python字符串去掉空行
如何在python中删除字符串中的空格 (How to remove whitespaces in a string in python)
- str.lstrip() str.lstrip()
- str.rstrip() str.rstrip()
- str.strip() str.strip()
- str.replace() str.replace()
- str.split() str.split()
- re.sub() re.sub()
By using the above-mentioned methods, let's see how to remove whitespaces in a string.
通过使用上述方法,让我们看看如何删除字符串中的空格。
Topic covered in this story
这个故事涉及的主题
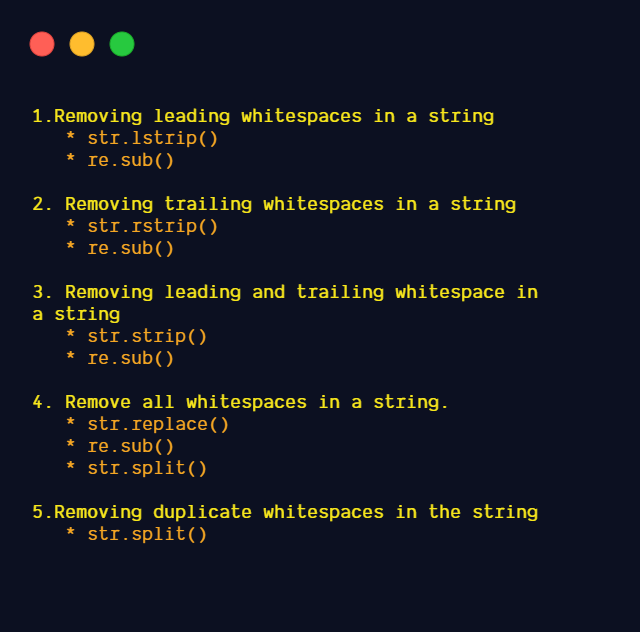
1.删除字符串中的前导空格 (1. Removing leading whitespaces in a string)
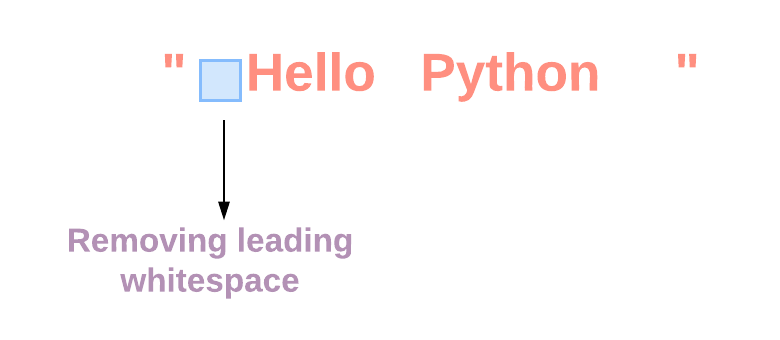
1. str.lstrip()Return a copy of the string with leading whitespaces removed.
1. str.lstrip()返回删除前导空格的字符串副本。
s=" Hello Python "print (s.lstrip())#Output:Hello Python
2. re.sub()Using re.sub(), we can remove leading whitespaces.
2. re.sub()使用re.sub(),我们可以删除前导空格。
Syntax:
句法:
re.sub(pattern, repl, string, count=0, flags=0)
re. sub ( pattern , repl , string , count=0 , flags=0 )
pattern - specify the pattern to be replacedrepl - replacing the leftmost non-overlapping occurrences of pattern in string by the replacement repl. count - The optional argument count is the maximum number of pattern occurrences to be replaced. If omitted or zero, all occurrences will be replaced.
图案 -指定图案被替换REPL -由替换REPL替换模式的最左边的非重叠出现在字符串 。 count-可选参数count是要替换的最大模式出现次数。 如果省略或为零,将替换所有出现的事件。
pattern= r”^\s+”
pattern= r”^\s+”
r
Python’s raw string notation for regular expression\s
matches whitespace characters.+
It will match one or more repetitions of whitespace character.^
(Caret.) Matches the start of the string
r
Python的正则表达式\s
的原始字符串表示法与空格字符匹配。 +
它将匹配一个或多个空白字符的重复。 ^
(Caret。)匹配字符串的开头
pattern mentioned is whitespace at the beginning of the string.s1=re.sub(pattern,””,s)
提到的模式是字符串开头的空格。 s1=re.sub(pattern, ”” ,s)
re.sub() will replace the pattern (whitespaces at the beginning of string) to empty string.
