How to Access Recycle-bin with Python, and get rid of the hassle for viewing deleted files directly.
如何使用Python访问回收站,以及摆脱直接查看已删除文件的麻烦。
All of our deleted files and folders move to Recycle-Bin temporarily, before they vanish from existence permanently. But have you ever deleted an important file or a folder by mistake and by the moment you realized it, the name of file is long gone from your memory. Traditional Recycle-Bin that comes with our very own Windows or any other OS, only shows you the list of files and folders that you’ve deleted, along with the location from where it was deleted, as a reference but it doesn’t allow you to open any file or folder unless you restore it. Too much hassle just to find one single file, Right?
我们所有已删除的文件和文件夹在永久消失之前会暂时移至回收站。 但是您是否曾经错误地删除过重要文件或文件夹,而当您意识到这一点时,文件名早已从您的记忆中消失了。 我们自己的Windows或任何其他OS附带的传统回收站,仅向您显示已删除的文件和文件夹的列表以及删除位置,作为参考,但不允许您可以打开任何文件或文件夹,除非您将其还原。 只是查找一个文件太麻烦了,对吧?

There’s a library in Python, with which you can access the Recycle-Bin and its items easily, through which you can code along to make the right program to find your deleted important file without that much of a hassle.
Python中有一个库,您可以通过该库轻松访问回收站及其项目,通过该库,您可以一起编写正确的程序来查找已删除的重要文件,而不会造成任何麻烦。
Start by installing the library “winshell”:
首先安装“ winshell ”库:
pip install winshell
Winshell library has a module named “recycle_bin()” , which returns a list of objects of recycle-bin items.
Winshell库具有一个名为“ recycle_bin() ”的模块,该模块返回回收站项目的对象列表。
In order to print the list of items present in recycle-bin, the code below can be used.
为了打印回收站中存在的项目列表,可以使用以下代码。
In the above code, the list of objects of recycle-bin items is being iterated over a loop inside the enumerate function.
在上面的代码中,循环枚举项目的对象列表通过枚举函数内部的循环进行迭代。
enumerate() is a python built-in function that returns 2 parameters i.e index and value of a list.
enumerate()是python的内置函数,它返回2个参数,即列表的索引和值。
Inside the loop the original_filename() method is called of every object coming form the list.
在循环内部,将对列表中的每个对象调用original_filename()方法。
Original_filename() method returns the path of the file/folder from which it was deleted.
Original_filename()方法返回从中删除文件/文件夹的路径。
Now comes the part of viewing the file or a folder right from the Recycle-bin.
现在是直接从回收站中查看文件或文件夹的部分。
In order to do this, the index of that file/folder should be noted first. Store its object inside a variable by the index and then undelete it with winshell.undelete() method. Once the file/folder is undeleted, Read its content by using its path, and after that delete it again immediately with winshell.delete_file() .
为此,应首先记录该文件/文件夹的索引。 通过索引将其对象存储在变量中,然后使用winshell.undelete()方法取消删除它。 取消删除文件/文件夹后,使用其路径读取其内容,然后使用winshell.delete_file()立即将其删除。
Accessing File:
访问文件:
In the above code:
在上面的代码中:
The deleted item object of a file was accessed by index and into variable “obj”.
文件的已删除项目对象通过索引访问,并进入变量“ obj ”。
- Since Python accepts path containing “\\” in it and obj.original_filename() return path with “\”. So that in the properPath variable, path containing the “\\” is saved. 由于Python接受其中包含“ \\”的路径,而obj.original_filename()返回带有“ \”的路径。 这样就可以在propertyPath变量中保存包含“ \\”的路径。
The selected file is undeleted by winshell.undelete() method, providing the path from obj.original_filename() method [winshell library methods accepts and returns the path containing “\” that’s why the path was given by the original_filename() method].
所选文件将由winshell.undelete()方法取消删除,并提供obj.original_filename()方法的路径[ winshell 库方法接受并返回包含“ \”的路径,这就是该路径由original_filename()方法提供的原因] 。
- Reading the content of file by opening the file with open() function with “r” as a parameter for reading purpose, and then file.read() is used to save all the content inside the file, then closed the file with file.close(). 通过使用open()函数以“ r”作为参数读取文件来打开文件来读取文件内容,然后使用file.read()将所有内容保存在文件内部,然后使用file关闭文件。关()。
- Once after the content is read successfully, the file is deleted again with winshell.delete_file() method by giving it path by obj.original_fileneme() method. 成功读取内容后,将使用obj.original_fileneme()方法为其提供路径,并使用winshell.delete_file()方法再次删除该文件。
Accessing Folder:
访问文件夹:
In the above code:
在上面的代码中:
- The deleted item object of a folder was accessed by index and into variable “obj”. 文件夹的已删除项目对象已通过索引访问,并进入变量“ obj”。
- The “\” path was updated to “\\” path in properPath variable. “ \”路径已在propertyPath变量中更新为“ \\”路径。
- The selected file is undeleted by winshell.undelete() method, providing the path from obj.original_filename() method. 所选文件通过winshell.undelete()方法取消删除,并提供obj.original_filename()方法的路径。
- Reading list of file/folders inside our selected folder by using os.listdir method in which the “\\” path is given as a parameter from the properPath variable. 使用os.listdir方法读取所选文件夹中的文件/文件夹列表,其中“ \\”路径作为来自正确路径变量的参数给出。
os.listdir() method returns the list of files/folders , of the folder given as parameter.
os.listdir()方法返回以参数形式给出的文件夹的文件/文件夹列表。
- Once after the content is read successfully, the folder is deleted again with winshell.delete_file() method by giving it path by obj.original_fileneme() method. 成功读取内容后,将使用obj.original_fileneme()方法为其指定路径,再次使用winshell.delete_file()方法将该文件夹删除。
This way we can view the content of all files/folders inside the recycle-bin without that much of a hassle.
这样,我们就可以轻松地查看回收站中所有文件/文件夹的内容。
You can now be sure of which file/folder to keep, and which to delete permanently , after viewing what’s inside it.
查看其中的文件/文件夹后,现在可以确定要保留哪个文件/文件夹,以及将其永久删除。
[ One thing to keep in mind is that winshell.recycle_bin() returns the list of deleted items and the list get shuffled on every execution. Means that your file/folder may be at index 3, but when you’ll re-run the program/code it may got to index 5 now ]
[要记住的一件事是winshell.recycle_bin()返回已删除项目的列表,并且该列表在每次执行时都会被重新排列。 意味着您的文件/文件夹可能在索引3处,但是当您重新运行程序/代码时,它可能现在在索引5处]
This was all about Accessing Recycle-Bin and deleted items in Python.
这全部与访问Python中的回收站和已删除项目有关。
There’s a whole program coded on Command line interface in the repository below.
在下面的存储库中,有一个完整的程序在命令行界面上编码。
In order to access the CLI program , follow the steps below.
为了访问CLI程序,请按照以下步骤操作。
Start by cloning this repository.
首先克隆此存储库。
$ git clone https://github.com/shahzaibk23/Trash-Visualiser
Once after the cloning is successful, cd into repo folder.
克隆成功后,将CD放入repo文件夹。
cd Trash-Visualiser/src/CLI/
Then:
然后:
python main.py

