表格验证
In this article, we are going to look at how we can make a form and validate it in Swift.
在本文中,我们将研究如何制作表格并在Swift中进行验证。
IECSE速成课程:Swift开发 (IECSE Crash Course: Development with Swift)
This article is going to be the seventh article of the series IECSE Crash Course: Development with Swift. Through the course of 10 articles, we are going to make a small app which will display a set of users that we will fetch from the net. While making the app, we will cover some of the most important topics that every iOS developer must know.
本文将成为IECSE速成课程系列:用Swift开发的第七篇文章。 在完成10篇文章的过程中,我们将制作一个小型应用程序,该程序将显示一组将从网上获取的用户。 制作应用程序时,我们将介绍每个iOS开发人员必须知道的一些最重要的主题。
If you haven’t been following the whole course, I’ll request you to go through and clone this repository as we are going to start from here.
如果您还没有完成整个课程,那么我们将要求您仔细阅读并克隆此存储库,因为我们将从此处开始。
Up till now, we have already presented the viewController in a navigationController. We’ll make the form in this viewController. So now, let’s have a look at how we are going to make the form!
到现在为止,我们已经提出了一个navigationController的的viewController。 我们将在此viewController中创建表单。 现在,让我们来看看如何制作表格!


In our form, we are going to have 6 textfields and are going to make a User object when submit button is pressed.
在我们的表单中,我们将有6个文本字段,并在按下Submit按钮时创建一个User对象。
First, let’s make all the elements that we are going to need.
首先,让我们确定我们需要的所有元素。
One label that tells the user what the form is about.
一个标签 ,告诉用户表单的内容。
Six TextFields, one for each field for our User.
六个TextField ,对于我们的用户,每个字段一个。
One submit button.
一个提交按钮 。
Now that we know what elements our ViewController is going to have embedded in it, let’s start making the ViewController. We’ll write this code in the SampleScreen class.
现在我们知道我们的ViewController将嵌入哪些元素,让我们开始制作ViewController。 我们将在SampleScreen类中编写此代码。

In the above lines, we make a label that will work as a title to our viewController.
在上述各行中,我们制作了一个标签,将其用作viewController的标题。
Before we start making the textfields, let’s take a look at the class UITextField. All the textfields that we are going to make are going to be objects of this class. Also, iOS allows us to configure and make several changes to our textfields. For this article, we are going to make one global function that will be responsible for configuring the textField.
在开始创建文本字段之前,让我们看一下UITextField类。 我们将要创建的所有文本字段都将是此类的对象。 此外,iOS允许我们配置文本字段并对其进行一些更改。 对于本文,我们将创建一个全局函数,该全局函数将负责配置textField。
Let’s first look at the function and then try and understand the lines of code in the function. This function will go outside our SampleScreen class.
首先让我们看一下函数,然后尝试了解函数中的代码行。 此函数将在我们的SampleScreen类之外。

The function setUpTextField requires 2 parameters, one is the textField that we want to configure and the other is the placeholder that we want to represent in our textField. Let's try and understand the code line by line. As we can see, most of the instance properties are self-explanatory, I’ll only be explaining certain properties that are important and a little difficult to understand.
函数setUpTextField需要2个参数,一个是我们要配置的textField,另一个是我们要在textField中表示的占位符。 让我们尝试逐行理解代码。 如我们所见,大多数实例属性都是不言自明的,我仅在解释某些重要且难以理解的属性。
textField.translatesAutoresizingMaskIntoConstraints = false
, like we have also done previously, this is done so that we can manually set Anchor Constraints for our textField.正如我们之前所做的一样,
textField.translatesAutoresizingMaskIntoConstraints = false
这样做是为了让我们可以手动为textField设置锚定约束。textField.placeholder = placeholder
, this is the line where we configure what the placeholder should be for our textField. This placeHolder is one of the parameters that we received in the function.textField.placeholder = placeholder
,这是我们为textField配置占位符的行。 这个placeHolder是我们在函数中收到的参数之一。Sometimes, when we tap on a textField, Phone Number, for example, you might want the keyboard that appears to be numeric.
textField.keyboardType
, is where we define the type of keys that you want to appear on the keyboard by default when the user clicks on a textField.有时,当我们点击一个文本字段(例如“电话号码”)时,您可能希望键盘看起来是数字的。
textField.keyboardType
,是我们定义您要在用户单击textField时默认显示在键盘上的键的类型的地方。returnKeyType
is the instance property that sets up the title of the return key on the keyboard.returnKeyType
是用于在键盘上设置返回键标题的instance属性。- If we want to change the colour of the textField, the syntax of attributedPlaceholder is how that is done. 如果我们要更改textField的颜色,则attributedPlaceholder的语法是如何实现的。
- If we want to change the colour of the border of the textField, it can only be done when you give your border some width. 如果我们要更改textField边框的颜色,则只有在给边框提供一定宽度时才能完成。
Now that we have a function that configures our textField, let’s make 6 instances for the textFields that we are going to need in our ViewController.
现在我们有了一个配置textField的函数,让我们为ViewController中需要的textField创建6个实例。

