swift 方法模板
When we think about the software development routine, we can quickly identify that many times, the solution to a specific problem has identical characteristics, if not equal to that found in a previously developed project but the solution and the problem had not been documented. So, you are facing a similar problem, that was solved in the past, but there’s no record of how it was solved, making it extremely hard to reuse ideas and solutions. In this way, the occurrence of identical problems that are repeated in other contexts, consume more time and resources then they should, since the solutions were already explored before.
当我们考虑软件开发例程时,我们可以快速地识别出很多次,针对特定问题的解决方案具有相同的特征,即使不等同于先前开发的项目中发现的特征,但是解决方案和问题尚未记录在案。 所以,你都面临类似的问题,这是在过去解决,但是没有它是如何解决的,使它非常难以重用理念和解决方案的纪录。 以这种方式,在其他情况下重复出现的相同问题会比原先应该花费的时间和资源更多,因为以前已经探讨过解决方案。
In this way, Design Pattern are great allies. A Design Pattern can be described as the recurring solution to a problem in a context, even if in different projects and areas. The key terms here are: context, problem and solution. One context concerns the environment, and the circumstances within which element exists. The problem is the undefined issue, something that needs to be investigated and resolved. It is usually tied to the context in which it occurs. Finally, the solution refers to the answer to the problem that helps to solve it.
这样,设计模式就是伟大的盟友。 设计模式可以描述为上下文中问题的重复解决方案,即使在不同的项目和领域中也是如此。 这里的关键术语是: 上下文 , 问题和解决方案 。 一种情况涉及环境和元素存在的环境。 问题是未定义的问题,需要进行调查和解决。 它通常与它发生的上下文相关。 最后,解决方案是指有助于解决问题的答案。
The design patterns are descriptions of communicating objects and classes that are customized to solve a general design problem in a particular context. ― Gamma Erich, Helm Richard, Johnson Ralph, Vlissides John. Design Patterns: Elements of Reusable Object-Oriented Software
设计模式是对通信对象和类的描述,这些对象和类被定制为解决特定上下文中的一般设计问题。 -Gamma Erich,Helm Richard,Johnson Ralph,Vlissides John。 设计模式:可重用的面向对象软件的元素
In this article, I would like to discuss about the Template Method, a Behavioral Pattern.
在本文中,我想讨论有关模板方法(一种行为模式)的问题。
Behavior patterns focus on algorithms and assignment of responsibilities between objects. They describe not only patterns of objects or classes, but also patterns of communication between objects.
行为模式着重于对象之间的算法和职责分配。 它们不仅描述了对象或类的模式,而且还描述了对象之间的通信模式。
模板方法 (Template Method)
A template method defines the steps of an algorithm and allows the redefinition of one or more of these steps by subclasses. In this way, the template method protects the algorithm, the order of execution and provides abstract methods that can be implemented by concrete types.
模板方法定义了算法的步骤,并允许通过子类重新定义这些步骤中的一个或多个。 这样,模板方法可以保护算法,执行顺序并提供可以由具体类型实现的抽象方法。
目的 (Purpose)
- Define an algorithm framework in the form of a method, allowing some steps to be implemented by specific concrete classes. 以方法的形式定义算法框架,从而允许某些步骤由特定的具体类来实现。
- Sub-classes can redefine certain steps of an algorithm without changing its general structure. 子类可以重新定义算法的某些步骤,而无需更改其一般结构。
适用性 (Applicability)
- The Template Method can be used to implement parts of an algorithm once and leave it up to the concrete classes to implement the behavior according to their needs. 模板方法可用于一次实现算法的各个部分,并将其留给具体的类来根据其需求实现行为。
- It can be used when code reusability is desired without losing control of the algorithm. 当需要代码可重用性时,可以使用它,而不会失去对算法的控制。
结构体 (Structure)
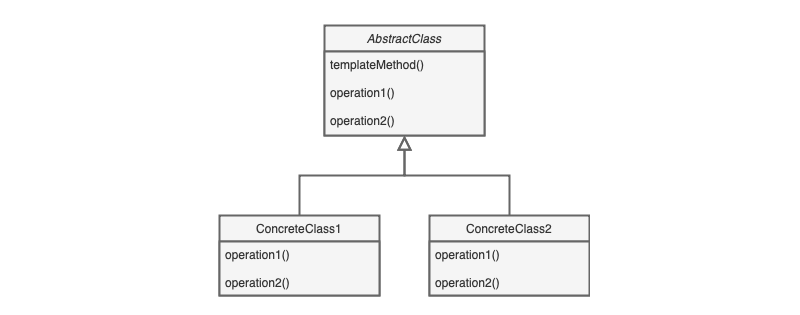
- It presents an abstract class with primitive operations that subclasses define to implement steps of an algorithm. 它提供了一个具有基本操作的抽象类,子类定义了这些操作以实现算法的步骤。
- The abstract class implements a template method defining the skeleton of the algorithm 抽象类实现了定义算法框架的模板方法
- The concrete classes implement the primitive operations to be executed in the template method. 具体的类实现要在模板方法中执行的基本操作。
It’s worth enforcing that `templateMethod()` should be final, and therefore should not be overridden.