re.sub()会将模式(字符串开头的空格)替换为空字符串。
s=" Hello Python "
import re
pattern= r"^\s+"s1=re.sub(pattern,"",s)
print (s1)#Output:Hello Python
2.删除字符串中的尾随空格 (2. Removing trailing whitespace in a string)
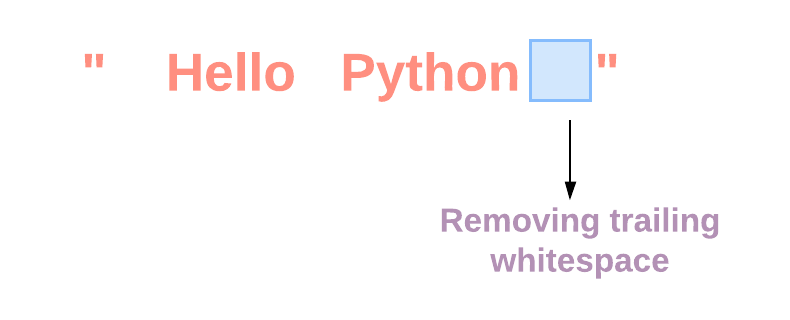
str.rstrip()
str.rstrip()
Return a copy of the string with trailing whitespaces removed.
返回已删除尾随空格的字符串副本。
s=" Hello Python "print (s.rstrip())#Output: Hello Python
re.sub()Using re.sub(), we can remove trailing whitespaces.
re.sub()使用re.sub(),我们可以删除结尾的空格。
pattern= r"\s+$"
r
-Python’s raw string notation for regular expression\s
— matches whitespace characters.+
It will match one or more repetitions of whitespace character.$
Matches the end of the string
r
正则表达式\s
Python原始字符串表示法-匹配空白字符。 +
它将匹配一个或多个空白字符的重复。 $
匹配字符串的结尾
pattern mentioned are whitespaces at the end of the string.s1=re.sub(pattern,””,s)
提到的模式是字符串末尾的空格。 s1=re.sub(pattern, ”” ,s)
re.sub() will replace the pattern (whitespaces at the end of the string) to empty string.
re.sub()会将模式(字符串末尾的空格)替换为空字符串。
s=" Hello Python "
import re
pattern= r"\s+$"s1=re.sub(pattern,"",s)
print (s1)#Output:Hello Python
3.删除字符串中的前导和尾随空格 (3. Removing leading and trailing whitespace in a string)
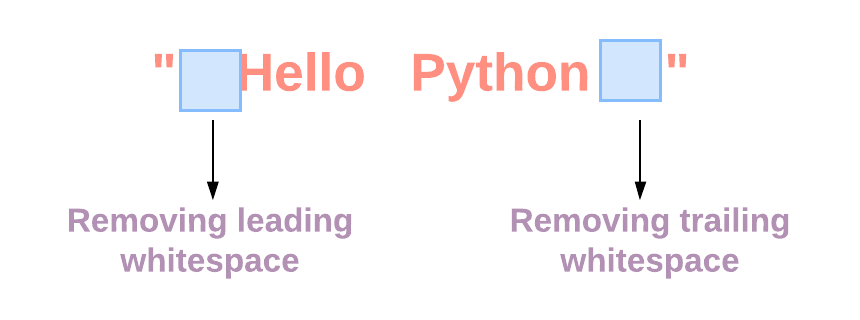
str.strip()
str.strip()
Return the copy of the string with leading whitespaces and trailing whitespaces removed.
返回删除前导空格和尾随空格的字符串副本。
s=" Hello Python "print(s.strip())#Output:Hello Python
re.sub()
re.sub()
Using re.sub(), we can remove leading whitespaces and trailing whitespaces.pattern="^\s+|\s+$"
使用re.sub(),我们可以删除前导空格和尾随空格。 pattern= "^\s+|\s+$"
^\s+
Matches one or more whitespaces at the beginning of the string
^\s+
匹配字符串开头的一个或多个空格
\s+$
Matches one or more whitespaces at the end of the string.
\s+$
匹配字符串末尾的一个或多个空格。
|
x|y matches either x or y
|
x | y匹配x或y
s=" Hello Python "
import re
pattern="^\s+|\s+$"s1 = re.sub(pattern, "",s)
print(s1)#Output:Hello Python
4.删除字符串中的所有空格。 (4. Remove all whitespaces in a string.)