Here, for every textfield that we instantiate, we call the setUpTextField
function with the placeholder that we want the textfield to have.
在这里,对于我们实例化的每个文本字段,我们都调用setUpTextField
函数,并使用我们希望文本字段具有的占位符。
Next, we add the submit button, which will be an object of UIButton.
接下来,我们添加Submit按钮,它将是UIButton的对象。

Most of the code is responsible for configuring the appearance of the button. The line that interests us most is button.addTarget(..)
, which tells the button what function to call when it is pressed.
大多数代码负责配置按钮的外观。 我们最感兴趣的行是button.addTarget(..)
,它告诉按钮在按下时要调用哪个函数。
The syntax for this is,
语法是
button.addTarget(self, action: #selector(<functionToBeCalled>),for: .touchUpInside)
button.addTarget(self, action: #selector(<functionToBeCalled>),for: .touchUpInside)
All the functions that you call from a button need to be exposed to Objective-C, that is what @objc
does. For now, we are going to leave this function empty.
您从按钮调用的所有功能都必须公开给Objective-C, @objc
就是@objc
做的。 现在,我们将使此功能为空。
Once we are done with all this, we need to add these elements that we have instantiated into our SampleController. So as you might have guessed we do this in the function viewDidLoad(). We are going to set the elements up in our ViewController depending on how we want our view to look.
完成所有这些操作后,我们需要将已实例化的这些元素添加到SampleController中。 因此,您可能已经猜到了,我们在函数viewDidLoad()中进行了此操作。 我们将根据我们希望视图的外观在ViewController中设置元素。