值得强调的是,templateMethod()应该是最终的,因此不应被覆盖。
后果 (Consequences)
- Template Method uses inheritance to vary parts of an algorithm. It may become a problem in the future. 模板方法使用继承来改变算法的各个部分。 将来可能会成为问题。
- It can be an important ally in the development of applications, as it provides a way to separate the variable behavior from the invariable behavior of an application. 它可以成为应用程序开发中的重要盟友,因为它提供了将可变行为与应用程序的不变行为分开的方法。
- It also contributes to the implementation of the dependency inversion principle. 它还有助于实现依赖倒置原则。
Swift中的实际示例 (Practical Example in Swift)
When creating the UI of a project programatically, i.e. setting up the interface without storyboards or XIBs, we need to perform a few basic steps, such as:
当以编程方式创建项目的UI时,即设置没有情节提要或XIB的界面时,我们需要执行一些基本步骤,例如:
- Add the sub view to the view. 将子视图添加到视图。
- Setup the constraints. 设置约束。
- Setup additional configuration. 设置其他配置。
So far, pretty simple. But these steps need to be implemented in the correct order. In case you try to run a piece of code that sets constraints to a view that has not been added yet, the application crashes, because there’s no view to apply those constraints to.
到目前为止,非常简单。 但是这些步骤需要以正确的顺序执行。 如果您尝试运行一段将约束设置到尚未添加的视图的代码,则应用程序将崩溃,因为没有视图可将这些约束应用到该视图。
This seems like a good use case for Template Method. So, let’s see how it goes.
这似乎是Template Method的一个很好的用例。 那么,让我们看看它如何进行。
抽象化 (Abstraction)
In Swift, we can create an abstract type to hold a blueprint of methods, and make our classes conform to it. This way we favor composition over inheritance, minimising one of the downsides of this pattern. So, we do that by declaring a Protocol.
在Swift中,我们可以创建一个抽象类型来保存方法的蓝图,并使我们的类与之兼容。 这样,我们更倾向于使用组合而不是继承,从而最大程度地减少了这种模式的缺点之一。 因此,我们通过声明一个协议来做到这一点。
Our protocol holds the three basic steps to configure views programmatically, and a fourth method setupView()
that's going to be out template.
我们的协议包含三个以编程方式配置视图的基本步骤,以及第四个将成为模板的方法setupView()
。
So we have the basic structure, but we don’t have our algorithm yet. In order to implement it, we are going to use Protocol Extensions.
因此,我们有了基本的结构,但是还没有算法。 为了实现它,我们将使用协议扩展。
Protocols can be extended to provide method, initializer, subscript, and computed property implementations to conforming types. This allows you to define behavior on protocols themselves, rather than in each type’s individual conformance or in a global function. — Apple documentation.
可以扩展协议以将方法,初始化程序,下标和计算属性实现提供给符合类型。 这使您可以定义协议本身的行为,而不是定义每种类型的单独一致性或全局函数。 — Apple文档。
This is how our extension will look like:
这是我们的扩展名如下所示:
实作 (Implementation)
Now we created our algorithm, in the classes that implement this protocol, setupView()
is the only method that needs to be called. The other ones will hold the implementation of the algorithm steps, for example:
现在我们创建了算法,在实现该协议的类中, setupView()
是唯一需要调用的方法。 其他将保留算法步骤的实现,例如:
The class conforms to the protocol, and configures the steps for the algorithm. And the only method called is our template method.
该类符合协议,并配置算法的步骤。 唯一调用的方法是我们的模板方法。
结论 (Conclusion)
So, as we could see, the Template Method allows us to approach a common task that needs to be done routinely, and create an abstraction that reinforces the steps to be executed, as well as the order of execution. Besides that, it becomes a standard in the codebase, facilitating the communication with the team and with new developers.
因此,正如我们所看到的,模板方法使我们能够处理需要日常执行的常见任务,并创建一个抽象,以增强要执行的步骤以及执行顺序。 除此之外,它已成为代码库中的标准,从而促进了与团队和新开发人员的沟通。
Originally published at https://vinileal.com on May 22, 2020.
最初于 2020年5月22日 发布在 https://vinileal.com 上。
翻译自: https://medium.com/swlh/design-patterns-in-swift-template-method-925b45218eec
swift 方法模板