send data from Xamarin iOS & Android apps, to instantly populate rows in a Google Sheet.
Ť这里一直在计算器上的问题询问如何使用谷歌表使用Xamarin存储数据,作为一个快速和简单的数据库。 在网上找不到任何文章后,我决定花几个小时弄清楚。 使用以下步骤,您可以使用Restful POST API调用从Xamarin iOS和Android应用程序发送数据,以立即填充Google表格中的行。
介绍 (Introduction)
We are going to demonstrate adding rows to a Google Sheet using a Feedback form to collect user feedback. Why did I choose this specific use case? If you are an Indie mobile developer, you probably know that collecting feedback can be important, so if you know a user is not satisfied with the app, you can redirect the user to this Feedback page instead of the Play/App Store. Note that this procedure doesn’t implement any authentication, so it cannot replace a backend, and I would also not recommend using this in production apps that require security.
我们将演示如何使用“反馈”表单将行添加到Google表格中,以收集用户反馈。 为什么选择此特定用例? 如果您是独立移动开发人员,则可能知道收集反馈很重要,因此,如果您知道用户对应用程序不满意,则可以将用户重定向到此“反馈”页面,而不是Play / App Store。 请注意,此过程不执行任何身份验证,因此它不能替换后端,我也不建议在需要安全性的生产应用程序中使用此过程。
What do you need to follow this? You just need Visual Studio with Xamarin installed on your Windows or Mac computer, and a Google Drive account, both of which are free. A simple understanding of C# and Javascript will help you understand this short tutorial.
您需要遵循什么? 您只需要在Windows或Mac计算机上安装了Xamarin的Visual Studio ,以及一个Google Drive帐户,这两个都是免费的。 对C#和Javascript的简单理解将帮助您理解此简短教程。
入门 (Getting Started)
There are three different parts:
分为三个部分:
- Create the Google Sheet that acts as your database 创建充当数据库的Google表格
- Add a script to the sheet that acts as a web services layer 将脚本添加到用作Web服务层的工作表中
- Code the front-end Xamarin iOS & Android apps 编码前端Xamarin iOS和Android应用程序
创建Google表格 (Creating the Google Sheet)

- Go to Google Drive and simply create a new Sheet with any Title, and populate the names of the columns in the first row as Name, Email, Phone & Feedback. 转到Google云端硬盘,只需创建一个带有任何标题的新工作表,然后在第一行中填充各列的名称,即名称,电子邮件,电话和反馈。
Note down the
SheetID
from the url of that webpage, 1ok…ZgQ in my case.- Then in the Google sheet toolbar, select “Tools”->“Script Editor”, to automatically create a Google Apps Script file that’s associated with your Google Sheet. 然后在Google工作表工具栏中,选择“工具”->“脚本编辑器”,以自动创建与您的Google工作表关联的Google Apps脚本文件。
Google App脚本 (Google App Script)
In the created GS file, paste in the code below, replacing the “{
SheetID
}” with the value from above, so “1ok…ZgQ” in my case.在创建的GS文件中,粘贴下面的代码,用上面的值替换“ {
SheetID
}”,在我的情况下为“ 1ok…ZgQ”。
The script above parses a POST request and appends the correct data into your Google sheet, into corresponding columns based on index. It also provides response content that can be read by the app.
上面的脚本会解析POST请求, 并将正确的数据附加到您的Google表格中,并根据索引添加到相应的列中。 它还提供了可由应用程序读取的响应内容。
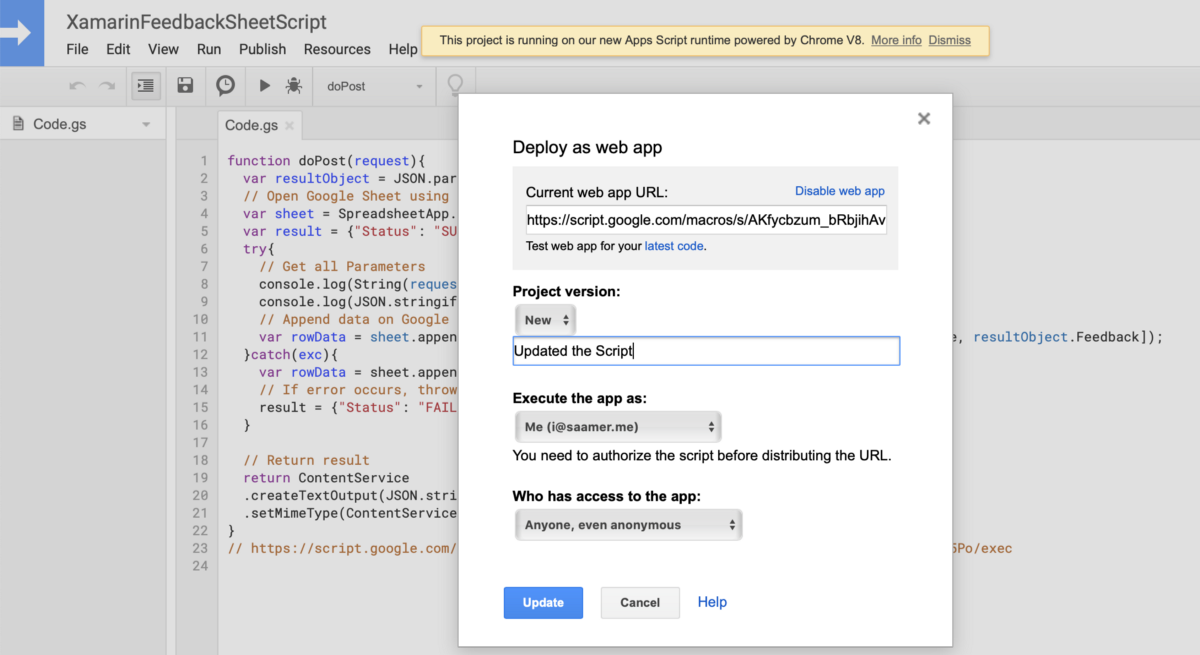
- After you paste the code, give the App Script file a name, then tap on “Publish”-> “Deploy as web app” and in the pop-up window, set access to “Anyone, even anonymous” and press “Deploy”. 粘贴代码后,为App Script文件命名,然后点击“发布”->“作为Web应用程序部署”,然后在弹出窗口中将访问权限设置为“任何人,甚至匿名”,然后按“部署” 。
Note down the
WebAppUrl
generated for your apps, so in my case: https://scr…/exec记下为您的应用程序生成的
WebAppUrl
,因此在我的情况下: https:// scr…/ exec- Note: Always set Project version to “New” when updating the script. 注意:更新脚本时,请始终将项目版本设置为“新建”。
编写应用程式 (Coding the app)
We will create the Feedback page UI from scratch and create models to handle the data transfer. Here’s a quick rundown of the code (stored here):
我们将从头开始创建“反馈”页面UI,并创建模型来处理数据传输。 这是代码的简短摘要( 存储在此处 ):