Once, we add all our elements to the SampleController, we call the function setUpView(). It’ll be in this function that we will set up constraints for all the elements.
一次,我们将所有元素添加到SampleController中,我们调用函数setUpView()。 正是在这个函数中,我们将为所有元素设置约束。
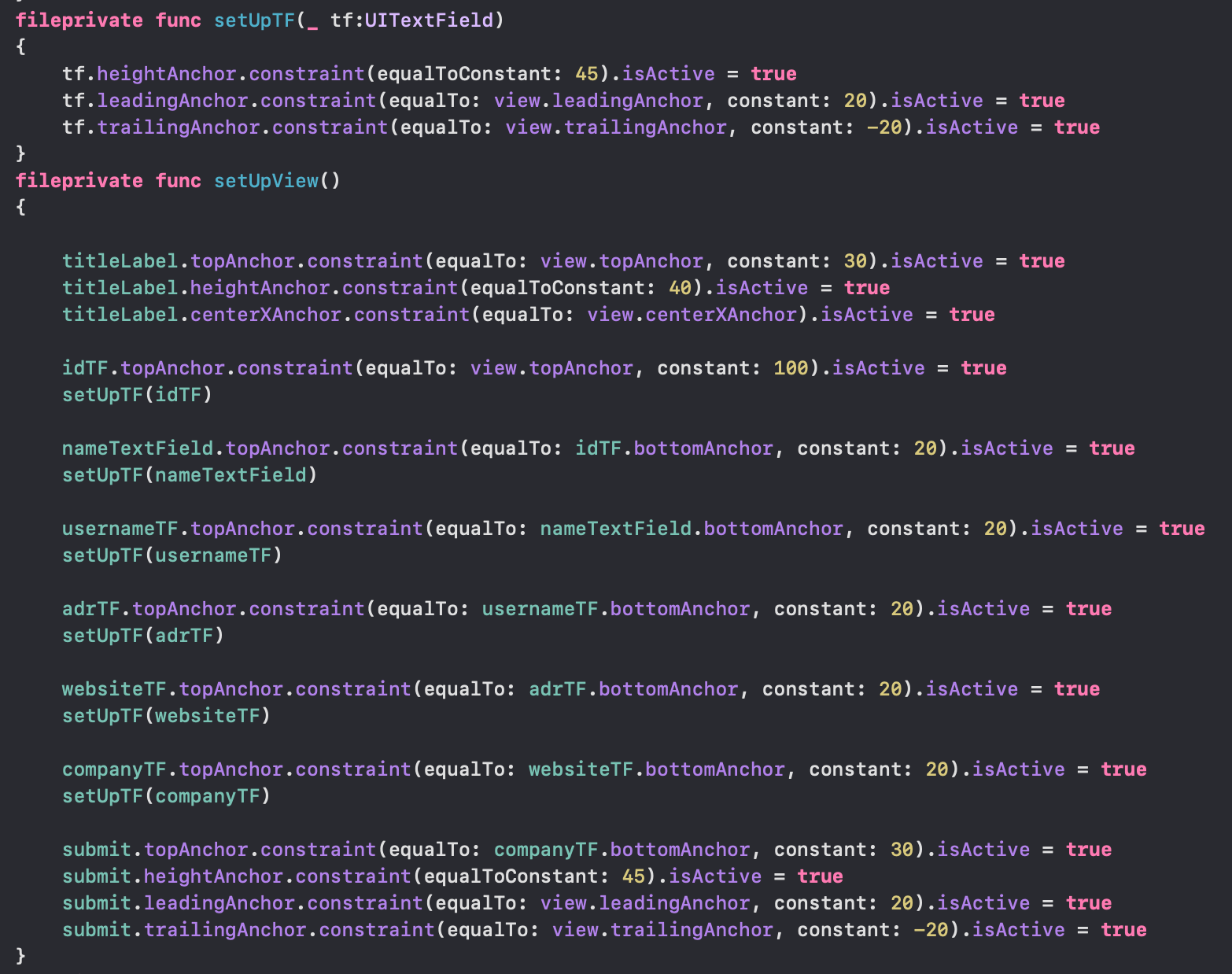
Here, we have made an additional function setUpTF(_ tf:UITextField)
, so that we can avoid a little redundancy as many constraints for all the textFields are going to be same to maintain uniformity.
在这里,我们做了一个额外的函数setUpTF(_ tf:UITextField)
,所以我们可以避免一点冗余,因为所有textField的许多约束都将相同,以保持一致性。
If you have doubts about the Auto Layout and constraints, I suggest that you go check out this article.
如果您有关于自动布局和约束的疑虑,我建议你去看看这个文章。
With this step our form is ready and all that is left is for us to write the function addUser, which we will do in the next section. If you have followed all the steps correctly until now, your SampleScreen looks like this.
通过此步骤,我们的表单已准备就绪,剩下的就是编写功能addUser了,我们将在下一部分中进行操作。 如果到目前为止,您正确地执行了所有步骤,则SampleScreen看起来像这样。

验证方式 (Validation)
When the submit button is pressed, we might want to make sure that all the fields are properly filled so that we have all the required fields to make our object. This is where validation comes in. Let’s have a look at how our addUser function looks.
当按下提交按钮时,我们可能要确保所有字段都被正确填充,以便我们拥有创建对象的所有必填字段。 这就是验证的来源。让我们看一下addUser函数的外观。

