人工智能python初学
In this article, we’ll look at an easy method to get started with Face Detection using Python and OpenCV. OpenCV is the most popular library for computer vision. Originally written in C/C++, it provides bindings for Python. Machine Learning Algorithm is used in OpenCV for searching faces within pictures. Because faces are all seen as complex, they cannot be determined by a simple test. The algorithms break the task of identifying the face into thousands of smaller, bite-sized tasks, each of which is easy to solve. These tasks are also called Classifiers.
在本文中,我们将介绍一种使用Python和OpenCV进行人脸检测的简便方法。 OpenCV是最受欢迎的计算机视觉库。 最初是用C / C ++编写的,它为Python提供了绑定。 OpenCV使用机器学习算法搜索图片中的人脸。 由于人脸都是复杂的,因此无法通过简单的测试来确定。 该算法将识别面部的任务分解为成千上万个较小的,一口大小的任务,每个任务都易于解决。 这些任务也称为C lassifiers 。
First we need to install openCV in our computer. So, we need to write that below code in command line
首先,我们需要在计算机中安装openCV。 因此,我们需要在命令行下面的代码中编写该代码
pip install python-opencv
Then we need to import that(openCV) library in our code. That library is called that cv2. it means openCV version 2.
然后,我们需要在代码中导入that(openCV)库。 该库称为cv2。 这意味着openCV版本2 。
import cv2
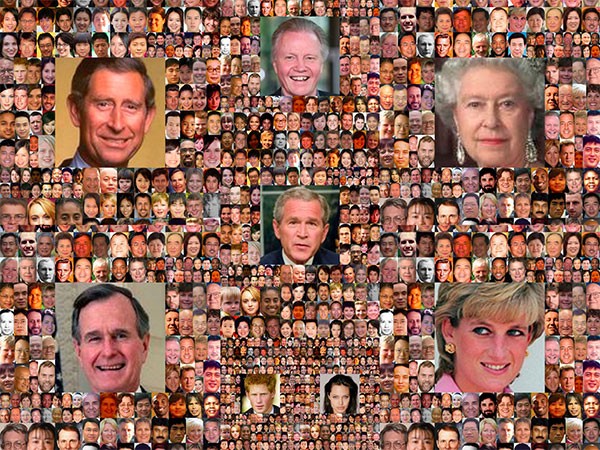
Then we need to download pre-trained data. openCV provides bunch of data and they actually trained bunch of face images. These data is actually trained on bunch of faces.
然后,我们需要下载预先训练的数据。 openCV提供了一堆数据,他们实际上训练了一堆人脸图像。 这些数据实际上是在一堆面Kong上训练的。
we need to download below marked(haarcascade_frontalface_default.xml) file. Put the downloaded file in the same working directory.
我们需要在下面下载标记的(haarcascade_frontalface_default.xml)文件。 将下载的文件放在相同的工作目录中。
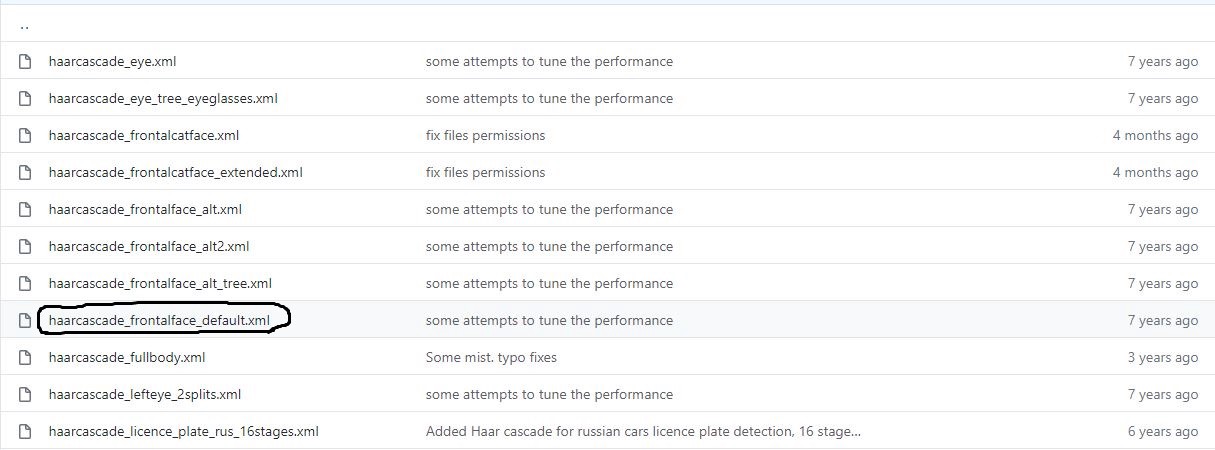
Then we can go and import it in our code. I assign the downloaded file to a variable called face_cascade. Here we call the opencv library(cv2) and call the function called CascadeClassifier() to classify the faces with the help of Cascade algorithm.