- Open Visual Studio and Create a new solution from a “Blank Forms App” template, targeting iOS & Android platforms. 打开Visual Studio并从“空白表单应用”模板创建一个针对iOS和Android平台的新解决方案。
Update the
MainPage.xaml
file with the code here, to add some entries to the view that accept data, and a Submit button. Add names to the controls so they can be referenced by the code behind file/partial class (MainPage.xaml.cs
), and assign theSubmitButton_Pressed
function to thePressed
event of the button.使用此处的代码更新
MainPage.xaml
文件,向视图中添加一些接受数据的条目 ,以及一个Submit 按钮 。 将名称添加到控件,以便文件/部分类(MainPage.xaml.cs
)后面的代码可以引用它们,并将SubmitButton_Pressed
函数分配给按钮的Pressed
事件。Create a C# class
FeedbackModel.cs
as shown here, with the Name, Email, Phone & Feedback properties that you want to send to the backend. TheFeedbackModel
is used to store data from the entries, and then convert the data into aJSON
string for sending the request content to the Google Apps Script created above.创建一个C#类
FeedbackModel.cs
如图所示这里 ,与你要发送到后端的名称,电子邮件,电话和反馈性。FeedbackModel
用于存储条目中的数据,然后将数据转换为JSON
字符串,用于将请求内容发送到上面创建的Google Apps脚本。Create another class
ResponseModel.cs
as shown here, which will be used to parse and store the response content from the success or failure of the POST API call.创建另一个类
ResponseModel.cs
如图所示这里 ,将被用于分析和存储从POST API调用的成功或失败的响应内容。Finally, in the
MainPage.xaml.cs
file shown here, create theSubmitButton_Pressed
event handler function that makes the POST call to your deployed web service, replacing the “{WebAppUrl
}” with the value from above, so “https://scr…/exec” in my case. You will need to add “Newtonsoft.Json” as a Nuget package for this to work, in order to convert the C# data into JSON format strings and vice-versa:最后,在此处显示的
MainPage.xaml.cs
文件中 ,创建SubmitButton_Pressed
事件处理函数,该函数将对已部署的Web服务进行POST调用,并用上方的值替换“ {WebAppUrl
}”,因此将“ https:// scr …/ exec ”。 您需要添加“ Newtonsoft.Json”作为Nuget程序包才能正常工作,以便将C#数据转换为JSON格式的字符串,反之亦然:
- Congratulations, that’s all there is to it! To test this, select the right configuration depending on your platform and press the play button to build the app on iOS or Android. Once you fill out the form in the mobile app, and press the Submit button, you will notice that the Google Sheet instantly populates with the Feedback data. 恭喜,这就是全部! 要进行测试,请根据您的平台选择正确的配置,然后按播放按钮以在iOS或Android上构建应用。 在移动应用中填写表格并按Submit(提交)按钮后,您会注意到Google表格会立即填充“反馈”数据。

To figure this out with Xamarin, I used this article that teaches using Flutter with Google Sheets, so thank you Shreyas Patil. If you would like to see how to do a GET REST API call to retrieve a list of values or if you have any questions, message me or email me. I love to hear your feedback.
为了通过Xamarin弄清楚这一点,我使用了这篇文章 , 该文章讲授了如何将Flutter与Google Sheets结合使用,因此感谢Shreyas Patil 。 如果您想了解如何执行GET REST API调用以检索值列表,或者有任何疑问,请给我发电子邮件或给我发电子邮件 。 我很高兴听到您的反馈。
翻译自: https://medium.com/the-kickstarter/storedataingooglesheetsusingxamarin-ed8ba5c4b1bc
xamarin ios