First, we make a custom error, this is the error that we are going to throw when the user presses the button without all the fields.
首先,我们犯了一个自定义错误,这是当用户在没有所有字段的情况下按下按钮时将抛出的错误。
重试捕捉 (Do-try-catch)
In Swift, error handling is done with the do-try-catch block to respond to recoverable errors in our code. As per our need, what we want to do is make sure that all the fields are filled before we make a User object. The do-try-catch block can be thought of as an if-else conditional. When we put some statement in do, we try certain things that we know might give an error. When that happens we throw the appropriate error and we leave the do block and enter the catch block, this is where we handle the error appropriately.
在Swift中,错误处理是通过do-try-catch块完成的,以响应代码中的可恢复错误。 根据我们的需要,我们要做的是确保在创建User对象之前,所有字段都已填充。 可以将do-try-catch块视为if-else条件 。 当我们在执行某条语句时,我们尝试某些已知会导致错误的事情。 当发生这种情况时,我们抛出适当的错误,然后离开do块并进入catch块,这就是我们适当处理错误的地方。
The do-try-catch block is preferred because the other alternative would be to force-unwrap the object, but when you do that it means that your app will crash if the unwrapped value is null. Hence, that is only done when you are sure that the value you are unwrapping will not be nil. Avoid force-unwrapping as much as possible.
首选do-try-catch块,因为另一种选择是强制解包对象,但是当您这样做时,这意味着如果解包值为null,则应用程序将崩溃。 因此,只有在确定要解包的值不会为nil时,才执行此操作。 尽可能避免强行展开。
In our code, we also use the guard statement. This can also be imagined like an if-else block. What happens here, is that if the condition after the guard statement is evaluated to false, the else block is invoked. One thing to note when using guard, is that it can only be used to “transfer program control out of scope”. This is a fancy way of saying that the body of the guard block, i.e. what’s inside the squiggly brackets, needs to exit the function. Hence in the else block, you are only allowed to use the following :
在我们的代码中,我们还使用了guard语句。 这也可以想象成if-else块。 这里发生的是,如果将guard语句之后的条件评估为false,则将调用else块。 使用防护时要注意的一件事是,它只能用于“ 将程序控制超出范围 ”。 这是一种怪异的说法,即保护块的主体(即,弯曲的括号内的内容)需要退出该功能。 因此,在else块中,仅允许您使用以下内容:
return
, to exit a function or closurereturn
,退出函数或闭包break
, to exit a loop likefor
break
,退出就像一个循环for
continue
, to continue to the next loop iterationcontinue
,继续进行下一个循环迭代throw
, to exit a function and throw an error.throw
,退出函数并引发错误。
In our code, what we do is try to unwrap the text in all the textFields one by one, there is a good chance that one of the textFields might be empty and give nil on being unwrapped, this is the case where our else block gets executed. It is this else block that we throw an error.
在我们的代码中,我们要做的是尝试将所有textFields中的文本一一解开,很有可能一个textFields可能为空,并且在解开时给出nil,在这种情况下,else块会得到被执行。 正是这个else块引发了错误。
Once we have taken care of all the fields that we need, ie if we have not entered the else block of the guard statement, we will make our object. So before we can make the User object, we are going to make an object for the city and company of struct Address and Company. We will use these objects to make the object for our new user.
一旦我们处理完所有需要的字段,即,如果我们没有输入guard语句的else块,我们将成为我们的对象。 因此,在创建用户对象之前,我们将为结构地址和公司的城市和公司创建一个对象。 我们将使用这些对象为新用户创建对象。
Next, we reset all the other fields so that new data can be entered.
接下来,我们重置所有其他字段,以便可以输入新数据。
It is in the catch block where we will take care of the case that an error was encountered while unwrapping the values, ie if one of the textFields was empty. Here, we are just going to print the error. But actually, we can also make a pop up that asks the user to fill in the fields properly.
在catch块中,我们将处理以下情况:展开值时遇到错误,即,如果textFields中的一个为空。 在这里,我们将打印错误。 但是实际上,我们还可以弹出一个窗口,要求用户正确填写字段。
结语 (Wrapping up)
In this tutorial, we have seen how we can make forms and validate them. We created an object for user which in the next tutorial we are going to pass to our ViewController and we will add this user to our tableView using Delegates.
在本教程中,我们了解了如何制作表单并验证它们。 我们为用户创建了一个对象,该对象将在下一个教程中传递给ViewController,然后使用Delegates将该用户添加到tableView中。
恭喜🎉! 您已经完成了第七教程! (Congratulations🎉! You have completed the 7th tutorial!!)
All of the above-written code is available on Github. Watch this space for more interesting challenges, up next in this series!
所有上面编写的代码都可以在Github上找到 。 观看此空间,了解更多有趣的挑战,本系列中的下一个!
Confused about something? Let us know in the responses below. 😄
对某事感到困惑? 在下面的回复中告诉我们。 😄
翻译自: https://medium.com/iecse-hashtag/swift-part-7-making-a-form-with-validation-4ee72f321ac4
表格验证