按位运算与逻辑运算的区别
At some point in your journey to learn programming and learning about Computer science, you’re bound to come across “bitwise operations”. You may not even get told what it’s called but you may come across an example that looks something like this:
在学习编程和学习计算机科学的过程中,一定会遇到“按位运算”。 您甚至可能不知道它叫什么,但是您可能会遇到一个看起来像这样的示例:
int x = 3 << 2;
int x = 3 << 2;
or maybe you’ll see something like this:
否则您可能会看到类似以下内容:
int y = 64|35;
整数y = 64 | 35;
If you’ve never encountered bitwise operations before, you may see that and think, “What in the world is that?” This article intends to give a very brief introduction to bitwise operations, what they mean and why they are used.
如果你从来没有位操作遇到过,你可以看到,并认为,“在世界上那是什么?” 本文旨在简要介绍按位运算,它们的含义以及使用它们的原因。
都是1和0 (It’s all 1’s and 0's)
To the untrained eye, bitwise operations may seem a bit cryptic…no pun intended. But we have to remember the fact that at the very root of everything, information is stored in computers with 1’s and 0’s. So we will be dealing with binary numbers when it comes to bitwise operations.
在未经训练的人看来,按位操作似乎有点神秘……没有双关语。 但是我们必须记住一个事实,即从根本上讲,信息以1和0的形式存储在计算机中。 因此,当涉及按位运算时,我们将处理二进制数。
在标题中 (It’s in the Title)
That’s right. Bitwise operations refer to dealing with numbers bit by bit. So for example, the number 5 in base 10 is “101” in binary. So using the number 5 in a bitwise operation means we will really look at “101” and apply an operation for each bit (“1”, “0”, and “1”).
那就对了。 按位运算是指逐位处理数字。 因此,例如,以10为底的数字5以二进制形式是“ 101”。 因此,在按位运算中使用数字5意味着我们将真正看到“ 101”并对每个位(“ 1”,“ 0”和“ 1”)应用一个运算。
w ^ HY它们如此重要? (Why are they important?)
Bitwise operations have its uses in cryptography, video games, hash functions. We are essentially manipulating bits, which means that this is applicable to low-level programming. This means that bitwise operations take advantage of a very important benefit that comes from dealing with purely 1’s and 0’s: speed. Now, modern processors are quite quick when it comes to calculating data but older processors are much faster when they use bitwise operations.
按位运算可用于加密,视频游戏,哈希函数。 我们实质上是在操纵位,这意味着它适用于低级编程。 这意味着按位运算利用了一个非常重要的好处,即处理纯1和0带来的好处: speed 。 现在,现代处理器在计算数据方面非常快,而较旧的处理器使用按位运算时则更快。
看运营商 (A Look at the operators)
Bitwise operations are conducted using bitwise operators (surprise, surprise). They are not much different from the logical boolean operators. We have “AND”, “OR”, and “NOT”. Of course, we can combine some of these to describe things like inclusivity or exclusivity, and we will look at these as well. Additionally, we will touch upon manipulating bits through the use of shifting.
使用按位运算符(惊奇,惊喜)进行按位运算。 它们与逻辑布尔运算符没有太大区别。 我们有“ AND”,“ OR”和“ NOT”。 当然,我们可以将其中一些结合起来描述诸如包容性或排他性之类的内容,我们也将对其进行研究。 此外,我们将介绍通过移位来操纵位。
AND运算符 (AND Operator)
For our examples, let’s use the C Programming language. C uses “&&” as the “AND” logical operator. When comparing data, both sides of the AND operator must be true for that part of the operation to be true. Depending on the programming language you are using, the possible values can be “true”/ “false” or “1”/ “0”. In C, our possible values are 1 and 0.
对于我们的示例,让我们使用C编程语言。 C使用“ &&”作为“ AND” 逻辑运算符。 比较数据时,AND运算符的两面都必须为真,以使该部分操作为真。 根据您使用的编程语言,可能的值为“ true” /“ false”或“ 1” /“ 0”。 在C语言中,我们可能的值为1和0。
The logical AND operator in C has two sides and can be written as:
C语言中的逻辑AND运算符具有两个方面,可以写成:
A && B
A && B
This is read as, “A and B”. So what does this mean? Simply that if A is true and B is true, then the expression yields, in C, the value of 1. So if I have something like
读作“ A和B”。 那么这是什么意思? 简单地说,如果A为true,B为true,则表达式在C中得出的值为1。因此,如果我有类似
int x = 10;
整数x = 10;
int y = 4;
整数y = 4;
int z = (x>y) && (x%2==0);
int z =(x> y)&&(x%2 == 0);
printf(“%d\n”, z);
printf(“%d \ n”,z);
The resulting console will print 1:
生成的控制台将打印1:
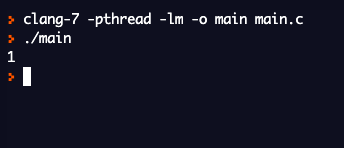
Briefly, going over the code, we have two integers, x and y, and set them equal to 10 and 4, respectively. Then we declare an integer z and set it equal to the result of the expression on the right side, namely,
简要地讲,在代码中,我们有两个整数x和y ,并将它们分别设置为10和4。 然后,我们声明一个整数z并将其设置为等于右侧表达式的结果,即
(x>y) && (x%2==0)
(x> y)&&(x%2 == 0)
This means to check if x is greater than y AND if x/2 has a remainder of 0. Both of these statements are true, so the expression yields a value of 1.
这意味着要检查x是否大于y,以及x / 2是否为0的余数。这两个语句均为true,因此表达式的值为1。