重构 灾难
Lately, I’ve been coding with Python and building my own small programs and utilities. After building a successful simple timer and calculator I got to wondering, “is my code in the simplest most efficient state it can be?”. How does it translate when being read or used by other people? Does it perform well?
最近,我一直在使用Python进行编码,并构建自己的小型程序和实用程序。 构建成功的简单计时器和计算器之后,我想知道:“我的代码是否处于最简单,最有效的状态?”。 当被他人阅读或使用时,它如何翻译? 效果好吗?
But what is Refactoring? Refactoring is the process of making your code easier to read and maintain usually without changing the runtime behavior of the program.
但是什么是重构? 重构是使代码在不更改程序的运行时行为的情况下通常更易于阅读和维护的过程。
“Refactoring is the process of making your code easier to read and maintain without changing the runtime behaviour of the program”. — Joe Marini, Senior Developer at Google
“重构是使您的代码更易于阅读和维护而不改变程序的运行时行为的过程”。 — Google的高级开发人员Joe Marini
In this article, I will go over some of the most common opportunities to refactor your code in the Python language. These include:
在本文中,我将介绍一些最常见的机会来用Python语言重构您的代码。 这些包括:
- Quality of comments 评论质量
- Managing for loops / Enumerate 管理循环/枚举
- Conditional Statements/Expressions 条件语句/表达式
- Deleting unnecessary code 删除不必要的代码
评论 (Commenting)
There is a saying that if a piece of code needs a comment then it’s too complicated! This school of thought believes that the code should tell a story and be identifiable on its own, few comments needed.
俗话说,如果一段代码需要注释,那就太复杂了! 这种思想流派认为,代码应该能够讲述一个故事,并且可以自己识别,几乎不需要注释。
Some programming practices do lean on comments more than others. Data Science projects can benefit more from comments among the code than software development from what I have found. As a Data Scientist, I can speak toward this, you want your methodology to be explainable to a viewer or stakeholder and it is useful to be able to explain what you are doing and what is going on every step of the way.
一些编程实践比其他实践更依赖注释。 数据科学项目可以从代码中的注释中受益,而不是从我发现的软件开发中受益。 作为一名数据科学家,我可以这样说,您希望您的方法可以向查看者或利益相关者解释,并且能够解释您的工作方式以及每一步的进展是很有用的。
Because the ability to communicate your methodology is so valuable and important, code comments are regarded differently in the field of Data Science.
因为交流您的方法论的能力是如此宝贵和重要,所以在数据科学领域,对代码注释的看法有所不同。
Example 1
例子1
# Create empty list
my_list = []# Collection to iterate over
some_iterable = ['Apple', 'Orange', 'Pear', 'Banana', 'Grapes']# loop over some_iterable and add each element to empty list
for i in some_iterable:
my_list.append(i)
The comments above are not adding any value. They can be ditched. Comments should always be adding value.
上面的评论没有增加任何价值。 他们可以被抛弃。 评论应该总是增加价值。
枚举 (Enumerate)
The use of enumerate()
often gets overlooked in the early stages of a python user’s journey. This handy tool handles an index or counter with your for loops in a more performant and elegant way.
enumerate()
的使用通常在python用户使用过程的早期就被忽略了。 这个方便的工具以更高性能和更优雅的方式处理for循环的索引或计数器。
Example 2
例子2
i = 0
for item in my_list:
i += 1
print(f'{i}: {item}')
The code in bold is initialising an index i
and looping over each element in my_list
while incrementing i
by 1. The final output will print:
粗体代码将初始化索引i
并遍历my_list
每个元素,同时将i
递增1。最终输出将输出:
item1
项目1
item2
item2
etc…
等等…
If you ever come across the code in bold in some form or another, know that there is an algorithm/function for this in Python and it is called enumerate.
如果您曾经以某种形式以粗体形式遇到过代码,请知道Python中有用于此的算法/函数,它称为enumerate 。
Using enumerate we can remove the initialisation and need to increment i
:
使用枚举,我们可以删除初始化,而需要增加i
:
for index, item in enumerate(my_list):
print(f'{index}: {item}')
We pass my_list
to enumerate()
and enumerate handles the indexing/counter for us by zipping them together.
