flutter自定义单元格
Recently I built a screen with a pretty complex layout containing self sizing cells. In the end it required a UICollectionView
using a custom UICollectionViewLayout
— UICollectionViewFlowLayout
was too simple for the screen design. Parts of this proved to be a real mission to get right, so I thought I’d share my findings in the hope of saving someone else the trouble.
最近,我用复杂的布局构建了一个屏幕,其中包含自定义尺寸的单元格。 最后,它需要一个UICollectionView
使用自定义UICollectionViewLayout
- UICollectionViewFlowLayout
是为屏幕设计过于简单。 事实证明,完成这项工作的一部分是正确的使命,所以我想与大家分享我的发现,以期避免给别人带来麻烦。
This post is split into three parts:
这篇文章分为三个部分:
Part 1 will go over the whole process in detail, starting with the general layout process, then adding self sizing cells to the mix.
第1部分将详细介绍整个过程,从总体布局过程开始,然后向混合中添加自定义大小的单元。
Part 2 will go over some workarounds to common problems and inconsistencies with layouts using self sizing views.
第2部分将使用自定义视图介绍一些解决方法,以解决常见问题和与布局不一致的情况。
Part 3 will optimise this layout with proper use of invalidation contexts and intelligent cache adjustment strategies, for silky smooth scrolling performance.
第3部分将通过正确使用无效上下文和智能缓存调整策略来优化此布局,以实现柔滑的滚动效果。
总体布局过程概述 (Overview of the General Layout Process)
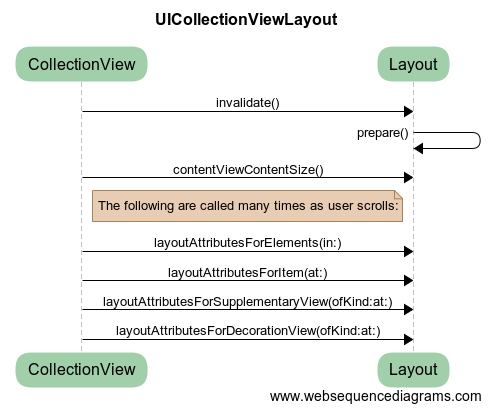
The thing that kicks the whole layout process off is a call to invalidate()
. This can happen for any number of reasons: initial load, view rotation, bounds change, and even directly by you when required. After it is invalidated, we are given an opportunity to prepare new layout data in prepare()
to support the later calls to the layoutAttributesFor...
methods, which are called on the main thread as the user scrolls.
开始整个布局过程的是对invalidate()
的调用。 发生这种情况的原因有很多:初始加载,视图旋转,边界改变,甚至在需要时直接由您自己决定。 无效后,我们有机会在prepare()
准备新的布局数据,以支持以后对layoutAttributesFor...
方法的调用,这些调用在用户滚动时在主线程上调用。
Preparation of new data for this purpose usually means precalculating things like frames, or even constructing whole UICollectionViewAttributes
objects, for all e