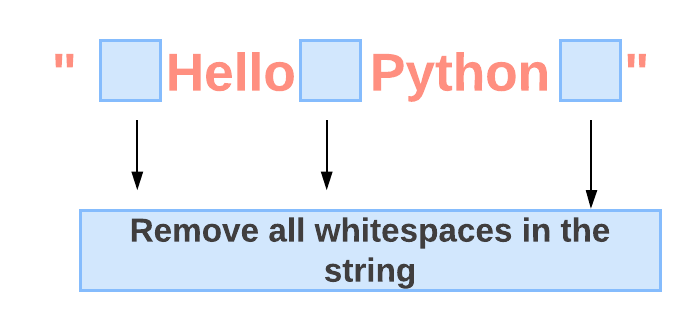
str.replace()Return a copy of the string with all occurrences of substring old replaced by new.
str.replace()返回该字符串的副本,其中所有出现的子字符串old都替换为new。
Syntax:
句法:
str.replace(old,new,count)
str.replace(old,new,count)
print (s.replace(" ",""))
It will replace all whitespace characters into an empty string.
它将所有空白字符替换为空字符串。
s=" Hello Python "print (s.replace(" ",""))#Output:HelloPython
re.sub()Using re.sub(), we can remove all whitespaces in the string.
re.sub()使用re.sub(),我们可以删除字符串中的所有空格。
pattern=r"\s+"s1 = re.sub(pattern, "", s)
pattern
matches all whitespaces in the string. Then re.sub(), replacing pattern(whitespaces ) to empty string.
pattern
匹配字符串中的所有空格。 然后re.sub(),将pattern(whitespaces)替换为空字符串。
s=" Hello Python "
import re
pattern=r"\s+"s1 = re.sub(pattern, "", s)
print (s1)#Output:HelloPython
str.split() Returns list of all words in the string separated using delimiter string. If the delimiter is not mentioned, by default whitespace is the delimiter.
str.split()返回使用定界符字符串分隔的字符串中所有单词的列表。 如果未提及定界符,则默认情况下空白为定界符。
join() — join () method takes all items in the iterable and combines them into a string using a separator
join() — join()方法将所有可迭代项都包含在内,并使用分隔符将它们组合成字符串
s1="".join(s.split())
Splitting the string and joining the elements using an empty string
分割字符串并使用空字符串连接元素
s=" Hello Python "print (s.split())#Output:['Hello', 'Python']
#joining the elements in the string using empty string.s1="".join(s.split())
print (s1)#Output:HelloPython
5.删除字符串中的重复空格 (5. Removing duplicate whitespaces in the string)
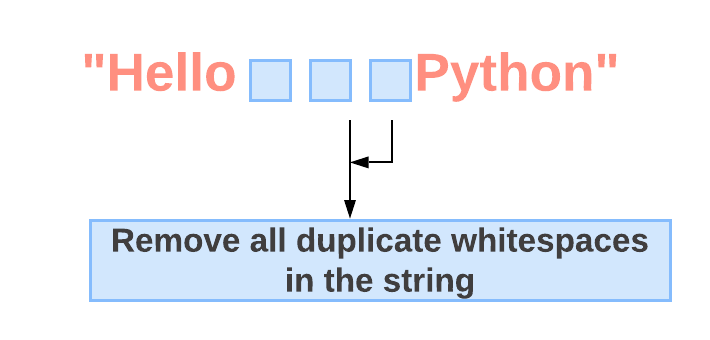
str.split() Returns list of all words in the string separated using delimiter string. If the delimiter is not mentioned, by default whitespace is the delimiter.
str.split()返回使用定界符字符串分隔的字符串中所有单词的列表。 如果未提及定界符,则默认情况下空白为定界符。
join() — join () method takes all items in the iterable and combines them into a string using a separator
join() — join()方法将所有可迭代项都包含在内,并使用分隔符将它们组合成字符串
s1=" ".join(s.split())
Splitting the string and joining the elements using a single whitespace string
使用单个空格字符串分割字符串并连接元素
s="Hello Python"print (s.split())#Output:['Hello', 'Python']
#joining the elements in the string using single whitespace string.s1=" ".join(s.split())
print (s1)#Output:Hello Python
翻译自: https://levelup.gitconnected.com/remove-whitespaces-from-strings-in-python-c5ee612ee9dc
python字符串去掉空行