然后,我们可以将其导入我们的代码中。 我将下载的文件分配给一个名为face_cascade的变量。 在这里,我们调用opencv库(cv2)并调用称为CascadeClassifier()的函数,以借助Cascade算法对人脸进行分类。
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + ‘haarcascade_frontalface_default.xml’)
Then we need to pass the input image. Here we using the image read function called imread() from openCV. Image is just an array like a big matrix of a bunch of numbers, here pixels are just numbers. So, here we reading the image into big two dimensional array.
然后,我们需要传递输入图像。 在这里,我们使用来自openCV的图像读取函数imread()。 图像只是一个数组,就像一堆数字组成的大矩阵一样,这里的像素就是数字。 因此,这里我们将图像读取为二维大数组。
img = cv2.imread(‘img.jpg’)
Then we need to turn the picture into black and white. The only way that algorithm knows, how to look at a face is in black and white. Because, It is easier to work with one number in each pixels, on a range from black and white. If it is a coloured picture(RGB) then it has 3 numbers in each pixels. so it may take some some time and may be less accurate to process.
然后我们需要将图片变成黑白。 该算法知道的唯一方法是如何以黑色和白色进行注视。 因为,在黑白范围内,每个像素使用一个数字更容易。 如果是彩色图片(RGB),则每个像素中有3个数字。 因此可能会花费一些时间,并且处理的准确性可能会降低。
grayscaled_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
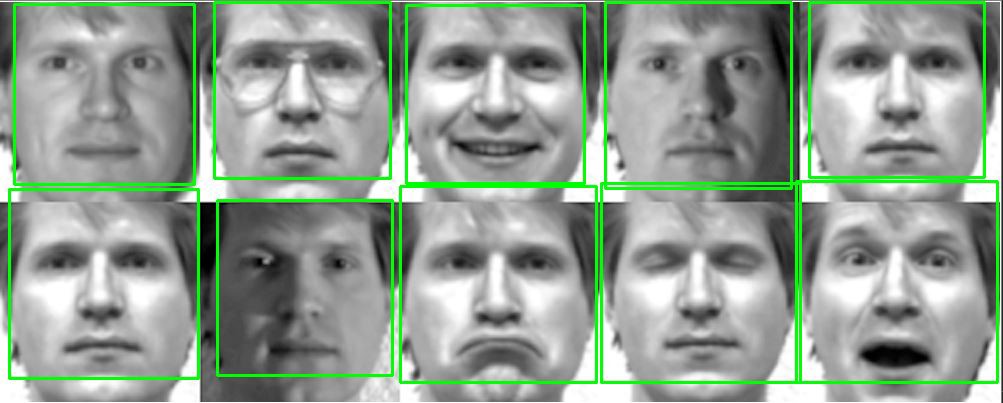
Next, we want to detect faces in the input image. Before, we created a classifier called face_cascade. From there, we’re gonna call a function called detectMultiScale(), this means, no matter the scale of the face whether it is small or big it’s just looking for the overall composition like the relations of eyes to the nose to the mouth whether it’s smaller up close or if there’s multiple of them then it just wants to detect all of them. Detected faces are returned as a list of rectangles. So it just returned the coordinates of those green rectangles
