lottie 使用
前提条件 (Pre-requisite)
- Install Lottie and Firebase SDK in to your app. 将Lottie和Firebase SDK安装到您的应用程序中。
You need a app registered to your Firebase account, follow this link to setup Firebase and Remote Config in to your iOS project.
您需要向您的Firebase帐户注册一个应用,然后点击此链接将Firebase和Remote Config设置到您的iOS项目中。
Get the URL as shown in screenshot of Lottie file you want to add from the Lottie site, follow this link.
从Lottie网站获取要添加的Lottie文件的屏幕快照中显示的URL,请单击此链接 。
Note: You can also download the Lottie file from the community site and host it on your platform just to be on safer side but for this tutorial I will be using Lottie URL
注意 :您也可以从社区站点下载Lottie文件,并将其托管在您的平台上,只是为了安全起见,但在本教程中,我将使用Lottie URL
Create a Remote Config parameter for your project in this case I named it as lottie_loader_url and add the Lottie URL and save it.
在这种情况下,为您的项目创建一个Remote Config参数,我将其命名为lottie_loader_url并添加Lottie URL并保存。
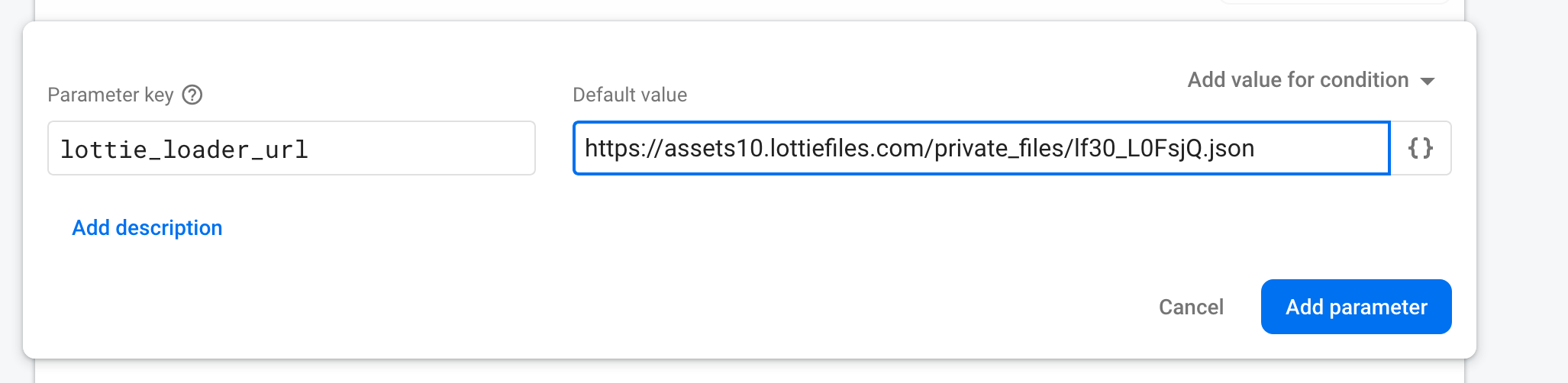
Note: We will also keep on default fail safe loader inside the project just in case if download fails or downloading is in progress from Lottie so the default one works till that time.