There’s also a GUI based version available, with which you can get out this hassle in a better visual representation of everything, found in the same repository.
还有一个基于GUI的版本,使用它可以更好地直观显示所有内容,而这些麻烦可以在同一存储库中找到。
In order to run it , follow the steps below:
为了运行它,请按照以下步骤操作:
Start by cloning the Repository
首先克隆存储库
$ git clone https://github.com/shahzaibk23/Trash-Visualiser
After cloning is successful, cd into repo folder
克隆成功后,将CD放入repo文件夹
cd Trash-Visualiser
Then run the launcher
然后运行启动器
python launcher.py
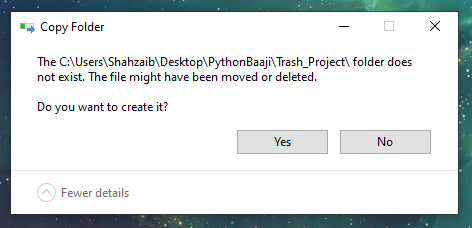
This may happen that the windows will ask you to create some deleted folders once again, so simply click YES, this way the folders you’d have deleted will be created once again temporarily, but they will be deleted automatically, so no need to Panic.
可能会发生这种情况,Windows会要求您再次创建一些已删除的文件夹,因此只需单击“是”,这样就可以暂时再次创建要删除的文件夹,但它们会自动删除,因此无需恐慌。
After a few seconds of processing, Trash-Visualiser main window will be opened.
经过几秒钟的处理后,将打开Trash-Visualiser主窗口。

From this GUI version you can view files and folders inside recycle-bin directly within the GUI. You can also view the files/folders inside the deleted folder as well. File/Folders inside the recycle-bin can be Restores and Deleted permanently with a single button click from the GUI.
从此GUI版本,您可以直接在GUI中查看回收站中的文件和文件夹。 您也可以查看已删除文件夹中的文件/文件夹。 只需单击GUI的一个按钮,就可以永久地恢复和删除回收站中的文件/文件夹。
More detailed information is found in the Repository of Trash-Visualiser.
可在“垃圾桶可视化工具”存储库中找到更多详细信息。

This was all about minimizing the Hassle of Viewing Files/Folders from the Recycle-Bin without restoring at all, simply and efficiently.
这全部是为了最大程度地减少从回收站查看文件/文件夹的麻烦,而又无需简单,有效地进行任何还原。
翻译自: https://medium.com/swlh/how-to-access-recycle-bin-in-python-192a685e31fb