接下来,我们要检测输入图像中的面部。 之前,我们创建了一个名为face_cascade的分类器。 从那里,我们将调用一个名为detectMultiScale()的函数,这意味着,无论脸部的大小是小是大,它都只是在寻找整体构图,例如眼睛,鼻子与嘴巴之间的关系。它会近距离变小,或者如果有多个,则只想检测所有它们。 检测到的面部将作为矩形列表返回。 所以它只是返回了这些绿色矩形的坐标
face_coordinates= face_cascade.detectMultiScale(grayscaled_img)
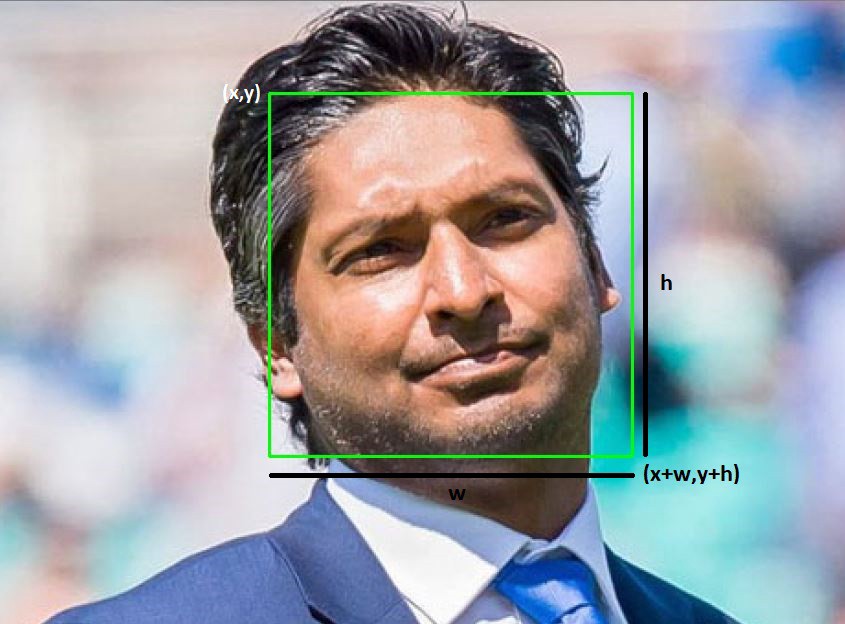
Once we have those coordinates, we are able to draw those rectangles on our image. If a lot of faces are found in our image, the coordinates of each face will be calculated using the for loop. The rectangle() function has 5 parameters. First one is the input of the image and the second parameter is the top left coordinate of the rectangle and the next parameter describes about the bottom right coordinate of the rectangle. The 4th parameter gives the colour of the rectangle and the final parameter describes the thickness of the circle.
一旦有了这些坐标,就可以在图像上绘制这些矩形。 如果在我们的图像中发现很多人脸,则将使用for循环计算每个人脸的坐标。 矩形函数有5个参数。 第一个参数是图像的输入,第二个参数是矩形的左上角坐标,下一个参数描述了矩形的右下角坐标。 第四个参数给出矩形的颜色,最后一个参数描述圆的粗细。
for (x,y,w,h) in face_coordinates:
cv2.rectangle(img, (x,y),(x+w,y+h),(0,255,0),2)
Finally we need to open the detected photo.
最后,我们需要打开检测到的照片。
cv2.imshow(‘Face detecter’,img)
cv2.waitKey()#ENTIRE CODE
import cv2#Load some pre-trained data on face frontals from opencv (haar cascade algorithm)face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')#choose an image to detect faces inimg = cv2.imread('av.jpg')#Must convert to grayscalegrayscaled_img = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)#Detect facesface_coordinates= face_cascade.detectMultiScale(grayscaled_img)#Draw rectangles around the facefor (x,y,w,h) in face_coordinates: cv2.rectangle(img, (x,y),(x+w,y+h),(0,255,0),2)cv2.imshow('Face detecter',img)cv2.waitKey()
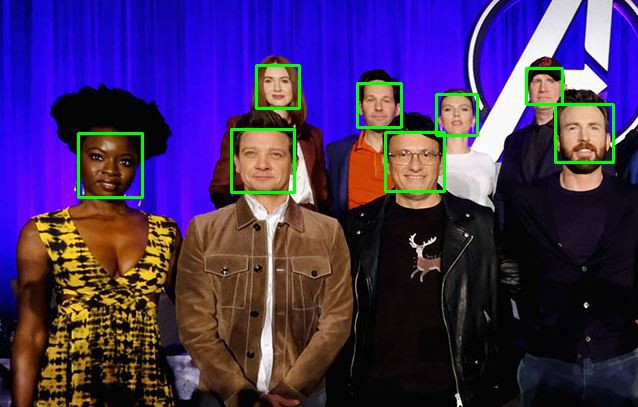
Just 10 lines of code…
仅10行代码…
翻译自: https://medium.com/@wdeva22/real-time-ai-face-detection-with-python-for-beginners-a2a097636205
人工智能python初学