注意 :我们还将在项目内部保留默认的故障安全加载程序,以防万一如果下载失败或正在从Lottie下载正在进行中,那么默认的安全加载程序将一直工作到那时。
用户默认值 (UserDefaults)
- We will be needing some sort of storage to save our loader in the app after downloading, In this tutorial we will tak UserDefaults help. 下载后,我们将需要某种存储方式以将加载程序保存在应用程序中。在本教程中,我们将向UserDefaults帮助。
- I have shared a gist of UserDefaults with propertywrapper which is most of inspired from John Sundell tutorial. 我与propertywrapper共享了UserDefaults的要旨,这主要是由John Sundell教程启发而来的。
- This code helps us to save custom Codable object in the UserDefaults. If you have look the Lottie file code it is mostly JSON code, The animation rule is written in JSON and Lottie framework plays it using that JSON file 此代码有助于我们将自定义的Codable对象保存在UserDefaults中。 如果您看过Lottie文件代码,它主要是JSON代码,那么动画规则是用JSON编写的,Lottie框架使用该JSON文件对其进行播放
- So this is reason we need custom UserDefaults wrapper to save our Lottie JSON file 所以这就是为什么我们需要自定义UserDefaults包装器来保存我们的Lottie JSON文件的原因
乐天模块代码 (Lottie Module Code)
I will not cover the part on how to integrate Lottie in iOS, follow this link
我不会介绍如何在iOS中集成Lottie,请点击此链接
- Lottie iOS framework has inbuilt method to load the file from URL we will we will use that. Lottie iOS框架具有内置方法可以从URL加载文件,我们将使用它。
AnimationView(url: lottieURL,
closure: completion,
animationCache: LRUAnimationCache.sharedCache)
Now here is the catch, Inside the Lottie iOS framework they use
LRUAnimationCache
which store the animation file only for that particular app session, If you kill the app it download again.现在要注意的是,在Lottie iOS框架内,他们使用
LRUAnimationCache
来存储仅用于特定应用会话的动画文件,如果您杀死该应用,则会再次下载。When you deep dive
LRUAnimationCache
code you will see it implementsAnimationCacheProvider
which is custom protocol and inside itLRUAnimationCache
has implemented the all the logic to store the animation file.深入研究
LRUAnimationCache
代码时,您会看到它实现了AnimationCacheProvider
,这是自定义协议,并且在其中,LRUAnimationCache
已经实现了存储动画文件的所有逻辑。Definitely
LRUAnimationCache
will not work for our use case, We will create our own customAnimationCacheProvider
, Below I have shared the gist ofLottieAnimationCache
for your reference.肯定
LRUAnimationCache
在我们的用例中不起作用,我们将创建自己的自定义AnimationCacheProvider
,下面我分享了LottieAnimationCache
的要旨供您参考。- You will see we have used our custom UserDefaults wrapper inside it to store our Lottie JSON Loader for our app. 您将看到我们在其中使用了自定义的UserDefaults包装器来存储我们应用程序的Lottie JSON Loader。
- Now you can create the Loader View with our own cache implementation like below 现在,您可以使用我们自己的缓存实现创建Loader View,如下所示
AnimationView(url: lottieURL,
closure: completion,
animationCache: LottieAnimationCache.sharedCache)
最后一步 (Final Step)
- Now we have setup the core module let’s play with it 现在我们已经设置了核心模块,让我们一起玩吧
We will first create the Lottie
AnimationView
which will load the JSON and add it to the subview, Consider the scenarion you are making network request and you have to show spinner , Instead if spinner you show LottieAnimationView
我们将首先创建Lottie
AnimationView
,它将加载JSON并将其添加到子视图中,考虑正在发出网络请求的方案,并且必须显示spinner,而如果spinner则显示LottieAnimationView
I have written custom method which return
AnimationView
. This method take care of fail safe condition also which we discussed earlier我已经编写了返回
AnimationView
自定义方法。 这种方法还照顾了我们先前讨论的故障安全条件

AnimationView
AnimationView
的代码的屏幕截图
以下是我们将遵循的步骤,如上面的屏幕快照所示 (Following are steps we will follow as shown in above screenshot)
We will first create
AnimationView
instance and load it with default fail safe loader.我们将首先创建
AnimationView
实例,并使用默认的故障安全加载器加载它。- We will first download the Lottie URL from Remote Config. 我们将首先从Remote Config下载Lottie URL。
- We will check if it URL is cache or not 我们将检查网址是否已缓存
If the URL is not cached we will download it and save it in our custom cache which we discussed above and store it in
UserDefaults
and return the default fail safe loader until our loader gets downloaded如果未缓存URL,我们将下载它并将其保存在我们上面讨论的自定义缓存中,并将其存储在
UserDefaults
并返回默认的故障安全加载器,直到下载加载器为止If the URL is cached we will load the
AnimationView
with our Lottie URL and return our Lottie from Remote Config如果URL被缓存,我们将用Lottie URL加载
AnimationView
并从Remote Config返回我们的Lottie。Now with just few line of code we can loader in any part of our app, I have created extension over
UIView
to add it for following example.现在只需几行代码,我们就可以在应用程序的任何部分加载程序,我在
UIView
上创建了扩展,以将其添加到以下示例中。

7. That’s it, We have successfully created our custom Dynamic Loader which we can update anytime over the air without an app update.
7.就是这样,我们已经成功创建了自定义的动态加载程序,无需进行应用程序更新即可随时进行空中更新。
感谢您的阅读 (Thank you for reading)
I hope this article will help you in customizing the spinner in your project
我希望本文能帮助您自定义项目中的微调器
参考文献和良好阅读 (References and Good Read)
John Sundell For UserDefaults
John Sundell对于UserDefaults
lottie 使用