我们将my_list
传递给enumerate()
并通过将它们压缩在一起来enumerate()
为我们处理索引/计数器。
All we need to do is ‘destructure’ the zipped index and items by explicitly passing index, item
in the for loop. The output will be the same as above and the performance is quicker.
我们需要做的就是通过显式传递index, item
来“分解”压缩的索引和项目 在for循环中。 输出将与上面相同,并且性能更快。
删除不必要的代码 (Deleting unnecessary code)
Often we find ourselves using extra print statements to visualise if our code is correct. Observe now a similar example to the one above
通常,我们发现自己使用额外的打印语句来可视化我们的代码是否正确。 现在观察与以上示例类似的示例
Example 3
例子3
my_list = []
some_iterable = ['Apple', 'Orange', 'Pear', 'Banana', 'Grapes']
for index, item in enumerate(some_iterable):
print(f'{index}: {item}')
my_list.append(item)
Above you can see that the only place we use our index
is in the print statement. It could be that we added the print statement to visualise how the code was working at one point. If there is no other reason to print the statement, then we can remove it along with the enumerate that is doing nothing else for us:
在上方可以看到,我们使用index
的唯一位置是在print语句中。 可能是我们添加了print语句以可视化代码在某一时刻的工作方式。 如果没有其他原因可以打印该语句,则可以将其与枚举一起删除,而枚举对我们没有任何其他作用:
my_list = []
some_iterable = ['Apple', 'Orange', 'Pear', 'Banana', 'Grapes']for item in some_iterable:
my_list.append(item)
清单理解: (List Comprehensions:)
The above code serves well and it has been refactored a little already but we can do even better.
上面的代码很好用,并且已经进行了一些重构,但是我们可以做得更好。
We can increase the performance of this code by using a list comprehension in place of the for loop. Simultaneously the code becomes much cleaner, neater, and more readable:
我们可以使用列表理解代替for循环来提高此代码的性能。 同时,代码变得更加整洁,整洁并且更具可读性:
some_iterable = ['Apple', 'Orange', 'Pear', 'Banana', 'Grapes']my_list = [item for item in some_iterable]
This may look like magic to you if its the first time you are seeing it. It was for me. A list comprehension does exactly what a for loop would in one line. My way of understanding this is that it ‘comprehends’ the expression on an iterable within a list and in place.
如果您是第一次看到它,这对您来说就像是魔术。 是给我的 列表推导的作用恰恰是for循环在一行中的作用。 我的理解方式是,它“理解”列表中适当位置的可迭代表达式。
The word list comprehension has a mathematical background you can find out more about it here.
结论: (Conclusion:)
New learners can often end up repeating tutorial level code practices because that is what they were first exposed to. Things like initialising an index and then modifying it in a for loop instead of using enumerate()
or using a for loop where you could have used a list comprehension are in the words of Connor Hoekstra the “Initialise then modify antipattern” and that is something you want to avoid.
新学习者经常最终会重复教程级别的代码实践,因为这是他们最初接触到的内容。 用Connor Hoekstra的话说,诸如初始化索引然后在for循环中修改索引,而不是使用enumerate()
或使用for循环(可以使用列表推导)之类的事情,是Connor Hoekstra所说的“初始化然后修改反模式”。你想避免。
“You want to avoid the Initialise Then Modify antipattern”
“你想避开我 nitialise 牛逼母鸡中号 odify反模式”
— Connor Hoekstra
—康纳·霍克斯特拉(Connor Hoekstra)
Using list comprehensions and the above enumerator algorithm in place of other more wordy methods can save you time performance and increase the readability of your code. But what's more, is that you are really tapping into the power of Python as a language and what it offers. Here is a list of inbuilt functions in Python if you want to go deeper.
使用列表推导和上面的枚举器算法代替其他更冗长的方法可以节省您的时间性能并提高代码的可读性。 但是,更重要的是,您确实在利用Python作为一种语言的力量及其提供的功能。 如果想更深入,这里是Python 内置函数的列表 。
Thank you for reading and have a great day!
