In JavaScript, you can check the equality of any two objects using ==
or ===
. Both the operators check whether the given two objects are equal or not, but in a whole different way.
在JavaScript中,可以使用==
或===
检查任何两个对象的相等性。 两位操作员都检查给定的两个对象是否相等,但检查方式完全不同。
Below, you can see in detail how each operator checks for equality of the given two objects.
在下面,您可以详细了解每个运算符如何检查给定两个对象的相等性。
等于运算符(==) (Equality operator (==))
The equality operator (==
) compares two operands to check whether they are equal or not and returns a Boolean result. It tries to convert the operand(s) before checking the equality if they are of different types.
相等运算符( ==
)比较两个操作数以检查它们是否相等,并返回布尔结果。 如果操作数类型不同,它将尝试在检查相等性之前转换操作数。
您可以在下面看到相等运算符( == )如何尝试比较两个操作数。 (You can see below how the equality operator (==) tries to compare the two operands.)
不同种类 (Different types)
- If you compare a string and a number, it tries to convert the string to a number before comparison. 如果您比较字符串和数字,则它会在比较之前尝试将字符串转换为数字。
When one operand is a boolean and another is a number, it tries to convert the boolean to a string, so
true
is converted to1
, andfalse
converts to0
.当一个操作数是布尔值而另一个是数字时,它将尝试将布尔值转换为字符串,因此将
true
转换为1
,将false
转换为0
。
When one of the operands is an object, and the other is a string or a number then, it tries to convert the object to a primitive type using either
valueOf()
ortoString()
.当一个操作数是一个对象,而另一个是字符串或数字时,它将尝试使用以下任一方法将该对象转换为原始类型
valueOf()
要么toString()
。
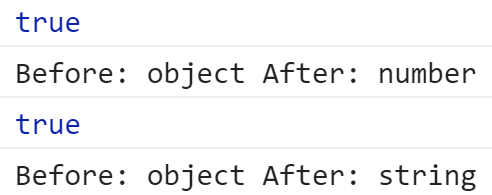
对象 (Objects)
When both the operands are objects, the result would be true
only when both the operands refer to the same object.
当两个操作数都是对象时,仅当两个操作数都引用相同的对象时,结果才为true
。
空和未定义 (null and undefined)
When one operand is null
, and the other is undefined
, it returns true
.
当一个操作数为null
且另一个操作数undefined
,它返回true
。
Note: The
null
is an assigned value, but it means empty or non-existing. Andundefined
means that the variable is declared but is not assigned with any value. So, in case of checking the equality, they both sum up as not referring to any value.注意:
null
是分配的值,但是它表示为空或不存在。undefined
表示变量已声明,但未分配任何值。 因此,在检查相等性的情况下,它们两者都总结为未引用任何值。
其他情况 (Other cases)
For all the other scenarios, the comparison is as follows.
对于所有其他方案,比较如下。
Strings are only equal when the characters and their sequence are the same in both the operands.
仅当两个操作数中的字符及其顺序相同时,字符串才相等。
Numbers are equal if they are of the same value, except in the case of
+0
and-0
, they are considered equal. If either of the operands isNaN
then it returnsfalse
.如果数字具有相同的值,则数字相等,但
+0
和-0
除外,它们被视为相等。 如果两个操作数中的任何一个为NaN
则返回false
。
Boolean values are equal only if both the operands are either
true
orfalse
.布尔值 仅当两个操作数均为
true
或false
时才相等。
严格相等运算符(===) (Strict Equality operator (===))
The strict equality operator compares two operands to check whether they are equal or not and returns a Boolean result. It considers the operands of different types to be different and doesn’t try to convert them to a common type before comparison.
严格相等运算符比较两个操作数以检查它们是否相等,并返回布尔结果。 它认为不同类型的操作数是不同的,并且在比较之前不会尝试将它们转换为通用类型。
您可以在下面看到严格相等运算符(===)如何比较两个操作数。 (You can see below how the strict equality operator (===) compares the two operands.)
不同种类 (Different types)
When both operands are of different types, it returns false
.
当两个操作数的类型不同时,它返回false
。
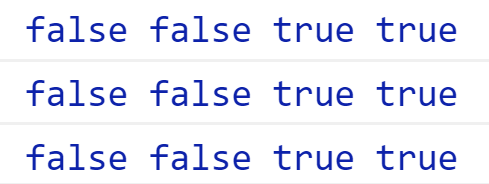
对象 (Objects)
When both the operands are objects, it returns true
only when both the operands refer to the same object.
当两个操作数都是对象时,仅当两个操作数都引用相同的对象时,它才返回true
。

空或未定义 (null or undefined)
When both operands are either null
or undefined
, it returns true
.
当两个操作数均为null
或undefined
,它将返回true
。

号码 (Numbers)
When either of the operands is NaN
in case of a numeric comparison, it returns false
.
NaN
数字比较的情况下,如果两个操作数中的任何一个为NaN
,则返回false
。

其他情况 (Other cases)
In all the other scenarios, the comparison is as follows.
在所有其他情况下,比较如下。
Numbers are equal if they have the same numeric value, except in the case of
+0
and-0
, they are equal.如果数字具有相同的数值,则数字相等,但
+0
和-0
除外。

Strings are equal when both operands have the same characters in the same sequence.
当两个操作数具有相同序列的相同字符时,字符串相等。

Boolean values are equal when both operands are either
true
orfalse
.当两个操作数均为
true
或false
时,布尔值相等。

结论 (Conclusion)
Let’s summarize what you have learned in this article:
让我们总结一下您在本文中学到的知识:
- As you have seen how the equality operator works, you need to be careful while using it, because it tries to convert the values to the same datatype before it checks for equality. 正如您所看到的相等运算符如何工作一样,在使用它时需要小心,因为它会在检查相等之前尝试将值转换为相同的数据类型。
- Using an equality operator may not lead to the results that you are expecting in some cases, which can cause unexpected problems in the functionality of your code. 在某些情况下,使用相等运算符可能不会导致您期望的结果,这可能会导致代码功能出现意外问题。
- The strict equality checks if the given objects are equal or not, by considering both their value and type. 严格相等性通过同时考虑它们的值和类型来检查给定对象是否相等。
- In the case of strict equality, you can be sure that it checks if the given two operands are equal or not without performing any background conversions. 在严格相等的情况下,可以确保它在不执行任何后台转换的情况下检查给定的两个操作数是否相等。
- So, if you need to check for equality the right way by taking into account both the value and the type of the operand, then using strict equality is the way to go. 因此,如果您需要通过同时考虑操作数的值和类型来以正确的方式检查相等性,那么使用严格的相等性是可行的方法。
The case when you can use the equality operator without any doubt is when you are checking equality of
null
andundefined
.毫无疑问,可以使用相等运算符的情况是在检查
null
和undefined
相等性时。
资源资源 (Resources)
Equality in MDN web docs
Strict Equality in MDN web docs
MDN网络文档中的严格平等
Comparison and Equality in MDN web docs
MDN Web文档中的比较和平等
Thanks for reading and happy learning!
感谢您的阅读和学习愉快!
普通英语JavaScript (JavaScript In Plain English)
Enjoyed this article? If so, get more similar content by subscribing to Decoded, our YouTube channel!
喜欢这篇文章吗? 如果是这样,请订阅我们的YouTube频道解码,以获得更多类似的内容!