感谢您的阅读,祝您有美好的一天!
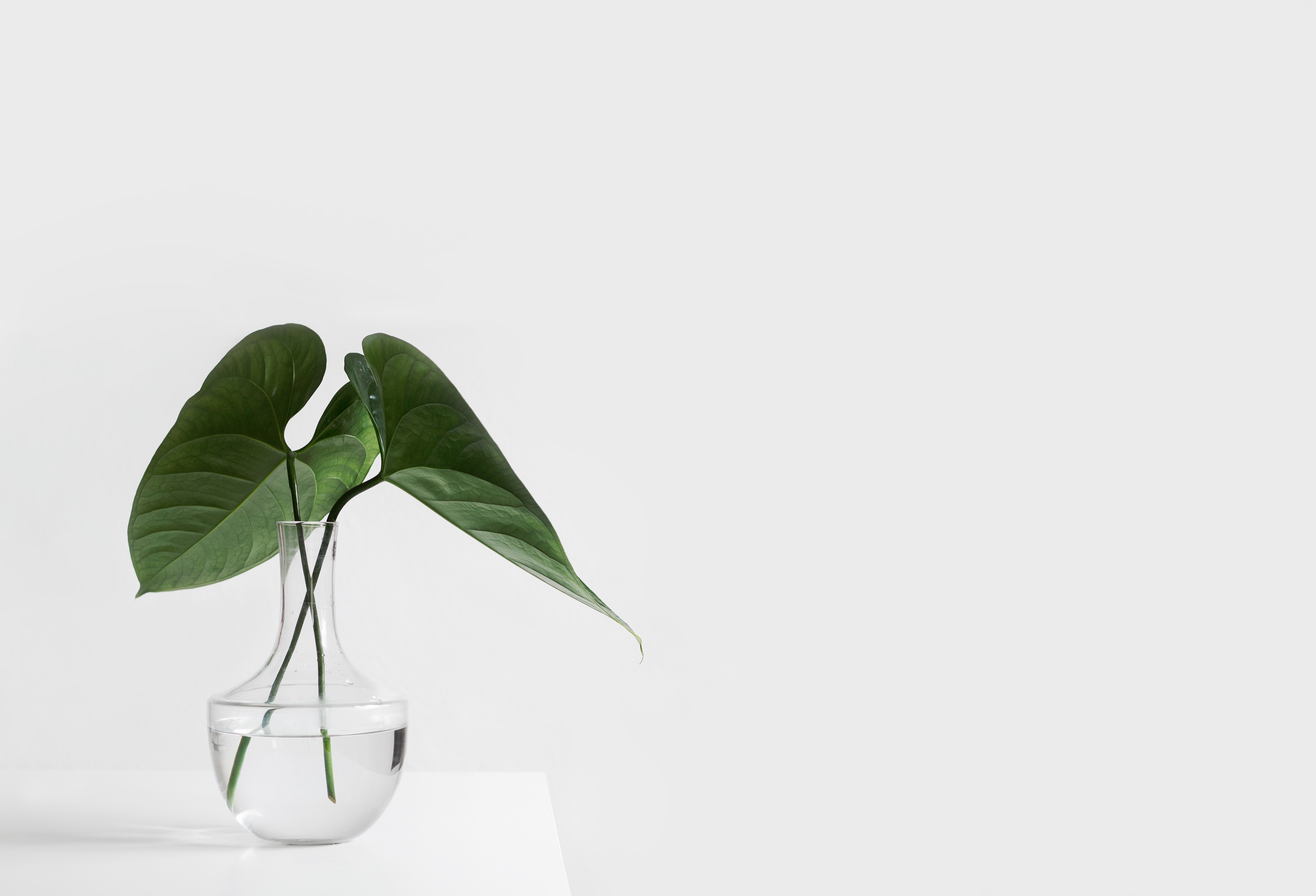
翻译自: https://medium.com/@algakovic/beautiful-pythonic-refactoring-bcfdd8b04240
重构 灾难